Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Base / System / Windows / Point.cs / 1 / Point.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001, 2002 // // File: Point.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Text; using System.Collections; using System.Globalization; using System.Windows; using System.Windows.Media; using System.Runtime.InteropServices; namespace System.Windows { ////// Point - Defaults to 0,0 /// public partial struct Point { #region Constructors ////// Constructor which accepts the X and Y values /// /// The value for the X coordinate of the new Point /// The value for the Y coordinate of the new Point public Point(double x, double y) { _x = x; _y = y; } #endregion Constructors #region Public Methods ////// Offset - update the location by adding offsetX to X and offsetY to Y /// /// The offset in the x dimension /// The offset in the y dimension public void Offset(double offsetX, double offsetY) { _x += offsetX; _y += offsetY; } ////// Operator Point + Vector /// ////// Point - The result of the addition /// /// The Point to be added to the Vector /// The Vectr to be added to the Point public static Point operator + (Point point, Vector vector) { return new Point(point._x + vector._x, point._y + vector._y); } ////// Add: Point + Vector /// ////// Point - The result of the addition /// /// The Point to be added to the Vector /// The Vector to be added to the Point public static Point Add(Point point, Vector vector) { return new Point(point._x + vector._x, point._y + vector._y); } ////// Operator Point - Vector /// ////// Point - The result of the subtraction /// /// The Point from which the Vector is subtracted /// The Vector which is subtracted from the Point public static Point operator - (Point point, Vector vector) { return new Point(point._x - vector._x, point._y - vector._y); } ////// Subtract: Point - Vector /// ////// Point - The result of the subtraction /// /// The Point from which the Vector is subtracted /// The Vector which is subtracted from the Point public static Point Subtract(Point point, Vector vector) { return new Point(point._x - vector._x, point._y - vector._y); } ////// Operator Point - Point /// ////// Vector - The result of the subtraction /// /// The Point from which point2 is subtracted /// The Point subtracted from point1 public static Vector operator - (Point point1, Point point2) { return new Vector(point1._x - point2._x, point1._y - point2._y); } ////// Subtract: Point - Point /// ////// Vector - The result of the subtraction /// /// The Point from which point2 is subtracted /// The Point subtracted from point1 public static Vector Subtract(Point point1, Point point2) { return new Vector(point1._x - point2._x, point1._y - point2._y); } ////// Operator Point * Matrix /// public static Point operator * (Point point, Matrix matrix) { return matrix.Transform(point); } ////// Multiply: Point * Matrix /// public static Point Multiply(Point point, Matrix matrix) { return matrix.Transform(point); } ////// Explicit conversion to Size. Note that since Size cannot contain negative values, /// the resulting size will contains the absolute values of X and Y /// ////// Size - A Size equal to this Point /// /// Point - the Point to convert to a Size public static explicit operator Size(Point point) { return new Size(Math.Abs(point._x), Math.Abs(point._y)); } ////// Explicit conversion to Vector /// ////// Vector - A Vector equal to this Point /// /// Point - the Point to convert to a Vector public static explicit operator Vector(Point point) { return new Vector(point._x, point._y); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001, 2002 // // File: Point.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Text; using System.Collections; using System.Globalization; using System.Windows; using System.Windows.Media; using System.Runtime.InteropServices; namespace System.Windows { ////// Point - Defaults to 0,0 /// public partial struct Point { #region Constructors ////// Constructor which accepts the X and Y values /// /// The value for the X coordinate of the new Point /// The value for the Y coordinate of the new Point public Point(double x, double y) { _x = x; _y = y; } #endregion Constructors #region Public Methods ////// Offset - update the location by adding offsetX to X and offsetY to Y /// /// The offset in the x dimension /// The offset in the y dimension public void Offset(double offsetX, double offsetY) { _x += offsetX; _y += offsetY; } ////// Operator Point + Vector /// ////// Point - The result of the addition /// /// The Point to be added to the Vector /// The Vectr to be added to the Point public static Point operator + (Point point, Vector vector) { return new Point(point._x + vector._x, point._y + vector._y); } ////// Add: Point + Vector /// ////// Point - The result of the addition /// /// The Point to be added to the Vector /// The Vector to be added to the Point public static Point Add(Point point, Vector vector) { return new Point(point._x + vector._x, point._y + vector._y); } ////// Operator Point - Vector /// ////// Point - The result of the subtraction /// /// The Point from which the Vector is subtracted /// The Vector which is subtracted from the Point public static Point operator - (Point point, Vector vector) { return new Point(point._x - vector._x, point._y - vector._y); } ////// Subtract: Point - Vector /// ////// Point - The result of the subtraction /// /// The Point from which the Vector is subtracted /// The Vector which is subtracted from the Point public static Point Subtract(Point point, Vector vector) { return new Point(point._x - vector._x, point._y - vector._y); } ////// Operator Point - Point /// ////// Vector - The result of the subtraction /// /// The Point from which point2 is subtracted /// The Point subtracted from point1 public static Vector operator - (Point point1, Point point2) { return new Vector(point1._x - point2._x, point1._y - point2._y); } ////// Subtract: Point - Point /// ////// Vector - The result of the subtraction /// /// The Point from which point2 is subtracted /// The Point subtracted from point1 public static Vector Subtract(Point point1, Point point2) { return new Vector(point1._x - point2._x, point1._y - point2._y); } ////// Operator Point * Matrix /// public static Point operator * (Point point, Matrix matrix) { return matrix.Transform(point); } ////// Multiply: Point * Matrix /// public static Point Multiply(Point point, Matrix matrix) { return matrix.Transform(point); } ////// Explicit conversion to Size. Note that since Size cannot contain negative values, /// the resulting size will contains the absolute values of X and Y /// ////// Size - A Size equal to this Point /// /// Point - the Point to convert to a Size public static explicit operator Size(Point point) { return new Size(Math.Abs(point._x), Math.Abs(point._y)); } ////// Explicit conversion to Vector /// ////// Vector - A Vector equal to this Point /// /// Point - the Point to convert to a Vector public static explicit operator Vector(Point point) { return new Vector(point._x, point._y); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
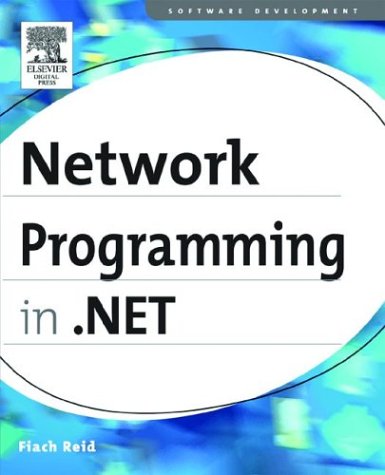
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- mansign.cs
- UnsafeNativeMethodsTablet.cs
- AlternateViewCollection.cs
- PageThemeParser.cs
- CreateUserWizard.cs
- Avt.cs
- SQLGuid.cs
- Base64Decoder.cs
- ApplyImportsAction.cs
- SelectionChangedEventArgs.cs
- ClickablePoint.cs
- MatrixCamera.cs
- ETagAttribute.cs
- ColumnResult.cs
- RegionInfo.cs
- Polygon.cs
- Light.cs
- XPathPatternParser.cs
- ListViewInsertedEventArgs.cs
- SQLUtility.cs
- PersonalizableAttribute.cs
- RtfToXamlReader.cs
- WizardSideBarListControlItemEventArgs.cs
- MeasureItemEvent.cs
- SingleSelectRootGridEntry.cs
- SqlAliaser.cs
- SmiConnection.cs
- BindingGroup.cs
- diagnosticsswitches.cs
- Filter.cs
- TypeConverterAttribute.cs
- PackageDigitalSignatureManager.cs
- XmlSignatureProperties.cs
- GacUtil.cs
- DNS.cs
- WorkflowServiceInstance.cs
- SymLanguageType.cs
- HwndStylusInputProvider.cs
- RegexInterpreter.cs
- XamlReaderHelper.cs
- Stylus.cs
- HttpValueCollection.cs
- Stylesheet.cs
- DataListCommandEventArgs.cs
- Table.cs
- _NegoState.cs
- HandlerMappingMemo.cs
- AddInServer.cs
- DetailsViewDeleteEventArgs.cs
- ProviderIncompatibleException.cs
- ScriptResourceAttribute.cs
- ScriptMethodAttribute.cs
- _NegotiateClient.cs
- CustomCategoryAttribute.cs
- WebPartHelpVerb.cs
- PublisherIdentityPermission.cs
- HttpModuleAction.cs
- TcpStreams.cs
- DelegateArgumentValue.cs
- XsltOutput.cs
- Automation.cs
- Point3DCollection.cs
- loginstatus.cs
- Unit.cs
- DataGridViewMethods.cs
- PasswordBox.cs
- QilStrConcat.cs
- CursorInteropHelper.cs
- EntityException.cs
- FlowLayoutSettings.cs
- CacheChildrenQuery.cs
- BitVec.cs
- BaseProcessor.cs
- ReservationCollection.cs
- EdmError.cs
- InputMethod.cs
- RectIndependentAnimationStorage.cs
- CounterSample.cs
- XmlValidatingReader.cs
- HtmlControl.cs
- CodeTypeDelegate.cs
- BatchServiceHost.cs
- NullableBoolConverter.cs
- AddingNewEventArgs.cs
- SingleAnimationBase.cs
- ListBox.cs
- AccessDataSourceView.cs
- FontUnitConverter.cs
- AsymmetricKeyExchangeFormatter.cs
- rsa.cs
- WindowsGrip.cs
- ContractMapping.cs
- SurrogateEncoder.cs
- WebBrowserProgressChangedEventHandler.cs
- DetailsViewModeEventArgs.cs
- GenericAuthenticationEventArgs.cs
- ContextProperty.cs
- CacheOutputQuery.cs
- MatchingStyle.cs
- SystemDiagnosticsSection.cs