Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Documents / DynamicDocumentPaginator.cs / 1 / DynamicDocumentPaginator.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DynamicDocumentPaginator.cs // // Description: Defines advanced methods and properties for paginating layouts, // such as background pagination and methods for tracking content // positions across repaginations. // // History: // 08/29/2005 : grzegorz - created. // //--------------------------------------------------------------------------- using System.ComponentModel; // AsyncCompletedEventArgs using MS.Internal.PresentationCore; // SR, SRID namespace System.Windows.Documents { ////// Defines advanced methods and properties for paginating layouts, such /// as background pagination and methods for tracking content positions /// across repaginations. /// public abstract class DynamicDocumentPaginator : DocumentPaginator { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Returns the page number on which the ContentPosition appears. /// /// Content position. ////// Returns the page number on which the ContentPosition appears. /// ////// Throws ArgumentException if the ContentPosition does not exist within /// this element's tree. /// public abstract int GetPageNumber(ContentPosition contentPosition); ////// Async version of /// Content position. ////// /// Throws ArgumentException if the ContentPosition does not exist within /// this element's tree. /// public virtual void GetPageNumberAsync(ContentPosition contentPosition) { GetPageNumberAsync(contentPosition, null); } ////// Async version of /// Content position. /// Unique identifier for the asynchronous task. ////// /// Throws ArgumentException if the ContentPosition does not exist within /// this element’s tree. /// public virtual void GetPageNumberAsync(ContentPosition contentPosition, object userState) { int pageNumber; // Content position cannot be null. if (contentPosition == null) { throw new ArgumentNullException("contentPosition"); } // Content position cannot be Missing. if (contentPosition == ContentPosition.Missing) { throw new ArgumentException(SR.Get(SRID.PaginatorMissingContentPosition), "contentPosition"); } pageNumber = GetPageNumber(contentPosition); OnGetPageNumberCompleted(new GetPageNumberCompletedEventArgs(contentPosition, pageNumber, null, false, userState)); } ////// Returns the ContentPosition for the given page. /// /// Document page. ///Returns the ContentPosition for the given page. ////// Throws ArgumentException if the page is not valid. /// public abstract ContentPosition GetPagePosition(DocumentPage page); ////// Returns the ContentPosition for an object within the content. /// /// Object within this element's tree. ///Returns the ContentPosition for an object within the content. ////// Throws ArgumentException if the object does not exist within this element's tree. /// public abstract ContentPosition GetObjectPosition(Object value); #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Whether content is paginated in the background. /// When True, the Paginator will paginate its content in the background, /// firing the PaginationCompleted and PaginationProgress events as appropriate. /// Background pagination begins immediately when set to True. If the /// PageSize is modified and this property is set to True, then all pages /// will be repaginated and existing pages may be destroyed. /// The default value is False. /// public virtual bool IsBackgroundPaginationEnabled { get { return false; } set { } } #endregion Public Properties //-------------------------------------------------------------------- // // Public Events // //-------------------------------------------------------------------- #region Public Events ////// Fired when a GetPageNumberAsync call has completed. /// public event GetPageNumberCompletedEventHandler GetPageNumberCompleted; ////// Fired when all document content has been paginated. After this event /// IsPageCountValid will be True. /// public event EventHandler PaginationCompleted; ////// Fired when background pagination is enabled, indicating which pages /// have been formatted and are