Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / XmlNavigatorFilter.cs / 1305376 / XmlNavigatorFilter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// XmlNavigatorFilter provides a flexible filtering abstraction over XPathNavigator. Callers do /// not know what type of filtering will occur; they simply call MoveToContent or MoveToSibling. /// The filter implementation invokes appropriate operation(s) on the XPathNavigator in order /// to skip over filtered nodes. /// [EditorBrowsable(EditorBrowsableState.Never)] public abstract class XmlNavigatorFilter { ////// Reposition the navigator to the first matching content node (inc. attributes); skip over /// filtered nodes. If there are no matching nodes, then don't move navigator and return false. /// public abstract bool MoveToContent(XPathNavigator navigator); ////// Reposition the navigator to the next matching content node (inc. attributes); skip over /// filtered nodes. If there are no matching nodes, then don't move navigator and return false. /// public abstract bool MoveToNextContent(XPathNavigator navigator); ////// Reposition the navigator to the next following sibling node (no attributes); skip over /// filtered nodes. If there are no matching nodes, then don't move navigator and return false. /// public abstract bool MoveToFollowingSibling(XPathNavigator navigator); ////// Reposition the navigator to the previous sibling node (no attributes); skip over filtered /// nodes. If there are no matching nodes, then don't move navigator and return false. /// public abstract bool MoveToPreviousSibling(XPathNavigator navigator); ////// Reposition the navigator to the next following node (inc. descendants); skip over filtered nodes. /// If there are no matching nodes, then return false. /// public abstract bool MoveToFollowing(XPathNavigator navigator, XPathNavigator navigatorEnd); ////// Return true if the navigator's current node matches the filter condition. /// public abstract bool IsFiltered(XPathNavigator navigator); } ////// Filters any non-element and any element with a non-matching local name or namespace uri. /// internal class XmlNavNameFilter : XmlNavigatorFilter { private string localName; private string namespaceUri; ////// Return an XmlNavigatorFilter that skips over nodes that do not match the specified name. /// public static XmlNavigatorFilter Create(string localName, string namespaceUri) { return new XmlNavNameFilter(localName, namespaceUri); } ////// Keep only elements with name = localName, namespaceUri. /// private XmlNavNameFilter(string localName, string namespaceUri) { this.localName = localName; this.namespaceUri = namespaceUri; } ////// Reposition the navigator on the first element child with a matching name. /// public override bool MoveToContent(XPathNavigator navigator) { return navigator.MoveToChild(this.localName, this.namespaceUri); } ////// Reposition the navigator on the next element child with a matching name. /// public override bool MoveToNextContent(XPathNavigator navigator) { return navigator.MoveToNext(this.localName, this.namespaceUri); } ////// Reposition the navigator on the next element sibling with a matching name. /// public override bool MoveToFollowingSibling(XPathNavigator navigator) { return navigator.MoveToNext(this.localName, this.namespaceUri); } ////// Reposition the navigator on the previous element sibling with a matching name. /// public override bool MoveToPreviousSibling(XPathNavigator navigator) { return navigator.MoveToPrevious(this.localName, this.namespaceUri); } ////// Reposition the navigator on the next following element with a matching name. /// public override bool MoveToFollowing(XPathNavigator navigator, XPathNavigator navEnd) { return navigator.MoveToFollowing(this.localName, this.namespaceUri, navEnd); } ////// Return false if the navigator is positioned on an element with a matching name. /// public override bool IsFiltered(XPathNavigator navigator) { return navigator.LocalName != this.localName || navigator.NamespaceURI != namespaceUri; } } ////// Filters any node not of the specified type (type may not be attribute or namespace). /// internal class XmlNavTypeFilter : XmlNavigatorFilter { private static XmlNavigatorFilter[] TypeFilters; private XPathNodeType nodeType; private int mask; ////// There are a limited number of types, so create all possible XmlNavTypeFilter objects just once. /// static XmlNavTypeFilter() { TypeFilters = new XmlNavigatorFilter[(int) XPathNodeType.Comment + 1]; TypeFilters[(int) XPathNodeType.Element] = new XmlNavTypeFilter(XPathNodeType.Element); TypeFilters[(int) XPathNodeType.Text] = new XmlNavTypeFilter(XPathNodeType.Text); TypeFilters[(int) XPathNodeType.ProcessingInstruction] = new XmlNavTypeFilter(XPathNodeType.ProcessingInstruction); TypeFilters[(int) XPathNodeType.Comment] = new XmlNavTypeFilter(XPathNodeType.Comment); } ////// Return a previously constructed XmlNavigatorFilter that skips over nodes that do not match the specified type. /// public static XmlNavigatorFilter Create(XPathNodeType nodeType) { Debug.Assert(TypeFilters[(int) nodeType] != null); return TypeFilters[(int) nodeType]; } ////// Keep only nodes with XPathNodeType = nodeType, where XPathNodeType.Text selects whitespace as well. /// private XmlNavTypeFilter(XPathNodeType nodeType) { Debug.Assert(nodeType != XPathNodeType.Attribute && nodeType != XPathNodeType.Namespace); this.nodeType = nodeType; this.mask = XPathNavigator.GetContentKindMask(nodeType); } ////// Reposition the navigator on the first child with a matching type. /// public override bool MoveToContent(XPathNavigator navigator) { return navigator.MoveToChild(this.nodeType); } ////// Reposition the navigator on the next child with a matching type. /// public override bool MoveToNextContent(XPathNavigator navigator) { return navigator.MoveToNext(this.nodeType); } ////// Reposition the navigator on the next non-attribute sibling with a matching type. /// public override bool MoveToFollowingSibling(XPathNavigator navigator) { return navigator.MoveToNext(this.nodeType); } ////// Reposition the navigator on the previous non-attribute sibling with a matching type. /// public override bool MoveToPreviousSibling(XPathNavigator navigator) { return navigator.MoveToPrevious(this.nodeType); } ////// Reposition the navigator on the next following element with a matching kind. /// public override bool MoveToFollowing(XPathNavigator navigator, XPathNavigator navEnd) { return navigator.MoveToFollowing(this.nodeType, navEnd); } ////// Return false if the navigator is positioned on a node with a matching type. /// public override bool IsFiltered(XPathNavigator navigator) { return ((1 << (int) navigator.NodeType) & this.mask) == 0; } } ////// Filters all attribute nodes. /// internal class XmlNavAttrFilter : XmlNavigatorFilter { private static XmlNavigatorFilter Singleton = new XmlNavAttrFilter(); ////// Return a singleton XmlNavigatorFilter that filters all attribute nodes. /// public static XmlNavigatorFilter Create() { return Singleton; } ////// Constructor. /// private XmlNavAttrFilter() { } ////// Reposition the navigator on the first non-attribute child. /// public override bool MoveToContent(XPathNavigator navigator) { return navigator.MoveToFirstChild(); } ////// Reposition the navigator on the next non-attribute sibling. /// public override bool MoveToNextContent(XPathNavigator navigator) { return navigator.MoveToNext(); } ////// Reposition the navigator on the next non-attribute sibling. /// public override bool MoveToFollowingSibling(XPathNavigator navigator) { return navigator.MoveToNext(); } ////// Reposition the navigator on the previous non-attribute sibling. /// public override bool MoveToPreviousSibling(XPathNavigator navigator) { return navigator.MoveToPrevious(); } ////// Reposition the navigator on the next following non-attribute. /// public override bool MoveToFollowing(XPathNavigator navigator, XPathNavigator navEnd) { return