Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Xml / System / Xml / ListBase.cs / 1 / ListBase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.Schema; using System.Collections.Generic; using System.Diagnostics; using System.Text; namespace System.Xml { ////// Implementation of read-only IList and IList internal abstract class ListBaseinterfaces. Derived classes can inherit from /// this class and implement only two methods, Count and Item, rather than the entire IList interface. /// : IList , System.Collections.IList { //----------------------------------------------- // Abstract IList methods that must be // implemented by derived classes //----------------------------------------------- public abstract int Count { get; } public abstract T this[int index] { get; set; } //----------------------------------------------- // Implemented by base class -- accessible on // ListBase //----------------------------------------------- public virtual bool Contains(T value) { return IndexOf(value) != -1; } public virtual int IndexOf(T value) { for (int i = 0; i < Count; i++) if (value.Equals(this[i])) return i; return -1; } public virtual void CopyTo(T[] array, int index) { for (int i = 0; i < Count; i++) array[index + i] = this[i]; } public virtual IListEnumerator GetEnumerator() { return new IListEnumerator (this); } public virtual bool IsFixedSize { get { return true; } } public virtual bool IsReadOnly { get { return true; } } public virtual void Add(T value) { Insert(Count, value); } public virtual void Insert(int index, T value) { throw new NotSupportedException(); } public virtual bool Remove(T value) { int index = IndexOf(value); if (index >= 0) { RemoveAt(index); return true; } return false; } public virtual void RemoveAt(int index) { throw new NotSupportedException(); } public virtual void Clear() { for (int index = Count - 1; index >= 0; index--) RemoveAt(index); } //----------------------------------------------- // Implemented by base class -- only accessible // after casting to IList //----------------------------------------------- IEnumerator IEnumerable .GetEnumerator() { return new IListEnumerator (this); } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new IListEnumerator (this); } bool System.Collections.ICollection.IsSynchronized { get { return IsReadOnly; } } object System.Collections.ICollection.SyncRoot { get { return this; } } void System.Collections.ICollection.CopyTo(Array array, int index) { for (int i = 0; i < Count; i++) array.SetValue(this[i], index); } object System.Collections.IList.this[int index] { get { return this[index]; } set { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); this[index] = (T) value; } } int System.Collections.IList.Add(object value) { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); Add((T) value); return Count - 1; } void System.Collections.IList.Clear() { Clear(); } bool System.Collections.IList.Contains(object value) { if (!IsCompatibleType(value.GetType())) return false; return Contains((T) value); } int System.Collections.IList.IndexOf(object value) { if (!IsCompatibleType(value.GetType())) return -1; return IndexOf((T) value); } void System.Collections.IList.Insert(int index, object value) { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); Insert(index, (T) value); } void System.Collections.IList.Remove(object value) { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); Remove((T) value); } //----------------------------------------------- // Helper methods and classes //----------------------------------------------- private static bool IsCompatibleType(object value) { if((value == null && !typeof(T).IsValueType) || (value is T)) return true; return false; } } /// /// Implementation of IEnumerator internal struct IListEnumeratorand IEnumerator over an IList . /// : IEnumerator , System.Collections.IEnumerator { private IList sequence; private int index; private T current; /// /// Constructor. /// public IListEnumerator(IListsequence) { this.sequence = sequence; this.index = 0; this.current = default(T); } /// /// No-op. /// public void Dispose() { } ////// Return current item. Return default value if before first item or after last item in the list. /// public T Current { get { return