Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Selectors / SecurityTokenAuthenticator.cs / 1305376 / SecurityTokenAuthenticator.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Selectors { using System.Collections.ObjectModel; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; public abstract class SecurityTokenAuthenticator { protected SecurityTokenAuthenticator() { } public bool CanValidateToken(SecurityToken token) { if (token == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("token"); } return this.CanValidateTokenCore(token); } public ReadOnlyCollectionValidateToken(SecurityToken token) { if (token == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("token"); } if (!CanValidateToken(token)) { // warning 56506: Parameter 'token' to this public method must be validated: A null-dereference can occur here. #pragma warning suppress 56506 throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenValidationException(SR.GetString(SR.CannotValidateSecurityTokenType, this, token.GetType()))); } ReadOnlyCollection authorizationPolicies = ValidateTokenCore(token); if (authorizationPolicies == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenValidationException(SR.GetString(SR.CannotValidateSecurityTokenType, this, token.GetType()))); } return authorizationPolicies; } protected abstract bool CanValidateTokenCore(SecurityToken token); protected abstract ReadOnlyCollection ValidateTokenCore(SecurityToken token); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
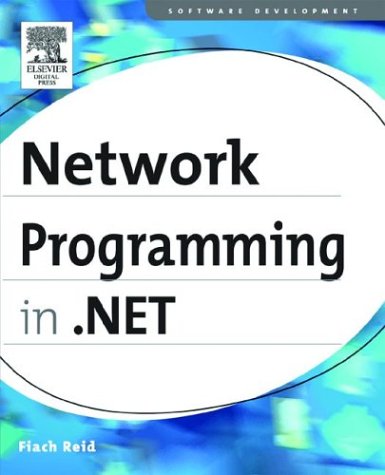
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlPhoneCallAdapter.cs
- NumberFunctions.cs
- DeviceFiltersSection.cs
- EdgeProfileValidation.cs
- XmlObjectSerializerWriteContextComplex.cs
- Drawing.cs
- FormsAuthenticationConfiguration.cs
- OdbcConnectionOpen.cs
- BroadcastEventHelper.cs
- CheckBox.cs
- DSASignatureDeformatter.cs
- ReferentialConstraint.cs
- SignatureDescription.cs
- FileDialog_Vista_Interop.cs
- _ShellExpression.cs
- InheritablePropertyChangeInfo.cs
- NavigationEventArgs.cs
- SecurityTokenTypes.cs
- GuidelineSet.cs
- DesignSurfaceEvent.cs
- PerformanceCounterPermission.cs
- FastEncoderWindow.cs
- DbProviderServices.cs
- EntityContainerEntitySet.cs
- DataSourceXmlSubItemAttribute.cs
- StandardCommandToolStripMenuItem.cs
- HierarchicalDataBoundControlAdapter.cs
- ConditionalAttribute.cs
- ParagraphResult.cs
- WizardPanel.cs
- CharacterMetricsDictionary.cs
- DesignerActionKeyboardBehavior.cs
- TabPanel.cs
- DetailsViewDeletedEventArgs.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- InvocationExpression.cs
- CompilerResults.cs
- KerberosSecurityTokenProvider.cs
- PeekCompletedEventArgs.cs
- HeaderLabel.cs
- AuthenticationModuleElementCollection.cs
- Wrapper.cs
- ClassHandlersStore.cs
- BufferModesCollection.cs
- Evidence.cs
- RunClient.cs
- SqlCommandSet.cs
- GlobalAclOperationRequirement.cs
- XmlCharacterData.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- MergeExecutor.cs
- Int32.cs
- SymmetricCryptoHandle.cs
- Rethrow.cs
- XDRSchema.cs
- ArraySet.cs
- SortKey.cs
- ControlDesigner.cs
- GiveFeedbackEventArgs.cs
- IPAddress.cs
- HandledMouseEvent.cs
- SmiRecordBuffer.cs
- JsonCollectionDataContract.cs
- MessageQueue.cs
- SmtpException.cs
- DashStyle.cs
- selecteditemcollection.cs
- ListViewInsertEventArgs.cs
- Privilege.cs
- PseudoWebRequest.cs
- BaseProcessor.cs
- CatalogPartCollection.cs
- FilterableAttribute.cs
- GridViewColumnHeader.cs
- MultiView.cs
- ISAPIWorkerRequest.cs
- ApplicationDirectoryMembershipCondition.cs
- SafeNativeMethods.cs
- FieldBuilder.cs
- x509store.cs
- TraceLevelStore.cs
- ParsedAttributeCollection.cs
- ElementInit.cs
- SID.cs
- TaskbarItemInfo.cs
- DataGridViewRowPostPaintEventArgs.cs
- TypeBuilder.cs
- TreeNodeBindingCollection.cs
- GeometryDrawing.cs
- OutputScopeManager.cs
- QueryCreatedEventArgs.cs
- Lasso.cs
- SimpleModelProvider.cs
- SQLDoubleStorage.cs
- BuilderInfo.cs
- CompletedAsyncResult.cs
- Asn1IntegerConverter.cs
- DataControlLinkButton.cs
- ExpressionList.cs
- MediaPlayer.cs