navigator.MoveToFollowing(XPathNodeType.All, navEnd); } ////// Return true if the navigator is positioned on an attribute. /// public override bool IsFiltered(XPathNavigator navigator) { return navigator.NodeType == XPathNodeType.Attribute; } } ////// Never filter nodes. /// internal class XmlNavNeverFilter : XmlNavigatorFilter { private static XmlNavigatorFilter Singleton = new XmlNavNeverFilter(); ////// Return a singleton XmlNavigatorFilter that never filters any nodes. /// public static XmlNavigatorFilter Create() { return Singleton; } ////// Constructor. /// private XmlNavNeverFilter() { } ////// Reposition the navigator on the first child (attribute or non-attribute). /// public override bool MoveToContent(XPathNavigator navigator) { return MoveToFirstAttributeContent(navigator); } ////// Reposition the navigator on the next child (attribute or non-attribute). /// public override bool MoveToNextContent(XPathNavigator navigator) { return MoveToNextAttributeContent(navigator); } ////// Reposition the navigator on the next sibling (no attributes). /// public override bool MoveToFollowingSibling(XPathNavigator navigator) { return navigator.MoveToNext(); } ////// Reposition the navigator on the previous sibling (no attributes). /// public override bool MoveToPreviousSibling(XPathNavigator navigator) { return navigator.MoveToPrevious(); } ////// Reposition the navigator on the next following node. /// public override bool MoveToFollowing(XPathNavigator navigator, XPathNavigator navEnd) { return navigator.MoveToFollowing(XPathNodeType.All, navEnd); } ////// Nodes are never filtered so always return false. /// public override bool IsFiltered(XPathNavigator navigator) { return false; } ////// Move to navigator's first attribute node. If no attribute's exist, move to the first content node. /// If no content nodes exist, return null. Otherwise, return navigator. /// public static bool MoveToFirstAttributeContent(XPathNavigator navigator) { if (!navigator.MoveToFirstAttribute()) return navigator.MoveToFirstChild(); return true; } ////// If navigator is positioned on an attribute, move to the next attribute node. If there are no more /// attributes, move to the first content node. If navigator is positioned on a content node, move to /// the next content node. If there are no more attributes and content nodes, return null. /// Otherwise, return navigator. /// public static bool MoveToNextAttributeContent(XPathNavigator navigator) { if (navigator.NodeType == XPathNodeType.Attribute) { if (!navigator.MoveToNextAttribute()) { navigator.MoveToParent(); if (!navigator.MoveToFirstChild()) { // No children, so reposition on original attribute navigator.MoveToFirstAttribute(); while (navigator.MoveToNextAttribute()) ; return false; } } return true; } return navigator.MoveToNext(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// XmlNavigatorFilter provides a flexible filtering abstraction over XPathNavigator. Callers do /// not know what type of filtering will occur; they simply call MoveToContent or MoveToSibling. /// The filter implementation invokes appropriate operation(s) on the XPathNavigator in order /// to skip over filtered nodes. /// [EditorBrowsable(EditorBrowsableState.Never)] public abstract class XmlNavigatorFilter { ////// Reposition the navigator to the first matching content node (inc. attributes); skip over /// filtered nodes. If there are no matching nodes, then don't move navigator and return false. /// public abstract bool MoveToContent(XPathNavigator navigator); ////// Reposition the navigator to the next matching content node (inc. attributes); skip over /// filtered nodes. If there are no matching nodes, then don't move navigator and return false. /// public abstract bool MoveToNextContent(XPathNavigator navigator); ////// Reposition the navigator to the next following sibling node (no attributes); skip over /// filtered nodes. If there are no matching nodes, then don't move navigator and return false. /// public abstract