Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Automation / Peers / FlowDocumentReaderAutomationPeer.cs / 1 / FlowDocumentReaderAutomationPeer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: FlowDocumentReaderAutomationPeer.cs // // Description: AutomationPeer associated with FlowDocumentReader. // //--------------------------------------------------------------------------- using System.Collections.Generic; // Listusing System.Windows.Automation.Provider; // IMultipleViewProvider using System.Windows.Controls; // FlowDocumentReader using System.Windows.Documents; // FlowDocument using MS.Internal; // Invariant namespace System.Windows.Automation.Peers { /// /// AutomationPeer associated with FlowDocumentScrollViewer. /// public class FlowDocumentReaderAutomationPeer : FrameworkElementAutomationPeer, IMultipleViewProvider { ////// Constructor. /// /// Owner of the AutomationPeer. public FlowDocumentReaderAutomationPeer(FlowDocumentReader owner) : base(owner) { } ////// public override object GetPattern(PatternInterface patternInterface) { object returnValue = null; if (patternInterface == PatternInterface.MultipleView) { returnValue = this; } return returnValue; } ////// /// ////// /// AutomationPeer associated with FlowDocumentScrollViewer returns an AutomationPeer /// for hosted Document and for elements in the style. /// protected override ListGetChildrenCore() { // Get children for all elements in the style. List children = base.GetChildrenCore(); // Add AutomationPeer associated with the document. // Make it the first child of the collection. FlowDocument document = ((FlowDocumentReader)Owner).Document; if (document != null) { AutomationPeer documentPeer = ContentElementAutomationPeer.CreatePeerForElement(document); if (_documentPeer != documentPeer) { if (_documentPeer != null) { _documentPeer.OnDisconnected(); } _documentPeer = documentPeer as DocumentAutomationPeer; } if (documentPeer != null) { if (children == null) { children = new List (); } children.Add(documentPeer); } } return children; } /// /// protected override string GetClassNameCore() { return "FlowDocumentReader"; } ////// /// This helper synchronously fires automation PropertyChange event /// in responce to current view mode change. /// // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseCurrentViewChangedEvent(FlowDocumentReaderViewingMode newMode, FlowDocumentReaderViewingMode oldMode) { if (newMode != oldMode) { RaisePropertyChangedEvent(MultipleViewPatternIdentifiers.CurrentViewProperty, ConvertModeToViewId(newMode), ConvertModeToViewId(oldMode)); } } ////// This helper synchronously fires automation PropertyChange event /// in responce to supported views change. /// // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseSupportedViewsChangedEvent(DependencyPropertyChangedEventArgs e) { bool newSingle, oldSingle, newFacing, oldFacing, newScroll, oldScroll; if (e.Property == FlowDocumentReader.IsPageViewEnabledProperty) { newSingle = (bool)e.NewValue; oldSingle = (bool)e.OldValue; newFacing = oldFacing = FlowDocumentReader.IsTwoPageViewEnabled; newScroll = oldScroll = FlowDocumentReader.IsScrollViewEnabled; } else if (e.Property == FlowDocumentReader.IsTwoPageViewEnabledProperty) { newSingle = oldSingle = FlowDocumentReader.IsPageViewEnabled; newFacing = (bool)e.NewValue; oldFacing = (bool)e.OldValue; newScroll = oldScroll = FlowDocumentReader.IsScrollViewEnabled; } else// if (e.Property == FlowDocumentReader.IsScrollViewEnabledProperty) { newSingle = oldSingle = FlowDocumentReader.IsPageViewEnabled; newFacing = oldFacing = FlowDocumentReader.IsTwoPageViewEnabled; newScroll = (bool)e.NewValue; oldScroll = (bool)e.OldValue; } if (newSingle != oldSingle || newFacing != oldFacing || newScroll != oldScroll) { int[] newViews = GetSupportedViews(newSingle, newFacing, newScroll); int[] oldViews = GetSupportedViews(oldSingle, oldFacing, oldScroll); RaisePropertyChangedEvent(MultipleViewPatternIdentifiers.SupportedViewsProperty, newViews, oldViews); } } //------------------------------------------------------------------- // // Private Members // //------------------------------------------------------------------- #region Private Members private int[] GetSupportedViews(bool single, bool facing, bool scroll) { int count = 0; if (single) { count++; } if (facing) { count++; } if (scroll) { count++; } int[] views = count > 0 ? new int[count] : null; count = 0; if (single) { views[count++] = ConvertModeToViewId(FlowDocumentReaderViewingMode.Page); } if (facing) { views[count++] = ConvertModeToViewId(FlowDocumentReaderViewingMode.TwoPage); } if (scroll) { views[count++] = ConvertModeToViewId(FlowDocumentReaderViewingMode.Scroll); } return views; } ////// Converts viewing mode to view id. /// private int ConvertModeToViewId(FlowDocumentReaderViewingMode mode) { return (int)mode; } ////// Converts view id to viewing mode. /// private FlowDocumentReaderViewingMode ConvertViewIdToMode(int viewId) { Invariant.Assert(viewId >= 0 && viewId <= 2); return (FlowDocumentReaderViewingMode)viewId; } ////// FlowDocumentReader associated with the peer. /// private FlowDocumentReader FlowDocumentReader { get { return (FlowDocumentReader)Owner; } } private DocumentAutomationPeer _documentPeer; #endregion Private Members //-------------------------------------------------------------------- // // IMultipleViewProvider Members // //------------------------------------------------------------------- #region IMultipleViewProvider Members ////// string IMultipleViewProvider.GetViewName(int viewId) { string name = string.Empty; if (viewId >= 0 && viewId <= 2) { FlowDocumentReaderViewingMode mode = ConvertViewIdToMode(viewId); if (mode == FlowDocumentReaderViewingMode.Page) { name = SR.Get(SRID.FlowDocumentReader_MultipleViewProvider_PageViewName); } else if (mode == FlowDocumentReaderViewingMode.TwoPage) { name = SR.Get(SRID.FlowDocumentReader_MultipleViewProvider_TwoPageViewName); } else if (mode == FlowDocumentReaderViewingMode.Scroll) { name = SR.Get(SRID.FlowDocumentReader_MultipleViewProvider_ScrollViewName); } } return name; } ////// /// void IMultipleViewProvider.SetCurrentView(int viewId) { if (viewId >= 0 && viewId <= 2) { FlowDocumentReader.ViewingMode = ConvertViewIdToMode(viewId); } } ////// /// int IMultipleViewProvider.CurrentView { get { return ConvertModeToViewId(FlowDocumentReader.ViewingMode); } } ////// /// int[] IMultipleViewProvider.GetSupportedViews() { return GetSupportedViews( FlowDocumentReader.IsPageViewEnabled, FlowDocumentReader.IsTwoPageViewEnabled, FlowDocumentReader.IsScrollViewEnabled); } #endregion IMultipleViewProvider Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: FlowDocumentReaderAutomationPeer.cs // // Description: AutomationPeer associated with FlowDocumentReader. // //--------------------------------------------------------------------------- using System.Collections.Generic; // List/// using System.Windows.Automation.Provider; // IMultipleViewProvider using System.Windows.Controls; // FlowDocumentReader using System.Windows.Documents; // FlowDocument using MS.Internal; // Invariant namespace System.Windows.Automation.Peers { /// /// AutomationPeer associated with FlowDocumentScrollViewer. /// public class FlowDocumentReaderAutomationPeer : FrameworkElementAutomationPeer, IMultipleViewProvider { ////// Constructor. /// /// Owner of the AutomationPeer. public FlowDocumentReaderAutomationPeer(FlowDocumentReader owner) : base(owner) { } ////// public override object GetPattern(PatternInterface patternInterface) { object returnValue = null; if (patternInterface == PatternInterface.MultipleView) { returnValue = this; } return returnValue; } ////// /// ////// /// AutomationPeer associated with FlowDocumentScrollViewer returns an AutomationPeer /// for hosted Document and for