Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntityDesign / Design / System / Data / EntityModel / LazyTextWriterCreator.cs / 1305376 / LazyTextWriterCreator.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.IO; using System.Diagnostics; using System.Runtime.Versioning; namespace System.Data.Entity.Design { ////// This class is responsible for abstracting the knowledge /// of whether the user provided a TextWriter or a FilePath. /// /// If the user gave us a filePath we try not to create the TextWriter /// till we absolutely need it in order to prevent the file from being created /// in error cases. /// internal class LazyTextWriterCreator : IDisposable { private bool _ownTextWriter; private TextWriter _writer = null; private string _targetFilePath = null; internal LazyTextWriterCreator(TextWriter writer) { Debug.Assert(writer != null, "writer parameter is null"); _ownTextWriter = false; _writer = writer; } [ResourceExposure(ResourceScope.Machine)] //The target file path is used to open a stream which is a machine resource. internal LazyTextWriterCreator(string targetFilePath) { Debug.Assert(targetFilePath != null, "targetFilePath parameter is null"); _ownTextWriter = true; _targetFilePath = targetFilePath; } [ResourceExposure(ResourceScope.None)] //The resource( target file path) is not exposed to the callers of this method [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] //For StreamWriter constructor and we pick //the target file path from class variable. internal TextWriter GetOrCreateTextWriter() { if (_writer == null) { // lazy creating the writer _writer = new StreamWriter(_targetFilePath); } return _writer; } internal string TargetFilePath { get { return _targetFilePath; } } internal bool IsUserSuppliedTextWriter { get { return !_ownTextWriter; } } public void Dispose() { // Technically, calling GC.SuppressFinalize is not required because the class does not // have a finalizer, but it does no harm, protects against the case where a finalizer is added // in the future, and prevents an FxCop warning. GC.SuppressFinalize(this); if (_ownTextWriter && _writer != null) { _writer.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
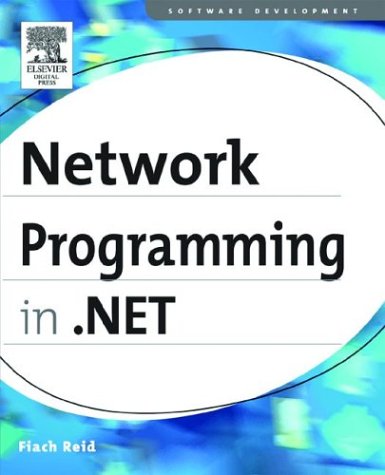
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLGuidStorage.cs
- ResizingMessageFilter.cs
- XmlWriter.cs
- WindowsUpDown.cs
- NamespaceCollection.cs
- ChangeNode.cs
- TextViewBase.cs
- PublisherIdentityPermission.cs
- DrawTreeNodeEventArgs.cs
- BmpBitmapDecoder.cs
- AnnotationService.cs
- DesignSurfaceServiceContainer.cs
- Command.cs
- GridViewAutomationPeer.cs
- TaskHelper.cs
- XmlComment.cs
- SamlSerializer.cs
- NetSectionGroup.cs
- BamlVersionHeader.cs
- RtType.cs
- MouseEvent.cs
- NameValueCollection.cs
- _ConnectionGroup.cs
- Relationship.cs
- CommandLibraryHelper.cs
- PrintControllerWithStatusDialog.cs
- XmlSchemaFacet.cs
- MediaPlayerState.cs
- LoadedOrUnloadedOperation.cs
- XmlNavigatorFilter.cs
- ProfileService.cs
- OrderablePartitioner.cs
- OdbcException.cs
- CheckBoxList.cs
- dtdvalidator.cs
- SafeThreadHandle.cs
- X509SecurityToken.cs
- XmlArrayItemAttribute.cs
- ReadOnlyCollectionBase.cs
- WindowsSpinner.cs
- AssemblyName.cs
- ConfigurationSectionGroup.cs
- InlinedAggregationOperator.cs
- TemplateColumn.cs
- TemplateControl.cs
- ApplicationHost.cs
- PageThemeBuildProvider.cs
- EntityDataSourceReferenceGroup.cs
- ResourceContainer.cs
- BaseDataList.cs
- ConstrainedDataObject.cs
- SmtpException.cs
- ServiceModelConfigurationSectionCollection.cs
- XmlSchemaInclude.cs
- Activator.cs
- ColumnMap.cs
- ZoneLinkButton.cs
- TypeElement.cs
- HandlerFactoryWrapper.cs
- AssemblyBuilder.cs
- BaseProcessor.cs
- ImportFileRequest.cs
- RuleSetDialog.cs
- WindowsRichEdit.cs
- DataObjectPastingEventArgs.cs
- HttpServerVarsCollection.cs
- ScalarOps.cs
- BufferBuilder.cs
- XmlSchemaInfo.cs
- ToolStripContainer.cs
- OleDbEnumerator.cs
- ChtmlTextWriter.cs
- XmlSerializerAssemblyAttribute.cs
- BoundField.cs
- WebFaultClientMessageInspector.cs
- KnownBoxes.cs
- ErrorFormatterPage.cs
- JournalEntryListConverter.cs
- Delegate.cs
- ACE.cs
- BitmapCodecInfo.cs
- figurelength.cs
- ReturnEventArgs.cs
- InputQueueChannel.cs
- InputLanguage.cs
- Line.cs
- MessageRpc.cs
- DoubleAnimationClockResource.cs
- GetPageNumberCompletedEventArgs.cs
- TabControlCancelEvent.cs
- PagePropertiesChangingEventArgs.cs
- WebPartAuthorizationEventArgs.cs
- DbParameterHelper.cs
- DataGridColumn.cs
- TaskSchedulerException.cs
- PersonalizationStateInfoCollection.cs
- PointAnimationUsingPath.cs
- GridView.cs
- EventDescriptorCollection.cs
- OraclePermission.cs