bool MoveToFollowingSibling(XPathNavigator navigator); ////// Reposition the navigator to the previous sibling node (no attributes); skip over filtered /// nodes. If there are no matching nodes, then don't move navigator and return false. /// public abstract bool MoveToPreviousSibling(XPathNavigator navigator); ////// Reposition the navigator to the next following node (inc. descendants); skip over filtered nodes. /// If there are no matching nodes, then return false. /// public abstract bool MoveToFollowing(XPathNavigator navigator, XPathNavigator navigatorEnd); ////// Return true if the navigator's current node matches the filter condition. /// public abstract bool IsFiltered(XPathNavigator navigator); } ////// Filters any non-element and any element with a non-matching local name or namespace uri. /// internal class XmlNavNameFilter : XmlNavigatorFilter { private string localName; private string namespaceUri; ////// Return an XmlNavigatorFilter that skips over nodes that do not match the specified name. /// public static XmlNavigatorFilter Create(string localName, string namespaceUri) { return new XmlNavNameFilter(localName, namespaceUri); } ////// Keep only elements with name = localName, namespaceUri. /// private XmlNavNameFilter(string localName, string namespaceUri) { this.localName = localName; this.namespaceUri = namespaceUri; } ////// Reposition the navigator on the first element child with a matching name. /// public override bool MoveToContent(XPathNavigator navigator) { return navigator.MoveToChild(this.localName, this.namespaceUri); } ////// Reposition the navigator on the next element child with a matching name. /// public override bool MoveToNextContent(XPathNavigator navigator) { return navigator.MoveToNext(this.localName, this.namespaceUri); } ////// Reposition the navigator on the next element sibling with a matching name. /// public override bool MoveToFollowingSibling(XPathNavigator navigator) { return navigator.MoveToNext(this.localName, this.namespaceUri); } ////// Reposition the navigator on the previous element sibling with a matching name. /// public override bool MoveToPreviousSibling(XPathNavigator navigator) { return navigator.MoveToPrevious(this.localName, this.namespaceUri); } ////// Reposition the navigator on the next following element with a matching name. /// public override bool MoveToFollowing(XPathNavigator navigator, XPathNavigator navEnd) { return navigator.MoveToFollowing(this.localName, this.namespaceUri, navEnd); } ////// Return false if the navigator is positioned on an element with a matching name. /// public override bool IsFiltered(XPathNavigator navigator) { return navigator.LocalName != this.localName || navigator.NamespaceURI != namespaceUri; } } ////// Filters any node not of the specified type (type may not be attribute or namespace). /// internal class XmlNavTypeFilter : XmlNavigatorFilter { private static XmlNavigatorFilter[] TypeFilters; private XPathNodeType nodeType; private int mask; ////// There are a limited number of types, so create all possible XmlNavTypeFilter objects just once. /// static XmlNavTypeFilter() { TypeFilters = new XmlNavigatorFilter[(int) XPathNodeType.Comment + 1]; TypeFilters[(int) XPathNodeType.Element] = new XmlNavTypeFilter(XPathNodeType.Element); TypeFilters[(int) XPathNodeType.Text] = new XmlNavTypeFilter(XPathNodeType.Text); TypeFilters[(int) XPathNodeType.ProcessingInstruction] = new XmlNavTypeFilter(XPathNodeType.ProcessingInstruction); TypeFilters[(int) XPathNodeType.Comment] = new XmlNavTypeFilter(XPathNodeType.Comment); } ////// Return a previously constructed XmlNavigatorFilter that skips over nodes that do not match the specified type. /// public static XmlNavigatorFilter Create(XPathNodeType nodeType) { Debug.Assert(TypeFilters[(int) nodeType] != null); return TypeFilters[(int) nodeType]; } ////// Keep only nodes with XPathNodeType = nodeType, where XPathNodeType.Text selects whitespace as well. /// private XmlNavTypeFilter(XPathNodeType