Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Animation / RepeatBehavior.cs / 1 / RepeatBehavior.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: RepeatBehavior.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using MS.Internal; using System.ComponentModel; using System.Diagnostics; using System.Text; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// A RepeatBehavior describes how a Timeline object may repeat its simple duration. /// There are three types of RepeatBehavior behaviors: IterationCount, RepeatDuration, and Forever. /// [TypeConverter(typeof(RepeatBehaviorConverter))] public struct RepeatBehavior : IFormattable { private double _iterationCount; private TimeSpan _repeatDuration; private RepeatBehaviorType _type; #region Constructors ///An IterationCount RepeatBehavior specifies the number of times the simple duration of a Timeline will /// be repeated. An iteration count of 0.5 means the Timeline will only be active for half /// of its simple duration and will only reach 50% progress. An iteration count of 1.0 is the /// default and means a Timeline will be active for exactly one of its simple durations. An /// iteration count of 2.0 means a Timeline will run twice, or repeat its simple duration /// once after its initial simple duration. ///A RepeatDuration RepeatBehavior specifies the amount of time that a Timeline will repeat. /// For instance if a Timeline has a simple Duration value of 1 second and a RepeatBehavior with a /// RepeatDuration value of 2.5 seconds, then it will run for 2.5 iterations. ///A Forever RepeatBehavior specifies that a Timeline will repeat forever. ////// Creates a new RepeatBehavior that represents and iteration count. /// /// The number of iterations specified by this RepeatBehavior. public RepeatBehavior(double count) { if ( Double.IsInfinity(count) || DoubleUtil.IsNaN(count) || count < 0.0) { throw new ArgumentOutOfRangeException("count", SR.Get(SRID.Timing_RepeatBehaviorInvalidIterationCount, count)); } _repeatDuration = new TimeSpan(0); _iterationCount = count; _type = RepeatBehaviorType.IterationCount; } ////// Creates a new RepeatBehavior that represents a repeat duration for which a Timeline will repeat /// its simple duration. /// /// A TimeSpan representing the repeat duration specified by this RepeatBehavior. public RepeatBehavior(TimeSpan duration) { if (duration < new TimeSpan(0)) { throw new ArgumentOutOfRangeException("duration", SR.Get(SRID.Timing_RepeatBehaviorInvalidRepeatDuration, duration)); } _iterationCount = 0.0; _repeatDuration = duration; _type = RepeatBehaviorType.RepeatDuration; } ////// Creates and returns a RepeatBehavior that indicates that a Timeline should repeat its /// simple duration forever. /// ///A RepeatBehavior that indicates that a Timeline should repeat its simple duration /// forever. public static RepeatBehavior Forever { get { RepeatBehavior forever = new RepeatBehavior(); forever._type = RepeatBehaviorType.Forever; return forever; } } #endregion // Constructors #region Properties ////// Indicates whether this RepeatBehavior represents an iteration count. /// ///True if this RepeatBehavior represents an iteration count; otherwise false. public bool HasCount { get { return _type == RepeatBehaviorType.IterationCount; } } ////// Indicates whether this RepeatBehavior represents a repeat duration. /// ///True if this RepeatBehavior represents a repeat duration; otherwise false. public bool HasDuration { get { return _type == RepeatBehaviorType.RepeatDuration; } } ////// Returns the iteration count specified by this RepeatBehavior. /// ///The iteration count specified by this RepeatBehavior. ///Thrown if this RepeatBehavior does not represent an iteration count. public double Count { get { if (_type != RepeatBehaviorType.IterationCount) { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Timing_RepeatBehaviorNotIterationCount, this)); } return _iterationCount; } } ////// Returns the repeat duration specified by this RepeatBehavior. /// ///A TimeSpan representing the repeat duration specified by this RepeatBehavior. ///Thrown if this RepeatBehavior does not represent a repeat duration. public TimeSpan Duration { get { if (_type != RepeatBehaviorType.RepeatDuration) { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Timing_RepeatBehaviorNotRepeatDuration, this)); } return _repeatDuration; } } #endregion // Properties #region Methods ////// Indicates whether the specified Object is equal to this RepeatBehavior. /// /// ///true if value is a RepeatBehavior and is equal to this instance; otherwise false. public override bool Equals(Object value) { if (value is RepeatBehavior) { return this.Equals((RepeatBehavior)value); } else { return false; } } ////// Indicates whether the specified RepeatBehavior is equal to this RepeatBehavior. /// /// A RepeatBehavior to compare with this RepeatBehavior. ///true if repeatBehavior is equal to this instance; otherwise false. public bool Equals(RepeatBehavior repeatBehavior) { if (_type == repeatBehavior._type) { switch (_type) { case RepeatBehaviorType.Forever: return true; case RepeatBehaviorType.IterationCount: return _iterationCount == repeatBehavior._iterationCount; case RepeatBehaviorType.RepeatDuration: return _repeatDuration == repeatBehavior._repeatDuration; default: Debug.Fail("Unhandled RepeatBehaviorType"); return false; } } else { return false; } } ////// Indicates whether the specified RepeatBehaviors are equal to each other. /// /// /// ///true if repeatBehavior1 and repeatBehavior2 are equal; otherwise false. public static bool Equals(RepeatBehavior repeatBehavior1, RepeatBehavior repeatBehavior2) { return repeatBehavior1.Equals(repeatBehavior2); } ////// Generates a hash code for this RepeatBehavior. /// ///A hash code for this RepeatBehavior. public override int GetHashCode() { switch (_type) { case RepeatBehaviorType.IterationCount: return _iterationCount.GetHashCode(); case RepeatBehaviorType.RepeatDuration: return _repeatDuration.GetHashCode(); case RepeatBehaviorType.Forever: // We try to choose an unlikely hash code value for Forever. // All Forevers need to return the same hash code value. return int.MaxValue - 42; default: Debug.Fail("Unhandled RepeatBehaviorType"); return base.GetHashCode(); } } ////// Creates a string representation of this RepeatBehavior based on the current culture. /// ///A string representation of this RepeatBehavior based on the current culture. public override string ToString() { return InternalToString(null, null); } ////// /// /// ///public string ToString(IFormatProvider formatProvider) { return InternalToString(null, formatProvider); } /// /// /// /// /// ///string IFormattable.ToString(string format, IFormatProvider formatProvider) { return InternalToString(format, formatProvider); } /// /// /// /// /// ///internal string InternalToString(string format, IFormatProvider formatProvider) { switch (_type) { case RepeatBehaviorType.Forever: return "Forever"; case RepeatBehaviorType.IterationCount: StringBuilder sb = new StringBuilder(); sb.AppendFormat( formatProvider, "{0:" + format + "}x", _iterationCount); return sb.ToString(); case RepeatBehaviorType.RepeatDuration: return _repeatDuration.ToString(); default: Debug.Fail("Unhandled RepeatBehaviorType."); return null; } } #endregion // Methods #region Operators /// /// Indicates whether the specified RepeatBehaviors are equal to each other. /// /// /// ///true if repeatBehavior1 and repeatBehavior2 are equal; otherwise false. public static bool operator ==(RepeatBehavior repeatBehavior1, RepeatBehavior repeatBehavior2) { return repeatBehavior1.Equals(repeatBehavior2); } ////// Indicates whether the specified RepeatBehaviors are not equal to each other. /// /// /// ///true if repeatBehavior1 and repeatBehavior2 are not equal; otherwise false. public static bool operator !