Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / Collections / Specialized / StringCollection.cs / 1 / StringCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Collections.Specialized { using System.Diagnostics; using System.Collections; ////// [ Serializable, ] public class StringCollection : IList { private ArrayList data = new ArrayList(); ///Represents a collection of strings. ////// public string this[int index] { get { return ((string)data[index]); } set { data[index] = value; } } ///Represents the entry at the specified index of the ///. /// public int Count { get { return data.Count; } } bool IList.IsReadOnly { get { return false; } } bool IList.IsFixedSize { get { return false; } } ///Gets the number of strings in the /// ///. /// public int Add(string value) { return data.Add(value); } ///Adds a string with the specified value to the /// ///. /// public void AddRange(string[] value) { if (value == null) { throw new ArgumentNullException("value"); } data.AddRange(value); } ///Copies the elements of a string array to the end of the ///. /// public void Clear() { data.Clear(); } ///Removes all the strings from the /// ///. /// public bool Contains(string value) { return data.Contains(value); } ///Gets a value indicating whether the /// ///contains a string with the specified /// value. /// public void CopyTo(string[] array, int index) { data.CopyTo(array, index); } ///Copies the ///values to a one-dimensional instance at the /// specified index. /// public StringEnumerator GetEnumerator() { return new StringEnumerator(this); } ///Returns an enumerator that can iterate through /// the ///. /// public int IndexOf(string value) { return data.IndexOf(value); } ///Returns the index of the first occurrence of a string in /// the ///. /// public void Insert(int index, string value) { data.Insert(index, value); } ///Inserts a string into the ///at the specified /// index. /// public bool IsReadOnly { get { return false; } } ///Gets a value indicating whether the ///is read-only. /// public bool IsSynchronized { get { return false; } } ///Gets a value indicating whether access to the /// ////// is synchronized (thread-safe). /// public void Remove(string value) { data.Remove(value); } ///Removes a specific string from the /// ///. /// public void RemoveAt(int index) { data.RemoveAt(index); } ///Removes the string at the specified index of the ///. /// public object SyncRoot { get { return data.SyncRoot; } } object IList.this[int index] { get { return this[index]; } set { this[index] = (string)value; } } int IList.Add(object value) { return Add((string)value); } bool IList.Contains(object value) { return Contains((string) value); } int IList.IndexOf(object value) { return IndexOf((string)value); } void IList.Insert(int index, object value) { Insert(index, (string)value); } void IList.Remove(object value) { Remove((string)value); } void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } IEnumerator IEnumerable.GetEnumerator() { return data.GetEnumerator(); } } ///Gets an object that can be used to synchronize access to the ///. /// public class StringEnumerator { private System.Collections.IEnumerator baseEnumerator; private System.Collections.IEnumerable temp; internal StringEnumerator(StringCollection mappings) { this.temp = (IEnumerable)(mappings); this.baseEnumerator = temp.GetEnumerator(); } ///[To be supplied.] ////// public string Current { get { return (string)(baseEnumerator.Current); } } ///[To be supplied.] ////// public bool MoveNext() { return baseEnumerator.MoveNext(); } ///[To be supplied.] ////// public void Reset() { baseEnumerator.Reset(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Collections.Specialized { using System.Diagnostics; using System.Collections; ////// [ Serializable, ] public class StringCollection : IList { private ArrayList data = new ArrayList(); ///Represents a collection of strings. ////// public string this[int index] { get { return ((string)data[index]); } set { data[index] = value; } } ///Represents the entry at the specified index of the ///. /// public int Count { get { return data.Count; } } bool IList.IsReadOnly { get { return false; } } bool IList.IsFixedSize { get { return false; } } ///Gets the number of strings in the /// ///. /// public int Add(string value) { return data.Add(value); } ///Adds a string with the specified value to the /// ///. /// public void AddRange(string[] value) { if (value == null) { throw new ArgumentNullException("value"); } data.AddRange(value); } ///Copies the elements of a string array to the end of the ///. /// public void Clear() { data.Clear(); } ///Removes all the strings from the /// ///. /// public bool Contains(string value) { return data.Contains(value); } ///Gets a value indicating whether the /// ///contains a string with the specified /// value. /// public