nodeType) { Debug.Assert(nodeType != XPathNodeType.Attribute && nodeType != XPathNodeType.Namespace); this.nodeType = nodeType; this.mask = XPathNavigator.GetContentKindMask(nodeType); } ////// Reposition the navigator on the first child with a matching type. /// public override bool MoveToContent(XPathNavigator navigator) { return navigator.MoveToChild(this.nodeType); } ////// Reposition the navigator on the next child with a matching type. /// public override bool MoveToNextContent(XPathNavigator navigator) { return navigator.MoveToNext(this.nodeType); } ////// Reposition the navigator on the next non-attribute sibling with a matching type. /// public override bool MoveToFollowingSibling(XPathNavigator navigator) { return navigator.MoveToNext(this.nodeType); } ////// Reposition the navigator on the previous non-attribute sibling with a matching type. /// public override bool MoveToPreviousSibling(XPathNavigator navigator) { return navigator.MoveToPrevious(this.nodeType); } ////// Reposition the navigator on the next following element with a matching kind. /// public override bool MoveToFollowing(XPathNavigator navigator, XPathNavigator navEnd) { return navigator.MoveToFollowing(this.nodeType, navEnd); } ////// Return false if the navigator is positioned on a node with a matching type. /// public override bool IsFiltered(XPathNavigator navigator) { return ((1 << (int) navigator.NodeType) & this.mask) == 0; } } ////// Filters all attribute nodes. /// internal class XmlNavAttrFilter : XmlNavigatorFilter { private static XmlNavigatorFilter Singleton = new XmlNavAttrFilter(); ////// Return a singleton XmlNavigatorFilter that filters all attribute nodes. /// public static XmlNavigatorFilter Create() { return Singleton; } ////// Constructor. /// private XmlNavAttrFilter() { } ////// Reposition the navigator on the first non-attribute child. /// public override bool MoveToContent(XPathNavigator navigator) { return navigator.MoveToFirstChild(); } ////// Reposition the navigator on the next non-attribute sibling. /// public override bool MoveToNextContent(XPathNavigator navigator) { return navigator.MoveToNext(); } ////// Reposition the navigator on the next non-attribute sibling. /// public override bool MoveToFollowingSibling(XPathNavigator navigator) { return navigator.MoveToNext(); } ////// Reposition the navigator on the previous non-attribute sibling. /// public override bool MoveToPreviousSibling(XPathNavigator navigator) { return navigator.MoveToPrevious(); } ////// Reposition the navigator on the next following non-attribute. /// public override bool MoveToFollowing(XPathNavigator navigator, XPathNavigator navEnd) { return navigator.MoveToFollowing(XPathNodeType.All, navEnd); } ////// Return true if the navigator is positioned on an attribute. /// public override bool IsFiltered(XPathNavigator navigator) { return navigator.NodeType == XPathNodeType.Attribute; } } ////// Never filter nodes. /// internal class XmlNavNeverFilter : XmlNavigatorFilter { private static XmlNavigatorFilter Singleton = new XmlNavNeverFilter(); ////// Return a singleton XmlNavigatorFilter that never filters any nodes. /// public static XmlNavigatorFilter Create() { return Singleton; } ////// Constructor. /// private XmlNavNeverFilter() { } ////// Reposition the navigator on the first child (attribute or non-attribute). /// public override bool MoveToContent(XPathNavigator navigator) { return MoveToFirstAttributeContent(navigator); } ////// Reposition the navigator on the next child (attribute or non-attribute). /// public override bool MoveToNextContent(XPathNavigator navigator) { return MoveToNextAttributeContent(navigator); } ////// Reposition the navigator on the next sibling (no attributes). /// public override bool MoveToFollowingSibling(XPathNavigator navigator) { return navigator.MoveToNext(); } ////// Reposition the navigator on the previous sibling (no attributes). /// public override bool MoveToPreviousSibling(XPathNavigator