Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / SQLTypes / SQLInt16.cs / 1305376 / SQLInt16.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SqlInt16.cs // // Create by: JunFang // // Purpose: Implementation of SqlInt16 which is equivalent to // data type "smallint" in SQL Server // // Notes: // // History: // // 11/1/99 JunFang Created. // // @EndHeader@ //************************************************************************* using System; using System.Data.Common; using System.Runtime.InteropServices; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; namespace System.Data.SqlTypes { ////// [Serializable] [StructLayout(LayoutKind.Sequential)] [XmlSchemaProvider("GetXsdType")] public struct SqlInt16 : INullable, IComparable, IXmlSerializable { private bool m_fNotNull; // false if null private short m_value; private static readonly int O_MASKI2 = ~0x00007fff; // constructor // construct a Null private SqlInt16(bool fNull) { m_fNotNull = false; m_value = 0; } ////// Represents a 16-bit signed integer to be stored in /// or retrieved from a database. /// ////// public SqlInt16(short value) { m_value = value; m_fNotNull = true; } // INullable ///[To be supplied.] ////// public bool IsNull { get { return !m_fNotNull;} } // property: Value ///[To be supplied.] ////// public short Value { get { if (m_fNotNull) return m_value; else throw new SqlNullValueException(); } } // Implicit conversion from short to SqlInt16 ///[To be supplied.] ////// public static implicit operator SqlInt16(short x) { return new SqlInt16(x); } // Explicit conversion from SqlInt16 to short. Throw exception if x is Null. ///[To be supplied.] ////// public static explicit operator short(SqlInt16 x) { return x.Value; } ///[To be supplied.] ////// public override String ToString() { return IsNull ? SQLResource.NullString : m_value.ToString((IFormatProvider)null); } ///[To be supplied.] ////// public static SqlInt16 Parse(String s) { if (s == SQLResource.NullString) return SqlInt16.Null; else return new SqlInt16(Int16.Parse(s, (IFormatProvider)null)); } // Unary operators ///[To be supplied.] ////// public static SqlInt16 operator -(SqlInt16 x) { return x.IsNull ? Null : new SqlInt16((short)-x.m_value); } ///[To be supplied.] ////// public static SqlInt16 operator ~(SqlInt16 x) { return x.IsNull ? Null : new SqlInt16((short)~x.m_value); } // Binary operators // Arithmetic operators ///[To be supplied.] ////// public static SqlInt16 operator +(SqlInt16 x, SqlInt16 y) { if (x.IsNull || y.IsNull) return Null; int iResult = (int)x.m_value + (int)y.m_value; if ((((iResult >> 15) ^ (iResult >> 16)) & 1) != 0) // Bit 15 != bit 16 throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)iResult); } ///[To be supplied.] ////// public static SqlInt16 operator -(SqlInt16 x, SqlInt16 y) { if (x.IsNull || y.IsNull) return Null; int iResult = (int)x.m_value - (int)y.m_value; if ((((iResult >> 15) ^ (iResult >> 16)) & 1) != 0) // Bit 15 != bit 16 throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)iResult); } ///[To be supplied.] ////// public static SqlInt16 operator *(SqlInt16 x, SqlInt16 y) { if (x.IsNull || y.IsNull) return Null; int iResult = (int)x.m_value * (int)y.m_value; int iTemp = iResult & O_MASKI2; if (iTemp != 0 && iTemp != O_MASKI2) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)iResult); } ///[To be supplied.] ////// public static SqlInt16 operator /(SqlInt16 x, SqlInt16 y) { if (x.IsNull || y.IsNull) return Null; if (y.m_value != 0) { if ((x.m_value == Int16.MinValue) && (y.m_value == -1)) throw new OverflowException(SQLResource.ArithOverflowMessage); return new SqlInt16((short)(x.m_value / y.m_value)); } else throw new DivideByZeroException(SQLResource.DivideByZeroMessage); } ///[To be supplied.] ////// public static SqlInt16 operator %(SqlInt16 x, SqlInt16 y) { if (x.IsNull || y.IsNull) return Null; if (y.m_value != 0) { if ((x.m_value == Int16.MinValue) && (y.m_value == -1)) throw new OverflowException(SQLResource.ArithOverflowMessage); return new SqlInt16((short)(x.m_value % y.m_value)); } else throw new DivideByZeroException(SQLResource.DivideByZeroMessage); } // Bitwise operators ///[To be supplied.] ////// public static SqlInt16 operator &(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt16((short)(x.m_value & y.m_value)); } ///[To be supplied.] ////// public static SqlInt16 operator |(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt16((short)((ushort)x.m_value | (ushort)y.m_value)); } ///[To be supplied.] ////// public static SqlInt16 operator ^(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt16((short)(x.m_value ^ y.m_value)); } // Implicit conversions // Implicit conversion from SqlBoolean to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlBoolean x) { return x.IsNull ? Null : new SqlInt16((short)(x.ByteValue)); } // Implicit conversion from SqlByte to SqlInt16 ///[To be supplied.] ////// public static implicit operator SqlInt16(SqlByte x) { return x.IsNull ? Null : new SqlInt16((short)(x.Value)); } // Explicit conversions // Explicit conversion from SqlInt32 to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlInt32 x) { if (x.IsNull) return Null; int value = x.Value; if (value > (int)Int16.MaxValue || value < (int)Int16.MinValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)value); } // Explicit conversion from SqlInt64 to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlInt64 x) { if (x.IsNull) return Null; long value = x.Value; if (value > (long)Int16.MaxValue || value < (long)Int16.MinValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)value); } // Explicit conversion from SqlSingle to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlSingle x) { if (x.IsNull) return Null; float value = x.Value; if (value < (float)Int16.MinValue || value > (float)Int16.MaxValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)value); } // Explicit conversion from SqlDouble to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlDouble x) { if (x.IsNull) return Null; double value = x.Value; if (value < (double)Int16.MinValue || value > (double)Int16.MaxValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)value); } // Explicit conversion from SqlMoney to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlMoney x) { return x.IsNull ? Null : new SqlInt16(checked((short)x.ToInt32())); } // Explicit conversion from SqlDecimal to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlDecimal x) { return(SqlInt16)(SqlInt32)x; } // Explicit conversion from SqlString to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlString x) { return x.IsNull ? Null : new SqlInt16(Int16.Parse(x.Value, (IFormatProvider)null)); } // Overloading comparison operators ///[To be supplied.] ////// public static SqlBoolean operator==(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value == y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator!=(SqlInt16 x, SqlInt16 y) { return ! (x == y); } ///[To be supplied.] ////// public static SqlBoolean operator<(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value < y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value > y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator<=(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value <= y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>=(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value >= y.m_value); } //-------------------------------------------------- // Alternative methods for overloaded operators //-------------------------------------------------- // Alternative method for operator ~ public static SqlInt16 OnesComplement(SqlInt16 x) { return ~x; } // Alternative method for operator + public static SqlInt16 Add(SqlInt16 x, SqlInt16 y) { return x + y; } // Alternative method for operator - public static SqlInt16 Subtract(SqlInt16 