Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripButton.cs / 1 / ToolStripButton.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Windows.Forms; using System.Drawing.Imaging; using System.ComponentModel; using System.Windows.Forms.Design; ////// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.ToolStrip)] public class ToolStripButton : ToolStripItem { private CheckState checkState = CheckState.Unchecked; private bool checkOnClick = false; private const int StandardButtonWidth = 23; private static readonly object EventCheckedChanged = new object(); private static readonly object EventCheckStateChanged = new object(); /// /// /// Summary of ToolStripButton. /// public ToolStripButton() { Initialize(); } public ToolStripButton(string text):base(text,null,null) { Initialize(); } public ToolStripButton(Image image):base(null,image,null) { Initialize(); } public ToolStripButton(string text, Image image):base(text,image,null) { Initialize(); } public ToolStripButton(string text, Image image, EventHandler onClick):base(text,image,onClick) { Initialize(); } public ToolStripButton(string text, Image image, EventHandler onClick, string name):base(text,image,onClick,name) { Initialize(); } [DefaultValue(true)] public new bool AutoToolTip { get { return base.AutoToolTip; } set { base.AutoToolTip = value; } } ////// /// Summary of CanSelect. /// public override bool CanSelect { get { return true; } } ///[ DefaultValue(false), SRCategory(SR.CatBehavior), SRDescription(SR.ToolStripButtonCheckOnClickDescr) ] public bool CheckOnClick { get { return checkOnClick; } set { checkOnClick = value; } } /// /// /// [ DefaultValue(false), SRCategory(SR.CatAppearance), SRDescription(SR.ToolStripButtonCheckedDescr) ] public bool Checked { get { return checkState != CheckState.Unchecked; } set { if (value != Checked) { CheckState = value ? CheckState.Checked : CheckState.Unchecked; InvokePaint(); } } } ////// Gets or sets a value indicating whether the item is checked. /// ////// /// [ SRCategory(SR.CatAppearance), DefaultValue(CheckState.Unchecked), SRDescription(SR.CheckBoxCheckStateDescr) ] public CheckState CheckState { get { return checkState; } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)CheckState.Unchecked, (int)CheckState.Indeterminate)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(CheckState)); } if (value != checkState) { checkState = value; Invalidate(); OnCheckedChanged(EventArgs.Empty); OnCheckStateChanged(EventArgs.Empty); } } } ///Gets /// or sets a value indicating whether the check box is checked. ////// /// [SRDescription(SR.CheckBoxOnCheckedChangedDescr)] public event EventHandler CheckedChanged { add { Events.AddHandler(EventCheckedChanged, value); } remove { Events.RemoveHandler(EventCheckedChanged, value); } } ///Occurs when the /// value of the ////// property changes. /// /// [SRDescription(SR.CheckBoxOnCheckStateChangedDescr)] public event EventHandler CheckStateChanged { add { Events.AddHandler(EventCheckStateChanged, value); } remove { Events.RemoveHandler(EventCheckStateChanged, value); } } protected override bool DefaultAutoToolTip { get { return true; } } ///Occurs when the /// value of the ////// property changes. /// /// constructs the new instance of the accessibility object for this ToolStripItem. Subclasses /// should not call base.CreateAccessibilityObject. /// [EditorBrowsable(EditorBrowsableState.Advanced)] protected override AccessibleObject CreateAccessibilityInstance() { return new ToolStripButtonAccessibleObject(this); } public override Size GetPreferredSize(Size constrainingSize) { Size prefSize = base.GetPreferredSize(constrainingSize); prefSize.Width = Math.Max(prefSize.Width, StandardButtonWidth); return prefSize; } ////// Called by all constructors of ToolStripButton. /// private void Initialize() { SupportsSpaceKey = true; } ////// /// protected virtual void OnCheckedChanged(EventArgs e) { EventHandler handler = (EventHandler)Events[EventCheckedChanged]; if (handler != null) handler(this,e); } ///Raises the ////// event. /// /// protected virtual void OnCheckStateChanged(EventArgs