available. /// public event PaginationProgressEventHandler PaginationProgress; #endregion Public Events //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the GetPageNumberCompleted event. protected virtual void OnGetPageNumberCompleted(GetPageNumberCompletedEventArgs e) { if (this.GetPageNumberCompleted != null) { this.GetPageNumberCompleted(this, e); } } ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the PaginationProgress event. protected virtual void OnPaginationProgress(PaginationProgressEventArgs e) { if (this.PaginationProgress != null) { this.PaginationProgress(this, e); } } ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the PaginationCompleted event. protected virtual void OnPaginationCompleted(EventArgs e) { if (this.PaginationCompleted != null) { this.PaginationCompleted(this, e); } } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DynamicDocumentPaginator.cs // // Description: Defines advanced methods and properties for paginating layouts, // such as background pagination and methods for tracking content // positions across repaginations. // // History: // 08/29/2005 : grzegorz - created. // //--------------------------------------------------------------------------- using System.ComponentModel; // AsyncCompletedEventArgs using MS.Internal.PresentationCore; // SR, SRID namespace System.Windows.Documents { ////// Defines advanced methods and properties for paginating layouts, such /// as background pagination and methods for tracking content positions /// across repaginations. /// public abstract class DynamicDocumentPaginator : DocumentPaginator { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Returns the page number on which the ContentPosition appears. /// /// Content position. ////// Returns the page number on which the ContentPosition appears. /// ////// Throws ArgumentException if the ContentPosition does not exist within /// this element's tree. /// public abstract int GetPageNumber(ContentPosition contentPosition); ////// Async version of /// Content position. ////// /// Throws ArgumentException if the ContentPosition does not exist within /// this element's tree. /// public virtual void GetPageNumberAsync(ContentPosition contentPosition) { GetPageNumberAsync(contentPosition, null); } ////// Async version of /// Content position. /// Unique identifier for the asynchronous task. ////// /// Throws ArgumentException if the ContentPosition does not exist within /// this element’s tree. /// public virtual void GetPageNumberAsync(ContentPosition contentPosition, object userState) { int pageNumber; // Content position cannot be null. if (contentPosition == null) { throw new ArgumentNullException("contentPosition"); } // Content position cannot be Missing. if (contentPosition == ContentPosition.Missing) { throw new ArgumentException(SR.Get(SRID.PaginatorMissingContentPosition), "contentPosition"); } pageNumber = GetPageNumber(contentPosition); OnGetPageNumberCompleted(new GetPageNumberCompletedEventArgs(contentPosition, pageNumber, null, false, userState)); } ////// Returns the ContentPosition for the given page. /// /// Document page. ///Returns the ContentPosition for the given page. ////// Throws ArgumentException if the page is not valid. /// public abstract ContentPosition GetPagePosition(DocumentPage page); ////// Returns the ContentPosition for an object within the content. /// /// Object within this element's tree. ///Returns the ContentPosition for an object within the content. ////// Throws ArgumentException if the object does not exist within this element's tree. /// public abstract ContentPosition GetObjectPosition(Object value); #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Whether content is paginated in the background. /// When True, the Paginator will paginate its content in the background, /// firing the PaginationCompleted and PaginationProgress events as appropriate. /// Background pagination begins immediately when set to True. If the /// PageSize is modified and this property is set to True, then all pages /// will be repaginated and existing pages may be destroyed. /// The default value is False. /// public virtual