Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / xsp / System / Web / Extensions / Compilation / WCFModel / ProxyGenerationError.cs / 1 / ProxyGenerationError.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- // // This code is shared between ndp\fx\src\xsp\System\Web\Extensions\Compilation\WCFModel // and wizard\vsdesigner\designer\microsoft\vsdesigner\WCFModel. // Please make sure the code files between those two directories are alway in [....] when you make any changes to this code. // And always test these code in both places before check in. // The code under ndp\fx\src\xsp\System\Web\Extensions\Compilation\XmlSerializer might have to be regerenated when // the format of the svcmap file is changed, or class structure has been changed in this directory. Please follow the HowTo file // under Compilation directory to see how to regerenate that code. // using System; using System.Diagnostics; using System.Globalization; using System.IO; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Configuration; using System.ServiceModel.Description; using System.Xml; using System.Xml.Schema; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// This class represents an error message happens when we generate code /// ///#if WEB_EXTENSIONS_CODE internal class ProxyGenerationError #else [CLSCompliant(true)] public class ProxyGenerationError #endif { private bool m_IsWarning; private string m_Message; private string m_MetadataFile; private int m_LineNumber; private int m_LinePosition; private GeneratorState m_ErrorGeneratorState; /// /// Constructor /// /// MetadataConversionError ///public ProxyGenerationError(MetadataConversionError errorMessage) { m_ErrorGeneratorState = GeneratorState.GenerateCode; m_IsWarning = errorMessage.IsWarning; m_Message = errorMessage.Message; m_MetadataFile = String.Empty; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An IOException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, Exception errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An IOException /// An IOException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, Exception errorException, bool isWarning) { m_ErrorGeneratorState = generatorState; m_IsWarning = isWarning; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlException errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// Constructor /// /// /// /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlSchemaException errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// Constructor /// /// /// /// An XmlException /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlSchemaException errorException, bool isWarning) { m_ErrorGeneratorState = generatorState; m_IsWarning = isWarning; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// This property represents when an error message happens /// ////// public GeneratorState ErrorGeneratorState { get { return m_ErrorGeneratorState; } } /// /// True: if it is a warning message, otherwise, an error /// ////// public bool IsWarning { get { return m_IsWarning; } } /// /// Line Number when error happens /// ////// public int LineNumber { get { return m_LineNumber; } } /// /// Column Number in the line when error happens /// ////// public int LinePosition { get { return m_LinePosition; } } /// /// return the error message /// ////// public string Message { get { return m_Message; } } /// /// return the error message /// ////// public string MetadataFile { get { return m_MetadataFile; } } /// /// This enum represents when an error message happens /// ///public enum GeneratorState { LoadMetadata = 0, MergeMetadata = 1, GenerateCode = 2, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- // // This code is shared between ndp\fx\src\xsp\System\Web\Extensions\Compilation\WCFModel // and wizard\vsdesigner\designer\microsoft\vsdesigner\WCFModel. // Please make sure the code files between those two directories are alway in [....] when you make any changes to this code. // And always test these code in both places before check in. // The code under ndp\fx\src\xsp\System\Web\Extensions\Compilation\XmlSerializer might have to be regerenated when // the format of the svcmap file is changed, or class structure has been changed in this directory. Please follow the HowTo file // under Compilation directory to see how to regerenate that code. // using System; using System.Diagnostics; using System.Globalization; using System.IO; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Configuration; using System.ServiceModel.Description; using System.Xml; using System.Xml.Schema; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// This class represents an error message happens when we generate code /// ///#if WEB_EXTENSIONS_CODE internal class ProxyGenerationError #else [CLSCompliant(true)] public class ProxyGenerationError #endif { private bool m_IsWarning; private string m_Message; private string m_MetadataFile; private int m_LineNumber; private int m_LinePosition; private GeneratorState m_ErrorGeneratorState; /// /// Constructor /// /// MetadataConversionError ///public ProxyGenerationError(MetadataConversionError errorMessage) { m_ErrorGeneratorState = GeneratorState.GenerateCode; m_IsWarning = errorMessage.IsWarning; m_Message = errorMessage.Message; m_MetadataFile = String.Empty; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An IOException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, Exception errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An IOException /// An IOException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, Exception errorException, bool isWarning) { m_ErrorGeneratorState = generatorState; m_IsWarning = isWarning; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlException errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// Constructor /// /// /// /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlSchemaException errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// Constructor /// /// /// /// An XmlException /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlSchemaException errorException, bool isWarning) { m_ErrorGeneratorState = generatorState; m_IsWarning = isWarning; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// This property represents when an error message happens /// ////// public GeneratorState ErrorGeneratorState { get { return m_ErrorGeneratorState; } } /// /// True: if it is a warning message, otherwise, an error /// ////// public bool IsWarning { get { return m_IsWarning; } } /// /// Line Number when error happens /// ////// public int LineNumber { get { return m_LineNumber; } } /// /// Column Number in the line when error happens /// ////// public int LinePosition { get { return m_LinePosition; } } /// /// return the error message /// ////// public string Message { get { return m_Message; } } /// /// return the error message /// ////// public string MetadataFile { get { return m_MetadataFile; } } /// /// This enum represents when an error message happens /// ///public enum GeneratorState { LoadMetadata = 0, MergeMetadata = 1, GenerateCode = 2, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
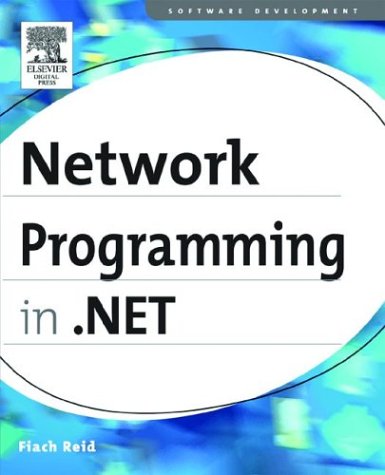
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextServicesManager.cs
- UriSection.cs
- SystemColorTracker.cs
- MachineSettingsSection.cs
- DigitShape.cs
- _NTAuthentication.cs
- ZipIOCentralDirectoryBlock.cs
- ToolStripContainer.cs
- COMException.cs
- BinaryMessageEncodingElement.cs
- Encoding.cs
- PageTheme.cs
- SecurityContext.cs
- SingleConverter.cs
- DateTimeFormatInfo.cs
- SHA1.cs
- SafeCryptContextHandle.cs
- VarRemapper.cs
- NoClickablePointException.cs
- HostingEnvironment.cs
- DbExpressionVisitor_TResultType.cs
- itemelement.cs
- WmiInstallComponent.cs
- FieldTemplateFactory.cs
- SmtpReplyReader.cs
- Int64Storage.cs
- QualifierSet.cs
- ButtonStandardAdapter.cs
- ProtocolsConfigurationEntry.cs
- SoapAttributeOverrides.cs
- ProfileServiceManager.cs
- LocationSectionRecord.cs
- PropertyHelper.cs
- TextBoxBase.cs
- XmlDataSourceView.cs
- typedescriptorpermissionattribute.cs
- SessionState.cs
- InputLanguageSource.cs
- CookieHandler.cs
- WindowsComboBox.cs
- RawStylusInputCustomDataList.cs
- OracleMonthSpan.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- CodeActivity.cs
- ObjectDataSourceMethodEventArgs.cs
- CodeTypeReferenceCollection.cs
- HtmlInputPassword.cs
- EdmSchemaError.cs
- WebPartCancelEventArgs.cs
- DynamicDiscoveryDocument.cs
- XmlSchemaParticle.cs
- SchemaCollectionPreprocessor.cs
- LateBoundBitmapDecoder.cs
- UIInitializationException.cs
- LambdaCompiler.Expressions.cs
- DecimalAnimationBase.cs
- NullReferenceException.cs
- SrgsGrammarCompiler.cs
- RemoteArgument.cs
- EncodingNLS.cs
- DBSchemaRow.cs
- ClonableStack.cs
- XsltContext.cs
- ConfigurationProperty.cs
- EntityClientCacheKey.cs
- RuntimeEnvironment.cs
- DataConnectionHelper.cs
- ExceptionHelpers.cs
- SevenBitStream.cs
- Pointer.cs
- SettingsPropertyValue.cs
- XmlSerializationReader.cs
- ReadOnlyCollection.cs
- ValidationResult.cs
- TransactionWaitAsyncResult.cs
- UnsafeNativeMethods.cs
- IDQuery.cs
- MasterPageBuildProvider.cs
- PeerCollaborationPermission.cs
- OLEDB_Util.cs
- OdbcErrorCollection.cs
- BookmarkUndoUnit.cs
- XmlSchemaSimpleContentExtension.cs
- WSSecurityPolicy.cs
- XmlDigitalSignatureProcessor.cs
- Button.cs
- RawStylusActions.cs
- Rule.cs
- Geometry.cs
- OleAutBinder.cs
- GeneralTransform3DCollection.cs
- DetailsViewUpdatedEventArgs.cs
- DebugView.cs
- DataServiceKeyAttribute.cs
- XmlCharacterData.cs
- DefaultParameterValueAttribute.cs
- XmlArrayAttribute.cs
- DecoderNLS.cs
- HttpResponse.cs
- NamespaceCollection.cs