Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / MS / Internal / TraceLevelStore.cs / 2 / TraceLevelStore.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Storage for the "TraceLevel" attached property. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; namespace MS.Internal { ////// This class stores values for the attached property /// PresentationTraceSources.TraceLevel. /// internal static class TraceLevelStore { #region Constructors // // Constructors // #endregion Constructors #region Internal Methods // // Internal Methods // ////// Reads the attached property TraceLevel from the given element. /// internal static PresentationTraceLevel GetTraceLevel(object element) { PresentationTraceLevel result; if (element == null || _dictionary.Count == 0) { result = PresentationTraceLevel.None; } else { lock (_dictionary) { using (SetLookupKey(element)) { if (!_dictionary.TryGetValue(_lookupKey, out result)) { result = PresentationTraceLevel.None; } } } } return result; } ////// Writes the attached property TraceLevel to the given element. /// internal static void SetTraceLevel(object element, PresentationTraceLevel traceLevel) { if (element == null) return; lock (_dictionary) { Key key = new Key(element); if (traceLevel > PresentationTraceLevel.None) { _dictionary[key] = traceLevel; } else { _dictionary.Remove(key); } } } #endregion Internal Methods #region Private Methods // set the lookup key - IDisposable ensures it gets cleared after // use, so that it doesn't hold a reference to the element static IDisposable SetLookupKey(object element) { _lookupKey.Set(element); return _lookupKeyDisposable; } #endregion Private Methods #region Private Fields // // Private Fields // private static Dictionary_dictionary = new Dictionary (); private static Key _lookupKey; private static LookupKeyDisposable _lookupKeyDisposable = new LookupKeyDisposable(); #endregion Private Fields #region Table Keys // the key for the dictionary: <((element)), hashcode> private struct Key { internal Key(object element) { _element = new WeakReference(element); _hashcode = element.GetHashCode(); } internal void Set(object element) { _element = element; _hashcode = element.GetHashCode(); } internal void Clear() { _element = null; _hashcode = 0; } public override int GetHashCode() { #if DEBUG WeakReference wr = _element as WeakReference; object element = (wr != null) ? wr.Target : _element; if (element != null) { int hashcode = element.GetHashCode(); Debug.Assert(hashcode == _hashcode, "hashcodes disagree"); } #endif return _hashcode; } public override bool Equals(object o) { if (o is Key) { WeakReference wr; Key that = (Key)o; if (this._hashcode != that._hashcode) return false; wr = this._element as WeakReference; object s1 = (wr != null) ? wr.Target : this._element; wr = that._element as WeakReference; object s2 = (wr != null) ? wr.Target : that._element; if (s1!=null && s2!=null) return (s1 == s2); else return (this._element == that._element); } else { return false; } } public static bool operator==(Key key1, Key key2) { return key1.Equals(key2); } public static bool operator!=(Key key1, Key key2) { return !key1.Equals(key2); } object _element; // lookup: direct ref. In table: WeakRef int _hashcode; // cached, in case source is GC'd } private class LookupKeyDisposable : IDisposable { public void Dispose() { TraceLevelStore._lookupKey.Clear(); } } #endregion Table Keys } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
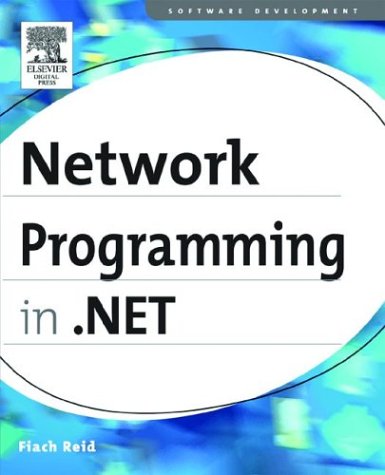
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FunctionImportElement.cs
- OptimalTextSource.cs
- _TransmitFileOverlappedAsyncResult.cs
- SqlDataSourceQueryEditor.cs
- RelationshipEndCollection.cs
- BuiltInExpr.cs
- DayRenderEvent.cs
- DataGridViewCell.cs
- DataControlImageButton.cs
- UIElementAutomationPeer.cs
- ProjectionPlan.cs
- ImplicitInputBrush.cs
- WsdlBuildProvider.cs
- ChildrenQuery.cs
- ClientFormsIdentity.cs
- SessionEndingCancelEventArgs.cs
- PartialTrustHelpers.cs
- XPathNavigatorKeyComparer.cs
- EmptyCollection.cs
- CodeIdentifiers.cs
- BuilderPropertyEntry.cs
- XhtmlBasicFormAdapter.cs
- FamilyTypeface.cs
- GridViewCommandEventArgs.cs
- SqlBuffer.cs
- TraceContextRecord.cs
- TrackPointCollection.cs
- IBuiltInEvidence.cs
- TemplateComponentConnector.cs
- IndentTextWriter.cs
- AssociationEndMember.cs
- UIPermission.cs
- ProfileProvider.cs
- EntityKey.cs
- GridViewRowCollection.cs
- WebBrowserNavigatedEventHandler.cs
- HttpChannelFactory.cs
- Latin1Encoding.cs
- TextBoxBase.cs
- SimpleType.cs
- MediaPlayer.cs
- SqlDataSourceQueryConverter.cs
- TraceUtils.cs
- EpmContentDeSerializer.cs
- WebException.cs
- HyperLinkColumn.cs
- ExtensionElementCollection.cs
- SID.cs
- MenuRendererClassic.cs
- ApplicationSecurityInfo.cs
- HyperLinkStyle.cs
- PersonalizationAdministration.cs
- VectorAnimationBase.cs
- CodeAccessPermission.cs
- CodeArrayCreateExpression.cs
- MergeLocalizationDirectives.cs
- XmlStreamNodeWriter.cs
- SHA384CryptoServiceProvider.cs
- PointF.cs
- XamlSerializerUtil.cs
- XmlSchemaChoice.cs
- DSASignatureFormatter.cs
- Polygon.cs
- DataViewManager.cs
- OracleCommand.cs
- MenuItemBindingCollection.cs
- Size.cs
- Imaging.cs
- CodeTypeReferenceExpression.cs
- HtmlMobileTextWriter.cs
- DocumentGrid.cs
- HttpClientCertificate.cs
- RequestSecurityTokenSerializer.cs
- Pen.cs
- JsonDataContract.cs
- NativeMethodsCLR.cs
- GridViewRowEventArgs.cs
- DescendentsWalker.cs
- ProviderConnectionPointCollection.cs
- WindowsRichEditRange.cs
- Group.cs
- SqlServices.cs
- WizardStepCollectionEditor.cs
- TraceSwitch.cs
- WindowsTooltip.cs
- ObjectViewQueryResultData.cs
- ConnectionStringsExpressionBuilder.cs
- DrawingState.cs
- DataBindingExpressionBuilder.cs
- XmlNodeList.cs
- _SSPIWrapper.cs
- RoleGroupCollection.cs
- PersonalizationEntry.cs
- SmiGettersStream.cs
- ToolStripContentPanelDesigner.cs
- WizardPanel.cs
- InstanceKeyNotReadyException.cs
- BroadcastEventHelper.cs
- EmptyStringExpandableObjectConverter.cs
- odbcmetadatafactory.cs