=(RepeatBehavior repeatBehavior1, RepeatBehavior repeatBehavior2) { return !repeatBehavior1.Equals(repeatBehavior2); } #endregion // Operators ////// An enumeration of the different types of RepeatBehavior behaviors. /// private enum RepeatBehaviorType { IterationCount, RepeatDuration, Forever } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: RepeatBehavior.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using MS.Internal; using System.ComponentModel; using System.Diagnostics; using System.Text; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// A RepeatBehavior describes how a Timeline object may repeat its simple duration. /// There are three types of RepeatBehavior behaviors: IterationCount, RepeatDuration, and Forever. /// [TypeConverter(typeof(RepeatBehaviorConverter))] public struct RepeatBehavior : IFormattable { private double _iterationCount; private TimeSpan _repeatDuration; private RepeatBehaviorType _type; #region Constructors ///An IterationCount RepeatBehavior specifies the number of times the simple duration of a Timeline will /// be repeated. An iteration count of 0.5 means the Timeline will only be active for half /// of its simple duration and will only reach 50% progress. An iteration count of 1.0 is the /// default and means a Timeline will be active for exactly one of its simple durations. An /// iteration count of 2.0 means a Timeline will run twice, or repeat its simple duration /// once after its initial simple duration. ///A RepeatDuration RepeatBehavior specifies the amount of time that a Timeline will repeat. /// For instance if a Timeline has a simple Duration value of 1 second and a RepeatBehavior with a /// RepeatDuration value of 2.5 seconds, then it will run for 2.5 iterations. ///A Forever RepeatBehavior specifies that a Timeline will repeat forever. ////// Creates a new RepeatBehavior that represents and iteration count. /// /// The number of iterations specified by this RepeatBehavior. public RepeatBehavior(double count) { if ( Double.IsInfinity(count) || DoubleUtil.IsNaN(count) || count < 0.0) { throw new ArgumentOutOfRangeException("count", SR.Get(SRID.Timing_RepeatBehaviorInvalidIterationCount, count)); } _repeatDuration = new TimeSpan(0); _iterationCount = count; _type = RepeatBehaviorType.IterationCount; } ////// Creates a new RepeatBehavior that represents a repeat duration for which a Timeline will repeat /// its simple duration. /// /// A TimeSpan representing the repeat duration specified by this RepeatBehavior. public RepeatBehavior(TimeSpan duration) { if (duration < new TimeSpan(0)) { throw new ArgumentOutOfRangeException("duration", SR.Get(SRID.Timing_RepeatBehaviorInvalidRepeatDuration, duration)); } _iterationCount = 0.0; _repeatDuration = duration; _type = RepeatBehaviorType.RepeatDuration; } ////// Creates and returns a RepeatBehavior that indicates that a Timeline should repeat its /// simple duration forever. /// ///A RepeatBehavior that indicates that a Timeline should repeat its simple duration /// forever. public static RepeatBehavior Forever { get { RepeatBehavior forever = new RepeatBehavior(); forever._type = RepeatBehaviorType.Forever; return forever; } } #endregion // Constructors #region Properties ////// Indicates whether this RepeatBehavior represents an iteration count. /// ///True if this RepeatBehavior represents an iteration count; otherwise false. public bool HasCount { get { return _type == RepeatBehaviorType.IterationCount; } } ////// Indicates whether this RepeatBehavior represents a repeat duration. /// ///True if this RepeatBehavior represents a repeat duration; otherwise false. public bool HasDuration { get { return _type == RepeatBehaviorType.RepeatDuration; } } ////// Returns the iteration count specified by this RepeatBehavior. /// ///The iteration count specified by this RepeatBehavior. ///Thrown if this RepeatBehavior does not represent an iteration count. public double Count { get { if (_type != RepeatBehaviorType.IterationCount) { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Timing_RepeatBehaviorNotIterationCount, this)); } return _iterationCount; } } ////// Returns the repeat duration specified by this RepeatBehavior. /// ///A TimeSpan representing the repeat duration specified by this RepeatBehavior. ///Thrown if this RepeatBehavior does not represent a repeat duration. public TimeSpan Duration { get { if (_type != RepeatBehaviorType.RepeatDuration) { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Timing_RepeatBehaviorNotRepeatDuration, this)); } return _repeatDuration; } } #endregion // Properties #region Methods ////// Indicates whether the specified Object is equal to this RepeatBehavior. /// /// ///true if value is a RepeatBehavior and is equal to this instance; otherwise false. public override bool Equals(Object value) { if (value is RepeatBehavior) { return this.Equals((RepeatBehavior)value); } else { return false; } } ////// Indicates whether the specified RepeatBehavior is equal to this RepeatBehavior. /// /// A RepeatBehavior to compare with this RepeatBehavior. ///true if repeatBehavior is equal to this instance; otherwise false. public bool Equals(RepeatBehavior repeatBehavior) { if (_type == repeatBehavior._type) { switch (_type) { case RepeatBehaviorType.Forever: return true; case RepeatBehaviorType.IterationCount: return _iterationCount == repeatBehavior._iterationCount; case RepeatBehaviorType.RepeatDuration: return _repeatDuration == repeatBehavior._repeatDuration; default: Debug.Fail("Unhandled RepeatBehaviorType"); return false; } } else { return false; } } ////// Indicates whether the specified RepeatBehaviors are equal to each other. /// /// /// ///true if repeatBehavior1 and repeatBehavior2 are equal; otherwise false. public static bool Equals(RepeatBehavior repeatBehavior1, RepeatBehavior repeatBehavior2) { return repeatBehavior1.Equals(repeatBehavior2); } ////// Generates a hash code for this RepeatBehavior. /// ///A hash code for this RepeatBehavior. public override int GetHashCode() { switch (_type) { case RepeatBehaviorType.IterationCount: return _iterationCount.GetHashCode(); case RepeatBehaviorType.RepeatDuration: return _repeatDuration.GetHashCode(); case RepeatBehaviorType.Forever: // We try to choose an unlikely hash code value for Forever. // All Forevers need to return the same hash code value. return int.MaxValue - 42; default: Debug.Fail("Unhandled RepeatBehaviorType"); return base.GetHashCode(); } } ////// Creates a string representation of this RepeatBehavior based on the current culture. /// ///A string representation of this RepeatBehavior based on the current culture. public override string ToString() { return InternalToString(null, null); } ////// /// /// ///public string ToString(IFormatProvider formatProvider) { return InternalToString(null, formatProvider); } /// /// /// /// /// ///string IFormattable.ToString(string format, IFormatProvider formatProvider) { return InternalToString(format, formatProvider); } /// /// /// /// /// ///internal string InternalToString(string format, IFormatProvider formatProvider) { switch (_type) { case RepeatBehaviorType.Forever: return "Forever"; case