Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / Common.cs / 1305376 / Common.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Common defintions and functions for the server and client lib // //--------------------------------------------------------------------- #if ASTORIA_CLIENT namespace System.Data.Services.Client #else namespace System.Data.Services #endif { using System.Linq; using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; using System.Xml; ////// Common defintions and functions for the server and client lib /// internal static class CommonUtil { ////// List of types unsupported by the client /// private static readonly Type[] unsupportedTypes = new Type[] { #if !ASTORIA_LIGHT // System.Dynamic & tuples not available (as of SL 2.0) typeof(System.Dynamic.IDynamicMetaObjectProvider), typeof(System.Tuple<>), // 1-Tuple typeof(System.Tuple<,>), // 2-Tuple typeof(System.Tuple<,,>), // 3-Tuple typeof(System.Tuple<,,,>), // 4-Tuple typeof(System.Tuple<,,,,>), // 5-Tuple typeof(System.Tuple<,,,,,>), // 6-Tuple typeof(System.Tuple<,,,,,,>), // 7-Tuple typeof(System.Tuple<,,,,,,,>) // 8-Tuple #endif }; ////// Test whether a type is unsupported by the client lib /// /// The type to test ///Returns true if the type is not supported internal static bool IsUnsupportedType(Type type) { if (type.IsGenericType) { type = type.GetGenericTypeDefinition(); } if (unsupportedTypes.Any(t => t.IsAssignableFrom(type))) { return true; } Debug.Assert(!type.FullName.StartsWith("System.Tuple", StringComparison.Ordinal), "System.Tuple is not blocked by unsupported type check"); return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Common defintions and functions for the server and client lib // //--------------------------------------------------------------------- #if ASTORIA_CLIENT namespace System.Data.Services.Client #else namespace System.Data.Services #endif { using System.Linq; using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; using System.Xml; ////// Common defintions and functions for the server and client lib /// internal static class CommonUtil { ////// List of types unsupported by the client /// private static readonly Type[] unsupportedTypes = new Type[] { #if !ASTORIA_LIGHT // System.Dynamic & tuples not available (as of SL 2.0) typeof(System.Dynamic.IDynamicMetaObjectProvider), typeof(System.Tuple<>), // 1-Tuple typeof(System.Tuple<,>), // 2-Tuple typeof(System.Tuple<,,>), // 3-Tuple typeof(System.Tuple<,,,>), // 4-Tuple typeof(System.Tuple<,,,,>), // 5-Tuple typeof(System.Tuple<,,,,,>), // 6-Tuple typeof(System.Tuple<,,,,,,>), // 7-Tuple typeof(System.Tuple<,,,,,,,>) // 8-Tuple #endif }; ////// Test whether a type is unsupported by the client lib /// /// The type to test ///Returns true if the type is not supported internal static bool IsUnsupportedType(Type type) { if (type.IsGenericType) { type = type.GetGenericTypeDefinition(); } if (unsupportedTypes.Any(t => t.IsAssignableFrom(type))) { return true; } Debug.Assert(!type.FullName.StartsWith("System.Tuple", StringComparison.Ordinal), "System.Tuple is not blocked by unsupported type check"); return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
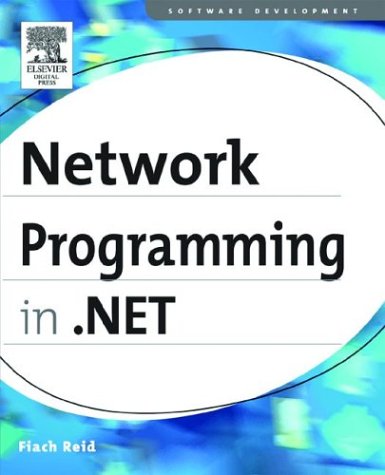
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewItem.cs
- FrameworkContentElementAutomationPeer.cs
- Section.cs
- StringArrayConverter.cs
- CompilerScopeManager.cs
- RadioButtonAutomationPeer.cs
- CodeMethodReturnStatement.cs
- InvalidPropValue.cs
- ApplicationException.cs
- IpcChannelHelper.cs
- AspProxy.cs
- TextSearch.cs
- WorkflowMessageEventArgs.cs
- ObjectHelper.cs
- DataProviderNameConverter.cs
- WebPartDisplayMode.cs
- ExtensionsSection.cs
- ZipIOExtraField.cs
- DateBoldEvent.cs
- SplitterDesigner.cs
- TextSelection.cs
- UnsafeNativeMethods.cs
- SystemIPv6InterfaceProperties.cs
- ServiceModelEnumValidatorAttribute.cs
- XmlSecureResolver.cs
- GeneralTransform3DCollection.cs
- SqlCacheDependencyDatabaseCollection.cs
- RowCache.cs
- KeyEvent.cs
- MenuTracker.cs
- TraceProvider.cs
- EventMappingSettingsCollection.cs
- XPathNodePointer.cs
- TypeDescriptionProvider.cs
- PartialList.cs
- View.cs
- SimpleLine.cs
- SystemResources.cs
- Point.cs
- GridViewDesigner.cs
- Encoder.cs
- QuotedPrintableStream.cs
- ProcessModuleCollection.cs
- MembershipValidatePasswordEventArgs.cs
- AnimatedTypeHelpers.cs
- CodeSnippetTypeMember.cs
- BasicViewGenerator.cs
- Literal.cs
- FormView.cs
- HandlerFactoryCache.cs
- TypeBuilderInstantiation.cs
- SectionUpdates.cs
- StyleHelper.cs
- IconHelper.cs
- UiaCoreTypesApi.cs
- SymmetricAlgorithm.cs
- DataGridViewLinkCell.cs
- ColorContextHelper.cs
- SqlCacheDependencySection.cs
- ToolStripContentPanelRenderEventArgs.cs
- ProxyHwnd.cs
- Zone.cs
- Parser.cs
- ActivityDesignerResources.cs
- RootBuilder.cs
- SecurityContext.cs
- CompositeDesignerAccessibleObject.cs
- ELinqQueryState.cs
- XsltException.cs
- SourceItem.cs
- HitTestResult.cs
- Freezable.cs
- BulletedList.cs
- ReadOnlyObservableCollection.cs
- MemberProjectionIndex.cs
- cookie.cs
- TextBoxLine.cs
- TabControlCancelEvent.cs
- RectAnimationUsingKeyFrames.cs
- PropertiesTab.cs
- TabControl.cs
- DeviceContexts.cs
- XmlSchemaNotation.cs
- IxmlLineInfo.cs
- CodeCastExpression.cs
- COM2PropertyBuilderUITypeEditor.cs
- ProxyGenerationError.cs
- ParallelDesigner.cs
- TextRange.cs
- InvokePatternIdentifiers.cs
- MappingItemCollection.cs
- DataSourceControlBuilder.cs
- CodeAccessPermission.cs
- SettingsProviderCollection.cs
- TypeInitializationException.cs
- WindowsEditBoxRange.cs
- Misc.cs
- TextEditorDragDrop.cs
- DataGridViewAutoSizeModeEventArgs.cs
- DiscoveryClientDocuments.cs