void CopyTo(string[] array, int index) { data.CopyTo(array, index); } ///Copies the ///values to a one-dimensional instance at the /// specified index. /// public StringEnumerator GetEnumerator() { return new StringEnumerator(this); } ///Returns an enumerator that can iterate through /// the ///. /// public int IndexOf(string value) { return data.IndexOf(value); } ///Returns the index of the first occurrence of a string in /// the ///. /// public void Insert(int index, string value) { data.Insert(index, value); } ///Inserts a string into the ///at the specified /// index. /// public bool IsReadOnly { get { return false; } } ///Gets a value indicating whether the ///is read-only. /// public bool IsSynchronized { get { return false; } } ///Gets a value indicating whether access to the /// ////// is synchronized (thread-safe). /// public void Remove(string value) { data.Remove(value); } ///Removes a specific string from the /// ///. /// public void RemoveAt(int index) { data.RemoveAt(index); } ///Removes the string at the specified index of the ///. /// public object SyncRoot { get { return data.SyncRoot; } } object IList.this[int index] { get { return this[index]; } set { this[index] = (string)value; } } int IList.Add(object value) { return Add((string)value); } bool IList.Contains(object value) { return Contains((string) value); } int IList.IndexOf(object value) { return IndexOf((string)value); } void IList.Insert(int index, object value) { Insert(index, (string)value); } void IList.Remove(object value) { Remove((string)value); } void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } IEnumerator IEnumerable.GetEnumerator() { return data.GetEnumerator(); } } ///Gets an object that can be used to synchronize access to the ///. /// public class StringEnumerator { private System.Collections.IEnumerator baseEnumerator; private System.Collections.IEnumerable temp; internal StringEnumerator(StringCollection mappings) { this.temp = (IEnumerable)(mappings); this.baseEnumerator = temp.GetEnumerator(); } ///[To be supplied.] ////// public string Current { get { return (string)(baseEnumerator.Current); } } ///[To be supplied.] ////// public bool MoveNext() { return baseEnumerator.MoveNext(); } ///[To be supplied.] ////// public void Reset() { baseEnumerator.Reset(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
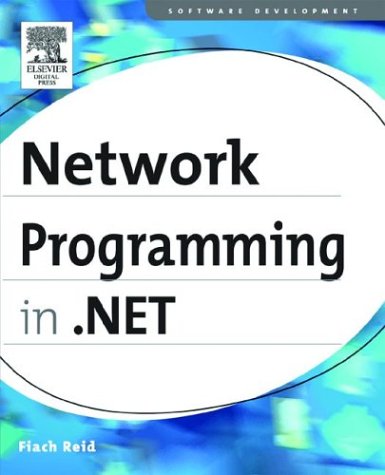
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityClientCacheEntry.cs
- OdbcTransaction.cs
- DefaultHttpHandler.cs
- ToolStripComboBox.cs
- InputDevice.cs
- BatchParser.cs
- ContextDataSourceView.cs
- WpfGeneratedKnownTypes.cs
- UriWriter.cs
- XmlMembersMapping.cs
- DataDocumentXPathNavigator.cs
- EasingQuaternionKeyFrame.cs
- PageEventArgs.cs
- QilParameter.cs
- ZeroOpNode.cs
- XmlConverter.cs
- DocumentPageHost.cs
- Delegate.cs
- ScriptResourceInfo.cs
- BamlWriter.cs
- DocumentReference.cs
- TypeDescriptor.cs
- MembershipUser.cs
- Hashtable.cs
- COSERVERINFO.cs
- DynamicEntity.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- NameValueConfigurationElement.cs
- StateChangeEvent.cs
- SafeThreadHandle.cs
- BamlResourceContent.cs
- GlobalAllocSafeHandle.cs
- KnownBoxes.cs
- EntityDataSourceDesignerHelper.cs
- DropShadowBitmapEffect.cs
- DLinqTableProvider.cs
- ContentType.cs
- ParamArrayAttribute.cs
- listitem.cs
- XMLSyntaxException.cs
- IgnoreFileBuildProvider.cs
- VirtualizedCellInfoCollection.cs
- DataRecordInfo.cs
- ToolStripPanel.cs
- ImagingCache.cs
- ExpressionBuilder.cs
- SymLanguageType.cs
- TcpAppDomainProtocolHandler.cs
- MailSettingsSection.cs
- FrameSecurityDescriptor.cs
- ProviderConnectionPoint.cs
- DetailsViewDeleteEventArgs.cs
- MatrixConverter.cs
- Vector3DCollectionValueSerializer.cs
- XPathNode.cs
- XmlSerializerAssemblyAttribute.cs
- CompiledQuery.cs
- BindingSource.cs
- NullReferenceException.cs
- SimplePropertyEntry.cs
- CustomPopupPlacement.cs
- UInt16.cs
- FrameworkElementFactoryMarkupObject.cs
- Math.cs
- SettingsAttributes.cs
- FixedPageAutomationPeer.cs
- LocationSectionRecord.cs
- StorageComplexTypeMapping.cs
- ConstructorNeedsTagAttribute.cs
- DbMetaDataCollectionNames.cs
- OciEnlistContext.cs
- XmlSchemaSimpleTypeUnion.cs
- KeyPressEvent.cs
- GridViewSelectEventArgs.cs
- TraceContext.cs
- ColumnCollection.cs
- NativeRecognizer.cs
- QuerySettings.cs
- CodeArrayIndexerExpression.cs
- ScrollBarRenderer.cs
- Int32Storage.cs
- SafeRegistryHandle.cs
- SqlFormatter.cs
- IdSpace.cs
- BindingList.cs
- XPathDocumentIterator.cs
- ThreadStateException.cs
- WpfWebRequestHelper.cs
- PerspectiveCamera.cs
- RtfFormatStack.cs
- SchemaImporter.cs
- PassportAuthentication.cs
- DynamicDiscoSearcher.cs
- ContainerControlDesigner.cs
- CodeAccessSecurityEngine.cs
- _NestedMultipleAsyncResult.cs
- HttpCacheVaryByContentEncodings.cs
- TopClause.cs
- EventManager.cs
- DataBindingHandlerAttribute.cs