Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / Data / DefaultAsyncDataDispatcher.cs / 1 / DefaultAsyncDataDispatcher.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Default async scheduler for data operations. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Threading; using System.Windows; using System.Windows.Data; using MS.Internal; namespace MS.Internal.Data { internal class DefaultAsyncDataDispatcher : IAsyncDataDispatcher { //----------------------------------------------------- // // Interface: IAsyncDataDispatcher // //----------------------------------------------------- ///Add a request to the dispatcher's queue void IAsyncDataDispatcher.AddRequest(AsyncDataRequest request) { lock (_list.SyncRoot) { _list.Add(request); } ThreadPool.QueueUserWorkItem(new WaitCallback(ProcessRequest), request); } ///Cancel all requests in the dispatcher's queue void IAsyncDataDispatcher.CancelAllRequests() { lock (_list.SyncRoot) { for (int i=0; i<_list.Count; ++i) { AsyncDataRequest request = (AsyncDataRequest)_list[i]; request.Cancel(); } _list.Clear(); } } //------------------------------------------------------ // // Private methods // //----------------------------------------------------- // Run a single request. This method gets scheduled on a worker thread // from the process ThreadPool. void ProcessRequest(object o) { AsyncDataRequest request = (AsyncDataRequest)o; // PreSharp uses message numbers that the C# compiler doesn't know about. // Disable the C# complaints, per the PreSharp documentation. #pragma warning disable 1634, 1691 // PreSharp complains about catching NullReference (and other) exceptions. // In this case, these are precisely the ones we want to catch the most, // so that a failure on a worker thread doesn't affect the main thread. #pragma warning disable 56500 // run the request - this may take a while try { request.Complete(request.DoWork()); } // Catch all exceptions. There is no app code on the stack, // so the exception isn't actionable by the app. // Yet we don't want to crash the app. catch (Exception ex) { if (CriticalExceptions.IsCriticalException(ex)) throw; request.Fail(ex); } catch // non CLS compliant exception { request.Fail(new InvalidOperationException(SR.Get(SRID.NonCLSException, "processing an async data request"))); } #pragma warning restore 56500 #pragma warning restore 1634, 1691 // remove the request from the list lock (_list.SyncRoot) { _list.Remove(request); } } //------------------------------------------------------ // // Private data // //------------------------------------------------------ ArrayList _list = new ArrayList(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
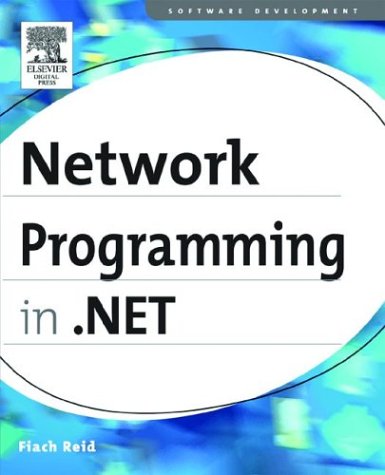
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RequestCachePolicy.cs
- RawStylusSystemGestureInputReport.cs
- DataGridViewCellValidatingEventArgs.cs
- LinearKeyFrames.cs
- EllipseGeometry.cs
- DbConvert.cs
- EventSourceCreationData.cs
- QuaternionAnimation.cs
- NameTable.cs
- WebPartConnectionsCancelVerb.cs
- RecognizedWordUnit.cs
- SamlNameIdentifierClaimResource.cs
- EntityViewGenerator.cs
- CultureInfo.cs
- WindowsListViewGroup.cs
- XmlMemberMapping.cs
- SoapCodeExporter.cs
- TemplatePropertyEntry.cs
- StylusPlugInCollection.cs
- ColumnCollectionEditor.cs
- RedirectionProxy.cs
- SmtpReplyReader.cs
- DataGridViewCellValidatingEventArgs.cs
- LookupBindingPropertiesAttribute.cs
- TextDpi.cs
- ModuleConfigurationInfo.cs
- WinFormsUtils.cs
- XsdBuilder.cs
- UIElement.cs
- PropertyInformation.cs
- DbModificationCommandTree.cs
- TextServicesDisplayAttribute.cs
- HandleCollector.cs
- autovalidator.cs
- TextEffect.cs
- SqlGenerator.cs
- Emitter.cs
- VoiceInfo.cs
- AmbientLight.cs
- ClaimSet.cs
- MissingSatelliteAssemblyException.cs
- IdentityManager.cs
- SafeMemoryMappedFileHandle.cs
- ClientSideQueueItem.cs
- ComponentManagerBroker.cs
- CollectionView.cs
- RSAProtectedConfigurationProvider.cs
- ContentDefinition.cs
- MeshGeometry3D.cs
- RevocationPoint.cs
- SelectionPattern.cs
- DrawingVisualDrawingContext.cs
- EntitySqlQueryCacheEntry.cs
- XmlObjectSerializerWriteContext.cs
- XhtmlBasicListAdapter.cs
- ProtocolsConfigurationEntry.cs
- ConstructorExpr.cs
- MessageSecurityException.cs
- SqlWorkflowPersistenceService.cs
- PageCodeDomTreeGenerator.cs
- Size3D.cs
- AttributeCollection.cs
- CrossAppDomainChannel.cs
- XmlIlTypeHelper.cs
- ObjectDataSourceDisposingEventArgs.cs
- CorrelationManager.cs
- TTSEngineProxy.cs
- wgx_commands.cs
- ByeOperationAsyncResult.cs
- RuntimeWrappedException.cs
- BamlMapTable.cs
- CancellationHandler.cs
- IfJoinedCondition.cs
- SiteMap.cs
- TextSimpleMarkerProperties.cs
- CancellationTokenSource.cs
- SchemaTypeEmitter.cs
- CompositeFontParser.cs
- ScrollChrome.cs
- Visual3DCollection.cs
- AmbientLight.cs
- FaultFormatter.cs
- ObjectPersistData.cs
- CompilerScopeManager.cs
- SimpleWorkerRequest.cs
- WSSecurityOneDotOneSendSecurityHeader.cs
- WebExceptionStatus.cs
- ObjectNotFoundException.cs
- MsmqIntegrationBindingElement.cs
- EventItfInfo.cs
- ColumnMapVisitor.cs
- SoapTypeAttribute.cs
- SqlMetaData.cs
- WizardStepBase.cs
- WindowsRichEditRange.cs
- TextChangedEventArgs.cs
- DeclarativeCatalogPart.cs
- MatrixValueSerializer.cs
- InstanceHandleConflictException.cs
- BasicHttpMessageSecurityElement.cs