navigator) { return navigator.MoveToPrevious(); } ////// Reposition the navigator on the next following node. /// public override bool MoveToFollowing(XPathNavigator navigator, XPathNavigator navEnd) { return navigator.MoveToFollowing(XPathNodeType.All, navEnd); } ////// Nodes are never filtered so always return false. /// public override bool IsFiltered(XPathNavigator navigator) { return false; } ////// Move to navigator's first attribute node. If no attribute's exist, move to the first content node. /// If no content nodes exist, return null. Otherwise, return navigator. /// public static bool MoveToFirstAttributeContent(XPathNavigator navigator) { if (!navigator.MoveToFirstAttribute()) return navigator.MoveToFirstChild(); return true; } ////// If navigator is positioned on an attribute, move to the next attribute node. If there are no more /// attributes, move to the first content node. If navigator is positioned on a content node, move to /// the next content node. If there are no more attributes and content nodes, return null. /// Otherwise, return navigator. /// public static bool MoveToNextAttributeContent(XPathNavigator navigator) { if (navigator.NodeType == XPathNodeType.Attribute) { if (!navigator.MoveToNextAttribute()) { navigator.MoveToParent(); if (!navigator.MoveToFirstChild()) { // No children, so reposition on original attribute navigator.MoveToFirstAttribute(); while (navigator.MoveToNextAttribute()) ; return false; } } return true; } return navigator.MoveToNext(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
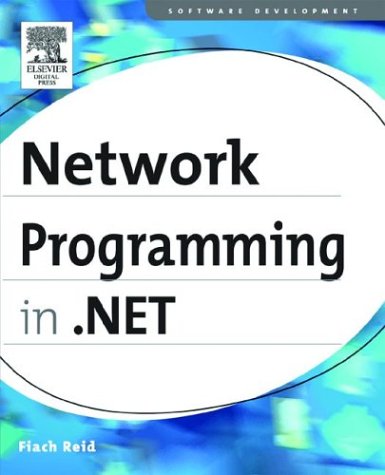
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SoapExtensionImporter.cs
- NameValueConfigurationCollection.cs
- XNodeValidator.cs
- FixedSOMTextRun.cs
- LookupBindingPropertiesAttribute.cs
- FontStretchConverter.cs
- TailCallAnalyzer.cs
- TextCollapsingProperties.cs
- CommandCollectionEditor.cs
- SectionUpdates.cs
- PtsPage.cs
- DeflateInput.cs
- WebExceptionStatus.cs
- MethodMessage.cs
- ContainerUIElement3D.cs
- Trace.cs
- Types.cs
- StrongName.cs
- ObjectStateManager.cs
- NetworkInformationException.cs
- StreamGeometry.cs
- TemplatePropertyEntry.cs
- SemanticResultKey.cs
- ResourceAttributes.cs
- IIS7WorkerRequest.cs
- DataMemberListEditor.cs
- GeneralTransform3D.cs
- SplayTreeNode.cs
- Clipboard.cs
- Color.cs
- Brushes.cs
- ProcessModule.cs
- UITypeEditor.cs
- AnnotationHighlightLayer.cs
- IssuedTokenParametersEndpointAddressElement.cs
- QueryResponse.cs
- Pens.cs
- DictionaryBase.cs
- WeakReference.cs
- ObjectItemCachedAssemblyLoader.cs
- Pool.cs
- ActivityIdHeader.cs
- CodeDirectiveCollection.cs
- FixedPosition.cs
- ReadOnlyDictionary.cs
- InvalidPropValue.cs
- StrokeCollection2.cs
- InitializerFacet.cs
- HandlerMappingMemo.cs
- DateTimeUtil.cs
- WebCategoryAttribute.cs
- _SafeNetHandles.cs
- MatchingStyle.cs
- ToolStripSplitStackLayout.cs
- MetadataItemEmitter.cs
- XmlLangPropertyAttribute.cs
- FileLevelControlBuilderAttribute.cs
- CodeArrayIndexerExpression.cs
- HttpHandlersSection.cs
- SqlBuilder.cs
- CssStyleCollection.cs
- CommonGetThemePartSize.cs
- GenericPrincipal.cs
- MulticastOption.cs
- CannotUnloadAppDomainException.cs
- ProcessStartInfo.cs
- BevelBitmapEffect.cs
- IList.cs
- OutArgumentConverter.cs
- GetPageNumberCompletedEventArgs.cs
- CachedFontFace.cs
- ErrorEventArgs.cs
- RegionIterator.cs
- Rss20ItemFormatter.cs
- WindowShowOrOpenTracker.cs
- Expressions.cs
- XmlNullResolver.cs
- DefinitionUpdate.cs
- ComNativeDescriptor.cs
- AnchorEditor.cs
- XmlNotation.cs
- HostingEnvironmentException.cs
- CodeSpit.cs
- Sql8ExpressionRewriter.cs
- ProviderConnectionPointCollection.cs
- AutomationElementCollection.cs
- OleDbWrapper.cs
- PointValueSerializer.cs
- NotCondition.cs
- ResourceKey.cs
- ToolStripManager.cs
- CalendarButtonAutomationPeer.cs
- HyperLink.cs
- PrivilegeNotHeldException.cs
- PolyBezierSegment.cs
- IncrementalCompileAnalyzer.cs
- ExpressionBuilderCollection.cs
- Style.cs
- LogSwitch.cs
- ColorAnimationUsingKeyFrames.cs