Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / PartialArray.cs / 1305600 / PartialArray.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: The PartialArray struct is used when the developer needs to pass a CLR array range to // a function that takes generic IList interface. For cases when the whole array needs to be passed, // CLR array already implements IList. // // // History: // 06/25/2004 : mleonov - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal { ////// The PartialArray struct is used when someone needs to pass a CLR array range to /// a function that takes generic IList interface. For cases when the whole array needs to be passed, /// CLR array already implements IList. /// internal struct PartialArray: IList { private T[] _array; private int _initialIndex; private int _count; public PartialArray(T[] array, int initialIndex, int count) { // make sure early that the caller didn't miscalculate index and count Debug.Assert(initialIndex >= 0 && initialIndex + count <= array.Length); _array = array; _initialIndex = initialIndex; _count = count; } /// /// Convenience helper for passing the whole array. /// /// public PartialArray(T[] array) : this(array, 0, array.Length) {} #region IListMembers public bool IsReadOnly { get { return false; } } public bool Contains(T item) { return IndexOf(item) >= 0; } public bool IsFixedSize { get { return true; } } public bool Remove(T item) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void RemoveAt(int index) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void Clear() { throw new NotSupportedException(); } public void Add(T item) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void Insert(int index, T item) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public T this[int index] { get { return _array[index + _initialIndex]; } set { _array[index + _initialIndex] = value; } } public int IndexOf(T item) { int index = Array.IndexOf (_array, item, _initialIndex, _count); if (index >= 0) { return index - _initialIndex; } else { return -1; } } #endregion #region ICollection Members public int Count { get { return _count; } } public void CopyTo(T[] array, int arrayIndex) { // parameter validations if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException( SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional), "array"); } if (arrayIndex < 0) { throw new ArgumentOutOfRangeException("arrayIndex"); } if (arrayIndex >= array.Length) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "arrayIndex", "array"), "arrayIndex"); } if ((array.Length - Count - arrayIndex) < 0) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); } // do the copying here for (int i = 0; i < Count; i++) { array[arrayIndex + i] = this[i]; } } #endregion #region IEnumerable Members IEnumerator IEnumerable .GetEnumerator() { for (int i = 0; i < Count; i++) { yield return this[i]; } } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
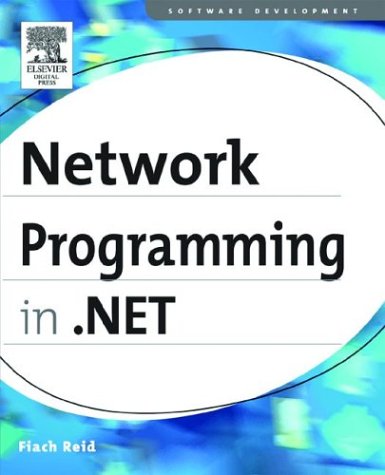
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegexCompilationInfo.cs
- LayoutInformation.cs
- XmlDigitalSignatureProcessor.cs
- ObjectTypeMapping.cs
- MailBnfHelper.cs
- ConfigurationSettings.cs
- GAC.cs
- CookieHandler.cs
- PathSegment.cs
- ArgIterator.cs
- HttpContextServiceHost.cs
- HebrewCalendar.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- SqlNode.cs
- GridViewUpdateEventArgs.cs
- TripleDES.cs
- LogConverter.cs
- ProcessInfo.cs
- LockCookie.cs
- versioninfo.cs
- WmlListAdapter.cs
- PropertyValueChangedEvent.cs
- CodeVariableDeclarationStatement.cs
- TypeConvertions.cs
- CurrentChangedEventManager.cs
- sortedlist.cs
- InfoCardService.cs
- ViewLoader.cs
- InitializeCorrelation.cs
- SettingsPropertyValue.cs
- AssociationSet.cs
- UIPermission.cs
- NoPersistScope.cs
- DbConnectionPoolGroup.cs
- TypeReference.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- ConfigurationCollectionAttribute.cs
- recordstatefactory.cs
- ToolStripButton.cs
- _TransmitFileOverlappedAsyncResult.cs
- CodeBlockBuilder.cs
- DataRecordObjectView.cs
- ContainerCodeDomSerializer.cs
- ConstantExpression.cs
- InlinedAggregationOperator.cs
- CodeGenerator.cs
- XsltLoader.cs
- WebPartConnection.cs
- AdornerHitTestResult.cs
- WindowsAuthenticationModule.cs
- PngBitmapEncoder.cs
- ProcessModuleDesigner.cs
- TagPrefixAttribute.cs
- ConstructorNeedsTagAttribute.cs
- AnimatedTypeHelpers.cs
- FormViewUpdateEventArgs.cs
- AlphabeticalEnumConverter.cs
- HttpModulesSection.cs
- WindowsIPAddress.cs
- SymLanguageVendor.cs
- OracleInternalConnection.cs
- StandardMenuStripVerb.cs
- WindowsFormsHostAutomationPeer.cs
- StaticFileHandler.cs
- WebResponse.cs
- OdbcInfoMessageEvent.cs
- NetMsmqSecurityMode.cs
- MailWriter.cs
- UserNameSecurityTokenAuthenticator.cs
- UrlAuthFailedErrorFormatter.cs
- SecureStringHasher.cs
- TransformedBitmap.cs
- Light.cs
- GenericTextProperties.cs
- SafeRightsManagementEnvironmentHandle.cs
- DoubleAnimation.cs
- TextEditorTables.cs
- Metadata.cs
- CodeGeneratorOptions.cs
- DebugHandleTracker.cs
- CacheDependency.cs
- ApplicationProxyInternal.cs
- PrintDialog.cs
- HostProtectionPermission.cs
- PageThemeCodeDomTreeGenerator.cs
- ToolStripLocationCancelEventArgs.cs
- Utils.cs
- StartUpEventArgs.cs
- TriggerBase.cs
- LocalValueEnumerator.cs
- DBSqlParserTableCollection.cs
- AtlasWeb.Designer.cs
- BufferedGraphics.cs
- _UriTypeConverter.cs
- DefaultShape.cs
- SecurityManager.cs
- EventDescriptor.cs
- SystemIcmpV6Statistics.cs
- PersistenceTypeAttribute.cs
- Popup.cs