Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / TextEditorTables.cs / 1305600 / TextEditorTables.cs
//---------------------------------------------------------------------------- // // File: TextEditorTables.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A Component of TextEditor supporting Table editing commands // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Globalization; using System.Threading; using System.ComponentModel; using System.Text; using System.Collections; // ArrayList using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows.Input; using System.Windows.Controls; // ScrollChangedEventArgs using System.Windows.Controls.Primitives; // CharacterCasing, TextBoxBase using System.Windows.Media; using System.Windows.Markup; using MS.Utility; using MS.Win32; using MS.Internal.Documents; using MS.Internal.Commands; // CommandHelpers ////// Text editing service for controls. /// internal static class TextEditorTables { //----------------------------------------------------- // // Class Internal Methods // //----------------------------------------------------- #region Class Internal Methods // Registers all text editing command handlers for a given control type internal static void _RegisterClassHandlers(Type controlType, bool registerEventListeners) { var onTableCommand = new ExecutedRoutedEventHandler(OnTableCommand); var onQueryStatusNYI = new CanExecuteRoutedEventHandler(OnQueryStatusNYI); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertTable , onTableCommand, onQueryStatusNYI, SRID.KeyInsertTable, SRID.KeyInsertTableDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertRows , onTableCommand, onQueryStatusNYI, SRID.KeyInsertRows, SRID.KeyInsertRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertColumns , onTableCommand, onQueryStatusNYI, SRID.KeyInsertColumns, SRID.KeyInsertColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteRows , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteRows, SRID.KeyDeleteRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteColumns , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteColumns, SRID.KeyDeleteColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.MergeCells , onTableCommand, onQueryStatusNYI, SRID.KeyMergeCells, SRID.KeyMergeCellsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.SplitCell , onTableCommand, onQueryStatusNYI, SRID.KeySplitCell, SRID.KeySplitCellDisplayString); } #endregion Class Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods private static void OnTableCommand(object target, ExecutedRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null || !This._IsEnabled || This.IsReadOnly || !This.AcceptsRichContent || !(This.Selection is TextSelection)) { return; } TextEditorTyping._FlushPendingInputItems(This); // Forget previously suggested horizontal position TextEditorSelection._ClearSuggestedX(This); // Execute the command if (args.Command == EditingCommands.InsertTable) { ((TextSelection)This.Selection).InsertTable(/*rowCount:*/4, /*columnCount:*/4); } else if (args.Command == EditingCommands.InsertRows) { ((TextSelection)This.Selection).InsertRows(+1); } else if (args.Command == EditingCommands.InsertColumns) { ((TextSelection)This.Selection).InsertColumns(+1); } else if (args.Command == EditingCommands.DeleteRows) { ((TextSelection)This.Selection).DeleteRows(); } else if (args.Command == EditingCommands.DeleteColumns) { ((TextSelection)This.Selection).DeleteColumns(); } else if (args.Command == EditingCommands.MergeCells) { ((TextSelection)This.Selection).MergeCells(); } else if (args.Command == EditingCommands.SplitCell) { ((TextSelection)This.Selection).SplitCell(1000, 1000); // Split all ways to possible maximum } } // ---------------------------------------------------------- // // Misceleneous Commands // // ---------------------------------------------------------- #region Misceleneous Commands ////// StartInputCorrection command QueryStatus handler /// private static void OnQueryStatusNYI(object target, CanExecuteRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null) { return; } args.CanExecute = true; } #endregion Misceleneous Commands #endregion Private methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: TextEditorTables.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A Component of TextEditor supporting Table editing commands // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Globalization; using System.Threading; using System.ComponentModel; using System.Text; using System.Collections; // ArrayList using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows.Input; using System.Windows.Controls; // ScrollChangedEventArgs using System.Windows.Controls.Primitives; // CharacterCasing, TextBoxBase using System.Windows.Media; using System.Windows.Markup; using MS.Utility; using MS.Win32; using MS.Internal.Documents; using MS.Internal.Commands; // CommandHelpers ////// Text editing service for controls. /// internal static class TextEditorTables { //----------------------------------------------------- // // Class Internal Methods // //----------------------------------------------------- #region Class Internal Methods // Registers all text editing command handlers for a given control type internal static void _RegisterClassHandlers(Type controlType, bool registerEventListeners) { var onTableCommand = new