x, SqlInt16 y) { return x - y; } // Alternative method for operator * public static SqlInt16 Multiply(SqlInt16 x, SqlInt16 y) { return x * y; } // Alternative method for operator / public static SqlInt16 Divide(SqlInt16 x, SqlInt16 y) { return x / y; } // Alternative method for operator % public static SqlInt16 Mod(SqlInt16 x, SqlInt16 y) { return x % y; } public static SqlInt16 Modulus(SqlInt16 x, SqlInt16 y) { return x % y; } // Alternative method for operator & public static SqlInt16 BitwiseAnd(SqlInt16 x, SqlInt16 y) { return x & y; } // Alternative method for operator | public static SqlInt16 BitwiseOr(SqlInt16 x, SqlInt16 y) { return x | y; } // Alternative method for operator ^ public static SqlInt16 Xor(SqlInt16 x, SqlInt16 y) { return x ^ y; } // Alternative method for operator == public static SqlBoolean Equals(SqlInt16 x, SqlInt16 y) { return (x == y); } // Alternative method for operator != public static SqlBoolean NotEquals(SqlInt16 x, SqlInt16 y) { return (x != y); } // Alternative method for operator < public static SqlBoolean LessThan(SqlInt16 x, SqlInt16 y) { return (x < y); } // Alternative method for operator > public static SqlBoolean GreaterThan(SqlInt16 x, SqlInt16 y) { return (x > y); } // Alternative method for operator <= public static SqlBoolean LessThanOrEqual(SqlInt16 x, SqlInt16 y) { return (x <= y); } // Alternative method for operator >= public static SqlBoolean GreaterThanOrEqual(SqlInt16 x, SqlInt16 y) { return (x >= y); } // Alternative method for conversions. public SqlBoolean ToSqlBoolean() { return (SqlBoolean)this; } public SqlByte ToSqlByte() { return (SqlByte)this; } public SqlDouble ToSqlDouble() { return (SqlDouble)this; } public SqlInt32 ToSqlInt32() { return (SqlInt32)this; } public SqlInt64 ToSqlInt64() { return (SqlInt64)this; } public SqlMoney ToSqlMoney() { return (SqlMoney)this; } public SqlDecimal ToSqlDecimal() { return (SqlDecimal)this; } public SqlSingle ToSqlSingle() { return (SqlSingle)this; } public SqlString ToSqlString() { return (SqlString)this; } // IComparable // Compares this object to another object, returning an integer that // indicates the relationship. // Returns a value less than zero if this < object, zero if this = object, // or a value greater than zero if this > object. // null is considered to be less than any instance. // If object is not of same type, this method throws an ArgumentException. ///[To be supplied.] ////// public int CompareTo(Object value) { if (value is SqlInt16) { SqlInt16 i = (SqlInt16)value; return CompareTo(i); } throw ADP.WrongType(value.GetType(), typeof(SqlInt16)); } public int CompareTo(SqlInt16 value) { // If both Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) return value.IsNull ? 0 : -1; else if (value.IsNull) return 1; if (this < value) return -1; if (this > value) return 1; return 0; } // Compares this instance with a specified object ///[To be supplied.] ////// public override bool Equals(Object value) { if (!(value is SqlInt16)) { return false; } SqlInt16 i = (SqlInt16)value; if (i.IsNull || IsNull) return (i.IsNull && IsNull); else return (this == i).Value; } // For hashing purpose ///[To be supplied.] ////// public override int GetHashCode() { return IsNull ? 0 : Value.GetHashCode(); } ///[To be supplied.] ////// XmlSchema IXmlSerializable.GetSchema() { return null; } ///[To be supplied.] ////// void IXmlSerializable.ReadXml(XmlReader reader) { string isNull = reader.GetAttribute("nil", XmlSchema.InstanceNamespace); if (isNull != null && XmlConvert.ToBoolean(isNull)) { // VSTFDevDiv# 479603 - SqlTypes read null value infinitely and never read the next value. Fix - Read the next value. reader.ReadElementString(); m_fNotNull = false; } else { m_value = XmlConvert.ToInt16(reader.ReadElementString()); m_fNotNull = true; } } ///[To be supplied.] ////// void IXmlSerializable.WriteXml(XmlWriter writer) { if (IsNull) { writer.WriteAttributeString("xsi", "nil", XmlSchema.InstanceNamespace, "true"); } else { writer.WriteString(XmlConvert.ToString(m_value)); } } ///[To be supplied.] ////// public static XmlQualifiedName GetXsdType(XmlSchemaSet schemaSet) { return new XmlQualifiedName("short", XmlSchema.Namespace); } ///[To be supplied.] ////// public static readonly SqlInt16 Null = new SqlInt16(true); ///[To be supplied.] ////// public static readonly SqlInt16 Zero = new SqlInt16(0); ///[To be supplied.] ////// public static readonly SqlInt16 MinValue = new SqlInt16(Int16.MinValue); ///[To be supplied.] ////// public static readonly SqlInt16 MaxValue = new SqlInt16(Int16.MaxValue); } // SqlInt16 } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SqlInt16.cs // // Create by: JunFang // // Purpose: Implementation of SqlInt16 which is equivalent to // data type "smallint" in SQL Server // // Notes: // // History: // // 11/1/99 JunFang Created. // // @EndHeader@ //************************************************************************* using System; using System.Data.Common; using System.Runtime.InteropServices; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; namespace System.Data.SqlTypes { ////// [Serializable] [StructLayout(LayoutKind.Sequential)] [XmlSchemaProvider("GetXsdType")] public struct SqlInt16 : INullable, IComparable, IXmlSerializable { private bool m_fNotNull; // false if null private short m_value; private static readonly int O_MASKI2 = ~0x00007fff; // constructor // construct a Null private SqlInt16(bool fNull) { m_fNotNull = false; m_value = 0; } ////// Represents a 16-bit signed integer to be stored in /// or retrieved from a database. /// ////// public SqlInt16(short value) { m_value = value; m_fNotNull = true; } // INullable ///[To be supplied.] ////// public bool IsNull { get { return !m_fNotNull;} } // property: Value ///[To be supplied.] ////// public short Value { get { if (m_fNotNull) return m_value; else throw new SqlNullValueException(); } } // Implicit conversion from short to SqlInt16 ///[To be supplied.] ////// public static implicit operator SqlInt16(short x) { return new SqlInt16(x); } // Explicit conversion from SqlInt16 to short. Throw exception if x is Null. ///[To be supplied.] ////// public static explicit operator short(SqlInt16 x) { return x.Value; } ///[To be supplied.] ////// public override String ToString() { return IsNull ? SQLResource.NullString : m_value.ToString((IFormatProvider)null); } ///[To be supplied.] ////// public static SqlInt16 Parse(String s) { if (s == SQLResource.NullString) return SqlInt16.Null; else return new SqlInt16(Int16.Parse(s, (IFormatProvider)null)); } // Unary operators ///[To be supplied.] ////// public static SqlInt16 operator -(SqlInt16 x) { return x.IsNull ? Null : new SqlInt16((short)-x.m_value); } ///[To be supplied.] ////// public static SqlInt16 operator ~(SqlInt16 x) { return x.IsNull ? Null : new SqlInt16((short)~x.m_value); } // Binary operators // Arithmetic operators ///[To be supplied.] ////// public static SqlInt16 operator +(SqlInt16 x, SqlInt16 y) { if (x.IsNull || y.IsNull) return Null; int iResult = (int)x.m_value + (int)y.m_value; if ((((iResult >> 15) ^ (iResult >> 16)) & 1) != 0) // Bit 15 != bit 16 throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)iResult); } ///[To be supplied.] ////// public static SqlInt16 operator -(SqlInt16 x, SqlInt16 y) { if (x.IsNull || y.IsNull) return Null; int iResult = (int)x.m_value - (int)y.m_value; if ((((iResult >> 15) ^ (iResult >> 16)) & 1) != 0) // Bit 15 != bit 16 throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)iResult); } ///[To be supplied.] ////// public static SqlInt16 operator *(SqlInt16 x, SqlInt16 y) { if (x.IsNull || y.IsNull) return Null; int iResult = (int)x.m_value * (int)y.m_value; int iTemp = iResult & O_MASKI2; if (iTemp != 0 && iTemp != O_MASKI2) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)iResult); } ///[To