Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SrgsCompiler / SrgsElementFactoryCompiler.cs / 1 / SrgsElementFactoryCompiler.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 [....] Created from the Kurosawa Code //--------------------------------------------------------------------------- #region Using directives using System; using System.Collections.Generic; using System.Globalization; using System.Text; using System.Speech.Internal.SrgsParser; #endregion #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Internal.SrgsCompiler { internal class SrgsElementCompilerFactory : IElementFactory { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors #if !NO_STG internal SrgsElementCompilerFactory (Backend backend, CustomGrammar cg) { _backend = backend; _cg = cg; _grammar = new GrammarElement (backend, cg); } #else internal SrgsElementCompilerFactory (Backend backend) { _backend = backend; _grammar = new GrammarElement (backend); } #endif #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods ////// Clear all the rules /// void IElementFactory.RemoveAllRules () { } IPropertyTag IElementFactory.CreatePropertyTag (IElement parent) { return new PropertyTag ((ParseElementCollection) parent, _backend); } ISemanticTag IElementFactory.CreateSemanticTag (IElement parent) { return new SemanticTag ((ParseElementCollection) parent, _backend); } IElementText IElementFactory.CreateText (IElement parent, string value) { return null; } IToken IElementFactory.CreateToken (IElement parent, string content, string pronunciation, string display, float reqConfidence) { ParseToken ((ParseElementCollection) parent, content, pronunciation, display, reqConfidence); return null; } IItem IElementFactory.CreateItem (IElement parent, IRule rule, int minRepeat, int maxRepeat, float repeatProbability, float weight) { return (IItem) new Item (_backend, (Rule) rule, minRepeat, maxRepeat, repeatProbability, weight); } IRuleRef IElementFactory.CreateRuleRef (IElement parent, Uri srgsUri) { throw new NotImplementedException (); } IRuleRef IElementFactory.CreateRuleRef (IElement parent, Uri srgsUri, string semanticKey, string parameters) { return new RuleRef ((ParseElementCollection) parent, _backend, srgsUri, _grammar.UndefRules, semanticKey, parameters); } void IElementFactory.InitSpecialRuleRef (IElement parent, IRuleRef specialRule) { ((RuleRef) specialRule).InitSpecialRuleRef (_backend, (ParseElementCollection) parent); } IOneOf IElementFactory.CreateOneOf (IElement parent, IRule rule) { return (IOneOf) new OneOf ((Rule) rule, _backend); } ISubset IElementFactory.CreateSubset (IElement parent, string text, MatchMode mode) { return (ISubset) new Subset ((ParseElementCollection) parent, _backend, text, mode); } #if !NO_STG void IElementFactory.AddScript (IGrammar grammar, string rule, string code) { ((GrammarElement) grammar).AddScript (rule, code); } string IElementFactory.AddScript (IGrammar grammar, string rule, string code, string filename, int line) { // add the #line information if (line >= 0) { if (_cg._language == "C#") { // C# return String.Format (CultureInfo.InvariantCulture, "#line {0} \"{1}\"\n{2}", line.ToString (CultureInfo.InvariantCulture), filename, code); } else { // VB.Net return String.Format (CultureInfo.InvariantCulture, "#ExternalSource (\"{1}\",{0}) \n{2}\n#End ExternalSource\n", line.ToString (CultureInfo.InvariantCulture), filename, code); } } return code; } void IElementFactory.AddScript (IGrammar grammar, string script, string filename, int line) { // add the #line information if (line >= 0) { if (_cg._language == "C#") { // C# _cg._script.Append ("#line "); _cg._script.Append (line.ToString (CultureInfo.InvariantCulture)); _cg._script.Append (" \""); _cg._script.Append (filename); _cg._script.Append ("\"\n"); _cg._script.Append (script); } else { // VB.Net _cg._script.Append ("#ExternalSource ("); _cg._script.Append (" \""); _cg._script.Append (filename); _cg._script.Append ("\","); _cg._script.Append (line.ToString (CultureInfo.InvariantCulture)); _cg._script.Append (")\n"); _cg._script.Append (script); _cg._script.Append ("#End #ExternalSource\n"); } } else { _cg._script.Append (script); } } #endif void IElementFactory.AddItem (IOneOf oneOf, IItem item) { } void IElementFactory.AddElement (IRule rule, IElement value) { } void IElementFactory.AddElement (IItem item, IElement value) { } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties IGrammar IElementFactory.Grammar { get { return _grammar; } } IRuleRef IElementFactory.Null { get { return RuleRef.Null; } } IRuleRef IElementFactory.Void { get { return RuleRef.Void; } } IRuleRef IElementFactory.Garbage { get { return RuleRef.Garbage; } } #endregion //******************************************************************* // // Private Methods // //******************************************************************** #region Private Methods // Disable parameter validation check #pragma warning disable 56507 ////// Add transition representing the normalized token. /// /// White Space Normalization - Trim leading/trailing white spaces. /// Collapse white space sequences to a single ' '. /// Restrictions - Normalized token cannot be empty. /// Normalized token cannot contain double-quote. /// /// If (Parent == Token) And (Parent.SAPIPron.Length > 0) Then /// Escape normalized token. "/" -> "\/", "\" -> "\\" /// Build /D/L/P; form from the escaped token and SAPIPron. /// /// SAPIPron may be a semi-colon delimited list of pronunciations. /// In this case, a transition for each of the pronuncitions will be added. /// /// AddTransition(NormalizedToken, Parent.EndState, NewState) /// Parent.EndState = NewState /// /// Parent element /// Token string /// /// /// private void ParseToken (ParseElementCollection parent, string sToken, string pronunciation, string display, float reqConfidence) { int requiredConfidence = (parent != null) ? parent._confidence : CfgGrammar.SP_NORMAL_CONFIDENCE; // Performs white space normalization in place sToken = Backend.NormalizeTokenWhiteSpace (sToken); if (string.IsNullOrEmpty (sToken)) { return; } // "sapi:reqconf" Attribute parent._confidence = CfgGrammar.SP_NORMAL_CONFIDENCE; // Default to normal if (reqConfidence < 0 || reqConfidence.Equals (0.5f)) { parent._confidence = CfgGrammar.SP_NORMAL_CONFIDENCE; // Default to normal } else if (reqConfidence < 0.5) { parent._confidence = CfgGrammar.SP_LOW_CONFIDENCE; } else { parent._confidence = CfgGrammar.SP_HIGH_CONFIDENCE; } // If SAPIPron is specified, use /D/L/P; as the transition text, for each of the pronunciations. if (pronunciation != null || display != null) { // Escape normalized token. "/" -> "\/", "\" -> "\\" string sEscapedToken = EscapeToken (sToken); string sDisplayToken = display == null ? sEscapedToken : EscapeToken (display); if (pronunciation != null) { // Garbage transition is optional whereas Wildcard is not. So we need additional epsilon transition. OneOf oneOf = pronunciation.IndexOf (';') >= 0 ? new OneOf (parent._rule, _backend) : null; for (int iCurPron = 0, iDeliminator = 0; iCurPron < pronunciation.Length; iCurPron = iDeliminator + 1) { // Find semi-colon deliminator and replace with null iDeliminator = pronunciation.IndexOf (';', iCurPron); if (iDeliminator == -1) { iDeliminator = pronunciation.Length; } string pron = pronunciation.Substring (iCurPron, iDeliminator - iCurPron); string sSubPron = null; switch (_backend.Alphabet) { case AlphabetType.Sapi: sSubPron = PhonemeConverter.ConvertPronToId (pron, _grammar.Backend.LangId); break; case AlphabetType.Ipa: sSubPron = pron; PhonemeConverter.ValidateUpsIds (sSubPron); break; case AlphabetType.Ups: sSubPron = PhonemeConverter.UpsConverter.ConvertPronToId (pron); break; } // Build /D/L/P; form for this pronunciation. string sDLP = string.Format (CultureInfo.InvariantCulture, "/{0}/{1}/{2};", sDisplayToken, sEscapedToken, sSubPron); // Add /D/L/P; transition to the new state. if (oneOf != null) { oneOf.AddArc (_backend.WordTransition (sDLP, 1.0f, requiredConfidence)); } else { parent.AddArc (_backend.WordTransition (sDLP, 