e) { AccessibilityNotifyClients(AccessibleEvents.StateChange); EventHandler handler = (EventHandler)Events[EventCheckStateChanged]; if (handler != null) handler(this,e); } ///Raises the ///event. /// /// Inheriting classes should override this method to handle this event. /// protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { if (this.Owner != null) { ToolStripRenderer renderer = this.Renderer; renderer.DrawButtonBackground(new ToolStripItemRenderEventArgs(e.Graphics, this)); if ((DisplayStyle & ToolStripItemDisplayStyle.Image) == ToolStripItemDisplayStyle.Image) { ToolStripItemImageRenderEventArgs rea = new ToolStripItemImageRenderEventArgs(e.Graphics, this, InternalLayout.ImageRectangle); rea.ShiftOnPress = true; renderer.DrawItemImage(rea); } if ((DisplayStyle & ToolStripItemDisplayStyle.Text) == ToolStripItemDisplayStyle.Text) { renderer.DrawItemText(new ToolStripItemTextRenderEventArgs(e.Graphics, this, this.Text, InternalLayout.TextRectangle, this.ForeColor, this.Font, InternalLayout.TextFormat)); } } } ///protected override void OnClick(EventArgs e) { if (checkOnClick) { this.Checked = !this.Checked; } base.OnClick(e); } /// /// An implementation of AccessibleChild for use with ToolStripItems /// [System.Runtime.InteropServices.ComVisible(true)] internal class ToolStripButtonAccessibleObject : ToolStripItemAccessibleObject { private ToolStripButton ownerItem = null; public ToolStripButtonAccessibleObject(ToolStripButton ownerItem): base(ownerItem) { this.ownerItem = ownerItem; } public override AccessibleStates State { get { if (ownerItem.Enabled && ownerItem.Checked) { return base.State | AccessibleStates.Checked; } return base.State; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
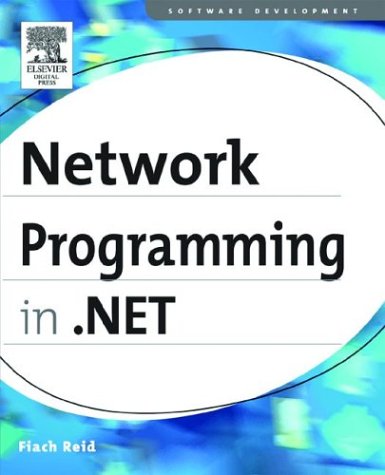
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaComplexType.cs
- Guid.cs
- WindowsTooltip.cs
- LogReservationCollection.cs
- _Semaphore.cs
- MimeTypePropertyAttribute.cs
- OleDbInfoMessageEvent.cs
- UniqueSet.cs
- DataTemplate.cs
- RichTextBoxConstants.cs
- BinaryReader.cs
- PageMediaSize.cs
- SqlOuterApplyReducer.cs
- DataGridCellsPanel.cs
- ColumnMapCopier.cs
- SQLGuid.cs
- RemoteWebConfigurationHost.cs
- DefaultAssemblyResolver.cs
- CounterSample.cs
- DetailsViewPagerRow.cs
- CqlLexerHelpers.cs
- UnsafeNativeMethods.cs
- SchemaInfo.cs
- OutputCacheSettingsSection.cs
- SqlClientWrapperSmiStream.cs
- DbBuffer.cs
- StringOutput.cs
- AppSettingsExpressionBuilder.cs
- WorkflowDataContext.cs
- KeyFrames.cs
- Vector3DValueSerializer.cs
- MatrixUtil.cs
- XmlNamedNodeMap.cs
- WebPartAddingEventArgs.cs
- ListViewUpdatedEventArgs.cs
- DataGridViewCell.cs
- DragCompletedEventArgs.cs
- XmlObjectSerializerContext.cs
- StatusBarItemAutomationPeer.cs
- CompilationLock.cs
- RSAPKCS1SignatureDeformatter.cs
- CancelRequestedQuery.cs
- ConstraintEnumerator.cs
- ShapingWorkspace.cs
- odbcmetadatacolumnnames.cs
- SizeAnimationClockResource.cs
- LogReserveAndAppendState.cs
- BrushConverter.cs
- ChameleonKey.cs
- XamlPathDataSerializer.cs
- DATA_BLOB.cs
- GregorianCalendarHelper.cs
- InputQueueChannel.cs
- Page.cs
- DataServiceProcessingPipeline.cs
- Switch.cs
- DbParameterCollection.cs
- Size.cs
- XmlILModule.cs
- MetadataPropertyAttribute.cs
- RedBlackList.cs
- EpmCustomContentDeSerializer.cs
- DocumentPaginator.cs
- mactripleDES.cs
- OrderedDictionary.cs
- XmlTextAttribute.cs
- AssemblyUtil.cs
- VisualBrush.cs
- RealizationContext.cs
- FileDialog.cs
- ByteKeyFrameCollection.cs
- Resources.Designer.cs
- OneToOneMappingSerializer.cs
- JumpTask.cs
- ScopelessEnumAttribute.cs
- MappingMetadataHelper.cs
- DataGridViewCellStyleConverter.cs
- Transform3DGroup.cs
- ParserExtension.cs
- TextBoxLine.cs
- QilLoop.cs
- CodeIdentifier.cs
- WebBrowserUriTypeConverter.cs
- BoundPropertyEntry.cs
- DatatypeImplementation.cs
- DataGridViewCellMouseEventArgs.cs
- DataGridCellAutomationPeer.cs
- MergeFilterQuery.cs
- NullReferenceException.cs
- Frame.cs
- ModuleBuilder.cs
- DocumentPageHost.cs
- DbModificationClause.cs
- QilUnary.cs
- Int16.cs
- BitmapMetadataEnumerator.cs
- ToolBarButton.cs
- QueryTask.cs
- DiagnosticsConfiguration.cs
- sqlinternaltransaction.cs