this.current; } } ////// Return current item. Throw exception if before first item or after last item in the list. /// object System.Collections.IEnumerator.Current { get { if (this.index == 0) throw new InvalidOperationException(Res.GetString(Res.Sch_EnumNotStarted, string.Empty)); if (this.index > this.sequence.Count) throw new InvalidOperationException(Res.GetString(Res.Sch_EnumFinished, string.Empty)); return this.current; } } ////// Advance enumerator to next item in list. Return false if there are no more items. /// public bool MoveNext() { if (this.index < this.sequence.Count) { this.current = this.sequence[this.index]; this.index++; return true; } this.current = default(T); return false; } ////// Set the enumerator to its initial position, which is before the first item in the list. /// void System.Collections.IEnumerator.Reset() { this.index = 0; this.current = default(T); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.Schema; using System.Collections.Generic; using System.Diagnostics; using System.Text; namespace System.Xml { ////// Implementation of read-only IList and IList internal abstract class ListBaseinterfaces. Derived classes can inherit from /// this class and implement only two methods, Count and Item, rather than the entire IList interface. /// : IList , System.Collections.IList { //----------------------------------------------- // Abstract IList methods that must be // implemented by derived classes //----------------------------------------------- public abstract int Count { get; } public abstract T this[int index] { get; set; } //----------------------------------------------- // Implemented by base class -- accessible on // ListBase //----------------------------------------------- public virtual bool Contains(T value) { return IndexOf(value) != -1; } public virtual int IndexOf(T value) { for (int i = 0; i < Count; i++) if (value.Equals(this[i])) return i; return -1; } public virtual void CopyTo(T[] array, int index) { for (int i = 0; i < Count; i++) array[index + i] = this[i]; } public virtual IListEnumerator GetEnumerator() { return new IListEnumerator (this); } public virtual bool IsFixedSize { get { return true; } } public virtual bool IsReadOnly { get { return true; } } public virtual void Add(T value) { Insert(Count, value); } public virtual void Insert(int index, T value) { throw new NotSupportedException(); } public virtual bool Remove(T value) { int index = IndexOf(value); if (index >= 0) { RemoveAt(index); return true; } return false; } public virtual void RemoveAt(int index) { throw new NotSupportedException(); } public virtual void Clear() { for (int index = Count - 1; index >= 0; index--) RemoveAt(index); } //----------------------------------------------- // Implemented by base class -- only accessible // after casting to IList //----------------------------------------------- IEnumerator IEnumerable .GetEnumerator() { return new IListEnumerator (this); } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new IListEnumerator (this); } bool System.Collections.ICollection.IsSynchronized { get { return IsReadOnly; } } object System.Collections.ICollection.SyncRoot { get { return this; } } void System.Collections.ICollection.CopyTo(Array array, int index) { for (int i = 0; i < Count; i++) array.SetValue(this[i], index); } object System.Collections.IList.this[int index] { get { return this[index]; } set { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); this[index] = (T) value; } } int System.Collections.IList.Add(object value) { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); Add((T) value); return Count - 1; } void System.Collections.IList.Clear() { Clear(); } bool System.Collections.IList.Contains(object value) { if (!IsCompatibleType(value.GetType())) return false; return Contains((T) value); } int System.Collections.IList.IndexOf(object value) { if (!IsCompatibleType(value.GetType())) return -1; return IndexOf((T) value); } void System.Collections.IList.Insert(int index, object value) { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); Insert(index, (T) value); } void System.Collections.IList.Remove(object value) { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); Remove((T) value); } //----------------------------------------------- // Helper