Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / InputQueueChannel.cs / 1 / InputQueueChannel.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; abstract class InputQueueChannel: ChannelBase where TDisposable : class, IDisposable { InputQueue inputQueue; protected InputQueueChannel(ChannelManagerBase channelManager) : base(channelManager) { this.inputQueue = new InputQueue (); } public int InternalPendingItems { get { return this.inputQueue.PendingCount; } } public int PendingItems { get { ThrowIfDisposedOrNotOpen(); return InternalPendingItems; } } public void EnqueueAndDispatch(TDisposable item) { EnqueueAndDispatch(item, null); } public void EnqueueAndDispatch(TDisposable item, ItemDequeuedCallback dequeuedCallback, bool canDispatchOnThisThread) { OnEnqueueItem(item); // NOTE: don't need to check IsDisposed here: InputQueue will handle dispose inputQueue.EnqueueAndDispatch(item, dequeuedCallback, canDispatchOnThisThread); } public void EnqueueAndDispatch(Exception exception, ItemDequeuedCallback dequeuedCallback, bool canDispatchOnThisThread) { // NOTE: don't need to check IsDisposed here: InputQueue will handle dispose inputQueue.EnqueueAndDispatch(exception, dequeuedCallback, canDispatchOnThisThread); } public void EnqueueAndDispatch(TDisposable item, ItemDequeuedCallback dequeuedCallback) { OnEnqueueItem(item); // NOTE: don't need to check IsDisposed here: InputQueue will handle dispose inputQueue.EnqueueAndDispatch(item, dequeuedCallback); } public bool EnqueueWithoutDispatch(Exception exception, ItemDequeuedCallback dequeuedCallback) { // NOTE: don't need to check IsDisposed here: InputQueue will handle dispose return inputQueue.EnqueueWithoutDispatch(exception, dequeuedCallback); } public bool EnqueueWithoutDispatch(TDisposable item, ItemDequeuedCallback dequeuedCallback) { OnEnqueueItem(item); // NOTE: don't need to check IsDisposed here: InputQueue will handle dispose return inputQueue.EnqueueWithoutDispatch(item, dequeuedCallback); } public void Dispatch() { // NOTE: don't need to check IsDisposed here: InputQueue will handle dispose inputQueue.Dispatch(); } public void Shutdown() { inputQueue.Shutdown(); } protected override void OnFaulted() { base.OnFaulted(); inputQueue.Shutdown(this); } protected virtual void OnEnqueueItem(TDisposable item) { } protected IAsyncResult BeginDequeue(TimeSpan timeout, AsyncCallback callback, object state) { this.ThrowIfNotOpened(); return inputQueue.BeginDequeue(timeout, callback, state); } protected bool EndDequeue(IAsyncResult result, out TDisposable item) { bool dequeued = inputQueue.EndDequeue(result, out item); if (item == null) { this.ThrowIfFaulted(); this.ThrowIfAborted(); } return dequeued; } protected bool Dequeue(TimeSpan timeout, out TDisposable item) { this.ThrowIfNotOpened(); bool dequeued = inputQueue.Dequeue(timeout, out item); if (item == null) { this.ThrowIfFaulted(); this.ThrowIfAborted(); } return dequeued; } protected bool WaitForItem(TimeSpan timeout) { this.ThrowIfNotOpened(); bool dequeued = inputQueue.WaitForItem(timeout); this.ThrowIfFaulted(); this.ThrowIfAborted(); return dequeued; } protected IAsyncResult BeginWaitForItem(TimeSpan timeout, AsyncCallback callback, object state) { this.ThrowIfNotOpened(); return inputQueue.BeginWaitForItem(timeout, callback, state); } protected