Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / QIL / QilList.cs / 1305376 / QilList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; namespace System.Xml.Xsl.Qil { ////// View over a Qil operator having N children. /// ////// Don't construct QIL nodes directly; instead, use the internal class QilList : QilNode { private int count; private QilNode[] members; //----------------------------------------------- // Constructor //----------------------------------------------- ///QilFactory . ////// Construct a new (empty) QilList /// public QilList(QilNodeType nodeType) : base(nodeType) { this.members = new QilNode[4]; this.xmlType = null; } //----------------------------------------------- // QilNode methods //----------------------------------------------- ////// Lazily create the XmlQueryType. /// public override XmlQueryType XmlType { get { if (this.xmlType == null) { XmlQueryType xt = XmlQueryTypeFactory.Empty; if (this.count > 0) { if (this.nodeType == QilNodeType.Sequence) { for (int i = 0; i < this.count; i++) xt = XmlQueryTypeFactory.Sequence(xt, this.members[i].XmlType); Debug.Assert(!xt.IsDod, "Sequences do not preserve DocOrderDistinct"); } else if (this.nodeType == QilNodeType.BranchList) { xt = this.members[0].XmlType; for (int i = 1; i < this.count; i++) xt = XmlQueryTypeFactory.Choice(xt, this.members[i].XmlType); } } this.xmlType = xt; } return this.xmlType; } } ////// Override in order to clone the "members" array. /// public override QilNode ShallowClone(QilFactory f) { QilList n = (QilList) MemberwiseClone(); n.members = (QilNode[]) this.members.Clone(); f.TraceNode(n); return n; } //----------------------------------------------- // IListmethods -- override //----------------------------------------------- public override int Count { get { return this.count; } } public override QilNode this[int index] { get { if (index >= 0 && index < this.count) return this.members[index]; throw new IndexOutOfRangeException(); } set { if (index >= 0 && index < this.count) this.members[index] = value; else throw new IndexOutOfRangeException(); // Invalidate XmlType this.xmlType = null; } } public override void Insert(int index, QilNode node) { if (index < 0 || index > this.count) throw new IndexOutOfRangeException(); if (this.count == this.members.Length) { QilNode[] membersNew = new QilNode[this.count * 2]; Array.Copy(this.members, membersNew, this.count); this.members = membersNew; } if (index < this.count) Array.Copy(this.members, index, this.members, index + 1, this.count - index); this.count++; this.members[index] = node; // Invalidate XmlType this.xmlType = null; } public override void RemoveAt(int index) { if (index < 0 || index >= this.count) throw new IndexOutOfRangeException(); this.count--; if (index < this.count) Array.Copy(this.members, index + 1, this.members, index, this.count - index); this.members[this.count] = null; // Invalidate XmlType this.xmlType = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; namespace System.Xml.Xsl.Qil { ////// View over a Qil operator having N children. /// ////// Don't construct QIL nodes directly; instead, use the internal class QilList : QilNode { private int count; private QilNode[] members; //----------------------------------------------- // Constructor //----------------------------------------------- ///QilFactory . ////// Construct a new (empty) QilList /// public QilList(QilNodeType nodeType) : base(nodeType) { this.members = new QilNode[4]; this.xmlType = null; } //----------------------------------------------- // QilNode methods //----------------------------------------------- ////// Lazily create the XmlQueryType. /// public override XmlQueryType XmlType { get { if (this.xmlType == null) { XmlQueryType xt = XmlQueryTypeFactory.Empty; if (this.count > 0) { if (this.nodeType == QilNodeType.Sequence) { for (int i = 0; i < this.count; i++) xt = XmlQueryTypeFactory.Sequence(xt, this.members[i].XmlType); Debug.Assert(!xt.IsDod, "Sequences do not preserve DocOrderDistinct"); } else if (this.nodeType == QilNodeType.BranchList) { xt = this.members[0].XmlType; for (int i = 1; i < this.count; i++) xt = XmlQueryTypeFactory.Choice(xt, this.members[i].XmlType); } } this.xmlType = xt; } return this.xmlType; } } ////// Override in order to clone the "members" array. /// public override QilNode ShallowClone(QilFactory f) { QilList n = (QilList) MemberwiseClone(); n.members = (QilNode[]) this.members.Clone(); f.TraceNode(n); return n; } //----------------------------------------------- // IListmethods -- override //----------------------------------------------- public override int Count { get { return this.count; } } public override QilNode this[int index] { get { if (index >= 0 && index < this.count) return this.members[index]; throw new IndexOutOfRangeException(); } set { if (index >= 0 && index < this.count) this.members[index] = value; else throw new IndexOutOfRangeException(); // Invalidate XmlType this.xmlType = null; } } public override void Insert(int index, QilNode node) { if (index < 0 || index > this.count) throw new IndexOutOfRangeException(); if (this.count == this.members.Length) { QilNode[] membersNew = new QilNode[this.count * 2]; Array.Copy(this.members, membersNew, this.count); this.members = membersNew; } if (index < this.count) Array.Copy(this.members, index, this.members, index + 1, this.count - index); this.count++; this.members[index] = node; // Invalidate XmlType this.xmlType = null; } public override void RemoveAt(int index) { if (index < 0 || index >= this.count) throw new IndexOutOfRangeException(); this.count--; if (index < this.count) Array.Copy(this.members, index + 1, this.members, index, this.count - index); this.members[this.count] = null; // Invalidate XmlType this.xmlType = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
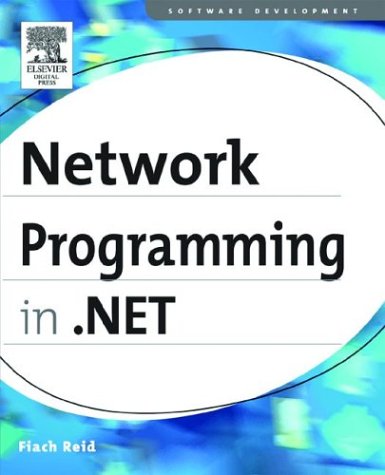
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PersistenceParticipant.cs
- HttpCacheParams.cs
- TrackingProfileManager.cs
- ClientData.cs
- ReadOnlyHierarchicalDataSource.cs
- Calendar.cs
- CustomSignedXml.cs
- PathTooLongException.cs
- DrawingGroupDrawingContext.cs
- ExpressionBindingCollection.cs
- CompilationUtil.cs
- EventProviderTraceListener.cs
- ToolStripRenderer.cs
- TreeWalker.cs
- XmlNamedNodeMap.cs
- DateBoldEvent.cs
- TableLayoutSettings.cs
- Automation.cs
- XmlRawWriterWrapper.cs
- MetadataProperty.cs
- ServicesUtilities.cs
- CFStream.cs
- MailMessageEventArgs.cs
- TreeView.cs
- RadioButtonBaseAdapter.cs
- DoWhileDesigner.xaml.cs
- NameValueFileSectionHandler.cs
- ContextStaticAttribute.cs
- HtmlTableRowCollection.cs
- ComNativeDescriptor.cs
- DATA_BLOB.cs
- TableRowCollection.cs
- Publisher.cs
- DeviceSpecific.cs
- StateManager.cs
- InfiniteTimeSpanConverter.cs
- ListViewSortEventArgs.cs
- DbProviderConfigurationHandler.cs
- UriExt.cs
- GlyphCache.cs
- ContractComponent.cs
- ToolStripCustomTypeDescriptor.cs
- Mutex.cs
- MD5HashHelper.cs
- DataGridViewUtilities.cs
- SafeReversePInvokeHandle.cs
- LinqDataSourceStatusEventArgs.cs
- FilterEventArgs.cs
- CFStream.cs
- FixedPosition.cs
- DataGridViewRowsAddedEventArgs.cs
- AnnotationMap.cs
- PowerEase.cs
- PersistChildrenAttribute.cs
- DataGridViewComboBoxCell.cs
- XmlSchemaAppInfo.cs
- LexicalChunk.cs
- StorageInfo.cs
- NotSupportedException.cs
- Effect.cs
- CellTreeNode.cs
- XmlExtensionFunction.cs
- XmlnsDefinitionAttribute.cs
- VisualBasicHelper.cs
- XmlIlTypeHelper.cs
- ViewDesigner.cs
- AuthenticationService.cs
- WindowsAltTab.cs
- HorizontalAlignConverter.cs
- ProjectionCamera.cs
- XsltSettings.cs
- CustomSignedXml.cs
- CompiledRegexRunnerFactory.cs
- StateMachineExecutionState.cs
- FlowDecision.cs
- SerialPinChanges.cs
- BuildProviderCollection.cs
- FtpCachePolicyElement.cs
- DocumentOrderComparer.cs
- CalendarDay.cs
- SynchronizationContext.cs
- AudioBase.cs
- AudioStateChangedEventArgs.cs
- Pkcs7Signer.cs
- WebPartConnection.cs
- OdbcTransaction.cs
- X509CertificateTrustedIssuerElement.cs
- ExpandableObjectConverter.cs
- NonBatchDirectoryCompiler.cs
- DependencyObjectProvider.cs
- RadialGradientBrush.cs
- SystemParameters.cs
- WebConfigurationFileMap.cs
- DomainUpDown.cs
- CodeTypeDeclarationCollection.cs
- WebColorConverter.cs
- LZCodec.cs
- ResourceExpressionBuilder.cs
- IdentifierCollection.cs
- OverlappedContext.cs