Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Threading / DispatcherProcessingDisabled.cs / 1305600 / DispatcherProcessingDisabled.cs
using System; namespace System.Windows.Threading { ////// A structure that allows for dispatcher processing to be /// enabled after a call to Dispatcher.DisableProcessing. /// public struct DispatcherProcessingDisabled : IDisposable { ////// Reenable processing in the dispatcher. /// public void Dispose() { if(_dispatcher != null) { _dispatcher.VerifyAccess(); _dispatcher._disableProcessingCount--; _dispatcher = null; } } ////// Checks whether this object is equal to another /// DispatcherProcessingDisabled object. /// /// /// Object to compare with. /// ////// Returns true when the object is equal to the specified object, /// and false otherwise. /// public override bool Equals(object obj) { if ((null == obj) || !(obj is DispatcherProcessingDisabled)) return false; return (this._dispatcher == ((DispatcherProcessingDisabled)obj)._dispatcher); } ////// Compute hash code for this object. /// ///A 32-bit signed integer hash code. public override int GetHashCode( ) { return base.GetHashCode(); } ////// Compare two DispatcherProcessingDisabled instances for equality. /// /// /// left operand /// /// /// right operand /// ////// Whether or not two operands are equal. /// public static bool operator ==(DispatcherProcessingDisabled left, DispatcherProcessingDisabled right) { return left.Equals(right); } ////// Compare two DispatcherProcessingDisabled instances for inequality. /// /// /// left operand /// /// /// right operand /// ////// Whether or not two operands are equal. /// public static bool operator !=(DispatcherProcessingDisabled left, DispatcherProcessingDisabled right) { return !(left.Equals(right)); } internal Dispatcher _dispatcher; // set by Dispatcher } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; namespace System.Windows.Threading { ////// A structure that allows for dispatcher processing to be /// enabled after a call to Dispatcher.DisableProcessing. /// public struct DispatcherProcessingDisabled : IDisposable { ////// Reenable processing in the dispatcher. /// public void Dispose() { if(_dispatcher != null) { _dispatcher.VerifyAccess(); _dispatcher._disableProcessingCount--; _dispatcher = null; } } ////// Checks whether this object is equal to another /// DispatcherProcessingDisabled object. /// /// /// Object to compare with. /// ////// Returns true when the object is equal to the specified object, /// and false otherwise. /// public override bool Equals(object obj) { if ((null == obj) || !(obj is DispatcherProcessingDisabled)) return false; return (this._dispatcher == ((DispatcherProcessingDisabled)obj)._dispatcher); } ////// Compute hash code for this object. /// ///A 32-bit signed integer hash code. public override int GetHashCode( ) { return base.GetHashCode(); } ////// Compare two DispatcherProcessingDisabled instances for equality. /// /// /// left operand /// /// /// right operand /// ////// Whether or not two operands are equal. /// public static bool operator ==(DispatcherProcessingDisabled left, DispatcherProcessingDisabled right) { return left.Equals(right); } ////// Compare two DispatcherProcessingDisabled instances for inequality. /// /// /// left operand /// /// /// right operand /// ////// Whether or not two operands are equal. /// public static bool operator !=(DispatcherProcessingDisabled left, DispatcherProcessingDisabled right) { return !(left.Equals(right)); } internal Dispatcher _dispatcher; // set by Dispatcher } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
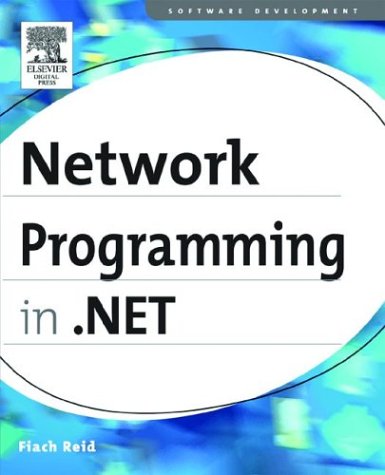
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BlockExpression.cs
- SpecialNameAttribute.cs
- AbsoluteQuery.cs
- Utilities.cs
- DockPanel.cs
- SafeTokenHandle.cs
- NullableFloatSumAggregationOperator.cs
- JavaScriptString.cs
- OleTxTransactionInfo.cs
- SafeViewOfFileHandle.cs
- XmlConverter.cs
- StyleTypedPropertyAttribute.cs
- DeadCharTextComposition.cs
- updateconfighost.cs
- Bold.cs
- XComponentModel.cs
- EncodingTable.cs
- DelegateBodyWriter.cs
- ManagementClass.cs
- DataGridTableStyleMappingNameEditor.cs
- QueryServiceConfigHandle.cs
- Command.cs
- DetailsViewCommandEventArgs.cs
- ReadContentAsBinaryHelper.cs
- CompiledQueryCacheKey.cs
- RectValueSerializer.cs
- RuntimeVariablesExpression.cs
- LinkClickEvent.cs
- EdmTypeAttribute.cs
- KeyGestureValueSerializer.cs
- InfoCardRequestException.cs
- DesignerProperties.cs
- SchemaNamespaceManager.cs
- DataGridViewCellStyleBuilderDialog.cs
- BuildProviderCollection.cs
- CompositionTarget.cs
- CodeIdentifier.cs
- MouseGestureConverter.cs
- Memoizer.cs
- InputBinder.cs
- EntityDataSourceSelectingEventArgs.cs
- HtmlShim.cs
- RecommendedAsConfigurableAttribute.cs
- ViewCellRelation.cs
- DataGridViewUtilities.cs
- ConnectionConsumerAttribute.cs
- PassportAuthenticationEventArgs.cs
- StagingAreaInputItem.cs
- _AuthenticationState.cs
- TextDecorationCollection.cs
- precedingsibling.cs
- GreenMethods.cs
- Attributes.cs
- QueuePathDialog.cs
- DataGridViewRowCancelEventArgs.cs
- RSACryptoServiceProvider.cs
- ColorTransform.cs
- RelationshipNavigation.cs
- ProfileServiceManager.cs
- WindowsScrollBarBits.cs
- PingOptions.cs
- SQLByteStorage.cs
- TypeElementCollection.cs
- UnsafeNativeMethods.cs
- CorrelationTokenTypeConvertor.cs
- RewritingValidator.cs
- UnmanagedMemoryStreamWrapper.cs
- bindurihelper.cs
- FontDriver.cs
- ComPlusServiceLoader.cs
- TransformCollection.cs
- TextEditorMouse.cs
- ServiceHttpHandlerFactory.cs
- TextServicesProperty.cs
- DbDeleteCommandTree.cs
- QueryContinueDragEvent.cs
- WmlImageAdapter.cs
- WebPartVerbCollection.cs
- GetRecipientRequest.cs
- Package.cs
- MailBnfHelper.cs
- FormatConvertedBitmap.cs
- _HelperAsyncResults.cs
- EventHandlersDesigner.cs
- ModifiableIteratorCollection.cs
- ProtocolImporter.cs
- Types.cs
- ExplicitDiscriminatorMap.cs
- EtwTrace.cs
- XmlBufferReader.cs
- Pen.cs
- ToolStripPanel.cs
- AspNetHostingPermission.cs
- XLinq.cs
- AdornerDecorator.cs
- FileChangesMonitor.cs
- AlternateView.cs
- LeftCellWrapper.cs
- SQLMembershipProvider.cs
- updatecommandorderer.cs