Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / MostlySingletonList.cs / 1305376 / MostlySingletonList.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel { using System.Collections.Generic; // Embed this struct in a class to represent a field of that class // that is logically a list, but contains just one item in all but // the rarest of scenarios. When this class must be passed around // in internal APIs, use it as a ref parameter. struct MostlySingletonListwhere T : class { int count; T singleton; List list; public T this[int index] { get { if (this.list == null) { EnsureValidSingletonIndex(index); return this.singleton; } else { return this.list[index]; } } } public int Count { get { return this.count; } } public void Add(T item) { if (this.list == null) { if (this.count == 0) { this.singleton = item; this.count = 1; return; } this.list = new List (); this.list.Add(this.singleton); this.singleton = null; } this.list.Add(item); this.count++; } static bool Compare(T x, T y) { return x == null ? y == null : x.Equals(y); } public bool Contains(T item) { return IndexOf(item) >= 0; } void EnsureValidSingletonIndex(int index) { if (this.count != 1 ) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("count", SR.GetString(SR.ValueMustBeOne))); } if (index != 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("index", SR.GetString(SR.ValueMustBeZero))); } } bool MatchesSingleton(T item) { return this.count == 1 && Compare(this.singleton, item); } public int IndexOf(T item) { if (this.list == null) { return MatchesSingleton(item) ? 0 : -1; } else { return this.list.IndexOf(item); } } public bool Remove(T item) { if (this.list == null) { if (MatchesSingleton(item)) { this.singleton = null; this.count = 0; return true; } else { return false; } } else { bool result = this.list.Remove(item); if (result) { this.count--; } return result; } } public void RemoveAt(int index) { if (this.list == null) { EnsureValidSingletonIndex(index); this.singleton = null; this.count = 0; } else { this.list.RemoveAt(index); this.count--; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel { using System.Collections.Generic; // Embed this struct in a class to represent a field of that class // that is logically a list, but contains just one item in all but // the rarest of scenarios. When this class must be passed around // in internal APIs, use it as a ref parameter. struct MostlySingletonList where T : class { int count; T singleton; List list; public T this[int index] { get { if (this.list == null) { EnsureValidSingletonIndex(index); return this.singleton; } else { return this.list[index]; } } } public int Count { get { return this.count; } } public void Add(T item) { if (this.list == null) { if (this.count == 0) { this.singleton = item; this.count = 1; return; } this.list = new List (); this.list.Add(this.singleton); this.singleton = null; } this.list.Add(item); this.count++; } static bool Compare(T x, T y) { return x == null ? y == null : x.Equals(y); } public bool Contains(T item) { return IndexOf(item) >= 0; } void EnsureValidSingletonIndex(int index) { if (this.count != 1 ) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("count", SR.GetString(SR.ValueMustBeOne))); } if (index != 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("index", SR.GetString(SR.ValueMustBeZero))); } } bool MatchesSingleton(T item) { return this.count == 1 && Compare(this.singleton, item); } public int IndexOf(T item) { if (this.list == null) { return MatchesSingleton(item) ? 