Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / XLinq / System / Xml / Linq / XNodeValidator.cs / 1 / XNodeValidator.cs
using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics.CodeAnalysis; using System.Xml; using System.Xml.Linq; namespace System.Xml.Schema { internal class XNodeValidator { XmlSchemaSet schemas; ValidationEventHandler validationEventHandler; XObject source; bool addSchemaInfo; XmlNamespaceManager namespaceManager; XmlSchemaValidator validator; DictionaryschemaInfos; ArrayList defaultAttributes; XName xsiTypeName; XName xsiNilName; public XNodeValidator(XmlSchemaSet schemas, ValidationEventHandler validationEventHandler) { this.schemas = schemas; this.validationEventHandler = validationEventHandler; XNamespace xsi = XNamespace.Get("http://www.w3.org/2001/XMLSchema-instance"); xsiTypeName = xsi.GetName("type"); xsiNilName = xsi.GetName("nil"); } public void Validate(XObject source, XmlSchemaObject partialValidationType, bool addSchemaInfo) { this.source = source; this.addSchemaInfo = addSchemaInfo; XmlSchemaValidationFlags validationFlags = XmlSchemaValidationFlags.AllowXmlAttributes; XmlNodeType nt = source.NodeType; switch (nt) { case XmlNodeType.Document: source = ((XDocument)source).Root; if (source == null) throw new InvalidOperationException(Res.GetString(Res.InvalidOperation_MissingRoot)); validationFlags |= XmlSchemaValidationFlags.ProcessIdentityConstraints; break; case XmlNodeType.Element: break; case XmlNodeType.Attribute: if (((XAttribute)source).IsNamespaceDeclaration) goto default; if (source.Parent == null) throw new InvalidOperationException(Res.GetString(Res.InvalidOperation_MissingParent)); break; default: throw new InvalidOperationException(Res.GetString(Res.InvalidOperation_BadNodeType, nt)); } namespaceManager = new XmlNamespaceManager(schemas.NameTable); PushAncestorsAndSelf(source.Parent); validator = new XmlSchemaValidator(schemas.NameTable, schemas, namespaceManager, validationFlags); validator.ValidationEventHandler += new ValidationEventHandler(ValidationCallback); validator.XmlResolver = null; if (partialValidationType != null) { validator.Initialize(partialValidationType); } else { validator.Initialize(); } if (nt == XmlNodeType.Attribute) { ValidateAttribute((XAttribute)source); } else { ValidateElement((XElement)source); } validator.EndValidation(); } XmlSchemaInfo GetDefaultAttributeSchemaInfo(XmlSchemaAttribute sa) { XmlSchemaInfo si = new XmlSchemaInfo(); si.IsDefault = true; si.IsNil = false; si.SchemaAttribute = sa; XmlSchemaSimpleType st = sa.AttributeSchemaType; si.SchemaType = st; if (st.Datatype.Variety == XmlSchemaDatatypeVariety.Union) { string value = GetDefaultValue(sa); foreach (XmlSchemaSimpleType mt in ((XmlSchemaSimpleTypeUnion)st.Content).BaseMemberTypes) { object typedValue = null; try { typedValue = mt.Datatype.ParseValue(value, schemas.NameTable, namespaceManager); } catch (XmlSchemaException) { } if (typedValue != null) { si.MemberType = mt; break; } } } si.Validity = XmlSchemaValidity.Valid; return si; } string GetDefaultValue(XmlSchemaAttribute sa) { XmlQualifiedName name = sa.RefName; if (!name.IsEmpty) { sa = schemas.GlobalAttributes[name] as XmlSchemaAttribute; if (sa == null) return null; } string s = sa.FixedValue; if (s != null) return s; return sa.DefaultValue; } string GetDefaultValue(XmlSchemaElement se) { XmlQualifiedName name = se.RefName; if (!name.IsEmpty) { se = schemas.GlobalElements[name] as XmlSchemaElement; if (se == null) return null; } string s = se.FixedValue; if (s != null) return s; return se.DefaultValue; } void ReplaceSchemaInfo(XObject o, XmlSchemaInfo schemaInfo) { if (schemaInfos == null) { schemaInfos = new Dictionary (new XmlSchemaInfoEqualityComparer()); } XmlSchemaInfo si = o.Annotation (); if (si != null) { if (!schemaInfos.ContainsValue(si)) { schemaInfos.Add(si, si); } o.RemoveAnnotations (); } if (!schemaInfos.TryGetValue(schemaInfo, out si)) { si = schemaInfo; schemaInfos.Add(si, si); } o.AddAnnotation(si); } void PushAncestorsAndSelf(XElement e) { while (e != null) { XAttribute a = e.lastAttr; if (a != null) { do { a = a.next; if (a.IsNamespaceDeclaration) { string localName = a.Name.LocalName; if (localName == "xmlns") { localName = string.Empty; } if (!namespaceManager.HasNamespace(localName)) { namespaceManager.AddNamespace(localName, a.Value); } } } while (a != e.lastAttr); } e = e.parent as XElement; } } void PushElement(XElement e, ref string xsiType, ref string xsiNil) { namespaceManager.PushScope(); XAttribute a = e.lastAttr; if (a != null) { do { a = a.next; if (a.IsNamespaceDeclaration) { string localName = a.Name.LocalName; if (localName == "xmlns") { localName = string.Empty; } namespaceManager.AddNamespace(localName, a.Value); } else { XName name = a.Name; if (name == xsiTypeName) { xsiType = a.Value; } else if (name == xsiNilName) { xsiNil = a.Value; } } } while (a != e.lastAttr); } } void ValidateAttribute(XAttribute a) { XmlSchemaInfo si = addSchemaInfo ? new XmlSchemaInfo() : null; source = a; validator.ValidateAttribute(a.Name.LocalName, a.Name.NamespaceName, a.Value, si); if (addSchemaInfo) { ReplaceSchemaInfo(a, si); } } void ValidateAttributes(XElement e) { XAttribute a = e.lastAttr; if (a != null) { do { a = a.next; if (!a.IsNamespaceDeclaration) { ValidateAttribute(a); } } while (a != e.lastAttr); source = e; } if (addSchemaInfo) { if (defaultAttributes == null) { defaultAttributes = new ArrayList(); } else { defaultAttributes.Clear(); } validator.GetUnspecifiedDefaultAttributes(defaultAttributes); foreach (XmlSchemaAttribute sa in defaultAttributes) { a = new XAttribute(XNamespace.Get(sa.QualifiedName.Namespace).GetName(sa.QualifiedName.Name), GetDefaultValue(sa)); ReplaceSchemaInfo(a, GetDefaultAttributeSchemaInfo(sa)); e.Add(a); } } } void ValidateElement(XElement e) { XmlSchemaInfo si = addSchemaInfo ? new XmlSchemaInfo() : null; string xsiType = null; string xsiNil = null; PushElement(e, ref xsiType, ref xsiNil); source = e; validator.ValidateElement(e.Name.LocalName, e.Name.NamespaceName, si, xsiType, xsiNil, null, null); ValidateAttributes(e); validator.ValidateEndOfAttributes(si); ValidateNodes(e); validator.ValidateEndElement(si); if (addSchemaInfo) { if (si.Validity == XmlSchemaValidity.Valid && si.IsDefault) { e.Value = GetDefaultValue(si.SchemaElement); } ReplaceSchemaInfo(e, si); } namespaceManager.PopScope(); } void ValidateNodes(XElement e) { XNode n = e.content as XNode; if (n != null) { do { n = n.next; XElement c = n as XElement; if (c != null) { ValidateElement(c); } else { XText t = n as XText; if (t != null) { string s = t.Value; if (s.Length > 0) { validator.ValidateText(s); } } } } while (n != e.content); source = e; } else { string s = e.content as string; if (s != null && s.Length > 0) { validator.ValidateText(s); } } } void ValidationCallback(object sender, ValidationEventArgs e) { if (validationEventHandler != null) { validationEventHandler(source, e); } else if (e.Severity == XmlSeverityType.Error) { throw e.Exception; } } } internal class XmlSchemaInfoEqualityComparer : IEqualityComparer { public bool Equals(XmlSchemaInfo si1, XmlSchemaInfo si2) { if (si1 == si2) return true; if (si1 == null || si2 == null) return false; return si1.ContentType == si2.ContentType && si1.IsDefault == si2.IsDefault && si1.IsNil == si2.IsNil && (object)si1.MemberType == (object)si2.MemberType && (object)si1.SchemaAttribute == (object)si2.SchemaAttribute && (object)si1.SchemaElement == (object)si2.SchemaElement && (object)si1.SchemaType == (object)si2.SchemaType && si1.Validity == si2.Validity; } public int GetHashCode(XmlSchemaInfo si) { if (si == null) return 0; int h = (int)si.ContentType; if (si.IsDefault) { h ^= 1; } if (si.IsNil) { h ^= 1; } XmlSchemaSimpleType memberType = si.MemberType; if (memberType != null) { h ^= memberType.GetHashCode(); } XmlSchemaAttribute schemaAttribute = si.SchemaAttribute; if (schemaAttribute != null) { h ^= schemaAttribute.GetHashCode(); } XmlSchemaElement schemaElement = si.SchemaElement; if (schemaElement != null) { h ^= schemaElement.GetHashCode(); } XmlSchemaType schemaType = si.SchemaType; if (schemaType != null) { h ^= schemaType.GetHashCode(); } h ^= (int)si.Validity; return h; } } /// /// Extension methods /// public static class Extensions { ////// Gets the schema information that has been assigned to the /// Extension point [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "Reviewed by the design group.")] public static IXmlSchemaInfo GetSchemaInfo(this XElement source) { if (source == null) throw new ArgumentNullException("source"); return source.Annotationas a result of schema validation. /// (); } /// /// Gets the schema information that has been assigned to the /// Extension point [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "Reviewed by the design group.")] public static IXmlSchemaInfo GetSchemaInfo(this XAttribute source) { if (source == null) throw new ArgumentNullException("source"); return source.Annotationas a result of schema validation. /// (); } /// /// Validate a /// Extension point /// The/// used for validation /// The /// that receives schema validation warnings and errors encountered during schema /// validation public static void Validate(this XDocument source, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler) { source.Validate(schemas, validationEventHandler, false); } /// /// Validate a /// Extension point /// The/// used for validation /// The /// that receives schema validation warnings and errors encountered during schema /// validation /// If enabled the and the corresponding /// subtree is augmented with PSVI in the form of annotations, /// default attributes and default element values [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "Reviewed by the design group.")] public static void Validate(this XDocument source, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler, bool addSchemaInfo) { if (source == null) throw new ArgumentNullException("source"); if (schemas == null) throw new ArgumentNullException("schemas"); new XNodeValidator(schemas, validationEventHandler).Validate(source, null, addSchemaInfo); } /// /// Validate a /// Extension point /// An/// or /// object used to initialize the partial validation /// context /// The used for validation /// The that /// receives schema validation warnings and errors encountered during schema /// validation public static void Validate(this XElement source, XmlSchemaObject partialValidationType, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler) { source.Validate(partialValidationType, schemas, validationEventHandler, false); } /// /// Validate a /// Extension point /// An/// or /// object used to initialize the partial validation /// context /// The used for validation /// The that /// receives schema validation warnings and errors encountered during schema /// validation /// If enabled the and the corresponding /// subtree is augmented with PSVI in the form of annotations, /// default attributes and default element values [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "Reviewed by the design group.")] public static void Validate(this XElement source, XmlSchemaObject partialValidationType, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler, bool addSchemaInfo) { if (source == null) throw new ArgumentNullException("source"); if (partialValidationType == null) throw new ArgumentNullException("partialValidationType"); if (schemas == null) throw new ArgumentNullException("schemas"); new XNodeValidator(schemas, validationEventHandler).Validate(source, partialValidationType, addSchemaInfo); } /// /// Validate a /// Extension point /// An/// or /// object used to initialize the partial validation /// context /// The used for validation /// The that /// receives schema validation warnings and errors encountered during schema /// validation public static void Validate(this XAttribute source, XmlSchemaObject partialValidationType, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler) { source.Validate(partialValidationType, schemas, validationEventHandler, false); } /// /// Validate a /// Extension point /// An/// or /// object used to initialize the partial validation /// context /// The used for validation /// The