Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / tx / System / Transactions / Oletx / HandleTable.cs / 1305376 / HandleTable.cs
using System; using System.Collections.Generic; using System.Diagnostics; using System.Threading; using System.Transactions.Diagnostics; namespace System.Transactions.Oletx { static class HandleTable { private static DictionaryhandleTable = new Dictionary (256); private static object syncRoot = new object(); private static int currentHandle; public static IntPtr AllocHandle(object target) { lock(syncRoot) { int handle = FindAvailableHandle(); handleTable.Add(handle, target); return new IntPtr(handle); } } public static bool FreeHandle(IntPtr handle) { Debug.Assert(handle != IntPtr.Zero, "handle is invalid"); lock(syncRoot) { return handleTable.Remove(handle.ToInt32()); } } public static object FindHandle(IntPtr handle) { Debug.Assert(handle != IntPtr.Zero, "handle is invalid"); lock(syncRoot) { object target; if (!handleTable.TryGetValue(handle.ToInt32(), out target)) { return null; } return target; } } private static int FindAvailableHandle() { int handle = 0; do { handle = (++currentHandle != 0) ? currentHandle : ++currentHandle; } while(handleTable.ContainsKey(handle)); Debug.Assert(handle != 0, "invalid handle selected"); return handle; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Diagnostics; using System.Threading; using System.Transactions.Diagnostics; namespace System.Transactions.Oletx { static class HandleTable { private static Dictionary handleTable = new Dictionary (256); private static object syncRoot = new object(); private static int currentHandle; public static IntPtr AllocHandle(object target) { lock(syncRoot) { int handle = FindAvailableHandle(); handleTable.Add(handle, target); return new IntPtr(handle); } } public static bool FreeHandle(IntPtr handle) { Debug.Assert(handle != IntPtr.Zero, "handle is invalid"); lock(syncRoot) { return handleTable.Remove(handle.ToInt32()); } } public static object FindHandle(IntPtr handle) { Debug.Assert(handle != IntPtr.Zero, "handle is invalid"); lock(syncRoot) { object target; if (!handleTable.TryGetValue(handle.ToInt32(), out target)) { return null; } return target; } } private static int FindAvailableHandle() { int handle = 0; do { handle = (++currentHandle != 0) ? currentHandle : ++currentHandle; } while(handleTable.ContainsKey(handle)); Debug.Assert(handle != 0, "invalid handle selected"); return handle; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
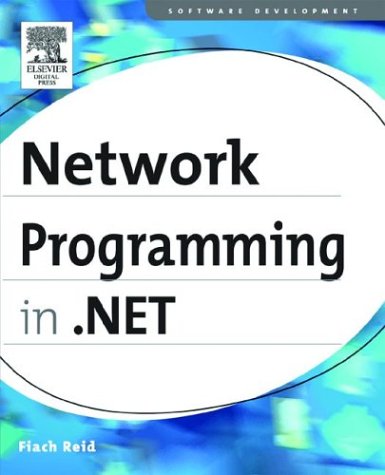
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataColumnChangeEvent.cs
- SystemIPInterfaceProperties.cs
- ReferenceEqualityComparer.cs
- DefaultSerializationProviderAttribute.cs
- StringConverter.cs
- WebPartConnectionsConnectVerb.cs
- ProjectionCamera.cs
- LambdaCompiler.Address.cs
- TickBar.cs
- SqlRowUpdatingEvent.cs
- StrokeNodeEnumerator.cs
- View.cs
- SecurityMode.cs
- SoapCodeExporter.cs
- ValidationHelpers.cs
- FrameworkElementFactory.cs
- StoryFragments.cs
- ComplexObject.cs
- BufferModesCollection.cs
- ComponentDispatcherThread.cs
- DynamicActionMessageFilter.cs
- ToolStripPanelRow.cs
- ErrorEventArgs.cs
- PasswordPropertyTextAttribute.cs
- InstanceKeyNotReadyException.cs
- UIElement.cs
- Graphics.cs
- SchemaTableOptionalColumn.cs
- BitmapSizeOptions.cs
- CapiSymmetricAlgorithm.cs
- CodeEntryPointMethod.cs
- ClientUtils.cs
- XmlDataProvider.cs
- WebInvokeAttribute.cs
- ImageIndexConverter.cs
- X509ScopedServiceCertificateElementCollection.cs
- ConvertEvent.cs
- VisualStyleElement.cs
- MultiPageTextView.cs
- TransactionContext.cs
- WaitHandle.cs
- AdornerPresentationContext.cs
- SspiNegotiationTokenProviderState.cs
- AtlasWeb.Designer.cs
- FieldAccessException.cs
- ListViewGroupConverter.cs
- HandlerBase.cs
- PkcsMisc.cs
- ServiceHttpHandlerFactory.cs
- CodeTypeParameter.cs
- TextTabProperties.cs
- OutputCacheSettingsSection.cs
- ConfigXmlCDataSection.cs
- _PooledStream.cs
- OleDbDataReader.cs
- DependencyObjectProvider.cs
- FieldMetadata.cs
- MonthChangedEventArgs.cs
- FilterQuery.cs
- SQLBoolean.cs
- DictionaryEntry.cs
- HttpFileCollection.cs
- StylusPointPropertyUnit.cs
- ReadOnlyDataSourceView.cs
- ManagementEventArgs.cs
- AssociationSetEnd.cs
- JournalEntryListConverter.cs
- SecurityStateEncoder.cs
- AbsoluteQuery.cs
- MemberExpressionHelper.cs
- MultiSelectRootGridEntry.cs
- SocketPermission.cs
- HtmlInputRadioButton.cs
- PropertyChangedEventArgs.cs
- ErrorsHelper.cs
- DataListItemEventArgs.cs
- ProjectionPathSegment.cs
- ObjectNotFoundException.cs
- Parameter.cs
- PrimitiveXmlSerializers.cs
- PersonalizationEntry.cs
- GroupBox.cs
- DropShadowBitmapEffect.cs
- DeviceContexts.cs
- DocumentViewerBaseAutomationPeer.cs
- ContainerFilterService.cs
- X509CertificateInitiatorServiceCredential.cs
- AssemblyInfo.cs
- QilVisitor.cs
- Matrix3DStack.cs
- ResourceManager.cs
- ExtensibleClassFactory.cs
- SByteConverter.cs
- MenuCommand.cs
- ColumnMapCopier.cs
- ToolStripLabel.cs
- DependencyPropertyAttribute.cs
- ScrollChangedEventArgs.cs
- AndAlso.cs
- DateTimeOffset.cs