Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / Ink / InkSerializedFormat / LZCodec.cs / 1 / LZCodec.cs
using MS.Utility; using System; using System.Runtime.InteropServices; using System.Security; using System.Globalization; using System.Windows; using System.Windows.Input; using System.Windows.Ink; using MS.Internal.Ink.InkSerializedFormat; using MS.Internal.Ink; using System.Collections.Generic; using System.Diagnostics; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// LZCodec /// internal class LZCodec { ////// LZCodec /// internal LZCodec() { } ////// Uncompress /// /// /// ///internal byte[] Uncompress(byte[] input, int inputIndex) { //first things first Debug.Assert(input != null); Debug.Assert(input.Length > 1); Debug.Assert(inputIndex < input.Length); Debug.Assert(inputIndex >= 0); List output = new List (); BitStreamWriter writer = new BitStreamWriter(output); BitStreamReader reader = new BitStreamReader(input, inputIndex); //decode int index = 0, countBytes = 0, start = 0; byte byte1 = 0, byte2 = 0; _maxMatchLength = FirstMaxMatchLength; // initialize the ring buffer for (index = 0; index < RingBufferLength - _maxMatchLength; index++) { _ringBuffer[index] = 0; } //initialize decoding globals _flags = 0; _currentRingBufferPosition = RingBufferLength - _maxMatchLength; while (!reader.EndOfStream) { byte1 = reader.ReadByte(Native.BitsPerByte); // High order byte counts the number of bits used in the low order // byte. if (((_flags >>= 1) & 0x100) == 0) { // Set bit mask describing the next 8 bytes. _flags = (((int)byte1) | 0xff00); byte1 = reader.ReadByte(Native.BitsPerByte); } if ((_flags & 1) != 0) { // Just store the literal byte in the buffer. writer.Write(byte1, Native.BitsPerByte); _ringBuffer[_currentRingBufferPosition++] = byte1; _currentRingBufferPosition &= RingBufferLength - 1; } else { // Extract the offset and count to copy from the ring buffer. byte2 = reader.ReadByte(Native.BitsPerByte); countBytes = (int)byte2; start = (countBytes & 0xf0) << 4 | (int)byte1; countBytes = (countBytes & 0x0f) + MaxLiteralLength; for (index = 0; index <= countBytes; index++) { byte1 = _ringBuffer[(start + index) & (RingBufferLength - 1)]; writer.Write(byte1, Native.BitsPerByte); _ringBuffer[_currentRingBufferPosition++] = byte1; _currentRingBufferPosition &= RingBufferLength - 1; } } } return output.ToArray(); } /// /// Privates /// private byte[] _ringBuffer = new byte[RingBufferLength]; private int _maxMatchLength = 0; private int _flags = 0; private int _currentRingBufferPosition = 0; ////// Statics / constants /// private static readonly int FirstMaxMatchLength = 0x10; private static readonly int RingBufferLength = 4069; private static readonly int MaxLiteralLength = 2; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using MS.Utility; using System; using System.Runtime.InteropServices; using System.Security; using System.Globalization; using System.Windows; using System.Windows.Input; using System.Windows.Ink; using MS.Internal.Ink.InkSerializedFormat; using MS.Internal.Ink; using System.Collections.Generic; using System.Diagnostics; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// LZCodec /// internal class LZCodec { ////// LZCodec /// internal LZCodec() { } ////// Uncompress /// /// /// ///internal byte[] Uncompress(byte[] input, int inputIndex) { //first things first Debug.Assert(input != null); Debug.Assert(input.Length > 1); Debug.Assert(inputIndex < input.Length); Debug.Assert(inputIndex >= 0); List output = new List (); BitStreamWriter writer = new BitStreamWriter(output); BitStreamReader reader = new BitStreamReader(input, inputIndex); //decode int index = 0, countBytes = 0, start = 0; byte byte1 = 0, byte2 = 0; _maxMatchLength = FirstMaxMatchLength; // initialize the ring buffer for (index = 0; index < RingBufferLength - _maxMatchLength; index++) { _ringBuffer[index] = 0; } //initialize decoding globals _flags = 