Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Services / Monitoring / system / Diagnosticts / ProcessModule.cs / 1 / ProcessModule.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Diagnostics { using System.Diagnostics; using System; using System.Collections; using System.IO; using Microsoft.Win32; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; // using System.Windows.Forms; ////// A process module component represents a DLL or EXE loaded into /// a particular process. Using this component, you can determine /// information about the module. /// [Designer("System.Diagnostics.Design.ProcessModuleDesigner, " + AssemblyRef.SystemDesign)] [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] public class ProcessModule : Component { internal ModuleInfo moduleInfo; FileVersionInfo fileVersionInfo; ////// Initialize the module. /// ///internal ProcessModule(ModuleInfo moduleInfo) { this.moduleInfo = moduleInfo; GC.SuppressFinalize(this); } /// /// Make sure we are running on NT. /// ///internal void EnsureNtProcessInfo() { if (Environment.OSVersion.Platform != PlatformID.Win32NT) throw new PlatformNotSupportedException(SR.GetString(SR.WinNTRequired)); } /// /// Returns the name of the Module. /// [MonitoringDescription(SR.ProcModModuleName)] public string ModuleName { get { return moduleInfo.baseName; } } ////// Returns the full file path for the location of the module. /// [MonitoringDescription(SR.ProcModFileName)] public string FileName { get { return moduleInfo.fileName; } } ////// Returns the memory address that the module was loaded at. /// [MonitoringDescription(SR.ProcModBaseAddress)] public IntPtr BaseAddress { get { return moduleInfo.baseOfDll; } } ////// Returns the amount of memory required to load the module. This does /// not include any additional memory allocations made by the module once /// it is running; it only includes the size of the static code and data /// in the module file. /// [MonitoringDescription(SR.ProcModModuleMemorySize)] public int ModuleMemorySize { get { return moduleInfo.sizeOfImage; } } ////// Returns the memory address for function that runs when the module is /// loaded and run. /// [MonitoringDescription(SR.ProcModEntryPointAddress)] public IntPtr EntryPointAddress { get { EnsureNtProcessInfo(); return moduleInfo.entryPoint; } } ////// Returns version information about the module. /// [Browsable(false)] public FileVersionInfo FileVersionInfo { get { if (fileVersionInfo == null) fileVersionInfo = FileVersionInfo.GetVersionInfo(FileName); return fileVersionInfo; } } public override string ToString() { return String.Format(CultureInfo.CurrentCulture, "{0} ({1})", base.ToString(), this.ModuleName); } } }
Link Menu
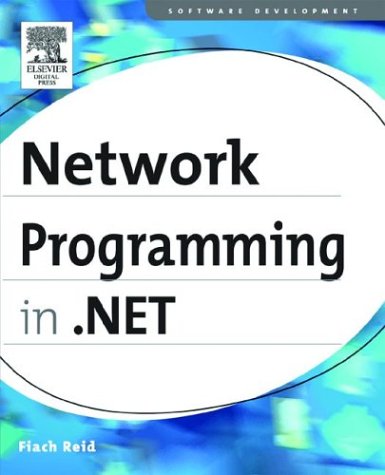
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlTextEncoder.cs
- DataGridViewImageColumn.cs
- TraceHandlerErrorFormatter.cs
- MarkupCompilePass1.cs
- ViewStateException.cs
- EdmMember.cs
- BlockUIContainer.cs
- ApplicationActivator.cs
- BaseCodeDomTreeGenerator.cs
- ContextMenuService.cs
- FormsAuthenticationCredentials.cs
- TextViewElement.cs
- References.cs
- AuthStoreRoleProvider.cs
- AdjustableArrowCap.cs
- ParameterCollection.cs
- WindowsSlider.cs
- WebContext.cs
- ClientType.cs
- VirtualPath.cs
- DataGridViewComboBoxCell.cs
- TextChangedEventArgs.cs
- FunctionDescription.cs
- DataGridViewCellParsingEventArgs.cs
- OperationAbortedException.cs
- RegistryDataKey.cs
- DynamicMetaObject.cs
- ByteConverter.cs
- ObjectAssociationEndMapping.cs
- SourceFilter.cs
- OracleDataAdapter.cs
- Site.cs
- ServiceParser.cs
- DefaultHttpHandler.cs
- OletxEnlistment.cs
- OrthographicCamera.cs
- FixedStringLookup.cs
- PerformanceCounters.cs
- OracleCommandSet.cs
- HtmlControlPersistable.cs
- ImportCatalogPart.cs
- AutoSizeToolBoxItem.cs
- WebBrowserContainer.cs
- ProjectionCamera.cs
- DataGridViewHitTestInfo.cs
- PeerToPeerException.cs
- XmlAttributes.cs
- BindingCollection.cs
- DragCompletedEventArgs.cs
- ProtocolState.cs
- HwndStylusInputProvider.cs
- ButtonBaseDesigner.cs
- SiteIdentityPermission.cs
- SID.cs
- BitmapEffectInputConnector.cs
- BehaviorEditorPart.cs
- XmlSchemaObject.cs
- SystemPens.cs
- Int64.cs
- NameValuePair.cs
- DataControlButton.cs
- DSASignatureDeformatter.cs
- TrackPointCollection.cs
- HitTestFilterBehavior.cs
- SequenceDesigner.cs
- CredentialCache.cs
- EntityViewGenerator.cs
- PrintDialog.cs
- ListControl.cs
- OleDbTransaction.cs
- AsymmetricSignatureDeformatter.cs
- Events.cs
- FixedPageAutomationPeer.cs
- XmlLangPropertyAttribute.cs
- PartialCachingControl.cs
- ScriptReferenceBase.cs
- ChangeDirector.cs
- FixedSOMPageConstructor.cs
- PartialCachingAttribute.cs
- HeaderUtility.cs
- SiteMapSection.cs
- ModifierKeysValueSerializer.cs
- PageAsyncTaskManager.cs
- ProfessionalColorTable.cs
- CodeDomDesignerLoader.cs
- SineEase.cs
- CharEntityEncoderFallback.cs
- UrlMappingsModule.cs
- NullableIntAverageAggregationOperator.cs
- EventLogPermissionAttribute.cs
- AtomEntry.cs
- QuadraticBezierSegment.cs
- Parser.cs
- NavigationEventArgs.cs
- QilNode.cs
- PassportAuthenticationModule.cs
- XPathExpr.cs
- HostProtectionPermission.cs
- xsdvalidator.cs
- DataGridViewAccessibleObject.cs