bool EndWaitForItem(IAsyncResult result) { bool dequeued = inputQueue.EndWaitForItem(result); this.ThrowIfFaulted(); this.ThrowIfAborted(); return dequeued; } protected override void OnClosing() { base.OnClosing(); inputQueue.Shutdown(this); } protected override void OnAbort() { inputQueue.Close(); } protected override void OnClose(TimeSpan timeout) { inputQueue.Close(); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { inputQueue.Close(); return new CompletedAsyncResult(callback, state); } protected override void OnEndClose(IAsyncResult result) { CompletedAsyncResult.End(result); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
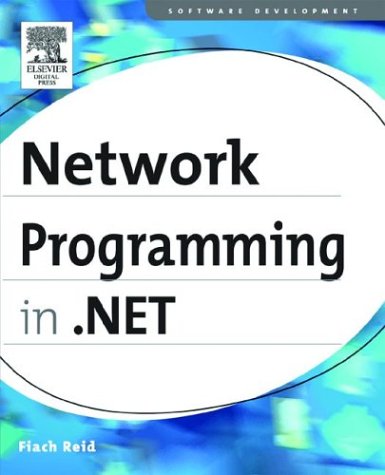
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompositeKey.cs
- SecureUICommand.cs
- AssemblyUtil.cs
- PackageRelationshipSelector.cs
- SequenceDesigner.xaml.cs
- SafeFileHandle.cs
- SpinWait.cs
- OleDbMetaDataFactory.cs
- GPRECT.cs
- CssClassPropertyAttribute.cs
- Tokenizer.cs
- WebPartCancelEventArgs.cs
- MeshGeometry3D.cs
- SqlDataSourceConfigureSelectPanel.cs
- BitmapPalette.cs
- SupportsEventValidationAttribute.cs
- DirectoryInfo.cs
- MD5CryptoServiceProvider.cs
- ListViewItemMouseHoverEvent.cs
- CapabilitiesPattern.cs
- SkinBuilder.cs
- WebBrowserPermission.cs
- DocumentViewerAutomationPeer.cs
- SmtpNegotiateAuthenticationModule.cs
- InplaceBitmapMetadataWriter.cs
- ReflectionUtil.cs
- CodeCommentStatementCollection.cs
- ThreadPool.cs
- NullEntityWrapper.cs
- ClosableStream.cs
- TextModifier.cs
- ColorInterpolationModeValidation.cs
- ReflectionTypeLoadException.cs
- _DisconnectOverlappedAsyncResult.cs
- TemplateColumn.cs
- Monitor.cs
- TemplateNameScope.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- XamlStyleSerializer.cs
- TemplateBindingExpressionConverter.cs
- BindingMemberInfo.cs
- Range.cs
- PeerNameResolver.cs
- WindowsFormsSynchronizationContext.cs
- GregorianCalendarHelper.cs
- BaseHashHelper.cs
- _HeaderInfoTable.cs
- WSDualHttpSecurityElement.cs
- OleDbInfoMessageEvent.cs
- WindowProviderWrapper.cs
- GenericWebPart.cs
- UnionExpr.cs
- XmlExtensionFunction.cs
- CollectionChangeEventArgs.cs
- RepeatBehavior.cs
- MenuEventArgs.cs
- WpfPayload.cs
- SkipStoryboardToFill.cs
- SrgsItemList.cs
- MessagingDescriptionAttribute.cs
- DataMisalignedException.cs
- ButtonFlatAdapter.cs
- Journal.cs
- CurrentChangingEventArgs.cs
- DbUpdateCommandTree.cs
- CodeRegionDirective.cs
- QueryContinueDragEvent.cs
- SiteIdentityPermission.cs
- BindingCollection.cs
- RegexFCD.cs
- ExpressionBinding.cs
- FactoryRecord.cs
- HtmlInputRadioButton.cs
- OletxTransactionManager.cs
- ProviderException.cs
- CharacterMetricsDictionary.cs
- DeclaredTypeValidator.cs
- ProtocolViolationException.cs
- ObjectTypeMapping.cs
- UriPrefixTable.cs
- WebBrowserUriTypeConverter.cs
- Storyboard.cs
- FullTextLine.cs
- HttpCacheParams.cs
- PropertyValueChangedEvent.cs
- FixedTextContainer.cs
- Matrix3D.cs
- XhtmlConformanceSection.cs
- Sql8ExpressionRewriter.cs
- DataGridViewCellConverter.cs
- HasCopySemanticsAttribute.cs
- XmlSchemaObjectTable.cs
- ToolStrip.cs
- DecimalAverageAggregationOperator.cs
- DataGridItemCollection.cs
- WebSysDefaultValueAttribute.cs
- DataViewManagerListItemTypeDescriptor.cs
- UnsafeNativeMethods.cs
- SystemGatewayIPAddressInformation.cs
- ClientScriptManager.cs