1.0f, requiredConfidence)); } } if (oneOf != null) { ((IOneOf) oneOf).PostParse (parent); } } else { // Build /D/L; form for this pronunciation. string sDLP = string.Format (CultureInfo.InvariantCulture, "/{0}/{1};", sDisplayToken, sEscapedToken); // Add /D/L; transition to the new state. parent.AddArc (_backend.WordTransition (sDLP, 1.0f, requiredConfidence)); } } else { // Add transition to the new state with normalized token. // parent.AddArc (_backend.WordTransition (sToken, 1.0f, requiredConfidence)); } } #pragma warning restore 56507 ////// Escape token. "/" -> "\/", "\" -> "\\" /// /// ///private static string EscapeToken (string sToken) // String to escape { System.Diagnostics.Debug.Assert (!string.IsNullOrEmpty (sToken)); // Easy out if no escape characters if (sToken.IndexOf ("\\/", StringComparison.Ordinal) == -1) { return sToken; } char [] achSrc = sToken.ToCharArray (); char [] achDest = new char [achSrc.Length * 2]; int iDest = 0; // Escape slashes and backslashes. for (int i = 0; i < achSrc.Length; ) { if ((achSrc [i] == '\\') || (achSrc [i] == '/')) { achDest [iDest++] = '\\'; // Escape special character } achDest [iDest++] = achSrc [i++]; } // null terminate and update string length return new string (achDest, 0, iDest); } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields // Callers param private Backend _backend; // Grammar private GrammarElement _grammar; #if !NO_STG // Callers param private CustomGrammar _cg; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
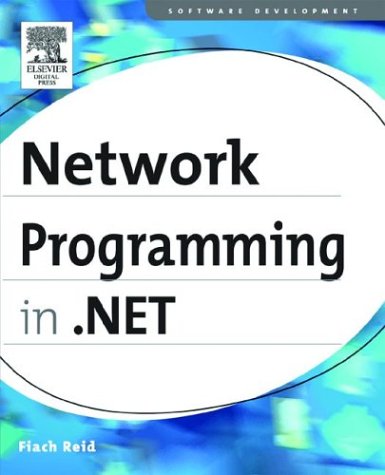
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntitySetDataBindingList.cs
- SharedStatics.cs
- WorkflowRuntime.cs
- DnsPermission.cs
- SafeCryptHandles.cs
- StorageScalarPropertyMapping.cs
- SafeReadContext.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- StyleXamlParser.cs
- sqlpipe.cs
- ReadContentAsBinaryHelper.cs
- ObjectSecurityT.cs
- SiteMapNodeItemEventArgs.cs
- ObjectDataSourceMethodEventArgs.cs
- CustomError.cs
- DeviceFilterDictionary.cs
- InfoCardBaseException.cs
- AuthenticationService.cs
- XmlSchemaAll.cs
- documentsequencetextcontainer.cs
- DateTimeConverter.cs
- DataGridViewColumn.cs
- SymDocumentType.cs
- GeneralTransform3DGroup.cs
- IMembershipProvider.cs
- UIElement.cs
- HwndHost.cs
- XXXInfos.cs
- CategoryNameCollection.cs
- XamlValidatingReader.cs
- securestring.cs
- TimeSpanMinutesConverter.cs
- BamlResourceContent.cs
- DictionaryBase.cs
- WebPartChrome.cs
- StickyNoteContentControl.cs
- PersonalizableAttribute.cs
- TaskForm.cs
- IFormattable.cs
- FileDialogCustomPlace.cs
- RawStylusSystemGestureInputReport.cs
- AttributeUsageAttribute.cs
- LinkedList.cs
- Lasso.cs
- ListViewInsertionMark.cs
- GenericTypeParameterConverter.cs
- ListQueryResults.cs
- SQLDecimalStorage.cs
- ApplicationServicesHostFactory.cs
- FacetChecker.cs
- PngBitmapDecoder.cs
- Semaphore.cs
- ComponentEditorPage.cs
- MessageSecurityOverHttp.cs
- LayoutEditorPart.cs
- SqlTypesSchemaImporter.cs
- RetrieveVirtualItemEventArgs.cs
- DataBindingList.cs
- AutomationPropertyInfo.cs
- HMACSHA1.cs
- XmlComment.cs
- AddInPipelineAttributes.cs
- ConvertersCollection.cs
- SqlLiftWhereClauses.cs
- SecureUICommand.cs
- ProtocolViolationException.cs
- CellCreator.cs
- ListControlActionList.cs
- SQLRoleProvider.cs
- ManagementNamedValueCollection.cs
- HttpProfileBase.cs
- LongValidator.cs
- SqlUDTStorage.cs
- RoamingStoreFile.cs
- TextMessageEncodingElement.cs
- StoreUtilities.cs
- ToolStripItemTextRenderEventArgs.cs
- FixedSOMPageConstructor.cs
- VariableAction.cs
- TimeSpanSecondsConverter.cs
- ObjectStorage.cs
- DataGridViewElement.cs
- AsymmetricKeyExchangeFormatter.cs
- InvalidFilterCriteriaException.cs
- FrameworkTemplate.cs
- UIntPtr.cs
- StyleSheet.cs
- UserPersonalizationStateInfo.cs
- ListViewInsertionMark.cs
- InputScope.cs
- Currency.cs
- OrderedDictionary.cs
- XmlCharCheckingWriter.cs
- Line.cs
- unsafeIndexingFilterStream.cs
- KerberosSecurityTokenAuthenticator.cs
- Line.cs
- ClipboardData.cs
- ConvertEvent.cs
- BamlLocalizerErrorNotifyEventArgs.cs