Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / NetTcpBinding.cs / 1 / NetTcpBinding.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel { using System; using System.Diagnostics; using System.Text; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Configuration; using System.Globalization; using System.Net; using System.Net.Security; using System.Runtime.Serialization; using System.Security.Principal; using System.ServiceModel.Channels; using System.ServiceModel.Configuration; using System.ServiceModel.Security; using System.Xml; using System.Net.Sockets; public class NetTcpBinding : Binding, IBindingRuntimePreferences { OptionalReliableSession reliableSession; // private BindingElements TcpTransportBindingElement transport; BinaryMessageEncodingBindingElement encoding; TransactionFlowBindingElement context; ReliableSessionBindingElement session; NetTcpSecurity security = new NetTcpSecurity(); public NetTcpBinding() { Initialize(); } public NetTcpBinding(SecurityMode securityMode) : this() { this.security.Mode = securityMode; } public NetTcpBinding(SecurityMode securityMode, bool reliableSessionEnabled) : this(securityMode) { this.ReliableSession.Enabled = reliableSessionEnabled; } public NetTcpBinding(string configurationName) : this() { ApplyConfiguration(configurationName); } NetTcpBinding(TcpTransportBindingElement transport, BinaryMessageEncodingBindingElement encoding, TransactionFlowBindingElement context, ReliableSessionBindingElement session, NetTcpSecurity security) : this() { this.security = security; this.ReliableSession.Enabled = session != null; InitializeFrom(transport, encoding, context, session); } public bool TransactionFlow { get { return context.Transactions; } set { context.Transactions = value; } } public TransactionProtocol TransactionProtocol { get { return this.context.TransactionProtocol; } set { this.context.TransactionProtocol = value; } } public TransferMode TransferMode { get { return this.transport.TransferMode; } set { this.transport.TransferMode = value; } } public HostNameComparisonMode HostNameComparisonMode { get { return transport.HostNameComparisonMode; } set { transport.HostNameComparisonMode = value; } } public long MaxBufferPoolSize { get { return transport.MaxBufferPoolSize; } set { transport.MaxBufferPoolSize = value; } } public int MaxBufferSize { get { return transport.MaxBufferSize; } set { transport.MaxBufferSize = value; } } public int MaxConnections { get { return transport.MaxPendingConnections; } set { transport.MaxPendingConnections = value; transport.ConnectionPoolSettings.MaxOutboundConnectionsPerEndpoint = value; } } public int ListenBacklog { get { return transport.ListenBacklog; } set { transport.ListenBacklog = value; } } public long MaxReceivedMessageSize { get { return transport.MaxReceivedMessageSize; } set { transport.MaxReceivedMessageSize = value; } } public bool PortSharingEnabled { get { return transport.PortSharingEnabled; } set { transport.PortSharingEnabled = value; } } public XmlDictionaryReaderQuotas ReaderQuotas { get { return encoding.ReaderQuotas; } set { if (value == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); value.CopyTo(encoding.ReaderQuotas); } } bool IBindingRuntimePreferences.ReceiveSynchronously { get { return false; } } public OptionalReliableSession ReliableSession { get { return reliableSession; } } public override string Scheme { get { return transport.Scheme; } } public EnvelopeVersion EnvelopeVersion { get { return EnvelopeVersion.Soap12; } } public NetTcpSecurity Security { get { return security; } } static TransactionFlowBindingElement GetDefaultTransactionFlowBindingElement() { return new TransactionFlowBindingElement(false); } void Initialize() { transport = new TcpTransportBindingElement(); encoding = new BinaryMessageEncodingBindingElement(); context = GetDefaultTransactionFlowBindingElement(); session = new ReliableSessionBindingElement(); this.reliableSession = new OptionalReliableSession(session); } void InitializeFrom(TcpTransportBindingElement transport, BinaryMessageEncodingBindingElement encoding, TransactionFlowBindingElement context, ReliableSessionBindingElement session) { DiagnosticUtility.DebugAssert(transport != null, "Invalid (null) transport value."); DiagnosticUtility.DebugAssert(encoding != null, "Invalid (null) encoding value."); DiagnosticUtility.DebugAssert(context != null, "Invalid (null) context value."); DiagnosticUtility.DebugAssert(security != null, "Invalid (null) security value."); // transport this.HostNameComparisonMode = transport.HostNameComparisonMode; this.MaxBufferPoolSize = transport.MaxBufferPoolSize; this.MaxBufferSize = transport.MaxBufferSize; this.MaxConnections = transport.MaxPendingConnections; this.ListenBacklog = transport.ListenBacklog; this.MaxReceivedMessageSize = transport.MaxReceivedMessageSize; this.PortSharingEnabled = transport.PortSharingEnabled; this.TransferMode = transport.TransferMode; // encoding this.ReaderQuotas = encoding.ReaderQuotas; // context this.TransactionFlow = context.Transactions; this.TransactionProtocol = context.TransactionProtocol; //session if (session != null) { // only set properties that have standard binding manifestations this.session.InactivityTimeout = session.InactivityTimeout; this.session.Ordered = session.Ordered; } } // check that properties of the HttpTransportBindingElement and // MessageEncodingBindingElement not exposed as properties on BasicHttpBinding // match default values of the binding elements bool IsBindingElementsMatch(TcpTransportBindingElement transport, BinaryMessageEncodingBindingElement encoding, TransactionFlowBindingElement context, ReliableSessionBindingElement session) { if (!this.transport.IsMatch(transport)) return false; if (!this.encoding.IsMatch(encoding)) return false; if (!this.context.IsMatch(context)) return false; if (reliableSession.Enabled) { if (!this.session.IsMatch(session)) return false; } else if (session != null) return false; return true; } void ApplyConfiguration(string configurationName) { NetTcpBindingCollectionElement section = NetTcpBindingCollectionElement.GetBindingCollectionElement(); NetTcpBindingElement element = section.Bindings[configurationName]; if (element == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ConfigurationErrorsException( SR.GetString(SR.ConfigInvalidBindingConfigurationName, configurationName, ConfigurationStrings.NetTcpBindingCollectionElementName))); } else { element.ApplyConfiguration(this); } } public override BindingElementCollection CreateBindingElements() { // return collection of BindingElements BindingElementCollection bindingElements = new BindingElementCollection(); // order of BindingElements is important // add context bindingElements.Add(context); // add session if (reliableSession.Enabled) bindingElements.Add(session); // add security (*optional) SecurityBindingElement wsSecurity = CreateMessageSecurity(); if (wsSecurity != null) bindingElements.Add(wsSecurity); // add encoding bindingElements.Add(encoding); // add transport security BindingElement transportSecurity = CreateTransportSecurity(); if (transportSecurity != null) { bindingElements.Add(transportSecurity); } // add transport (tcp) bindingElements.Add(transport); return bindingElements.Clone(); } internal static bool TryCreate(BindingElementCollection elements, out Binding binding) { binding = null; if (elements.Count > 6) return false; // collect all binding elements TcpTransportBindingElement transport = null; BinaryMessageEncodingBindingElement encoding = null; TransactionFlowBindingElement context = null; ReliableSessionBindingElement session = null; SecurityBindingElement wsSecurity = null; BindingElement transportSecurity = null; foreach (BindingElement element in elements) { if (element is SecurityBindingElement) wsSecurity = element as SecurityBindingElement; else if (element is TransportBindingElement) transport = element as TcpTransportBindingElement; else if (element is MessageEncodingBindingElement) encoding = element as BinaryMessageEncodingBindingElement; else if (element is TransactionFlowBindingElement) context = element as TransactionFlowBindingElement; else if (element is ReliableSessionBindingElement) session = element as ReliableSessionBindingElement; else { if (transportSecurity != null) return false; transportSecurity = element; } } if (transport == null) return false; if (encoding == null) return false; if (context == null) context = GetDefaultTransactionFlowBindingElement(); TcpTransportSecurity tcpTransportSecurity = new TcpTransportSecurity(); UnifiedSecurityMode mode = GetModeFromTransportSecurity(transportSecurity); NetTcpSecurity security; if (!TryCreateSecurity(wsSecurity, mode, session != null, transportSecurity, tcpTransportSecurity, out security)) return false; if (!SetTransportSecurity(transportSecurity, security.Mode, tcpTransportSecurity)) return false; NetTcpBinding netTcpBinding = new NetTcpBinding(transport, encoding, context, session, security); if (!netTcpBinding.IsBindingElementsMatch(transport, encoding, context, session)) return false; binding = netTcpBinding; return true; } BindingElement CreateTransportSecurity() { return this.security.CreateTransportSecurity(); } static UnifiedSecurityMode GetModeFromTransportSecurity(BindingElement transport) { return NetTcpSecurity.GetModeFromTransportSecurity(transport); } static bool SetTransportSecurity(BindingElement transport, SecurityMode mode, TcpTransportSecurity transportSecurity) { return NetTcpSecurity.SetTransportSecurity(transport, mode, transportSecurity); } SecurityBindingElement CreateMessageSecurity() { if (this.security.Mode == SecurityMode.Message || this.security.Mode == SecurityMode.TransportWithMessageCredential) { return this.security.CreateMessageSecurity(this.ReliableSession.Enabled); } else { return null; } } static bool TryCreateSecurity(SecurityBindingElement sbe, UnifiedSecurityMode mode, bool isReliableSession, BindingElement transportSecurity, TcpTransportSecurity tcpTransportSecurity, out NetTcpSecurity security) { if (sbe != null) mode &= UnifiedSecurityMode.Message | UnifiedSecurityMode.TransportWithMessageCredential; else mode &= ~(UnifiedSecurityMode.Message | UnifiedSecurityMode.TransportWithMessageCredential); SecurityMode securityMode = SecurityModeHelper.ToSecurityMode(mode); DiagnosticUtility.DebugAssert(SecurityModeHelper.IsDefined(securityMode), string.Format("Invalid SecurityMode value: {0}.", securityMode.ToString())); if (NetTcpSecurity.TryCreate(sbe, securityMode, isReliableSession, transportSecurity, tcpTransportSecurity, out security)) return true; return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
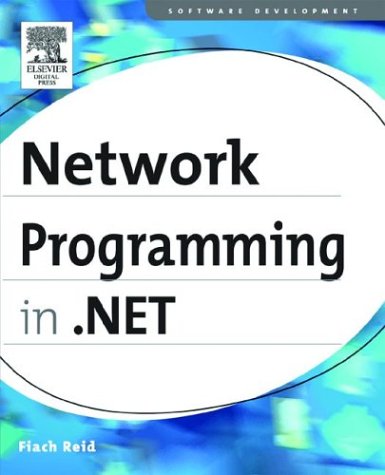
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlRootAttribute.cs
- PaginationProgressEventArgs.cs
- DataSourceHelper.cs
- ListItemViewControl.cs
- dsa.cs
- ColumnPropertiesGroup.cs
- ActivationServices.cs
- SystemWebSectionGroup.cs
- NativeRecognizer.cs
- HebrewCalendar.cs
- EventPrivateKey.cs
- ServiceManager.cs
- AppDomainInfo.cs
- DisplayNameAttribute.cs
- SpellerStatusTable.cs
- DurableEnlistmentState.cs
- PassportIdentity.cs
- SimpleWorkerRequest.cs
- SmtpException.cs
- MultiByteCodec.cs
- Process.cs
- VirtualPathProvider.cs
- TileModeValidation.cs
- SqlFormatter.cs
- ICollection.cs
- XmlSchemas.cs
- formatter.cs
- ClassData.cs
- EventLogTraceListener.cs
- ColumnReorderedEventArgs.cs
- HwndProxyElementProvider.cs
- Stylesheet.cs
- DictionaryBase.cs
- Matrix.cs
- SqlBulkCopyColumnMapping.cs
- SystemEvents.cs
- HtmlToClrEventProxy.cs
- BindingManagerDataErrorEventArgs.cs
- MetaType.cs
- Utils.cs
- GridViewCellAutomationPeer.cs
- InputProcessorProfilesLoader.cs
- ApplicationHost.cs
- ValidationErrorInfo.cs
- basecomparevalidator.cs
- VarRefManager.cs
- storepermission.cs
- XmlSerializerAssemblyAttribute.cs
- SQLString.cs
- RSAPKCS1KeyExchangeFormatter.cs
- RuleSettingsCollection.cs
- WindowsGraphicsWrapper.cs
- TraversalRequest.cs
- WindowsTab.cs
- SoapHelper.cs
- DataKeyCollection.cs
- TdsValueSetter.cs
- ChameleonKey.cs
- WindowsAuthenticationEventArgs.cs
- MatrixTransform.cs
- RenderData.cs
- PerformanceCounterTraceRecord.cs
- SubtreeProcessor.cs
- ADConnectionHelper.cs
- AutomationPatternInfo.cs
- WbmpConverter.cs
- EpmSyndicationContentSerializer.cs
- RuntimeCompatibilityAttribute.cs
- CustomWebEventKey.cs
- DesignerAutoFormat.cs
- MailWebEventProvider.cs
- ContextMenuStrip.cs
- DataControlLinkButton.cs
- FaultConverter.cs
- NativeMethods.cs
- CodeMemberField.cs
- CompensateDesigner.cs
- FileFormatException.cs
- SqlDataReaderSmi.cs
- DataTableReader.cs
- DataGridViewTextBoxColumn.cs
- FileDataSourceCache.cs
- ApplicationFileCodeDomTreeGenerator.cs
- EventLogEntryCollection.cs
- ObjectDataSourceSelectingEventArgs.cs
- BaseConfigurationRecord.cs
- MapPathBasedVirtualPathProvider.cs
- Reference.cs
- ContextDataSource.cs
- LeftCellWrapper.cs
- ResourcesChangeInfo.cs
- DataKey.cs
- Monitor.cs
- FrameworkElementFactory.cs
- DictionaryContent.cs
- TraversalRequest.cs
- UrlMapping.cs
- ConfigurationPermission.cs
- Scene3D.cs
- DataConnectionHelper.cs