0 : -1; } else { return this.list.IndexOf(item); } } public bool Remove(T item) { if (this.list == null) { if (MatchesSingleton(item)) { this.singleton = null; this.count = 0; return true; } else { return false; } } else { bool result = this.list.Remove(item); if (result) { this.count--; } return result; } } public void RemoveAt(int index) { if (this.list == null) { EnsureValidSingletonIndex(index); this.singleton = null; this.count = 0; } else { this.list.RemoveAt(index); this.count--; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
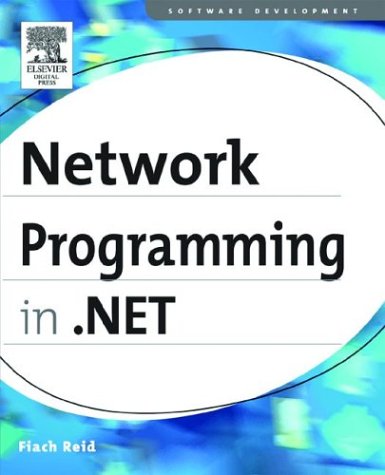
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConvertersCollection.cs
- GridViewColumnCollectionChangedEventArgs.cs
- ResXBuildProvider.cs
- IRCollection.cs
- DataGridViewTopRowAccessibleObject.cs
- UriTemplateLiteralQueryValue.cs
- TextBoxAutoCompleteSourceConverter.cs
- WinFormsSpinner.cs
- MenuRenderer.cs
- NetCodeGroup.cs
- OdbcTransaction.cs
- EntityKey.cs
- thaishape.cs
- SizeChangedInfo.cs
- CustomExpression.cs
- DataGridViewCellConverter.cs
- IPHostEntry.cs
- BitmapEffectDrawingContent.cs
- WebPartTransformer.cs
- HttpConfigurationContext.cs
- CodeDOMUtility.cs
- AttributeSetAction.cs
- SignalGate.cs
- FaultFormatter.cs
- DesignerObjectListAdapter.cs
- ImageAttributes.cs
- FormViewDesigner.cs
- MdiWindowListStrip.cs
- FastPropertyAccessor.cs
- TcpChannelHelper.cs
- TrustManagerMoreInformation.cs
- ServiceReference.cs
- RenderContext.cs
- ObjectCloneHelper.cs
- WebPartDescription.cs
- DetailsViewAutoFormat.cs
- ByteStreamGeometryContext.cs
- HttpCapabilitiesEvaluator.cs
- SplineKeyFrames.cs
- ThreadSafeList.cs
- ValidatorCompatibilityHelper.cs
- NotConverter.cs
- GeometryValueSerializer.cs
- ServiceDurableInstanceContextProvider.cs
- ApplicationFileCodeDomTreeGenerator.cs
- PerformanceCounterPermissionEntry.cs
- TextBox.cs
- RawKeyboardInputReport.cs
- COM2IDispatchConverter.cs
- HtmlListAdapter.cs
- InfoCard.cs
- OwnerDrawPropertyBag.cs
- ZipIOLocalFileDataDescriptor.cs
- MatrixTransform.cs
- DependencyPropertyValueSerializer.cs
- CombinedGeometry.cs
- OleStrCAMarshaler.cs
- cookiecontainer.cs
- QilStrConcatenator.cs
- ModelVisual3D.cs
- SQLInt32.cs
- MailHeaderInfo.cs
- PageBuildProvider.cs
- BitmapVisualManager.cs
- PatternMatcher.cs
- PrincipalPermission.cs
- BCLDebug.cs
- TdsRecordBufferSetter.cs
- SqlRowUpdatingEvent.cs
- ImageSourceConverter.cs
- ConfigurationSectionCollection.cs
- ButtonDesigner.cs
- TabPanel.cs
- CollaborationHelperFunctions.cs
- Solver.cs
- PropertyOverridesDialog.cs
- CacheEntry.cs
- WindowsStreamSecurityUpgradeProvider.cs
- InstanceStoreQueryResult.cs
- DataBinder.cs
- FormsIdentity.cs
- PrintEvent.cs
- ConsumerConnectionPointCollection.cs
- DataDocumentXPathNavigator.cs
- BinaryConverter.cs
- ClockController.cs
- PersonalizationStateQuery.cs
- WinFormsComponentEditor.cs
- ConfigXmlWhitespace.cs
- SliderAutomationPeer.cs
- WebEvents.cs
- EntityDataSourceSelectedEventArgs.cs
- TabPanel.cs
- PrivacyNoticeBindingElementImporter.cs
- ToolbarAUtomationPeer.cs
- BooleanAnimationBase.cs
- SafeNativeMethods.cs
- Part.cs
- BidOverLoads.cs
- SecurityTokenAuthenticator.cs