that /// receives schema validation warnings and errors encountered during schema /// validation /// If enabled the is augmented with PSVI /// in the form of annotations, default attributes and /// default element values [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "Reviewed by the design group.")] public static void Validate(this XAttribute source, XmlSchemaObject partialValidationType, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler, bool addSchemaInfo) { if (source == null) throw new ArgumentNullException("source"); if (partialValidationType == null) throw new ArgumentNullException("partialValidationType"); if (schemas == null) throw new ArgumentNullException("schemas"); new XNodeValidator(schemas, validationEventHandler).Validate(source, partialValidationType, addSchemaInfo); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics.CodeAnalysis; using System.Xml; using System.Xml.Linq; namespace System.Xml.Schema { internal class XNodeValidator { XmlSchemaSet schemas; ValidationEventHandler validationEventHandler; XObject source; bool addSchemaInfo; XmlNamespaceManager namespaceManager; XmlSchemaValidator validator; Dictionary schemaInfos; ArrayList defaultAttributes; XName xsiTypeName; XName xsiNilName; public XNodeValidator(XmlSchemaSet schemas, ValidationEventHandler validationEventHandler) { this.schemas = schemas; this.validationEventHandler = validationEventHandler; XNamespace xsi = XNamespace.Get("http://www.w3.org/2001/XMLSchema-instance"); xsiTypeName = xsi.GetName("type"); xsiNilName = xsi.GetName("nil"); } public void Validate(XObject source, XmlSchemaObject partialValidationType, bool addSchemaInfo) { this.source = source; this.addSchemaInfo = addSchemaInfo; XmlSchemaValidationFlags validationFlags = XmlSchemaValidationFlags.AllowXmlAttributes; XmlNodeType nt = source.NodeType; switch (nt) { case XmlNodeType.Document: source = ((XDocument)source).Root; if (source == null) throw new InvalidOperationException(Res.GetString(Res.InvalidOperation_MissingRoot)); validationFlags |= XmlSchemaValidationFlags.ProcessIdentityConstraints; break; case XmlNodeType.Element: break; case XmlNodeType.Attribute: if (((XAttribute)source).IsNamespaceDeclaration) goto default; if (source.Parent == null) throw new InvalidOperationException(Res.GetString(Res.InvalidOperation_MissingParent)); break; default: throw new InvalidOperationException(Res.GetString(Res.InvalidOperation_BadNodeType, nt)); } namespaceManager = new XmlNamespaceManager(schemas.NameTable); PushAncestorsAndSelf(source.Parent); validator = new XmlSchemaValidator(schemas.NameTable, schemas, namespaceManager, validationFlags); validator.ValidationEventHandler += new ValidationEventHandler(ValidationCallback); validator.XmlResolver = null; if (partialValidationType != null) { validator.Initialize(partialValidationType); } else { validator.Initialize(); } if (nt == XmlNodeType.Attribute) { ValidateAttribute((XAttribute)source); } else { ValidateElement((XElement)source); } validator.EndValidation(); } XmlSchemaInfo GetDefaultAttributeSchemaInfo(XmlSchemaAttribute sa) { XmlSchemaInfo si = new XmlSchemaInfo(); si.IsDefault = true; si.IsNil = false; si.SchemaAttribute = sa; XmlSchemaSimpleType st = sa.AttributeSchemaType; si.SchemaType = st; if (st.Datatype.Variety == XmlSchemaDatatypeVariety.Union) { string value = GetDefaultValue(sa); foreach (XmlSchemaSimpleType mt in ((XmlSchemaSimpleTypeUnion)st.Content).BaseMemberTypes) { object typedValue = null; try { typedValue = mt.Datatype.ParseValue(value, schemas.NameTable, namespaceManager); } catch (XmlSchemaException) { } if (typedValue != null) { si.MemberType = mt; break; } } } si.Validity = XmlSchemaValidity.Valid; return si; } string GetDefaultValue(XmlSchemaAttribute sa) { XmlQualifiedName name = sa.RefName; if (!name.IsEmpty) { sa = schemas.GlobalAttributes[name] as XmlSchemaAttribute; if (sa == null) return null; } string s = sa.FixedValue; if (s != null) return s; return sa.DefaultValue; } string GetDefaultValue(XmlSchemaElement se) { XmlQualifiedName name = se.RefName; if (!name.IsEmpty) { se = schemas.GlobalElements[name] as XmlSchemaElement; if (se == null) return null; } string s = se.FixedValue; if (s != null) return s; return se.DefaultValue; } void ReplaceSchemaInfo(XObject o, XmlSchemaInfo schemaInfo) { if (schemaInfos == null) { schemaInfos = new Dictionary (new XmlSchemaInfoEqualityComparer()); } XmlSchemaInfo si = o.Annotation (); if (si != null) { if (!schemaInfos.ContainsValue(si)) { schemaInfos.Add(si, si); } o.RemoveAnnotations (); } if (!schemaInfos.TryGetValue(schemaInfo, out si)) { si = schemaInfo; schemaInfos.Add(si, si); } o.AddAnnotation(si); } void PushAncestorsAndSelf(XElement e) { while (e != null) { XAttribute a = e.lastAttr; if (a != null) { do { a = a.next; if (a.IsNamespaceDeclaration) { string localName = a.Name.LocalName; if (localName == "xmlns") { localName = string.Empty; } if (!namespaceManager.HasNamespace(localName)) { namespaceManager.AddNamespace(localName, a.Value); } } } while (a != e.lastAttr); } e = e.parent as XElement; } } void PushElement(XElement e, ref string xsiType, ref string xsiNil) { namespaceManager.PushScope(); XAttribute a = e.lastAttr; if (a != null) { do { a = a.next; if (a.IsNamespaceDeclaration) { string localName = a.Name.LocalName; if (localName == "xmlns") { localName = string.Empty; } namespaceManager.AddNamespace(localName, a.Value); } else { XName name = a.Name; if (name == xsiTypeName) { xsiType = a.Value; } else if (name == xsiNilName) { xsiNil = a.Value; } } } while (a != e.lastAttr); } } void ValidateAttribute(XAttribute a) { XmlSchemaInfo si = addSchemaInfo ? new XmlSchemaInfo() : null; source = a; validator.ValidateAttribute(a.Name.LocalName, a.Name.NamespaceName, a.Value, si); if (addSchemaInfo) { ReplaceSchemaInfo(a, si); } } void ValidateAttributes(XElement e) { XAttribute a = e.lastAttr; if (a != null) { do { a = a.next; if (!a.IsNamespaceDeclaration) { ValidateAttribute(a); } } while (a != e.lastAttr); source = e; } if (addSchemaInfo) { if (defaultAttributes == null) { defaultAttributes = new ArrayList(); } else { defaultAttributes.Clear(); } validator.GetUnspecifiedDefaultAttributes(defaultAttributes); foreach (XmlSchemaAttribute sa in defaultAttributes) { a = new XAttribute(XNamespace.Get(sa.QualifiedName.Namespace).GetName(sa.QualifiedName.Name), GetDefaultValue(sa)); ReplaceSchemaInfo(a, GetDefaultAttributeSchemaInfo(sa)); e.Add(a); } } } void ValidateElement(XElement e) { XmlSchemaInfo si = addSchemaInfo ? new XmlSchemaInfo() : null; string xsiType = null; string xsiNil = null; PushElement(e, ref xsiType, ref xsiNil); source = e; validator.ValidateElement(e.Name.LocalName, e.Name.NamespaceName, si, xsiType, xsiNil, null, null); ValidateAttributes(e); validator.ValidateEndOfAttributes(si); ValidateNodes(e); validator.ValidateEndElement(si); if (addSchemaInfo) { if (si.Validity == XmlSchemaValidity.Valid && si.IsDefault) { e.Value = GetDefaultValue(si.SchemaElement); } ReplaceSchemaInfo(e, si); } namespaceManager.PopScope(); } void ValidateNodes(XElement e) { XNode n = e.content as XNode; if (n != null) { do { n = n.next; XElement c = n as XElement; if (c != null) { ValidateElement(c); } else { XText t = n as XText; if (t != null) { string s = t.Value; if (s.Length > 0) { validator.ValidateText(s); } } } } while (n != e.content); source = e; } else { string s = e.content as string; if (s != null && s.Length > 0) { validator.ValidateText(s); } } } void ValidationCallback(object sender, ValidationEventArgs e) { if (validationEventHandler != null) { validationEventHandler(source, e); } else if (e.Severity == XmlSeverityType.Error) { throw e.Exception; } } } internal class XmlSchemaInfoEqualityComparer : IEqualityComparer { public bool Equals(XmlSchemaInfo si1, XmlSchemaInfo si2) { if (si1 == si2) return true; if (si1 == null || si2 == null) return false; return si1.ContentType == si2.ContentType && si1.IsDefault == si2.IsDefault && si1.IsNil == si2.IsNil && (object)si1.MemberType == (object)si2.MemberType && (object)si1.SchemaAttribute == (object)si2.SchemaAttribute && (object)si1.SchemaElement == (object)si2.SchemaElement && (object)si1.SchemaType == (object)si2.SchemaType && si1.Validity == si2.Validity; } public int GetHashCode(XmlSchemaInfo si) { if (si == null) return 0; int h = (int)si.ContentType; if (si.IsDefault) { h ^= 1; } if (si.IsNil) { h ^= 1; } XmlSchemaSimpleType memberType = si.MemberType; if (memberType != null) { h ^= memberType.GetHashCode(); } XmlSchemaAttribute schemaAttribute = si.SchemaAttribute; if (schemaAttribute != null) { h ^= schemaAttribute.GetHashCode(); } XmlSchemaElement schemaElement = si.SchemaElement; if (schemaElement != null) { h ^= schemaElement.GetHashCode(); } XmlSchemaType schemaType = si.SchemaType; if (schemaType != null) { h ^= schemaType.GetHashCode(); } h ^= (int)si.Validity; return h; } } /// /// Extension methods /// public static class Extensions { ////// Gets the schema information that has been assigned to the /// Extension point [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "Reviewed by the design group.")] public static IXmlSchemaInfo GetSchemaInfo(this XElement source) { if (source == null) throw new ArgumentNullException("source"); return source.Annotationas a result of schema validation. /// (); } /// /// Gets the schema information that has been assigned to the /// Extension point [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "Reviewed by the design group.")] public static IXmlSchemaInfo GetSchemaInfo(this XAttribute source) { if (source == null) throw new ArgumentNullException("source"); return source.Annotationas a result of schema validation. /// (); } /// /// Validate a /// Extension point /// The/// used for validation /// The /// that receives schema validation warnings and errors encountered during schema /// validation public static void Validate(this XDocument source, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler) { source.Validate(schemas, validationEventHandler, false); } /// /// Validate a /// Extension point /// The/// used for validation /// The /// that receives schema validation warnings and errors encountered during schema /// validation /// If enabled the and the corresponding /// subtree is augmented with PSVI in the form of annotations, /// default attributes and default element values [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "Reviewed by the design group.")] public static void Validate(this XDocument source, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler, bool addSchemaInfo) { if (source == null) throw new ArgumentNullException("source"); if (schemas == null) throw new ArgumentNullException("schemas"); new XNodeValidator(schemas, validationEventHandler).Validate(source, null, addSchemaInfo); } /// /// Validate a /// Extension point /// An/// or /// object used to initialize the partial validation /// context /// The used for validation /// The that /// receives schema validation warnings and errors encountered during schema /// validation public static void Validate(this XElement source, XmlSchemaObject partialValidationType, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler) { source.Validate(partialValidationType, schemas, validationEventHandler, false); } /// /// Validate a /// Extension point /// An/// or /// object used to initialize the partial validation /// context /// The used for validation /// The that /// receives schema validation warnings and errors encountered during schema /// validation /// If enabled the and the corresponding /// subtree is augmented with PSVI in the form of annotations, /// default attributes and default element values [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "Reviewed by the design group.")] public static void Validate(this XElement source, XmlSchemaObject partialValidationType, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler, bool addSchemaInfo) { if (source == null) throw new ArgumentNullException("source"); if (partialValidationType == null) throw new ArgumentNullException("partialValidationType"); if (schemas == null) throw new ArgumentNullException("schemas"); new