RepeatBehaviorType.IterationCount: StringBuilder sb = new StringBuilder(); sb.AppendFormat( formatProvider, "{0:" + format + "}x", _iterationCount); return sb.ToString(); case RepeatBehaviorType.RepeatDuration: return _repeatDuration.ToString(); default: Debug.Fail("Unhandled RepeatBehaviorType."); return null; } } #endregion // Methods #region Operators /// /// Indicates whether the specified RepeatBehaviors are equal to each other. /// /// /// ///true if repeatBehavior1 and repeatBehavior2 are equal; otherwise false. public static bool operator ==(RepeatBehavior repeatBehavior1, RepeatBehavior repeatBehavior2) { return repeatBehavior1.Equals(repeatBehavior2); } ////// Indicates whether the specified RepeatBehaviors are not equal to each other. /// /// /// ///true if repeatBehavior1 and repeatBehavior2 are not equal; otherwise false. public static bool operator !=(RepeatBehavior repeatBehavior1, RepeatBehavior repeatBehavior2) { return !repeatBehavior1.Equals(repeatBehavior2); } #endregion // Operators ////// An enumeration of the different types of RepeatBehavior behaviors. /// private enum RepeatBehaviorType { IterationCount, RepeatDuration, Forever } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
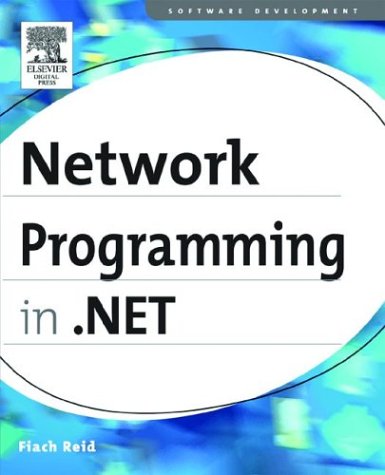
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegionIterator.cs
- AsyncResult.cs
- DrawingContext.cs
- DataListItemEventArgs.cs
- unsafenativemethodsother.cs
- Stack.cs
- SafeArrayRankMismatchException.cs
- EditorAttribute.cs
- SafeMILHandle.cs
- BindingRestrictions.cs
- StrongNameKeyPair.cs
- InvalidOleVariantTypeException.cs
- FailedToStartupUIException.cs
- BaseConfigurationRecord.cs
- UIAgentMonitor.cs
- RayMeshGeometry3DHitTestResult.cs
- smtppermission.cs
- FileRecordSequenceCompletedAsyncResult.cs
- GetPageCompletedEventArgs.cs
- MulticastNotSupportedException.cs
- HttpContextServiceHost.cs
- FileEnumerator.cs
- InternalConfigSettingsFactory.cs
- EncoderExceptionFallback.cs
- BamlBinaryReader.cs
- WindowsSpinner.cs
- ContentElementAutomationPeer.cs
- ExeConfigurationFileMap.cs
- ImplicitInputBrush.cs
- ZipIOBlockManager.cs
- OracleTransaction.cs
- AdapterDictionary.cs
- TextBoxBaseDesigner.cs
- CodeTypeMemberCollection.cs
- HttpProtocolImporter.cs
- BufferBuilder.cs
- ApplicationSecurityManager.cs
- FeatureManager.cs
- ErrorStyle.cs
- GroupBox.cs
- PropertyReferenceSerializer.cs
- AppDomainProtocolHandler.cs
- IFlowDocumentViewer.cs
- PropertyMapper.cs
- DataRelation.cs
- BitConverter.cs
- XslAstAnalyzer.cs
- ArithmeticException.cs
- HashLookup.cs
- ShaperBuffers.cs
- Int32Collection.cs
- EditorZone.cs
- Propagator.cs
- UnaryNode.cs
- ComponentResourceKey.cs
- SR.Designer.cs
- BuilderPropertyEntry.cs
- WebServiceResponseDesigner.cs
- MappingSource.cs
- EventLogPermissionEntry.cs
- DataServiceQueryProvider.cs
- HttpServerVarsCollection.cs
- xmlformatgeneratorstatics.cs
- DescendantBaseQuery.cs
- MimeFormatExtensions.cs
- ConfigXmlReader.cs
- ControlParameter.cs
- DataGridViewColumnHeaderCell.cs
- ContentElement.cs
- DbMetaDataColumnNames.cs
- _NetworkingPerfCounters.cs
- BaseInfoTable.cs
- MemoryMappedViewStream.cs
- KerberosSecurityTokenAuthenticator.cs
- QueryGeneratorBase.cs
- MemoryRecordBuffer.cs
- SQLBinary.cs
- ChangeDirector.cs
- XmlMapping.cs
- SoapElementAttribute.cs
- SymbolPair.cs
- WebAdminConfigurationHelper.cs
- DetailsViewInsertedEventArgs.cs
- SafePointer.cs
- ToolTip.cs
- listitem.cs
- PropertyMetadata.cs
- PageEventArgs.cs
- EqualityComparer.cs
- DataTableMapping.cs
- EntityCommandExecutionException.cs
- WsrmFault.cs
- RSAPKCS1SignatureDeformatter.cs
- HtmlInputPassword.cs
- NetTcpBinding.cs
- ResourceManagerWrapper.cs
- SqlBooleanMismatchVisitor.cs
- ExpressionTable.cs
- RightsManagementEncryptionTransform.cs
- XmlSchemaAppInfo.cs