be supplied.] ////// public static SqlInt16 operator /(SqlInt16 x, SqlInt16 y) { if (x.IsNull || y.IsNull) return Null; if (y.m_value != 0) { if ((x.m_value == Int16.MinValue) && (y.m_value == -1)) throw new OverflowException(SQLResource.ArithOverflowMessage); return new SqlInt16((short)(x.m_value / y.m_value)); } else throw new DivideByZeroException(SQLResource.DivideByZeroMessage); } ///[To be supplied.] ////// public static SqlInt16 operator %(SqlInt16 x, SqlInt16 y) { if (x.IsNull || y.IsNull) return Null; if (y.m_value != 0) { if ((x.m_value == Int16.MinValue) && (y.m_value == -1)) throw new OverflowException(SQLResource.ArithOverflowMessage); return new SqlInt16((short)(x.m_value % y.m_value)); } else throw new DivideByZeroException(SQLResource.DivideByZeroMessage); } // Bitwise operators ///[To be supplied.] ////// public static SqlInt16 operator &(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt16((short)(x.m_value & y.m_value)); } ///[To be supplied.] ////// public static SqlInt16 operator |(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt16((short)((ushort)x.m_value | (ushort)y.m_value)); } ///[To be supplied.] ////// public static SqlInt16 operator ^(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? Null : new SqlInt16((short)(x.m_value ^ y.m_value)); } // Implicit conversions // Implicit conversion from SqlBoolean to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlBoolean x) { return x.IsNull ? Null : new SqlInt16((short)(x.ByteValue)); } // Implicit conversion from SqlByte to SqlInt16 ///[To be supplied.] ////// public static implicit operator SqlInt16(SqlByte x) { return x.IsNull ? Null : new SqlInt16((short)(x.Value)); } // Explicit conversions // Explicit conversion from SqlInt32 to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlInt32 x) { if (x.IsNull) return Null; int value = x.Value; if (value > (int)Int16.MaxValue || value < (int)Int16.MinValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)value); } // Explicit conversion from SqlInt64 to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlInt64 x) { if (x.IsNull) return Null; long value = x.Value; if (value > (long)Int16.MaxValue || value < (long)Int16.MinValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)value); } // Explicit conversion from SqlSingle to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlSingle x) { if (x.IsNull) return Null; float value = x.Value; if (value < (float)Int16.MinValue || value > (float)Int16.MaxValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)value); } // Explicit conversion from SqlDouble to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlDouble x) { if (x.IsNull) return Null; double value = x.Value; if (value < (double)Int16.MinValue || value > (double)Int16.MaxValue) throw new OverflowException(SQLResource.ArithOverflowMessage); else return new SqlInt16((short)value); } // Explicit conversion from SqlMoney to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlMoney x) { return x.IsNull ? Null : new SqlInt16(checked((short)x.ToInt32())); } // Explicit conversion from SqlDecimal to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlDecimal x) { return(SqlInt16)(SqlInt32)x; } // Explicit conversion from SqlString to SqlInt16 ///[To be supplied.] ////// public static explicit operator SqlInt16(SqlString x) { return x.IsNull ? Null : new SqlInt16(Int16.Parse(x.Value, (IFormatProvider)null)); } // Overloading comparison operators ///[To be supplied.] ////// public static SqlBoolean operator==(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value == y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator!=(SqlInt16 x, SqlInt16 y) { return ! (x == y); } ///[To be supplied.] ////// public static SqlBoolean operator<(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value < y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value > y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator<=(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value <= y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>=(SqlInt16 x, SqlInt16 y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value >= y.m_value); } //-------------------------------------------------- // Alternative methods for overloaded operators //-------------------------------------------------- // Alternative method for operator ~ public static SqlInt16 OnesComplement(SqlInt16 x) { return ~x; } // Alternative method for operator + public static SqlInt16 Add(SqlInt16 x, SqlInt16 y) { return x + y; } // Alternative method for operator - public static SqlInt16 Subtract(SqlInt16 x, SqlInt16 y) { return x - y; } // Alternative method for operator * public static SqlInt16 Multiply(SqlInt16 x, SqlInt16 y) { return x * y; } // Alternative method for operator / public static SqlInt16 Divide(SqlInt16 x, SqlInt16 y) { return x / y; } // Alternative method for operator % public static SqlInt16 Mod(SqlInt16 x, SqlInt16 y) { return x % y; } public static SqlInt16 Modulus(SqlInt16 x, SqlInt16 y) { return x % y; } // Alternative method for operator & public static SqlInt16 BitwiseAnd(SqlInt16 x, SqlInt16 y) { return x & y; } // Alternative method for operator | public static SqlInt16 BitwiseOr(SqlInt16 x, SqlInt16 y) { return x | y; } // Alternative method for operator ^ public static SqlInt16 Xor(SqlInt16 x, SqlInt16 y) { return x ^ y; } // Alternative method for operator == public static SqlBoolean Equals(SqlInt16 x, SqlInt16 y) { return (x == y); } // Alternative method for operator != public static SqlBoolean NotEquals(SqlInt16 x, SqlInt16 y) { return (x != y); } // Alternative method for operator < public static SqlBoolean LessThan(SqlInt16 x, SqlInt16 y) { return (x < y); } // Alternative method for operator > public static SqlBoolean GreaterThan(SqlInt16 x, SqlInt16 y) { return (x > y); } // Alternative method for operator <= public static SqlBoolean LessThanOrEqual(SqlInt16 x, SqlInt16 y) { return (x <= y); } // Alternative method for operator >= public static SqlBoolean GreaterThanOrEqual(SqlInt16 x, SqlInt16 y) { return (x >= y); } // Alternative method for conversions. public SqlBoolean ToSqlBoolean() { return (SqlBoolean)this; } public SqlByte ToSqlByte() { return (SqlByte)this; } public SqlDouble ToSqlDouble() { return (SqlDouble)this; } public SqlInt32 ToSqlInt32() { return (SqlInt32)this; } public SqlInt64 ToSqlInt64() { return (SqlInt64)this; } public SqlMoney ToSqlMoney() { return (SqlMoney)this; } public SqlDecimal ToSqlDecimal() { return (SqlDecimal)this; } public SqlSingle ToSqlSingle() { return (SqlSingle)this; } public SqlString ToSqlString() { return (SqlString)this; } // IComparable // Compares this object to another object, returning an integer that // indicates the relationship. // Returns a value less than zero if this < object, zero if this = object, // or a value greater than zero if this > object. // null is considered to be less than any instance. // If object is not of same type, this method throws an ArgumentException. ///[To be supplied.] ////// public int CompareTo(Object value) { if (value is SqlInt16) { SqlInt16 i = (SqlInt16)value; return CompareTo(i); } throw ADP.WrongType(value.GetType(), typeof(SqlInt16)); } public int CompareTo(SqlInt16 value) { // If both Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) return value.IsNull ? 0 : -1; else if (value.IsNull) return 1; if (this < value) return -1; if (this > value) return 1; return 0; } // Compares this instance with a specified object ///[To be supplied.] ////// public override bool Equals(Object value) { if (!(value is SqlInt16)) { return false; } SqlInt16 i = (SqlInt16)value; if (i.IsNull || IsNull) return (i.IsNull && IsNull); else return (this == i).Value; } // For hashing purpose ///[To be supplied.] ////// public override int GetHashCode() { return IsNull ? 