Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Globalization / BamlResourceContent.cs / 1305600 / BamlResourceContent.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Utility that handles parsing Baml Resource Content // // History: 11/29/2003 garyyang Rewrote // //--------------------------------------------------------------------------- using System; using System.Text; using System.Text.RegularExpressions; using System.Collections; using System.Diagnostics; using System.Collections.Generic; using System.Windows; namespace MS.Internal.Globalization { internal static class BamlResourceContentUtil { //------------------------------------- // Internal methods //------------------------------------- ////// Escape a string /// internal static string EscapeString(string content) { if (content == null) return null; StringBuilder builder = new StringBuilder(); for (int i = 0; i < content.Length; i++) { switch (content[i]) { case BamlConst.ChildStart : case BamlConst.ChildEnd : case BamlConst.EscapeChar : { builder.Append(BamlConst.EscapeChar); builder.Append(content[i]); break; } case '&' : { builder.Append("&"); break; } case '<' : { builder.Append("<"); break; } case '>' : { builder.Append(">"); break; } case '\'': { builder.Append("'"); break; } case '\"': { builder.Append("""); break; } default : { builder.Append(content[i]); break; } } } return builder.ToString(); } ////// Unescape a string. Note: /// Backslash following any character will become that character. /// Backslash by itself will be skipped. /// internal static string UnescapeString(string content) { return UnescapePattern.Replace( content, UnescapeMatchEvaluator ); } // Regular expression // need to use 4 backslash here because it is escaped by compiler and regular expressions private static Regex UnescapePattern = new Regex("(\\\\.?|<|>|"|'|&)", RegexOptions.CultureInvariant | RegexOptions.Compiled); // delegates to escape and unesacpe a matched pattern private static MatchEvaluator UnescapeMatchEvaluator = new MatchEvaluator(UnescapeMatch); ////// the delegate to Unescape the matched pattern /// private static string UnescapeMatch(Match match) { switch (match.Value) { case "<" : return "<"; case ">" : return ">"; case "&" : return "&"; case "'": return "'"; case """: return "\""; default: { // this is a '\' followed by 0 or 1 character Debug.Assert(match.Value.Length > 0 && match.Value[0] == BamlConst.EscapeChar); if (match.Value.Length == 2) { return match.Value[1].ToString(); } else { return string.Empty; } } } } ////// Parse the input string into an array of text/child-placeholder tokens. /// Element placeholders start with '#' and end with ';'. /// In case of error, a null array is returned. /// internal static BamlStringToken[] ParseChildPlaceholder(string input) { if (input == null) return null; Listtokens = new List (8); int tokenStart = 0; bool inPlaceHolder = false; for (int i = 0; i < input.Length; i++) { if (input[i] == BamlConst.ChildStart) { if (i == 0 || input[i - 1]!