Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Activation / ServiceHttpHandlerFactory.cs / 1 / ServiceHttpHandlerFactory.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System.Threading; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Diagnostics; using System.Web; using System.Web.SessionState; using System.Security; class ServiceHttpHandlerFactory : IHttpHandlerFactory { IHttpHandler handler; ////// RequiresReview - Called outside PermitOnly context. /// [SecurityRequiresReview] public IHttpHandler GetHandler(HttpContext context, string requestType, string url, string pathTranslated) { if (this.handler == null) { this.handler = new ServiceHttpHandler(); } return this.handler; } ////// RequiresReview - Called outside PermitOnly context. /// [SecurityRequiresReview] public void ReleaseHandler(IHttpHandler handler) { DiagnosticUtility.DebugAssert(handler is ServiceHttpHandler, "ASP.NET asked to release the wrong handler."); } class ServiceHttpHandler : IHttpAsyncHandler, IRequiresSessionState { public bool IsReusable { ////// RequiresReview - Called outside PermitOnly context. /// [SecurityRequiresReview] get { return true; } } ////// Critical - Entry-point from ASP.NET, called outside PermitOnly context. /// ASP.NET calls are critical. /// ExecuteSynchronous is critical because it captures HostedImpersonationContext /// (and makes it available later) so caller must ensure that this is called in the right place. /// [SecurityCritical] public void ProcessRequest(HttpContext context) { ServiceHostingEnvironment.SafeEnsureInitialized(); HostedHttpRequestAsyncResult.ExecuteSynchronous(context.ApplicationInstance, true); } ////// Critical - Entry-point from ASP.NET, called outside PermitOnly context. /// ASP.NET calls are critical. /// ExecuteSynchronous is critical because it captures HostedImpersonationContext /// (and makes it available later) so caller must ensure that this is called in the right place. /// [SecurityCritical] public IAsyncResult BeginProcessRequest(HttpContext context, AsyncCallback cb, object extraData) { ServiceHostingEnvironment.SafeEnsureInitialized(); return new HostedHttpRequestAsyncResult(context.ApplicationInstance, true, cb, extraData); } ////// RequiresReview - Called outside PermitOnly context. /// [SecurityRequiresReview] public void EndProcessRequest(IAsyncResult result) { HostedHttpRequestAsyncResult.End(result); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
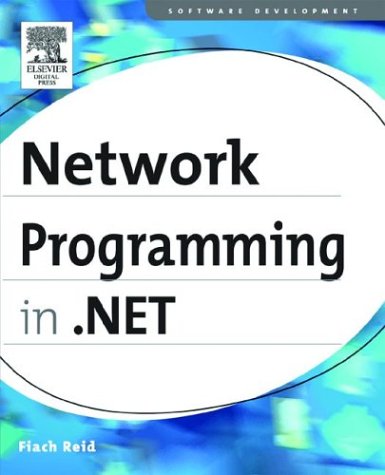
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientScriptManager.cs
- CompositeFontFamily.cs
- DependencyPropertyChangedEventArgs.cs
- SchemaDeclBase.cs
- DoubleAnimationUsingKeyFrames.cs
- NamespaceImport.cs
- ProfileSection.cs
- InstanceNotReadyException.cs
- ZoneMembershipCondition.cs
- RemotingSurrogateSelector.cs
- streamingZipPartStream.cs
- PasswordRecovery.cs
- COAUTHIDENTITY.cs
- PartialCachingControl.cs
- PropertyMetadata.cs
- ReferencedAssembly.cs
- MessageDroppedTraceRecord.cs
- MultipleViewPatternIdentifiers.cs
- ObjectConverter.cs
- AvTrace.cs
- OpenTypeMethods.cs
- ActiveXHelper.cs
- TreeViewItem.cs
- DataPager.cs
- GenerateScriptTypeAttribute.cs
- EditorZoneDesigner.cs
- Deflater.cs
- SelectorAutomationPeer.cs
- DateTimeOffsetStorage.cs
- PointCollection.cs
- CustomMenuItemCollection.cs
- CancelEventArgs.cs
- Exceptions.cs
- DataBindingCollection.cs
- ObjectConverter.cs
- ContextMenuStrip.cs
- DataServiceResponse.cs
- EditorAttribute.cs
- DataViewManagerListItemTypeDescriptor.cs
- XmlQueryTypeFactory.cs
- _RequestCacheProtocol.cs
- ImageMapEventArgs.cs
- ContainerParagraph.cs
- DataServiceProcessingPipelineEventArgs.cs
- SqlWriter.cs
- MethodBody.cs
- RequestResizeEvent.cs
- Comparer.cs
- EdmScalarPropertyAttribute.cs
- MailMessageEventArgs.cs
- DesignerActionUIService.cs
- MessageHeaderAttribute.cs
- GZipStream.cs
- StreamInfo.cs
- RtfNavigator.cs
- EntitySetBase.cs
- TreeNode.cs
- ListenUriMode.cs
- CategoryValueConverter.cs
- LocatorPart.cs
- DataSourceView.cs
- SortedSet.cs
- CodePageUtils.cs
- SqlDeflator.cs
- ComboBoxRenderer.cs
- Marshal.cs
- SqlExpander.cs
- ApplicationContext.cs
- FileDialog.cs
- Symbol.cs
- XmlException.cs
- PaperSize.cs
- Validator.cs
- CharacterMetrics.cs
- SqlReferenceCollection.cs
- PathSegment.cs
- GeometryCollection.cs
- HitTestWithPointDrawingContextWalker.cs
- DebuggerAttributes.cs
- SqlDependency.cs
- LiteralTextContainerControlBuilder.cs
- WindowsFormsHostPropertyMap.cs
- XmlNodeReader.cs
- SqlDataAdapter.cs
- SecurityContext.cs
- dsa.cs
- SoapAttributeOverrides.cs
- Button.cs
- Quaternion.cs
- BamlResourceDeserializer.cs
- XmlText.cs
- TextEditorCopyPaste.cs
- TextUtf8RawTextWriter.cs
- TextCompositionEventArgs.cs
- ParseHttpDate.cs
- XmlElementAttributes.cs
- PropertyMetadata.cs
- IndexedGlyphRun.cs
- IncrementalReadDecoders.cs
- PasswordPropertyTextAttribute.cs