methods and classes //----------------------------------------------- private static bool IsCompatibleType(object value) { if((value == null && !typeof(T).IsValueType) || (value is T)) return true; return false; } } /// /// Implementation of IEnumerator internal struct IListEnumeratorand IEnumerator over an IList . /// : IEnumerator , System.Collections.IEnumerator { private IList sequence; private int index; private T current; /// /// Constructor. /// public IListEnumerator(IListsequence) { this.sequence = sequence; this.index = 0; this.current = default(T); } /// /// No-op. /// public void Dispose() { } ////// Return current item. Return default value if before first item or after last item in the list. /// public T Current { get { return this.current; } } ////// Return current item. Throw exception if before first item or after last item in the list. /// object System.Collections.IEnumerator.Current { get { if (this.index == 0) throw new InvalidOperationException(Res.GetString(Res.Sch_EnumNotStarted, string.Empty)); if (this.index > this.sequence.Count) throw new InvalidOperationException(Res.GetString(Res.Sch_EnumFinished, string.Empty)); return this.current; } } ////// Advance enumerator to next item in list. Return false if there are no more items. /// public bool MoveNext() { if (this.index < this.sequence.Count) { this.current = this.sequence[this.index]; this.index++; return true; } this.current = default(T); return false; } ////// Set the enumerator to its initial position, which is before the first item in the list. /// void System.Collections.IEnumerator.Reset() { this.index = 0; this.current = default(T); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
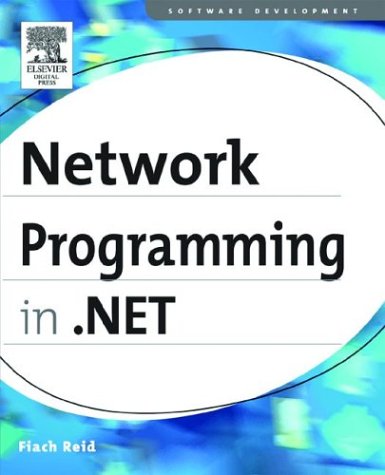
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextTreeTextBlock.cs
- GacUtil.cs
- TemplateEditingFrame.cs
- EdmToObjectNamespaceMap.cs
- HttpStreamMessageEncoderFactory.cs
- HiddenFieldPageStatePersister.cs
- Ipv6Element.cs
- SchemaElement.cs
- Memoizer.cs
- Propagator.Evaluator.cs
- SQLBytes.cs
- RecommendedAsConfigurableAttribute.cs
- OutOfProcStateClientManager.cs
- PerformanceCounterNameAttribute.cs
- HttpMethodAttribute.cs
- FileDialogPermission.cs
- IIS7WorkerRequest.cs
- CompensatableTransactionScopeActivity.cs
- SecurityPolicySection.cs
- TextEditorTyping.cs
- XmlChoiceIdentifierAttribute.cs
- XsltCompileContext.cs
- GetLedgerEntryForRecipientRequest.cs
- SingleQueryOperator.cs
- AutomationPeer.cs
- CultureInfo.cs
- DisableDpiAwarenessAttribute.cs
- UnsafeNativeMethods.cs
- WindowsEditBox.cs
- StringWriter.cs
- InheritanceUI.cs
- ValidateNames.cs
- SystemTcpStatistics.cs
- XmlToDatasetMap.cs
- InvocationExpression.cs
- WindowsSlider.cs
- QilList.cs
- HttpModulesSection.cs
- InvalidOperationException.cs
- Config.cs
- DataViewSetting.cs
- Compiler.cs
- SourceFilter.cs
- DynamicActivityTypeDescriptor.cs
- DLinqColumnProvider.cs
- Properties.cs
- DatagridviewDisplayedBandsData.cs
- File.cs
- Internal.cs
- StylusEditingBehavior.cs
- TextDecorationUnitValidation.cs
- DataSpaceManager.cs
- IntSecurity.cs
- ParentUndoUnit.cs
- UnmanagedMemoryStream.cs
- TypeGeneratedEventArgs.cs
- NamespaceQuery.cs
- StringCollection.cs
- WindowsListViewItemStartMenu.cs
- HostSecurityManager.cs
- ObjectHelper.cs
- OneOfConst.cs
- SignedPkcs7.cs
- CalendarDateRange.cs
- AnnotationComponentChooser.cs
- ToolStripSplitButton.cs
- TileBrush.cs
- PolygonHotSpot.cs
- OracleCommand.cs
- XmlSequenceWriter.cs
- XamlTreeBuilderBamlRecordWriter.cs
- RawUIStateInputReport.cs
- DoubleCollectionValueSerializer.cs
- EventListener.cs
- ArrowControl.xaml.cs
- SpeakProgressEventArgs.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- Hex.cs
- FeatureSupport.cs
- DbConnectionPoolGroupProviderInfo.cs
- NoPersistHandle.cs
- WebPartEditorCancelVerb.cs
- LexicalChunk.cs
- SafeCryptoKeyHandle.cs
- HostingPreferredMapPath.cs
- WindowsEditBoxRange.cs
- AlphabetConverter.cs
- HttpModuleActionCollection.cs
- FontNamesConverter.cs
- ComponentEvent.cs
- TableLayoutPanel.cs
- XmlUtil.cs
- ExpressionBuilder.cs
- ProfileProvider.cs
- InternalConfigSettingsFactory.cs
- ObfuscationAttribute.cs
- SqlClientFactory.cs
- CheckedListBox.cs
- GeometryModel3D.cs