XNodeValidator(schemas, validationEventHandler).Validate(source, partialValidationType, addSchemaInfo); } /// /// Validate a /// Extension point /// An/// or /// object used to initialize the partial validation /// context /// The used for validation /// The that /// receives schema validation warnings and errors encountered during schema /// validation public static void Validate(this XAttribute source, XmlSchemaObject partialValidationType, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler) { source.Validate(partialValidationType, schemas, validationEventHandler, false); } /// /// Validate a /// Extension point /// An/// or /// object used to initialize the partial validation /// context /// The used for validation /// The that /// receives schema validation warnings and errors encountered during schema /// validation /// If enabled the is augmented with PSVI /// in the form of annotations, default attributes and /// default element values [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "Reviewed by the design group.")] public static void Validate(this XAttribute source, XmlSchemaObject partialValidationType, XmlSchemaSet schemas, ValidationEventHandler validationEventHandler, bool addSchemaInfo) { if (source == null) throw new ArgumentNullException("source"); if (partialValidationType == null) throw new ArgumentNullException("partialValidationType"); if (schemas == null) throw new ArgumentNullException("schemas"); new XNodeValidator(schemas, validationEventHandler).Validate(source, partialValidationType, addSchemaInfo); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
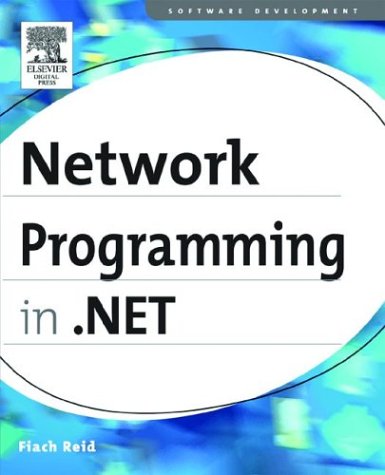
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbConnectionPoolCounters.cs
- InputReportEventArgs.cs
- ContainerActivationHelper.cs
- CookielessHelper.cs
- _BaseOverlappedAsyncResult.cs
- HMACMD5.cs
- SqlAliasesReferenced.cs
- ConditionalDesigner.cs
- LayoutUtils.cs
- ObjectResult.cs
- AdRotator.cs
- DecimalAnimation.cs
- Identity.cs
- ListBoxItemAutomationPeer.cs
- SqlUserDefinedAggregateAttribute.cs
- SeparatorAutomationPeer.cs
- SettingsSavedEventArgs.cs
- WebPartRestoreVerb.cs
- EntryPointNotFoundException.cs
- _AutoWebProxyScriptEngine.cs
- ControllableStoryboardAction.cs
- FrameworkElement.cs
- DataControlFieldCollection.cs
- ConnectivityStatus.cs
- WebPartConnectVerb.cs
- CryptoConfig.cs
- ConstNode.cs
- SendMailErrorEventArgs.cs
- TextRangeEditLists.cs
- externdll.cs
- Interlocked.cs
- RemoteCryptoRsaServiceProvider.cs
- ValueQuery.cs
- XmlSchemaValidationException.cs
- RSAPKCS1KeyExchangeFormatter.cs
- DocumentXmlWriter.cs
- AuthenticationService.cs
- ExpressionBinding.cs
- HotCommands.cs
- ProfilePropertyNameValidator.cs
- ConfigurationSectionCollection.cs
- XmlTextAttribute.cs
- ValidatorUtils.cs
- ResumeStoryboard.cs
- TemplateLookupAction.cs
- GeometryModel3D.cs
- NavigationProperty.cs
- AdvancedBindingPropertyDescriptor.cs
- AssemblyNameProxy.cs
- Section.cs
- CodeTypeDeclaration.cs
- WhitespaceSignificantCollectionAttribute.cs
- EventRouteFactory.cs
- Helpers.cs
- SignalGate.cs
- ClusterUtils.cs
- SrgsElementFactoryCompiler.cs
- SizeFConverter.cs
- AbandonedMutexException.cs
- IntSecurity.cs
- PageThemeCodeDomTreeGenerator.cs
- EntryIndex.cs
- AutoResetEvent.cs
- SoapMessage.cs
- RolePrincipal.cs
- Common.cs
- contentDescriptor.cs
- QuadraticBezierSegment.cs
- XmlnsCompatibleWithAttribute.cs
- ListBase.cs
- DataGridViewCheckBoxCell.cs
- NegatedConstant.cs
- DataContractSerializerOperationFormatter.cs
- DebugHandleTracker.cs
- TrackingStringDictionary.cs
- HyperLinkStyle.cs
- InvalidComObjectException.cs
- OdbcReferenceCollection.cs
- CodeDomDesignerLoader.cs
- AsymmetricAlgorithm.cs
- DynamicVirtualDiscoSearcher.cs
- GeneralTransform3D.cs
- SingleResultAttribute.cs
- DataContractJsonSerializerOperationBehavior.cs
- SqlCacheDependency.cs
- Logging.cs
- View.cs
- ElapsedEventArgs.cs
- Command.cs
- Quaternion.cs
- DataGridViewRowStateChangedEventArgs.cs
- MatchAttribute.cs
- FixedTextView.cs
- InstallerTypeAttribute.cs
- ErrorView.xaml.cs
- ServerIdentity.cs
- EdmType.cs
- CommandEventArgs.cs
- SequenceDesigner.cs
- KeyPullup.cs