Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / security / system / security / authentication / ExtendedProtection / ExtendedProtectionPolicy.cs / 1305376 / ExtendedProtectionPolicy.cs
//------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.Net; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Security.Permissions; using System.Text; namespace System.Security.Authentication.ExtendedProtection { ////// This class contains the necessary settings for specifying how Extended Protection /// should behave. Use one of the Build* methods to create an instance of this type. /// [Serializable] [TypeConverter(typeof(ExtendedProtectionPolicyTypeConverter))] public class ExtendedProtectionPolicy : ISerializable { private const string policyEnforcementName = "policyEnforcement"; private const string protectionScenarioName = "protectionScenario"; private const string customServiceNamesName = "customServiceNames"; private const string customChannelBindingName = "customChannelBinding"; private ServiceNameCollection customServiceNames; private PolicyEnforcement policyEnforcement; private ProtectionScenario protectionScenario; private ChannelBinding customChannelBinding; public ExtendedProtectionPolicy(PolicyEnforcement policyEnforcement, ProtectionScenario protectionScenario, ServiceNameCollection customServiceNames) { if (policyEnforcement == PolicyEnforcement.Never) { throw new ArgumentException(SR.GetString(SR.security_ExtendedProtectionPolicy_UseDifferentConstructorForNever), "policyEnforcement"); } if (customServiceNames != null && customServiceNames.Count == 0) { throw new ArgumentException(SR.GetString(SR.security_ExtendedProtectionPolicy_NoEmptyServiceNameCollection), "customServiceNames"); } this.policyEnforcement = policyEnforcement; this.protectionScenario = protectionScenario; this.customServiceNames = customServiceNames; } public ExtendedProtectionPolicy(PolicyEnforcement policyEnforcement, ProtectionScenario protectionScenario, ICollection customServiceNames) : this(policyEnforcement, protectionScenario, customServiceNames == null ? (ServiceNameCollection)null : new ServiceNameCollection(customServiceNames)) { } public ExtendedProtectionPolicy(PolicyEnforcement policyEnforcement, ChannelBinding customChannelBinding) { if (policyEnforcement == PolicyEnforcement.Never) { throw new ArgumentException(SR.GetString(SR.security_ExtendedProtectionPolicy_UseDifferentConstructorForNever), "policyEnforcement"); } if (customChannelBinding == null) { throw new ArgumentNullException("customChannelBinding"); } this.policyEnforcement = policyEnforcement; this.protectionScenario = ProtectionScenario.TransportSelected; this.customChannelBinding = customChannelBinding; } public ExtendedProtectionPolicy(PolicyEnforcement policyEnforcement) { // this is the only constructor which allows PolicyEnforcement.Never. this.policyEnforcement = policyEnforcement; this.protectionScenario = ProtectionScenario.TransportSelected; } protected ExtendedProtectionPolicy(SerializationInfo info, StreamingContext context) { policyEnforcement = (PolicyEnforcement)info.GetInt32(policyEnforcementName); protectionScenario = (ProtectionScenario)info.GetInt32(protectionScenarioName); customServiceNames = (ServiceNameCollection)info.GetValue(customServiceNamesName, typeof(ServiceNameCollection)); byte[] channelBindingData = (byte[])info.GetValue(customChannelBindingName, typeof(byte[])); if (channelBindingData != null) { customChannelBinding = SafeLocalFreeChannelBinding.LocalAlloc(channelBindingData.Length); Marshal.Copy(channelBindingData, 0, customChannelBinding.DangerousGetHandle(), channelBindingData.Length); } } public ServiceNameCollection CustomServiceNames { get { return customServiceNames; } } public PolicyEnforcement PolicyEnforcement { get { return policyEnforcement; } } public ProtectionScenario ProtectionScenario { get { return protectionScenario; } } public ChannelBinding CustomChannelBinding { get { return customChannelBinding; } } public override string