elements in the style. /// protected override ListGetChildrenCore() { // Get children for all elements in the style. List children = base.GetChildrenCore(); // Add AutomationPeer associated with the document. // Make it the first child of the collection. FlowDocument document = ((FlowDocumentReader)Owner).Document; if (document != null) { AutomationPeer documentPeer = ContentElementAutomationPeer.CreatePeerForElement(document); if (_documentPeer != documentPeer) { if (_documentPeer != null) { _documentPeer.OnDisconnected(); } _documentPeer = documentPeer as DocumentAutomationPeer; } if (documentPeer != null) { if (children == null) { children = new List (); } children.Add(documentPeer); } } return children; } /// /// protected override string GetClassNameCore() { return "FlowDocumentReader"; } ////// /// This helper synchronously fires automation PropertyChange event /// in responce to current view mode change. /// // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseCurrentViewChangedEvent(FlowDocumentReaderViewingMode newMode, FlowDocumentReaderViewingMode oldMode) { if (newMode != oldMode) { RaisePropertyChangedEvent(MultipleViewPatternIdentifiers.CurrentViewProperty, ConvertModeToViewId(newMode), ConvertModeToViewId(oldMode)); } } ////// This helper synchronously fires automation PropertyChange event /// in responce to supported views change. /// // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseSupportedViewsChangedEvent(DependencyPropertyChangedEventArgs e) { bool newSingle, oldSingle, newFacing, oldFacing, newScroll, oldScroll; if (e.Property == FlowDocumentReader.IsPageViewEnabledProperty) { newSingle = (bool)e.NewValue; oldSingle = (bool)e.OldValue; newFacing = oldFacing = FlowDocumentReader.IsTwoPageViewEnabled; newScroll = oldScroll = FlowDocumentReader.IsScrollViewEnabled; } else if (e.Property == FlowDocumentReader.IsTwoPageViewEnabledProperty) { newSingle = oldSingle = FlowDocumentReader.IsPageViewEnabled; newFacing = (bool)e.NewValue; oldFacing = (bool)e.OldValue; newScroll = oldScroll = FlowDocumentReader.IsScrollViewEnabled; } else// if (e.Property == FlowDocumentReader.IsScrollViewEnabledProperty) { newSingle = oldSingle = FlowDocumentReader.IsPageViewEnabled; newFacing = oldFacing = FlowDocumentReader.IsTwoPageViewEnabled; newScroll = (bool)e.NewValue; oldScroll = (bool)e.OldValue; } if (newSingle != oldSingle || newFacing != oldFacing || newScroll != oldScroll) { int[] newViews = GetSupportedViews(newSingle, newFacing, newScroll); int[] oldViews = GetSupportedViews(oldSingle, oldFacing, oldScroll); RaisePropertyChangedEvent(MultipleViewPatternIdentifiers.SupportedViewsProperty, newViews, oldViews); } } //------------------------------------------------------------------- // // Private Members // //------------------------------------------------------------------- #region Private Members private int[] GetSupportedViews(bool single, bool facing, bool scroll) { int count = 0; if (single) { count++; } if (facing) { count++; } if (scroll) { count++; } int[] views = count > 0 ? new int[count] : null; count = 0; if (single) { views[count++] = ConvertModeToViewId(FlowDocumentReaderViewingMode.Page); } if (facing) { views[count++] = ConvertModeToViewId(FlowDocumentReaderViewingMode.TwoPage); } if (scroll) { views[count++] = ConvertModeToViewId(FlowDocumentReaderViewingMode.Scroll); } return views; } ////// Converts viewing mode to view id. /// private int ConvertModeToViewId(FlowDocumentReaderViewingMode mode) { return (int)mode; } ////// Converts view id to viewing mode. /// private FlowDocumentReaderViewingMode ConvertViewIdToMode(int viewId) { Invariant.Assert(viewId >= 0 && viewId <= 2); return (FlowDocumentReaderViewingMode)viewId; } ////// FlowDocumentReader associated with the peer. /// private FlowDocumentReader FlowDocumentReader { get { return (FlowDocumentReader)Owner; } } private DocumentAutomationPeer _documentPeer; #endregion Private Members //-------------------------------------------------------------------- // // IMultipleViewProvider Members // //------------------------------------------------------------------- #region IMultipleViewProvider Members ////// string IMultipleViewProvider.GetViewName(int viewId) { string name = string.Empty; if (viewId >= 0 && viewId <= 2) { FlowDocumentReaderViewingMode mode = ConvertViewIdToMode(viewId); if (mode == FlowDocumentReaderViewingMode.Page) { name = SR.Get(SRID.FlowDocumentReader_MultipleViewProvider_PageViewName); } else if (mode == FlowDocumentReaderViewingMode.TwoPage) { name = SR.Get(SRID.FlowDocumentReader_MultipleViewProvider_TwoPageViewName); } else if (mode == FlowDocumentReaderViewingMode.Scroll) { name = SR.Get(SRID.FlowDocumentReader_MultipleViewProvider_ScrollViewName); } } return name; } ////// /// void IMultipleViewProvider.SetCurrentView(int viewId) { if (viewId >= 0 && viewId <= 2) { FlowDocumentReader.ViewingMode = ConvertViewIdToMode(viewId); } } ////// /// int IMultipleViewProvider.CurrentView { get { return ConvertModeToViewId(FlowDocumentReader.ViewingMode); } } ////// /// int[] IMultipleViewProvider.GetSupportedViews() { return GetSupportedViews( FlowDocumentReader.IsPageViewEnabled, FlowDocumentReader.IsTwoPageViewEnabled, FlowDocumentReader.IsScrollViewEnabled); } #endregion IMultipleViewProvider Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
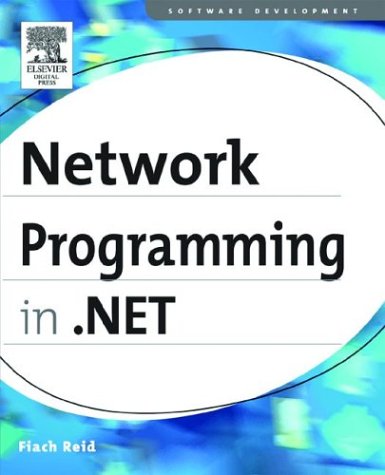
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeEntryPointMethod.cs
- DataSysAttribute.cs
- BrowserCapabilitiesFactory.cs
- DataGridViewDataErrorEventArgs.cs
- XmlAnyElementAttribute.cs
- RuntimeResourceSet.cs
- GrammarBuilderDictation.cs
- UpdatePanelControlTrigger.cs
- AppDomain.cs
- SpecialNameAttribute.cs
- DbDataReader.cs
- TextSimpleMarkerProperties.cs
- Drawing.cs
- MethodSignatureGenerator.cs
- localization.cs
- ScriptServiceAttribute.cs
- WindowsFormsSynchronizationContext.cs
- GridViewHeaderRowPresenter.cs
- DesignerView.cs
- SelectionService.cs
- MessagePartProtectionMode.cs
- UnsafeNativeMethods.cs
- CaseInsensitiveOrdinalStringComparer.cs
- DispatcherHooks.cs
- Compensation.cs
- CatalogPartChrome.cs
- EncodingTable.cs
- SafeLibraryHandle.cs
- DataSourceHelper.cs
- WebPartAddingEventArgs.cs
- FirstMatchCodeGroup.cs
- Span.cs
- CryptoConfig.cs
- ContextStaticAttribute.cs
- PathData.cs
- XmlValidatingReader.cs
- WindowsListViewGroupSubsetLink.cs
- ServiceOperationInfoTypeConverter.cs
- JournalEntry.cs
- SafeHandle.cs
- WebPartsSection.cs
- coordinatorfactory.cs
- LeafCellTreeNode.cs
- ConnectionPoint.cs
- Model3D.cs
- PathFigureCollection.cs
- BinaryReader.cs
- GenericEnumConverter.cs
- RemotingConfigParser.cs
- FormViewInsertedEventArgs.cs
- Int64Storage.cs
- XamlFigureLengthSerializer.cs
- MessageProtectionOrder.cs
- ECDiffieHellmanCngPublicKey.cs
- SqlBulkCopyColumnMapping.cs
- XsltOutput.cs
- Zone.cs
- UriParserTemplates.cs
- TableItemPattern.cs
- QilValidationVisitor.cs
- Psha1DerivedKeyGenerator.cs
- PaperSource.cs
- XXXInfos.cs
- OnOperation.cs
- PostBackOptions.cs
- XmlCustomFormatter.cs
- MaterialCollection.cs
- ParameterBuilder.cs
- XPathBinder.cs
- IsolatedStorage.cs
- EditBehavior.cs
- FrameworkContentElement.cs
- ManagedIStream.cs
- VectorAnimation.cs
- TypeConstant.cs
- DateTimeValueSerializerContext.cs
- TransportConfigurationTypeElementCollection.cs
- SqlParameterCollection.cs
- StylusPlugInCollection.cs
- ExtenderControl.cs
- TableLayoutColumnStyleCollection.cs
- Rule.cs
- ExceptionValidationRule.cs
- Registry.cs
- CursorConverter.cs
- ListQueryResults.cs
- EditorPartCollection.cs
- baseaxisquery.cs
- DataServiceHostWrapper.cs
- Menu.cs
- ITextView.cs
- FileDialog.cs
- TableCellCollection.cs
- Debugger.cs
- MemoryStream.cs
- CodeGenerator.cs
- TrustLevelCollection.cs
- XmlSyndicationContent.cs
- QuinticEase.cs
- DocumentViewerBaseAutomationPeer.cs