Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / KeyEvent.cs / 1305376 / KeyEvent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Diagnostics.CodeAnalysis; ////// /// [System.Runtime.InteropServices.ComVisible(true)] public class KeyEventArgs : EventArgs { ////// Provides data for the ///or /// event. /// /// /// Contains key data for KeyDown and KeyUp events. This is a combination /// of keycode and modifer flags. /// private readonly Keys keyData; ////// /// Determines if this event has been handled by a handler. If handled, the /// key event will not be sent along to Windows. If not handled, the event /// will be sent to Windows for default processing. /// private bool handled = false; ////// /// private bool suppressKeyPress = false; ////// /// public KeyEventArgs(Keys keyData) { this.keyData = keyData; } ////// Initializes a new /// instance of the ///class. /// /// /// public virtual bool Alt { get { return (keyData & Keys.Alt) == Keys.Alt; } } ////// Gets a value indicating whether the ALT key was pressed. /// ////// /// public bool Control { get { return (keyData & Keys.Control) == Keys.Control; } } ////// Gets a value indicating whether the CTRL key was pressed. /// ////// /// // public bool Handled { get { return handled; } set { handled = value; } } ////// Gets or sets a value /// indicating whether the event was handled. /// ////// /// //subhag : changed the behaviour of the KeyCode as per the new requirements. public Keys KeyCode { [ // Keys is discontiguous so we have to use Enum.IsDefined. SuppressMessage("Microsoft.Performance", "CA1803:AvoidCostlyCallsWherePossible") ] get { Keys keyGenerated = keyData & Keys.KeyCode; // since Keys can be discontiguous, keeping Enum.IsDefined. if (!Enum.IsDefined(typeof(Keys),(int)keyGenerated)) return Keys.None; else return keyGenerated; } } ////// Gets the keyboard code for a ///or /// event. /// /// /// //subhag : added the KeyValue as per the new requirements. public int KeyValue { get { return (int)(keyData & Keys.KeyCode); } } ////// Gets the keyboard value for a ///or /// event. /// /// /// public Keys KeyData { get { return keyData; } } ////// Gets the key data for a ///or /// event. /// /// /// public Keys Modifiers { get { return keyData & Keys.Modifiers; } } ////// Gets the modifier flags for a ///or event. /// This indicates which modifier keys (CTRL, SHIFT, and/or ALT) were pressed. /// /// /// public virtual bool Shift { get { return (keyData & Keys.Shift) == Keys.Shift; } } ////// Gets /// a value indicating whether the SHIFT key was pressed. /// ////// /// // public bool SuppressKeyPress { get { return suppressKeyPress; } set { suppressKeyPress = value; handled = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Diagnostics.CodeAnalysis; ////// /// [System.Runtime.InteropServices.ComVisible(true)] public class KeyEventArgs : EventArgs { ////// Provides data for the ///or /// event. /// /// /// Contains key data for KeyDown and KeyUp events. This is a combination /// of keycode and modifer flags. /// private readonly Keys keyData; ////// /// Determines if this event has been handled by a handler. If handled, the /// key event will not be sent along to Windows. If not handled, the event /// will be sent to Windows for default processing. /// private bool handled = false; ////// /// private bool suppressKeyPress = false; ////// /// public KeyEventArgs(Keys keyData) { this.keyData = keyData; } ////// Initializes a new /// instance of the ///class. /// /// /// public virtual bool Alt { get { return (keyData & Keys.Alt) == Keys.Alt; } } ////// Gets a value indicating whether the ALT key was pressed. /// ////// /// public bool Control { get { return (keyData & Keys.Control) == Keys.Control; } } ////// Gets a value indicating whether the CTRL key was pressed. /// ////// /// // public bool Handled { get { return handled; } set { handled = value; } } ////// Gets or sets a value /// indicating whether the event was handled. /// ////// /// //subhag : changed