bool IsBackgroundPaginationEnabled { get { return false; } set { } } #endregion Public Properties //-------------------------------------------------------------------- // // Public Events // //-------------------------------------------------------------------- #region Public Events ////// Fired when a GetPageNumberAsync call has completed. /// public event GetPageNumberCompletedEventHandler GetPageNumberCompleted; ////// Fired when all document content has been paginated. After this event /// IsPageCountValid will be True. /// public event EventHandler PaginationCompleted; ////// Fired when background pagination is enabled, indicating which pages /// have been formatted and are available. /// public event PaginationProgressEventHandler PaginationProgress; #endregion Public Events //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the GetPageNumberCompleted event. protected virtual void OnGetPageNumberCompleted(GetPageNumberCompletedEventArgs e) { if (this.GetPageNumberCompleted != null) { this.GetPageNumberCompleted(this, e); } } ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the PaginationProgress event. protected virtual void OnPaginationProgress(PaginationProgressEventArgs e) { if (this.PaginationProgress != null) { this.PaginationProgress(this, e); } } ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the PaginationCompleted event. protected virtual void OnPaginationCompleted(EventArgs e) { if (this.PaginationCompleted != null) { this.PaginationCompleted(this, e); } } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
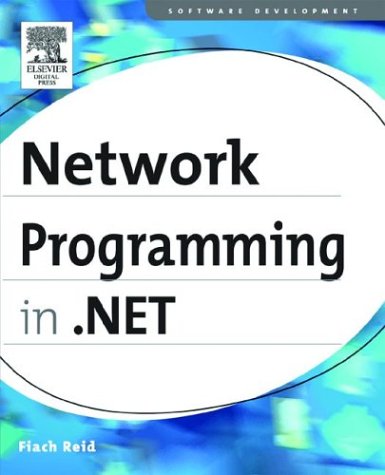
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentProperties.cs
- CodeDomLocalizationProvider.cs
- StubHelpers.cs
- Transform.cs
- NamespaceInfo.cs
- EntityCommandExecutionException.cs
- ExeContext.cs
- FixedNode.cs
- DataGridViewRowConverter.cs
- BeginEvent.cs
- ClickablePoint.cs
- XmlSchemaDocumentation.cs
- XmlChildEnumerator.cs
- XmlCountingReader.cs
- StorageComplexPropertyMapping.cs
- CharStorage.cs
- TabletDeviceInfo.cs
- LayoutDump.cs
- CompilationPass2Task.cs
- SecurityKeyUsage.cs
- _DynamicWinsockMethods.cs
- Currency.cs
- ResolvedKeyFrameEntry.cs
- StoreContentChangedEventArgs.cs
- SolidBrush.cs
- FlowDocumentScrollViewer.cs
- SqlTypesSchemaImporter.cs
- ForEach.cs
- DirectoryNotFoundException.cs
- Emitter.cs
- PropertyRef.cs
- InstanceBehavior.cs
- WsdlInspector.cs
- Mutex.cs
- ViewKeyConstraint.cs
- CorrelationTokenInvalidatedHandler.cs
- MailMessageEventArgs.cs
- WeakReferenceEnumerator.cs
- FormsAuthenticationModule.cs
- CompiledXpathExpr.cs
- RemoteCryptoSignHashRequest.cs
- ColumnMap.cs
- Binding.cs
- DrawingGroup.cs
- IISUnsafeMethods.cs
- StructuredProperty.cs
- EntityDataSourceValidationException.cs
- DataGridColumn.cs
- WindowsFormsHost.cs
- PartialCachingControl.cs
- ReversePositionQuery.cs
- DesignerMetadata.cs
- TailPinnedEventArgs.cs
- DropShadowEffect.cs
- DataGridRowClipboardEventArgs.cs
- UriExt.cs
- SymmetricKeyWrap.cs
- ProfileSettingsCollection.cs
- SqlDataSourceStatusEventArgs.cs
- WebPartConnectionsCancelVerb.cs
- Documentation.cs
- AdCreatedEventArgs.cs
- XsltException.cs
- DependencyPropertyKind.cs
- WindowsSecurityTokenAuthenticator.cs
- HitTestWithGeometryDrawingContextWalker.cs
- _RequestCacheProtocol.cs
- _AcceptOverlappedAsyncResult.cs
- TypedReference.cs
- FollowerQueueCreator.cs
- SHA1CryptoServiceProvider.cs
- DocumentGridPage.cs
- ScriptManager.cs
- ClockController.cs
- Timer.cs
- TimeoutStream.cs
- DataTablePropertyDescriptor.cs
- UnsafeNativeMethods.cs
- ValidationEventArgs.cs
- HtmlFormWrapper.cs
- PriorityBindingExpression.cs
- GPPOINT.cs
- DispatcherExceptionEventArgs.cs
- DataGridViewColumnHeaderCell.cs
- NamespaceInfo.cs
- ApplicationDirectoryMembershipCondition.cs
- DataGridViewSelectedColumnCollection.cs
- TextOnlyOutput.cs
- UnionCodeGroup.cs
- SystemException.cs
- XmlSerializerAssemblyAttribute.cs
- XmlNodeChangedEventManager.cs
- EpmContentSerializerBase.cs
- GeneralTransform3DTo2DTo3D.cs
- CdpEqualityComparer.cs
- MetaTable.cs
- PerformanceCountersElement.cs
- WindowsGraphicsCacheManager.cs
- ThemeableAttribute.cs
- IndexingContentUnit.cs