ExecutedRoutedEventHandler(OnTableCommand); var onQueryStatusNYI = new CanExecuteRoutedEventHandler(OnQueryStatusNYI); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertTable , onTableCommand, onQueryStatusNYI, SRID.KeyInsertTable, SRID.KeyInsertTableDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertRows , onTableCommand, onQueryStatusNYI, SRID.KeyInsertRows, SRID.KeyInsertRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertColumns , onTableCommand, onQueryStatusNYI, SRID.KeyInsertColumns, SRID.KeyInsertColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteRows , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteRows, SRID.KeyDeleteRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteColumns , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteColumns, SRID.KeyDeleteColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.MergeCells , onTableCommand, onQueryStatusNYI, SRID.KeyMergeCells, SRID.KeyMergeCellsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.SplitCell , onTableCommand, onQueryStatusNYI, SRID.KeySplitCell, SRID.KeySplitCellDisplayString); } #endregion Class Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods private static void OnTableCommand(object target, ExecutedRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null || !This._IsEnabled || This.IsReadOnly || !This.AcceptsRichContent || !(This.Selection is TextSelection)) { return; } TextEditorTyping._FlushPendingInputItems(This); // Forget previously suggested horizontal position TextEditorSelection._ClearSuggestedX(This); // Execute the command if (args.Command == EditingCommands.InsertTable) { ((TextSelection)This.Selection).InsertTable(/*rowCount:*/4, /*columnCount:*/4); } else if (args.Command == EditingCommands.InsertRows) { ((TextSelection)This.Selection).InsertRows(+1); } else if (args.Command == EditingCommands.InsertColumns) { ((TextSelection)This.Selection).InsertColumns(+1); } else if (args.Command == EditingCommands.DeleteRows) { ((TextSelection)This.Selection).DeleteRows(); } else if (args.Command == EditingCommands.DeleteColumns) { ((TextSelection)This.Selection).DeleteColumns(); } else if (args.Command == EditingCommands.MergeCells) { ((TextSelection)This.Selection).MergeCells(); } else if (args.Command == EditingCommands.SplitCell) { ((TextSelection)This.Selection).SplitCell(1000, 1000); // Split all ways to possible maximum } } // ---------------------------------------------------------- // // Misceleneous Commands // // ---------------------------------------------------------- #region Misceleneous Commands ////// StartInputCorrection command QueryStatus handler /// private static void OnQueryStatusNYI(object target, CanExecuteRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null) { return; } args.CanExecute = true; } #endregion Misceleneous Commands #endregion Private methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
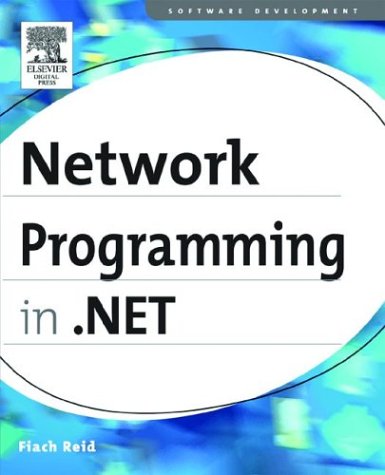
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CryptoApi.cs
- UseLicense.cs
- ConnectionPointCookie.cs
- ExtentKey.cs
- InputDevice.cs
- RSAPKCS1SignatureDeformatter.cs
- SqlCacheDependencySection.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- WebPartEditorCancelVerb.cs
- ImageKeyConverter.cs
- Evidence.cs
- HttpWebRequestElement.cs
- EventProperty.cs
- UnmanagedMemoryStreamWrapper.cs
- Int16Converter.cs
- ZipIOModeEnforcingStream.cs
- XmlIncludeAttribute.cs
- ArrangedElement.cs
- ExtendedProtectionPolicy.cs
- Int64Storage.cs
- ApplicationSecurityManager.cs
- XPathNavigatorException.cs
- MouseEventArgs.cs
- AttachInfo.cs
- TimeEnumHelper.cs
- AppSettingsReader.cs
- backend.cs
- VectorAnimationUsingKeyFrames.cs
- MinimizableAttributeTypeConverter.cs
- ConfigurationUtility.cs
- ValueOfAction.cs
- TrackingExtract.cs
- DateTimeConverter.cs
- StorageComplexPropertyMapping.cs
- ProfileParameter.cs
- WebDisplayNameAttribute.cs
- TickBar.cs
- Models.cs
- ProvidersHelper.cs
- ProbeDuplexCD1AsyncResult.cs
- MatrixAnimationUsingPath.cs
- Rect3DValueSerializer.cs
- ConfigurationException.cs
- DateBoldEvent.cs
- UInt64Storage.cs
- XmlAttributeOverrides.cs
- TripleDESCryptoServiceProvider.cs
- SystemWebSectionGroup.cs
- OdbcInfoMessageEvent.cs
- PropertyNames.cs
- EmptyStringExpandableObjectConverter.cs
- SortDescriptionCollection.cs
- StreamWithDictionary.cs
- WebPartConnectionsDisconnectVerb.cs
- AvtEvent.cs
- KnowledgeBase.cs
- NetworkAddressChange.cs
- Registry.cs
- Durable.cs
- TypeConverter.cs
- RIPEMD160Managed.cs
- Int32AnimationBase.cs
- VerificationAttribute.cs
- ImageEditor.cs
- XmlEncodedRawTextWriter.cs
- DBSchemaRow.cs
- DatagramAdapter.cs
- ConvertTextFrag.cs
- brushes.cs
- NativeMethodsCLR.cs
- ProgressBar.cs
- AutomationPattern.cs
- EmptyTextWriter.cs
- CancellableEnumerable.cs
- ListDataHelper.cs
- DataAdapter.cs
- PropertyBuilder.cs
- DeferredSelectedIndexReference.cs
- RuntimeResourceSet.cs
- SessionIDManager.cs
- ScopedKnownTypes.cs
- TextEditorMouse.cs
- BitmapEffectDrawing.cs
- WebBrowser.cs
- SortDescriptionCollection.cs
- AttributeQuery.cs
- RequestCacheEntry.cs
- ProtocolsConfigurationEntry.cs
- CookieHandler.cs
- UnSafeCharBuffer.cs
- DateTimeUtil.cs
- CommonDialog.cs
- RectangleHotSpot.cs
- Atom10FormatterFactory.cs
- BasicViewGenerator.cs
- SQLRoleProvider.cs
- DataGridColumnHeaderAutomationPeer.cs
- TemplateBindingExpression.cs
- DetailsViewDeleteEventArgs.cs
- ExpressionPrinter.cs