ToString() { StringBuilder sb = new StringBuilder(); sb.Append("ProtectionScenario="); sb.Append(protectionScenario.ToString()); sb.Append("; PolicyEnforcement="); sb.Append(policyEnforcement.ToString()); sb.Append("; CustomChannelBinding="); if (customChannelBinding == null) { sb.Append(""); } else { sb.Append(customChannelBinding.ToString()); } sb.Append("; ServiceNames="); if (customServiceNames == null) { sb.Append(" "); } else { bool first = true; foreach (string serviceName in customServiceNames) { if (first) { first = false; } else { sb.Append(", "); } sb.Append(serviceName); } } return sb.ToString(); } public static bool OSSupportsExtendedProtection { get { return AuthenticationManager.OSSupportsExtendedProtection; } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, SerializationFormatter = true)] void ISerializable.GetObjectData(SerializationInfo info, StreamingContext context) { info.AddValue(policyEnforcementName, (int)policyEnforcement); info.AddValue(protectionScenarioName, (int)protectionScenario); info.AddValue(customServiceNamesName, customServiceNames, typeof(ServiceNameCollection)); if (customChannelBinding == null) { info.AddValue(customChannelBindingName, null, typeof(byte[])); } else { byte[] channelBindingData = new byte[customChannelBinding.Size]; Marshal.Copy(customChannelBinding.DangerousGetHandle(), channelBindingData, 0, customChannelBinding.Size); info.AddValue(customChannelBindingName, channelBindingData, typeof(byte[])); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
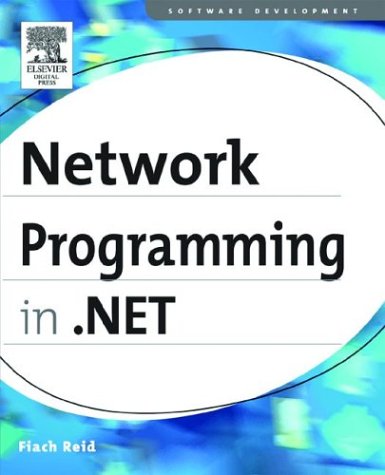
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebChannelFactory.cs
- PauseStoryboard.cs
- InstanceHandleReference.cs
- DynamicVirtualDiscoSearcher.cs
- TextParaLineResult.cs
- TextBoxBase.cs
- PropertyPath.cs
- CachedBitmap.cs
- ReadOnlyObservableCollection.cs
- CompositeKey.cs
- EncodingNLS.cs
- mongolianshape.cs
- ADConnectionHelper.cs
- SQLByteStorage.cs
- GridToolTip.cs
- MiniCustomAttributeInfo.cs
- webeventbuffer.cs
- DesigntimeLicenseContextSerializer.cs
- ToolStripContentPanel.cs
- MetadataItem.cs
- EventProviderBase.cs
- DataGridViewRowPostPaintEventArgs.cs
- JsonClassDataContract.cs
- BamlLocalizableResourceKey.cs
- HeaderedContentControl.cs
- Sql8ConformanceChecker.cs
- MetadataItemEmitter.cs
- WebPartUtil.cs
- SqlDataRecord.cs
- RuntimeComponentFilter.cs
- AsyncDataRequest.cs
- AnnotationService.cs
- HandoffBehavior.cs
- RegisteredHiddenField.cs
- BitmapImage.cs
- CorruptingExceptionCommon.cs
- SessionStateItemCollection.cs
- TableLayoutSettingsTypeConverter.cs
- CollectionAdapters.cs
- PageContentAsyncResult.cs
- TextEndOfParagraph.cs
- QilScopedVisitor.cs
- AssemblyCache.cs
- Pair.cs
- DeviceContext.cs
- PropertyItem.cs
- QilXmlReader.cs
- PhysicalAddress.cs
- ADConnectionHelper.cs
- HScrollProperties.cs
- IProvider.cs
- StringKeyFrameCollection.cs
- HTMLTagNameToTypeMapper.cs
- XmlNamedNodeMap.cs
- ProviderCollection.cs
- ThreadStaticAttribute.cs
- LinearKeyFrames.cs
- BitmapFrameDecode.cs
- EntityProviderFactory.cs
- TransactionException.cs
- TerminatorSinks.cs
- ResolveRequestResponseAsyncResult.cs
- BinHexEncoder.cs
- ContextMarshalException.cs
- _FtpControlStream.cs
- RotateTransform3D.cs
- ImageMap.cs
- SystemParameters.cs
- TextChange.cs
- AdjustableArrowCap.cs
- AnnotationHelper.cs
- XmlEnumAttribute.cs
- CodeMemberEvent.cs
- DocumentXPathNavigator.cs
- RowParagraph.cs
- ThumbAutomationPeer.cs
- JpegBitmapDecoder.cs
- GeneratedCodeAttribute.cs
- BoolExpr.cs
- ContainerSelectorActiveEvent.cs
- SchemaConstraints.cs
- httpstaticobjectscollection.cs
- Animatable.cs
- PreviewKeyDownEventArgs.cs
- MarkupCompiler.cs
- XmlObjectSerializerWriteContext.cs
- ImageClickEventArgs.cs
- Brush.cs
- TypeLibConverter.cs
- WorkflowOperationContext.cs
- XhtmlConformanceSection.cs
- FrameworkElementAutomationPeer.cs
- VersionPair.cs
- CodeAttachEventStatement.cs
- DataGridViewImageColumn.cs
- _SSPIWrapper.cs
- InfoCardBinaryReader.cs
- OleDbConnectionFactory.cs
- _ConnectionGroup.cs
- TextRangeSerialization.cs