0; _currentRingBufferPosition = RingBufferLength - _maxMatchLength; while (!reader.EndOfStream) { byte1 = reader.ReadByte(Native.BitsPerByte); // High order byte counts the number of bits used in the low order // byte. if (((_flags >>= 1) & 0x100) == 0) { // Set bit mask describing the next 8 bytes. _flags = (((int)byte1) | 0xff00); byte1 = reader.ReadByte(Native.BitsPerByte); } if ((_flags & 1) != 0) { // Just store the literal byte in the buffer. writer.Write(byte1, Native.BitsPerByte); _ringBuffer[_currentRingBufferPosition++] = byte1; _currentRingBufferPosition &= RingBufferLength - 1; } else { // Extract the offset and count to copy from the ring buffer. byte2 = reader.ReadByte(Native.BitsPerByte); countBytes = (int)byte2; start = (countBytes & 0xf0) << 4 | (int)byte1; countBytes = (countBytes & 0x0f) + MaxLiteralLength; for (index = 0; index <= countBytes; index++) { byte1 = _ringBuffer[(start + index) & (RingBufferLength - 1)]; writer.Write(byte1, Native.BitsPerByte); _ringBuffer[_currentRingBufferPosition++] = byte1; _currentRingBufferPosition &= RingBufferLength - 1; } } } return output.ToArray(); } /// /// Privates /// private byte[] _ringBuffer = new byte[RingBufferLength]; private int _maxMatchLength = 0; private int _flags = 0; private int _currentRingBufferPosition = 0; ////// Statics / constants /// private static readonly int FirstMaxMatchLength = 0x10; private static readonly int RingBufferLength = 4069; private static readonly int MaxLiteralLength = 2; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
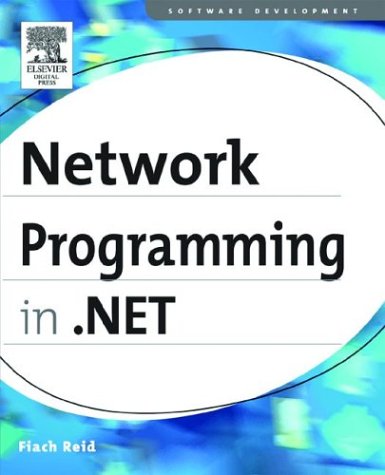
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateControlBuildProvider.cs
- QilFactory.cs
- Token.cs
- ProjectedSlot.cs
- GlyphRunDrawing.cs
- TreeWalker.cs
- CapabilitiesAssignment.cs
- PointF.cs
- DataKeyArray.cs
- ProbeMatchesApril2005.cs
- NullableBoolConverter.cs
- SystemException.cs
- RemoteWebConfigurationHostStream.cs
- StrongTypingException.cs
- SmtpNtlmAuthenticationModule.cs
- EventLogger.cs
- Console.cs
- LinkButton.cs
- JpegBitmapDecoder.cs
- AsyncMethodInvoker.cs
- Debug.cs
- EntityWithKeyStrategy.cs
- ConfigurationSectionGroupCollection.cs
- StorageEntityTypeMapping.cs
- PrintPreviewGraphics.cs
- DynamicRenderer.cs
- SingleObjectCollection.cs
- TypeLoadException.cs
- DbParameterCollectionHelper.cs
- XPathItem.cs
- XmlEventCache.cs
- TypeConvertions.cs
- CacheHelper.cs
- MethodBody.cs
- CompilerGlobalScopeAttribute.cs
- XmlUtil.cs
- CodeEventReferenceExpression.cs
- BlobPersonalizationState.cs
- SmtpFailedRecipientsException.cs
- DefaultSection.cs
- OleDbConnectionInternal.cs
- RoutedEventHandlerInfo.cs
- PeerNameRecordCollection.cs
- TreeIterator.cs
- FileIOPermission.cs
- Int32AnimationBase.cs
- GifBitmapEncoder.cs
- RuleInfoComparer.cs
- ServicePointManager.cs
- MetabaseServerConfig.cs
- DecodeHelper.cs
- ObjectPersistData.cs
- ECDiffieHellmanCng.cs
- Hashtable.cs
- DelegatedStream.cs
- FactoryMaker.cs
- ConnectionStringsExpressionEditor.cs
- Pen.cs
- BindingList.cs
- FormViewPagerRow.cs
- WindowsTooltip.cs
- DrawingVisual.cs
- GPStream.cs
- DbConnectionPoolGroup.cs
- StylusPointCollection.cs
- CompositeControlDesigner.cs
- DispatchChannelSink.cs
- NamespaceTable.cs
- DllNotFoundException.cs
- TemplateNameScope.cs
- ListViewCommandEventArgs.cs
- DataGridViewCheckBoxCell.cs
- OdbcTransaction.cs
- CommentEmitter.cs
- HttpAsyncResult.cs
- HeaderCollection.cs
- BinaryMethodMessage.cs
- HttpWriter.cs
- TraceContextRecord.cs
- RunClient.cs
- ButtonField.cs
- COAUTHIDENTITY.cs
- ProxyAttribute.cs
- SynchronousChannelMergeEnumerator.cs
- ImageFormatConverter.cs
- ZoneIdentityPermission.cs
- RestHandler.cs
- WebEventTraceProvider.cs
- PartialArray.cs
- ContentValidator.cs
- graph.cs
- AsyncResult.cs
- StateFinalizationActivity.cs
- TextViewElement.cs
- SqlCacheDependency.cs
- FieldAccessException.cs
- Button.cs
- DataGridCaption.cs
- ListBoxItemAutomationPeer.cs
- StrokeNodeData.cs