the behaviour of the KeyCode as per the new requirements. public Keys KeyCode { [ // Keys is discontiguous so we have to use Enum.IsDefined. SuppressMessage("Microsoft.Performance", "CA1803:AvoidCostlyCallsWherePossible") ] get { Keys keyGenerated = keyData & Keys.KeyCode; // since Keys can be discontiguous, keeping Enum.IsDefined. if (!Enum.IsDefined(typeof(Keys),(int)keyGenerated)) return Keys.None; else return keyGenerated; } } ////// Gets the keyboard code for a ///or /// event. /// /// /// //subhag : added the KeyValue as per the new requirements. public int KeyValue { get { return (int)(keyData & Keys.KeyCode); } } ////// Gets the keyboard value for a ///or /// event. /// /// /// public Keys KeyData { get { return keyData; } } ////// Gets the key data for a ///or /// event. /// /// /// public Keys Modifiers { get { return keyData & Keys.Modifiers; } } ////// Gets the modifier flags for a ///or event. /// This indicates which modifier keys (CTRL, SHIFT, and/or ALT) were pressed. /// /// /// public virtual bool Shift { get { return (keyData & Keys.Shift) == Keys.Shift; } } ////// Gets /// a value indicating whether the SHIFT key was pressed. /// ////// /// // public bool SuppressKeyPress { get { return suppressKeyPress; } set { suppressKeyPress = value; handled = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
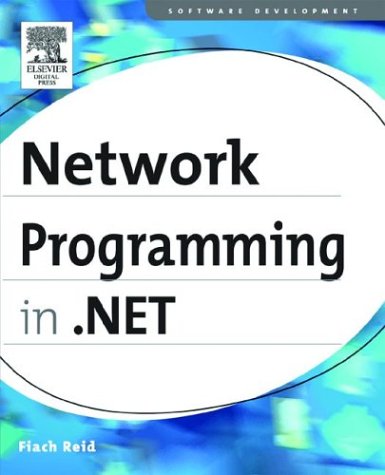
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlAttributeOverrides.cs
- Timeline.cs
- EventsTab.cs
- CodeRemoveEventStatement.cs
- WorkflowCompensationBehavior.cs
- HatchBrush.cs
- Viewport3DAutomationPeer.cs
- BitmapScalingModeValidation.cs
- SqlClientMetaDataCollectionNames.cs
- CommandDevice.cs
- WebPartManager.cs
- FixedSOMLineCollection.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- UnmanagedMemoryStream.cs
- _CommandStream.cs
- Wizard.cs
- FormViewDeletedEventArgs.cs
- ParserStreamGeometryContext.cs
- LayoutEngine.cs
- EnvironmentPermission.cs
- ReliabilityContractAttribute.cs
- CollectionBase.cs
- LogWriteRestartAreaAsyncResult.cs
- DefaultPropertyAttribute.cs
- ToolStripStatusLabel.cs
- WebServiceMethodData.cs
- SimpleExpression.cs
- TextServicesCompartmentContext.cs
- AssemblyAttributes.cs
- METAHEADER.cs
- IconBitmapDecoder.cs
- SignedPkcs7.cs
- HelpInfo.cs
- _ConnectionGroup.cs
- WindowsStatusBar.cs
- ProxyGenerationError.cs
- ContextStack.cs
- fixedPageContentExtractor.cs
- AlgoModule.cs
- SourceFilter.cs
- MarshalByValueComponent.cs
- _AuthenticationState.cs
- PaginationProgressEventArgs.cs
- control.ime.cs
- COSERVERINFO.cs
- _AutoWebProxyScriptWrapper.cs
- MenuItemCollection.cs
- ImpersonateTokenRef.cs
- DataGridViewUtilities.cs
- ValidatedControlConverter.cs
- RowsCopiedEventArgs.cs
- Nullable.cs
- CalendarDateChangedEventArgs.cs
- DependencyObjectProvider.cs
- OperatingSystem.cs
- UserPreferenceChangingEventArgs.cs
- XmlWrappingReader.cs
- ColorConvertedBitmapExtension.cs
- WindowsPrincipal.cs
- MiniParameterInfo.cs
- PlatformNotSupportedException.cs
- DeobfuscatingStream.cs
- InheritedPropertyChangedEventArgs.cs
- EntityDataSourceMemberPath.cs
- FontDialog.cs
- Assert.cs
- RC2CryptoServiceProvider.cs
- WebRequestModuleElement.cs
- ToolboxItemWrapper.cs
- BrowserTree.cs
- Stroke2.cs
- SqlVersion.cs
- ExpressionVisitor.cs
- XmlTextWriter.cs
- FileLevelControlBuilderAttribute.cs
- SplitContainer.cs
- PolyBezierSegmentFigureLogic.cs
- ItemsPresenter.cs
- RegexCaptureCollection.cs
- TerminateSequenceResponse.cs
- COSERVERINFO.cs
- EntityViewGenerationAttribute.cs
- DigestTraceRecordHelper.cs
- BinaryParser.cs
- GroupQuery.cs
- ChangeToolStripParentVerb.cs
- DivideByZeroException.cs
- Viewport3DVisual.cs
- SessionSymmetricTransportSecurityProtocolFactory.cs
- VoiceInfo.cs
- Random.cs
- XmlNamespaceDeclarationsAttribute.cs
- ObjectCloneHelper.cs
- WebConfigurationHostFileChange.cs
- ZipIOExtraFieldElement.cs
- InteropTrackingRecord.cs
- ReadOnlyHierarchicalDataSource.cs
- LocalClientSecuritySettings.cs
- StringValidator.cs
- SmiConnection.cs