= BamlConst.EscapeChar) { if (inPlaceHolder) { // All # needs to be escaped in a child place holder return null; // error } inPlaceHolder = true; if (tokenStart < i) { tokens.Add( new BamlStringToken( BamlStringToken.TokenType.Text, UnescapeString(input.Substring(tokenStart, i - tokenStart)) ) ); tokenStart = i; } } } else if (input[i] == BamlConst.ChildEnd) { if ( i > 0 && input[i - 1] != BamlConst.EscapeChar && inPlaceHolder) { // It is a valid child placeholder end tokens.Add( new BamlStringToken( BamlStringToken.TokenType.ChildPlaceHolder, UnescapeString(input.Substring(tokenStart + 1, i - tokenStart - 1)) ) ); // Advance the token index tokenStart = i + 1; inPlaceHolder = false; } } } if (inPlaceHolder) { // at the end of the string, all child placeholder must be closed return null; // error } if (tokenStart < input.Length) { tokens.Add( new BamlStringToken( BamlStringToken.TokenType.Text, UnescapeString(input.Substring(tokenStart)) ) ); } return tokens.ToArray(); } } internal struct BamlStringToken { internal readonly TokenType Type; internal readonly string Value; internal BamlStringToken(TokenType type, string value) { Type = type; Value = value; } internal enum TokenType { Text, ChildPlaceHolder, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
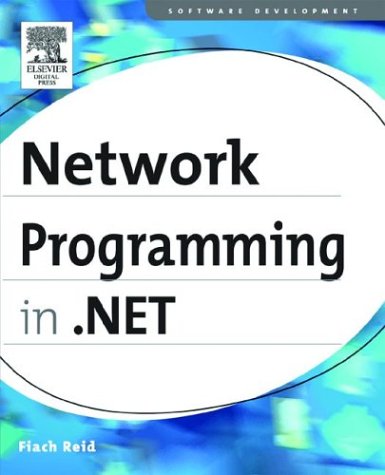
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSource.cs
- SapiRecoContext.cs
- KeysConverter.cs
- ManagedWndProcTracker.cs
- ClaimSet.cs
- OdbcErrorCollection.cs
- EventLogInformation.cs
- ActivityValidationServices.cs
- FormatterServices.cs
- ImmutableObjectAttribute.cs
- EffectiveValueEntry.cs
- ObjectSpanRewriter.cs
- Guid.cs
- MultipleViewProviderWrapper.cs
- InternalDispatchObject.cs
- PersistenceTypeAttribute.cs
- path.cs
- BinaryNode.cs
- PrintingPermission.cs
- RelativeSource.cs
- exports.cs
- Matrix3DConverter.cs
- JsonXmlDataContract.cs
- OperationAbortedException.cs
- WebRequest.cs
- NamespaceCollection.cs
- documentation.cs
- TraceSection.cs
- StorageScalarPropertyMapping.cs
- AutomationPatternInfo.cs
- altserialization.cs
- XamlStackWriter.cs
- ComplusTypeValidator.cs
- TextTreeExtractElementUndoUnit.cs
- ConnectionOrientedTransportElement.cs
- DynamicResourceExtensionConverter.cs
- _MultipleConnectAsync.cs
- XmlCustomFormatter.cs
- RemotingConfigParser.cs
- NameValueConfigurationElement.cs
- MSG.cs
- DataGridViewDataErrorEventArgs.cs
- ObjectParameter.cs
- NamedPipeTransportManager.cs
- SystemUnicastIPAddressInformation.cs
- DataServices.cs
- DynamicDocumentPaginator.cs
- JsonWriter.cs
- Pens.cs
- FilterRepeater.cs
- WpfKnownTypeInvoker.cs
- OrderPreservingMergeHelper.cs
- _AcceptOverlappedAsyncResult.cs
- SessionStateItemCollection.cs
- SqlBulkCopyColumnMapping.cs
- ButtonFieldBase.cs
- OdbcTransaction.cs
- CalendarDesigner.cs
- PageThemeCodeDomTreeGenerator.cs
- SimpleTypeResolver.cs
- RegexStringValidator.cs
- CodeExporter.cs
- DesignerVerbCollection.cs
- shaperfactory.cs
- WorkflowMarkupSerializationManager.cs
- COM2EnumConverter.cs
- Thread.cs
- TdsParserHelperClasses.cs
- CellQuery.cs
- ChannelServices.cs
- CaseCqlBlock.cs
- EraserBehavior.cs
- BasicHttpSecurityElement.cs
- BindingCollection.cs
- HtmlControlPersistable.cs
- GeometryHitTestResult.cs
- ECDsaCng.cs
- WSTrustFeb2005.cs
- FontInfo.cs
- NetDataContractSerializer.cs
- PersistenceTypeAttribute.cs
- CookieParameter.cs
- AutomationElementIdentifiers.cs
- XamlDesignerSerializationManager.cs
- Vector3DConverter.cs
- ExceptionUtil.cs
- LookupBindingPropertiesAttribute.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- MonitoringDescriptionAttribute.cs
- FileAuthorizationModule.cs
- TypeEnumerableViewSchema.cs
- GPPOINTF.cs
- PersonalizationStateQuery.cs
- COM2IDispatchConverter.cs
- CqlParser.cs
- InkCanvasFeedbackAdorner.cs
- HostingPreferredMapPath.cs
- Int16Storage.cs
- NameHandler.cs
- GradientBrush.cs