0 : Value.GetHashCode(); } ///[To be supplied.] ////// XmlSchema IXmlSerializable.GetSchema() { return null; } ///[To be supplied.] ////// void IXmlSerializable.ReadXml(XmlReader reader) { string isNull = reader.GetAttribute("nil", XmlSchema.InstanceNamespace); if (isNull != null && XmlConvert.ToBoolean(isNull)) { // VSTFDevDiv# 479603 - SqlTypes read null value infinitely and never read the next value. Fix - Read the next value. reader.ReadElementString(); m_fNotNull = false; } else { m_value = XmlConvert.ToInt16(reader.ReadElementString()); m_fNotNull = true; } } ///[To be supplied.] ////// void IXmlSerializable.WriteXml(XmlWriter writer) { if (IsNull) { writer.WriteAttributeString("xsi", "nil", XmlSchema.InstanceNamespace, "true"); } else { writer.WriteString(XmlConvert.ToString(m_value)); } } ///[To be supplied.] ////// public static XmlQualifiedName GetXsdType(XmlSchemaSet schemaSet) { return new XmlQualifiedName("short", XmlSchema.Namespace); } ///[To be supplied.] ////// public static readonly SqlInt16 Null = new SqlInt16(true); ///[To be supplied.] ////// public static readonly SqlInt16 Zero = new SqlInt16(0); ///[To be supplied.] ////// public static readonly SqlInt16 MinValue = new SqlInt16(Int16.MinValue); ///[To be supplied.] ////// public static readonly SqlInt16 MaxValue = new SqlInt16(Int16.MaxValue); } // SqlInt16 } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
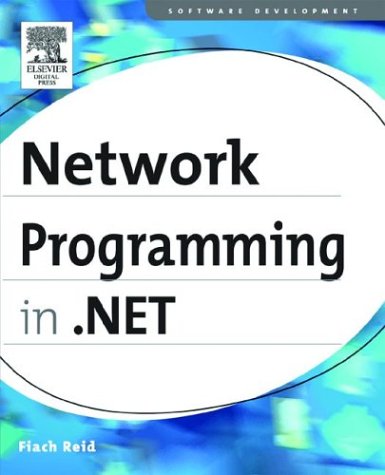
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventBuilder.cs
- WizardStepBase.cs
- DefaultAsyncDataDispatcher.cs
- AspNetSynchronizationContext.cs
- SchemaInfo.cs
- HttpServerVarsCollection.cs
- DataGridViewCellStateChangedEventArgs.cs
- PixelFormatConverter.cs
- DocumentPageView.cs
- HandlerBase.cs
- Models.cs
- BinaryObjectReader.cs
- AccessDataSource.cs
- TrackingStringDictionary.cs
- RadioButtonRenderer.cs
- DataGridViewCellStyle.cs
- QilIterator.cs
- XmlTextWriter.cs
- ColumnTypeConverter.cs
- ProtocolsConfigurationHandler.cs
- AVElementHelper.cs
- BehaviorEditorPart.cs
- BuildResultCache.cs
- ImageSource.cs
- CodeMemberMethod.cs
- GridViewDeleteEventArgs.cs
- CustomBindingCollectionElement.cs
- DataGridViewCellValidatingEventArgs.cs
- ByteAnimation.cs
- Bookmark.cs
- ListBoxItemWrapperAutomationPeer.cs
- OdbcUtils.cs
- MeshGeometry3D.cs
- ContextMenuStripGroup.cs
- RewritingValidator.cs
- TableRowGroupCollection.cs
- SecurityTokenProvider.cs
- StylusCollection.cs
- ListViewInsertEventArgs.cs
- QueryHandler.cs
- DBCSCodePageEncoding.cs
- ColumnResult.cs
- CharacterMetrics.cs
- GridViewCellAutomationPeer.cs
- Boolean.cs
- _Rfc2616CacheValidators.cs
- TcpAppDomainProtocolHandler.cs
- Converter.cs
- XmlSiteMapProvider.cs
- SafeSecurityHandles.cs
- CmsUtils.cs
- HashAlgorithm.cs
- Serializer.cs
- AffineTransform3D.cs
- DataObjectFieldAttribute.cs
- FileAuthorizationModule.cs
- DataGridViewCellFormattingEventArgs.cs
- RepeaterCommandEventArgs.cs
- SafeCoTaskMem.cs
- XmlName.cs
- FontDialog.cs
- XmlSiteMapProvider.cs
- MissingManifestResourceException.cs
- TableProviderWrapper.cs
- _OSSOCK.cs
- SafeRegistryKey.cs
- MouseButton.cs
- FormsAuthenticationTicket.cs
- RequestQueue.cs
- ConditionalAttribute.cs
- ConfigXmlWhitespace.cs
- OverrideMode.cs
- WorkflowMarkupSerializationProvider.cs
- PnrpPermission.cs
- FlowDocumentReaderAutomationPeer.cs
- CurrencyManager.cs
- RegexCaptureCollection.cs
- ApplicationSecurityInfo.cs
- ToolStripControlHost.cs
- AssemblyResourceLoader.cs
- HandleTable.cs
- FormsIdentity.cs
- util.cs
- BookmarkScopeManager.cs
- HtmlTableRow.cs
- RoutedEventConverter.cs
- TableAdapterManagerGenerator.cs
- ReferencedCategoriesDocument.cs
- SiteMapDataSourceView.cs
- DrawingGroupDrawingContext.cs
- xmlsaver.cs
- Size3D.cs
- SafeRightsManagementEnvironmentHandle.cs
- SimpleType.cs
- XPathNavigatorKeyComparer.cs
- DecoderFallback.cs
- SchemaImporterExtension.cs
- GridLengthConverter.cs
- TextStore.cs
- InputManager.cs