Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / Merging / OrderPreservingMergeHelper.cs / 1305376 / OrderPreservingMergeHelper.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // OrderPreservingMergeHelper.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Threading.Tasks; using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// The order preserving merge helper guarantees the output stream is in a specific order. This is done /// by comparing keys from a set of already-sorted input partitions, and coalescing output data using /// incremental key comparisons. /// ////// internal class OrderPreservingMergeHelper : IMergeHelper { private QueryTaskGroupState m_taskGroupState; // State shared among tasks. private PartitionedStream m_partitions; // Source partitions. private Shared m_results; // The array where results are stored. private TaskScheduler m_taskScheduler; // The task manager to execute the query. //------------------------------------------------------------------------------------ // Instantiates a new merge helper. // // Arguments: // partitions - the source partitions from which to consume data. // ignoreOutput - whether we're enumerating "for effect" or for output. // internal OrderPreservingMergeHelper(PartitionedStream partitions, TaskScheduler taskScheduler, CancellationState cancellationState, int queryId) { Contract.Assert(partitions != null); TraceHelpers.TraceInfo("KeyOrderPreservingMergeHelper::.ctor(..): creating an order preserving merge helper"); m_taskGroupState = new QueryTaskGroupState(cancellationState, queryId); m_partitions = partitions; m_results = new Shared (null); m_taskScheduler = taskScheduler; } //----------------------------------------------------------------------------------- // Schedules execution of the merge itself. // // Arguments: // ordinalIndexState - the state of the ordinal index of the merged partitions // void IMergeHelper .Execute() { OrderPreservingSpoolingTask .Spool(m_taskGroupState, m_partitions, m_results, m_taskScheduler); } //----------------------------------------------------------------------------------- // Gets the enumerator from which to enumerate output results. // IEnumerator IMergeHelper .GetEnumerator() { Contract.Assert(m_results.Value != null); return ((IEnumerable )m_results.Value).GetEnumerator(); } //----------------------------------------------------------------------------------- // Returns the results as an array. // public TInputOutput[] GetResultsAsArray() { return m_results.Value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
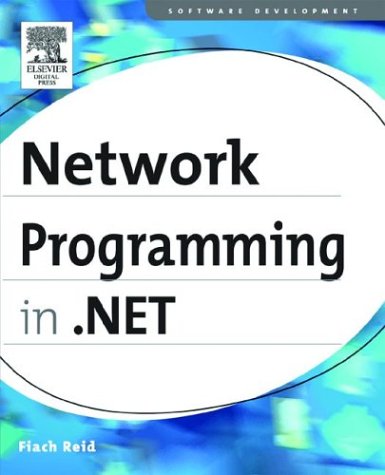
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttachedPropertyBrowsableForTypeAttribute.cs
- EpmContentDeSerializer.cs
- AuthenticationServiceManager.cs
- RawKeyboardInputReport.cs
- ToolStripOverflow.cs
- EntityCommand.cs
- DataGridPagerStyle.cs
- ImmutableObjectAttribute.cs
- DataKey.cs
- XmlSchemaAll.cs
- BitmapDecoder.cs
- connectionpool.cs
- SrgsRulesCollection.cs
- SqlMethods.cs
- MDIWindowDialog.cs
- SequenceDesigner.xaml.cs
- WindowsFormsSynchronizationContext.cs
- String.cs
- InputLangChangeEvent.cs
- ProviderSettings.cs
- XmlElementList.cs
- LocalBuilder.cs
- GcHandle.cs
- SmtpFailedRecipientsException.cs
- HtmlTableRowCollection.cs
- XMLUtil.cs
- StackSpiller.Temps.cs
- PropertyBuilder.cs
- CombinedTcpChannel.cs
- ProgressChangedEventArgs.cs
- IncrementalReadDecoders.cs
- DummyDataSource.cs
- CancellationHandlerDesigner.cs
- FilterUserControlBase.cs
- AuthenticationModuleElement.cs
- NamedPipeWorkerProcess.cs
- Crc32Helper.cs
- SQLStringStorage.cs
- _LazyAsyncResult.cs
- TraceSwitch.cs
- OrderToken.cs
- LocationSectionRecord.cs
- MemberPathMap.cs
- RowCache.cs
- XamlDesignerSerializationManager.cs
- Pkcs7Recipient.cs
- DataServiceRequestException.cs
- SerialPort.cs
- LinkDescriptor.cs
- FixedSOMPageElement.cs
- DesignerForm.cs
- XmlReflectionMember.cs
- sqlnorm.cs
- EntityType.cs
- RegexStringValidator.cs
- SqlDependencyListener.cs
- Rect.cs
- SQLResource.cs
- _SafeNetHandles.cs
- DeviceSpecific.cs
- CheckBoxRenderer.cs
- ParserExtension.cs
- LayoutTable.cs
- ExpandSegment.cs
- BoundConstants.cs
- tooltip.cs
- Paragraph.cs
- ScrollViewer.cs
- StorageComplexPropertyMapping.cs
- SHA384.cs
- CacheRequest.cs
- CommonXSendMessage.cs
- MergablePropertyAttribute.cs
- SecurityStateEncoder.cs
- DocumentReference.cs
- TextParaLineResult.cs
- DataGridColumnCollection.cs
- TypePresenter.xaml.cs
- LayoutEvent.cs
- Subtree.cs
- IndexedEnumerable.cs
- TdsParameterSetter.cs
- odbcmetadatacolumnnames.cs
- Transactions.cs
- Dictionary.cs
- XhtmlBasicTextBoxAdapter.cs
- SegmentInfo.cs
- MarkedHighlightComponent.cs
- safex509handles.cs
- Select.cs
- safelinkcollection.cs
- AssociationEndMember.cs
- FocusWithinProperty.cs
- Canonicalizers.cs
- XslTransform.cs
- WebResourceAttribute.cs
- ButtonRenderer.cs
- FontNamesConverter.cs
- ComponentDispatcher.cs
- ProgressBar.cs