Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / VisualTarget.cs / 1 / VisualTarget.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // // History: // 01/06/2005 : ABaioura - Created. // //----------------------------------------------------------------------------- using System.Windows.Media; using System.Windows.Media.Composition; using System.Security; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// /// public class VisualTarget : CompositionTarget { //--------------------------------------------------------------------- // // Constructors // //--------------------------------------------------------------------- #region Constructors ////// VisualTarget /// public VisualTarget(HostVisual hostVisual) { if (hostVisual == null) { throw new ArgumentNullException("hostVisual"); } _hostVisual = hostVisual; _connected = false; MediaContext.RegisterICompositionTarget(Dispatcher, this); } ////// This function gets called when VisualTarget is created on the channel /// i.e. from CreateUCEResources. /// private void BeginHosting() { Debug.Assert(!_connected); try { // // Initiate hosting by the specified host visual. // _hostVisual.BeginHosting(this); _connected = true; } catch { // // If exception has occurred after we have registered with // MediaContext, we need to unregister to properly release // allocated resources. // NOTE: We need to properly unregister in a disconnected state // MediaContext.UnregisterICompositionTarget(Dispatcher, this); throw; } } internal override void CreateUCEResources(DUCE.Channel channel, DUCE.Channel outOfBandChannel) { Debug.Assert(channel != null); Debug.Assert(outOfBandChannel != null); if (!channel.IsLayeredWindowChannel) { _outOfBandChannel = outOfBandChannel; // create visual target resources base.CreateUCEResources(channel, outOfBandChannel); // Update state to propagate flags as necessary StateChangedCallback( new object[] { HostStateFlags.None }); // // Addref content node on the channel. We need extra reference // on that node so that it does not get immediately released // when Dispose is called. Actual release of the node needs // to be synchronized with node disconnect by the host. // bool resourceCreated = _contentRoot.CreateOrAddRefOnChannel(outOfBandChannel, s_contentRootType); Debug.Assert(!resourceCreated); _contentRoot.CreateOrAddRefOnChannel(channel, s_contentRootType); BeginHosting(); } } #endregion Constructors //---------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties ////// Returns matrix that can be used to transform coordinates from this /// target to the rendering destination device. /// public override Matrix TransformToDevice { get { VerifyAPIReadOnly(); Matrix m = WorldTransform; m.Invert(); return m; } } ////// Returns matrix that can be used to transform coordinates from /// the rendering destination device to this target. /// public override Matrix TransformFromDevice { get { VerifyAPIReadOnly(); return WorldTransform; } } #endregion Public Properties //---------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Private Fields ////// Dispose cleans up the state associated with HwndTarget. /// ////// Critical: Sets RootVisual to null /// PublicOK: This code has the same affect as removing elements in a window /// [SecurityCritical] public override void Dispose() { try { VerifyAccess(); if (!IsDisposed) { if (_hostVisual != null && _connected) { RootVisual = null; // // Unregister this CompositionTarget from the MediaSystem. // // we need to properly unregister in a disconnected state MediaContext.UnregisterICompositionTarget(Dispatcher, this); } } } finally { base.Dispose(); } } ////// This function gets called when VisualTarget is removed on the channel /// i.e. from ReleaseUCEResources. /// private void EndHosting() { Debug.Assert(_connected); _hostVisual.EndHosting(); _connected = false; } ////// This method is used to release all uce resources either on Shutdown or session disconnect /// internal override void ReleaseUCEResources(DUCE.Channel channel, DUCE.Channel outOfBandChannel) { if (!channel.IsLayeredWindowChannel) { EndHosting(); _contentRoot.ReleaseOnChannel(channel); if (_contentRoot.IsOnChannel(outOfBandChannel)) { _contentRoot.ReleaseOnChannel(outOfBandChannel); } base.ReleaseUCEResources(channel, outOfBandChannel); } } #endregion Public Methods #region Internal Properties ////// The out of band channel on our the MediaContext this /// resource was created. /// This is needed by HostVisual for handle duplication. /// internal DUCE.Channel OutOfBandChannel { get { return _outOfBandChannel; } } #endregion Internal Properties //--------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields DUCE.Channel _outOfBandChannel; private HostVisual _hostVisual; // Flag indicating whether VisualTarget-HostVisual connection exists. private bool _connected; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // // History: // 01/06/2005 : ABaioura - Created. // //----------------------------------------------------------------------------- using System.Windows.Media; using System.Windows.Media.Composition; using System.Security; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// /// public class VisualTarget : CompositionTarget { //--------------------------------------------------------------------- // // Constructors // //--------------------------------------------------------------------- #region Constructors ////// VisualTarget /// public VisualTarget(HostVisual hostVisual) { if (hostVisual == null) { throw new ArgumentNullException("hostVisual"); } _hostVisual = hostVisual; _connected = false; MediaContext.RegisterICompositionTarget(Dispatcher, this); } ////// This function gets called when VisualTarget is created on the channel /// i.e. from CreateUCEResources. /// private void BeginHosting() { Debug.Assert(!_connected); try { // // Initiate hosting by the specified host visual. // _hostVisual.BeginHosting(this); _connected = true; } catch { // // If exception has occurred after we have registered with // MediaContext, we need to unregister to properly release // allocated resources. // NOTE: We need to properly unregister in a disconnected state // MediaContext.UnregisterICompositionTarget(Dispatcher, this); throw; } } internal override void CreateUCEResources(DUCE.Channel channel, DUCE.Channel outOfBandChannel) { Debug.Assert(channel != null); Debug.Assert(outOfBandChannel != null); if (!channel.IsLayeredWindowChannel) { _outOfBandChannel = outOfBandChannel; // create visual target resources base.CreateUCEResources(channel, outOfBandChannel); // Update state to propagate flags as necessary StateChangedCallback( new object[] { HostStateFlags.None }); // // Addref content node on the channel. We need extra reference // on that node so that it does not get immediately released // when Dispose is called. Actual release of the node needs // to be synchronized with node disconnect by the host. // bool resourceCreated = _contentRoot.CreateOrAddRefOnChannel(outOfBandChannel, s_contentRootType); Debug.Assert(!resourceCreated); _contentRoot.CreateOrAddRefOnChannel(channel, s_contentRootType); BeginHosting(); } } #endregion Constructors //---------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties ////// Returns matrix that can be used to transform coordinates from this /// target to the rendering destination device. /// public override Matrix TransformToDevice { get { VerifyAPIReadOnly(); Matrix m = WorldTransform; m.Invert(); return m; } } ////// Returns matrix that can be used to transform coordinates from /// the rendering destination device to this target. /// public override Matrix TransformFromDevice { get { VerifyAPIReadOnly(); return WorldTransform; } } #endregion Public Properties //---------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Private Fields ////// Dispose cleans up the state associated with HwndTarget. /// ////// Critical: Sets RootVisual to null /// PublicOK: This code has the same affect as removing elements in a window /// [SecurityCritical] public override void Dispose() { try { VerifyAccess(); if (!IsDisposed) { if (_hostVisual != null && _connected) { RootVisual = null; // // Unregister this CompositionTarget from the MediaSystem. // // we need to properly unregister in a disconnected state MediaContext.UnregisterICompositionTarget(Dispatcher, this); } } } finally { base.Dispose(); } } ////// This function gets called when VisualTarget is removed on the channel /// i.e. from ReleaseUCEResources. /// private void EndHosting() { Debug.Assert(_connected); _hostVisual.EndHosting(); _connected = false; } ////// This method is used to release all uce resources either on Shutdown or session disconnect /// internal override void ReleaseUCEResources(DUCE.Channel channel, DUCE.Channel outOfBandChannel) { if (!channel.IsLayeredWindowChannel) { EndHosting(); _contentRoot.ReleaseOnChannel(channel); if (_contentRoot.IsOnChannel(outOfBandChannel)) { _contentRoot.ReleaseOnChannel(outOfBandChannel); } base.ReleaseUCEResources(channel, outOfBandChannel); } } #endregion Public Methods #region Internal Properties ////// The out of band channel on our the MediaContext this /// resource was created. /// This is needed by HostVisual for handle duplication. /// internal DUCE.Channel OutOfBandChannel { get { return _outOfBandChannel; } } #endregion Internal Properties //--------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields DUCE.Channel _outOfBandChannel; private HostVisual _hostVisual; // Flag indicating whether VisualTarget-HostVisual connection exists. private bool _connected; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
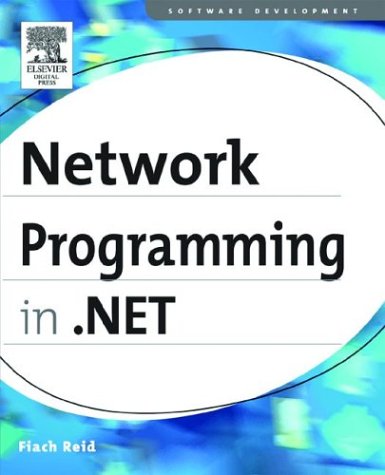
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlInputReset.cs
- _LocalDataStoreMgr.cs
- Int16Animation.cs
- HtmlTextArea.cs
- PropertyPushdownHelper.cs
- ClrPerspective.cs
- UIElementCollection.cs
- AnimatedTypeHelpers.cs
- AutomationProperty.cs
- RenamedEventArgs.cs
- SafeWaitHandle.cs
- MenuBase.cs
- Int32KeyFrameCollection.cs
- DataSourceControlBuilder.cs
- DrawingBrush.cs
- XmlSchemaSequence.cs
- SessionSwitchEventArgs.cs
- DataColumn.cs
- CryptoHelper.cs
- cookiecontainer.cs
- AuthorizationRule.cs
- PerfCounters.cs
- ValidationPropertyAttribute.cs
- EntityDataSourceMemberPath.cs
- EventRecordWrittenEventArgs.cs
- OletxVolatileEnlistment.cs
- SqlDataSourceSelectingEventArgs.cs
- ObjRef.cs
- SecurityPolicySection.cs
- SmiSettersStream.cs
- ClrPerspective.cs
- WebException.cs
- _NestedMultipleAsyncResult.cs
- ThicknessAnimation.cs
- PeerPresenceInfo.cs
- StylusCollection.cs
- ColumnPropertiesGroup.cs
- NamespaceMapping.cs
- PixelShader.cs
- QueryResult.cs
- DataGridItemEventArgs.cs
- DataGridViewRowHeaderCell.cs
- StandardToolWindows.cs
- RawTextInputReport.cs
- ParameterInfo.cs
- BitmapPalette.cs
- SqlInternalConnectionTds.cs
- OdbcParameter.cs
- ToolStripPanelDesigner.cs
- TypeTypeConverter.cs
- OutputScope.cs
- HttpWebRequestElement.cs
- StandardBindingElement.cs
- NullReferenceException.cs
- SmiContext.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- MemoryRecordBuffer.cs
- MetafileHeader.cs
- ScriptManagerProxy.cs
- TitleStyle.cs
- CounterCreationDataCollection.cs
- CodeCompileUnit.cs
- RightsManagementUser.cs
- XmlDeclaration.cs
- InkCanvasFeedbackAdorner.cs
- ListDictionaryInternal.cs
- AudioFileOut.cs
- StackOverflowException.cs
- MatrixUtil.cs
- Stream.cs
- LinkedDataMemberFieldEditor.cs
- ProjectedSlot.cs
- CompositeActivityMarkupSerializer.cs
- TextElementEnumerator.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- SecurityChannelFaultConverter.cs
- HttpWebRequestElement.cs
- ValidatorCompatibilityHelper.cs
- KeyValuePair.cs
- ArrayElementGridEntry.cs
- SafeRightsManagementPubHandle.cs
- ToolStripPanelRenderEventArgs.cs
- CompilationRelaxations.cs
- Avt.cs
- BuildManager.cs
- FlowDocumentScrollViewer.cs
- EncryptedData.cs
- ActivityPreviewDesigner.cs
- DataBoundControlAdapter.cs
- ExceptionValidationRule.cs
- ToolStripManager.cs
- EncodingTable.cs
- WebPartDescriptionCollection.cs
- UnderstoodHeaders.cs
- ComboBoxItem.cs
- ResourcePool.cs
- ObjectPropertyMapping.cs
- ExpressionVisitorHelpers.cs
- UmAlQuraCalendar.cs
- HasActivatableWorkflowEvent.cs