Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Effects / BitmapEffectInputConnector.cs / 1 / BitmapEffectInputConnector.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapEffectInputConnector.cs //----------------------------------------------------------------------------- using MS.Internal; using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; namespace System.Windows.Media.Effects { ////// BitmapEffectInputConnector /// internal class BitmapEffectInputConnector { SafeMILHandle /*IMILBitmapEffectInputConnector*/ connector; internal BitmapEffectInputConnector(SafeMILHandle nativeConnector) { Debug.Assert(nativeConnector != null); connector = nativeConnector; } #if never internal SafeMILHandle NativeConnectorSafeHandle { get { return connector; } } ////// Return true if the connector is connected. /// public bool IsConnected { get { Debug.Assert(connector != null); bool fConnected = false; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectInputConnector.IsConnected(connector, out fConnected)); return fConnected; } } ////// Returns the output connector /// public BitmapEffectOutputConnector OutputConnector { get { if (IsConnected == false) throw new Exception("The input is not connected"); SafeMILHandle connection; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectInputConnector.GetConnection(connector, out connection)); return new BitmapEffectOutputConnector(connection); } } #endif ////// Performs the connection /// /// the output to connect to public void ConnectTo(BitmapEffectOutputConnector output) { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectInputConnector.ConnectTo(connector, output.NativeConnectorSafeHandle)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapEffectInputConnector.cs //----------------------------------------------------------------------------- using MS.Internal; using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; namespace System.Windows.Media.Effects { ////// BitmapEffectInputConnector /// internal class BitmapEffectInputConnector { SafeMILHandle /*IMILBitmapEffectInputConnector*/ connector; internal BitmapEffectInputConnector(SafeMILHandle nativeConnector) { Debug.Assert(nativeConnector != null); connector = nativeConnector; } #if never internal SafeMILHandle NativeConnectorSafeHandle { get { return connector; } } ////// Return true if the connector is connected. /// public bool IsConnected { get { Debug.Assert(connector != null); bool fConnected = false; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectInputConnector.IsConnected(connector, out fConnected)); return fConnected; } } ////// Returns the output connector /// public BitmapEffectOutputConnector OutputConnector { get { if (IsConnected == false) throw new Exception("The input is not connected"); SafeMILHandle connection; HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectInputConnector.GetConnection(connector, out connection)); return new BitmapEffectOutputConnector(connection); } } #endif ////// Performs the connection /// /// the output to connect to public void ConnectTo(BitmapEffectOutputConnector output) { HRESULT.Check(MS.Win32.PresentationCore.UnsafeNativeMethods.IMILBitmapEffectInputConnector.ConnectTo(connector, output.NativeConnectorSafeHandle)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
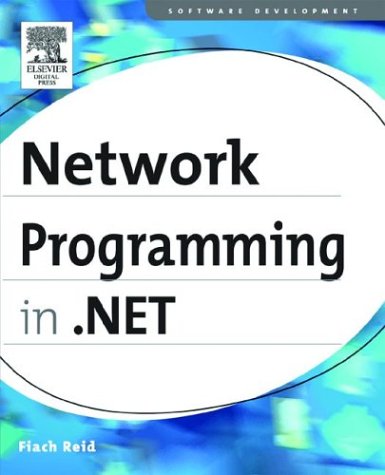
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HandleCollector.cs
- TypeSystem.cs
- DocumentGrid.cs
- CombinedGeometry.cs
- Style.cs
- AutoResetEvent.cs
- DBSqlParserColumn.cs
- PrintSchema.cs
- WebPartChrome.cs
- FixedHyperLink.cs
- SafeNativeMethods.cs
- HttpGetProtocolImporter.cs
- XmlSignatureManifest.cs
- ScrollViewerAutomationPeer.cs
- FontInfo.cs
- XmlSchemaParticle.cs
- ModelTypeConverter.cs
- MessageBox.cs
- ExceptionUtil.cs
- DeadCharTextComposition.cs
- ExpressionTable.cs
- HttpCookieCollection.cs
- SoapHelper.cs
- WindowsFont.cs
- RowBinding.cs
- DropTarget.cs
- SerializableTypeCodeDomSerializer.cs
- WebZone.cs
- MemberDescriptor.cs
- SqlXml.cs
- XamlDesignerSerializationManager.cs
- HitTestParameters.cs
- FloaterBaseParaClient.cs
- XmlNotation.cs
- ByteAnimationBase.cs
- HttpProfileGroupBase.cs
- WebPartUserCapability.cs
- FormClosingEvent.cs
- DataGridViewLayoutData.cs
- MessageEventSubscriptionService.cs
- CodeObjectCreateExpression.cs
- mda.cs
- PeerContact.cs
- FlowDocumentScrollViewer.cs
- PropertySourceInfo.cs
- MSAAWinEventWrap.cs
- PointHitTestResult.cs
- InProcStateClientManager.cs
- DependentList.cs
- ThrowOnMultipleAssignment.cs
- ContextStaticAttribute.cs
- TrackPoint.cs
- OLEDB_Enum.cs
- WorkflowViewStateService.cs
- TreeSet.cs
- LineBreak.cs
- ListenerElementsCollection.cs
- SrgsSubset.cs
- VarRefManager.cs
- HttpResponse.cs
- WrappedIUnknown.cs
- DbDeleteCommandTree.cs
- PasswordBox.cs
- TabControl.cs
- Deflater.cs
- HtmlWindowCollection.cs
- UrlAuthFailedErrorFormatter.cs
- SHA384Cng.cs
- StreamSecurityUpgradeInitiatorAsyncResult.cs
- CommunicationException.cs
- __Filters.cs
- DirectionalLight.cs
- ImmutableCollection.cs
- _NegoState.cs
- NameTable.cs
- GuidelineCollection.cs
- EmptyEnumerator.cs
- RenderData.cs
- SmiEventSink_DeferedProcessing.cs
- ConfigViewGenerator.cs
- WebControlsSection.cs
- SessionStateUtil.cs
- CultureInfo.cs
- IconBitmapDecoder.cs
- ForeignKeyConstraint.cs
- Group.cs
- PropertyFilterAttribute.cs
- UnsafeNativeMethods.cs
- Helper.cs
- DesignSurface.cs
- ProgressPage.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- ValueTypeFixupInfo.cs
- DateTimeConstantAttribute.cs
- GridViewEditEventArgs.cs
- DataTableClearEvent.cs
- TransportOutputChannel.cs
- CompositionTarget.cs
- PatternMatcher.cs
- TrustSection.cs