Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / XPath / Internal / precedingsibling.cs / 1 / precedingsibling.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Collections.Generic; // This class can be rewritten much more efficient. // Algorithm could be like one for FollowingSibling: // - Build InputArrays: pares (first, sentinel) // -- Cash all input nodes as sentinel // -- Add firts node of its parent for each input node. // -- Sort these pares by first nodes. // - Advance algorithm will look like: // -- For each row in InputArays we will output first node + all its following nodes which are < sentinel // -- Before outputing each node in row #I we will check that it is < first node in row #I+1 // --- if true we actualy output it // --- if false, we hold with row #I and apply this algorith starting for row #I+1 // --- when we done with #I+1 we continue with row #I internal class PreSiblingQuery : CacheAxisQuery { public PreSiblingQuery(Query qyInput, string name, string prefix, XPathNodeType typeTest) : base (qyInput, name, prefix, typeTest) {} protected PreSiblingQuery(PreSiblingQuery other) : base(other) {} private bool NotVisited(XPathNavigator nav, ListparentStk){ XPathNavigator nav1 = nav.Clone(); nav1.MoveToParent(); for (int i = 0; i < parentStk.Count; i++) { if (nav1.IsSamePosition(parentStk[i])) { return false; } } parentStk.Add(nav1); return true; } public override object Evaluate(XPathNodeIterator context) { base.Evaluate(context); // Fill up base.outputBuffer List parentStk = new List (); Stack inputStk = new Stack (); while ((currentNode = qyInput.Advance()) != null) { inputStk.Push(currentNode.Clone()); } while (inputStk.Count != 0) { XPathNavigator input = inputStk.Pop(); if (input.NodeType == XPathNodeType.Attribute || input.NodeType == XPathNodeType.Namespace) { continue; } if (NotVisited(input, parentStk)) { XPathNavigator prev = input.Clone(); if (prev.MoveToParent()) { bool test = prev.MoveToFirstChild(); Debug.Assert(test, "We just moved to parent, how we can not have first child?"); while (!prev.IsSamePosition(input)) { if (matches(prev)) { Insert(outputBuffer, prev); } if (!prev.MoveToNext()) { Debug.Fail("We managed to miss sentinel node (input)"); break; } } } } } return this; } public override XPathNodeIterator Clone() { return new PreSiblingQuery(this); } public override QueryProps Properties { get { return base.Properties | QueryProps.Reverse; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
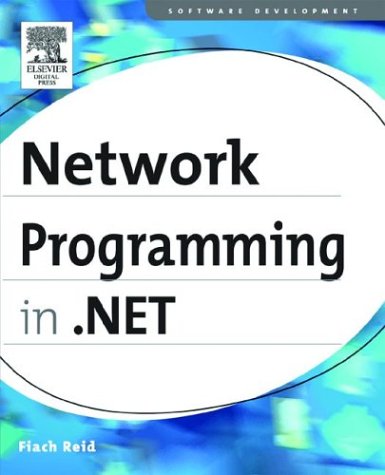
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeLibraryHandle.cs
- ToggleButton.cs
- UserControlAutomationPeer.cs
- OleDbConnectionInternal.cs
- Queue.cs
- Material.cs
- HyperlinkAutomationPeer.cs
- LazyTextWriterCreator.cs
- GetImportFileNameRequest.cs
- FixedSOMContainer.cs
- ConnectionStringsSection.cs
- ObjectNavigationPropertyMapping.cs
- XmlHierarchicalDataSourceView.cs
- HighlightComponent.cs
- Int32CollectionConverter.cs
- MetadataException.cs
- RelatedView.cs
- DefaultWorkflowTransactionService.cs
- HttpCapabilitiesEvaluator.cs
- DelegatingConfigHost.cs
- HopperCache.cs
- Point.cs
- dtdvalidator.cs
- ContextMarshalException.cs
- NameValueCache.cs
- OdbcConnectionOpen.cs
- CodeCastExpression.cs
- XmlILIndex.cs
- OleDbConnectionFactory.cs
- SqlBulkCopyColumnMapping.cs
- BamlLocalizer.cs
- SafeNativeMethods.cs
- SQLConvert.cs
- precedingquery.cs
- RequestNavigateEventArgs.cs
- __ConsoleStream.cs
- TcpHostedTransportConfiguration.cs
- DataChangedEventManager.cs
- DirectoryNotFoundException.cs
- IndexObject.cs
- SatelliteContractVersionAttribute.cs
- SolidColorBrush.cs
- LassoHelper.cs
- OrderByQueryOptionExpression.cs
- Decoder.cs
- XmlSchemaInfo.cs
- EventMappingSettingsCollection.cs
- GeneratedCodeAttribute.cs
- MetadataItemCollectionFactory.cs
- MsmqIntegrationElement.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- SqlConnectionStringBuilder.cs
- ButtonFieldBase.cs
- GregorianCalendar.cs
- HeaderCollection.cs
- ClassicBorderDecorator.cs
- securitycriticaldata.cs
- FileLevelControlBuilderAttribute.cs
- EncoderBestFitFallback.cs
- AnimationTimeline.cs
- TreePrinter.cs
- WebPartTransformer.cs
- Msmq4PoisonHandler.cs
- SelectionUIHandler.cs
- MediaElementAutomationPeer.cs
- TranslateTransform.cs
- HttpHandlersSection.cs
- HtmlElementCollection.cs
- Serializer.cs
- BaseUriHelper.cs
- TypeTypeConverter.cs
- MetadataArtifactLoaderComposite.cs
- InArgument.cs
- ClientOptions.cs
- LiteralControl.cs
- InfoCardProofToken.cs
- WindowsClaimSet.cs
- PreloadedPackages.cs
- XmlTypeAttribute.cs
- Rotation3DAnimationBase.cs
- MenuCommand.cs
- WebPartDeleteVerb.cs
- LogReservationCollection.cs
- AddInIpcChannel.cs
- InternalsVisibleToAttribute.cs
- MimeBasePart.cs
- NativeMethods.cs
- KeyProperty.cs
- KeySpline.cs
- CompiledQuery.cs
- ComplexObject.cs
- SettingsPropertyNotFoundException.cs
- _BufferOffsetSize.cs
- FixedSOMPageConstructor.cs
- InheritedPropertyChangedEventArgs.cs
- EntityDataSourceSelectingEventArgs.cs
- AutomationPatternInfo.cs
- ApplicationDirectoryMembershipCondition.cs
- BuilderInfo.cs
- SiteMapPath.cs