Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Administration / AppDomainInfo.cs / 1 / AppDomainInfo.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Administration { using System; using System.Diagnostics; using System.Runtime.Serialization; internal sealed class AppDomainInfo { static object syncRoot = new object(); static AppDomainInfo singleton; Guid instanceId; string friendlyName; bool isDefaultAppDomain; string processName; string machineName; int processId; int id; AppDomainInfo(AppDomain appDomain) { // Assumption: Only one AppDomainInfo is created per AppDomain DiagnosticUtility.DebugAssert(null != appDomain, ""); this.instanceId = Guid.NewGuid(); this.friendlyName = appDomain.FriendlyName; this.isDefaultAppDomain = appDomain.IsDefaultAppDomain(); Process process = Process.GetCurrentProcess(); this.processName = process.ProcessName; this.machineName = Environment.MachineName; this.processId = process.Id; this.id = appDomain.Id; } public int Id { get { return this.id; } } public Guid InstanceId { get { return this.instanceId; } } public string MachineName { get { return this.machineName; } } public string Name { get { return this.friendlyName; } } public bool IsDefaultAppDomain { get { return this.isDefaultAppDomain; } } public int ProcessId { get { return this.processId; } } public string ProcessName { get { return this.processName; } } internal static AppDomainInfo Current { get { if (null == singleton) { lock (AppDomainInfo.syncRoot) { if (null == singleton) { singleton = new AppDomainInfo(AppDomain.CurrentDomain); } } } return singleton; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
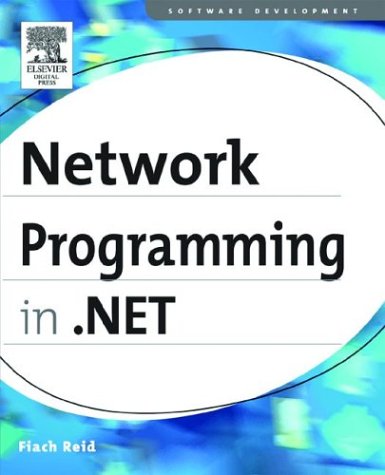
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CreatingCookieEventArgs.cs
- _UriSyntax.cs
- ProgressBar.cs
- RemoteX509Token.cs
- OleDbConnectionFactory.cs
- WorkflowWebHostingModule.cs
- TextParagraph.cs
- Array.cs
- MeshGeometry3D.cs
- Cursor.cs
- OperationFormatUse.cs
- ErrorWrapper.cs
- ClientSettingsSection.cs
- SynchronizationLockException.cs
- DbSourceParameterCollection.cs
- OdbcInfoMessageEvent.cs
- ManagementObject.cs
- TypedTableHandler.cs
- ParameterDataSourceExpression.cs
- _HeaderInfoTable.cs
- DataControlButton.cs
- ConfigPathUtility.cs
- Compiler.cs
- TemplateControlParser.cs
- RealizationContext.cs
- DynamicRendererThreadManager.cs
- SplayTreeNode.cs
- ControlTemplate.cs
- AliasExpr.cs
- NativeMethods.cs
- DataSpaceManager.cs
- DispatchWrapper.cs
- TransactionWaitAsyncResult.cs
- FlowDocumentReaderAutomationPeer.cs
- ExtentKey.cs
- WindowsAuthenticationEventArgs.cs
- recordstatescratchpad.cs
- StringDictionary.cs
- LinqDataSourceUpdateEventArgs.cs
- GeometryCollection.cs
- PcmConverter.cs
- ClockGroup.cs
- StdValidatorsAndConverters.cs
- String.cs
- DateTimeUtil.cs
- EmptyReadOnlyDictionaryInternal.cs
- HierarchicalDataSourceControl.cs
- EncoderNLS.cs
- PageFunction.cs
- OdbcFactory.cs
- designeractionbehavior.cs
- ListSortDescriptionCollection.cs
- MsmqTransportSecurityElement.cs
- GridToolTip.cs
- HostProtectionPermission.cs
- HwndSubclass.cs
- OptionUsage.cs
- TreeWalkHelper.cs
- RawStylusInputCustomData.cs
- CultureInfo.cs
- PhonemeEventArgs.cs
- SiteMapHierarchicalDataSourceView.cs
- WindowsToolbarAsMenu.cs
- TreeBuilderBamlTranslator.cs
- MenuItemStyle.cs
- TextHidden.cs
- Block.cs
- ValidatedMobileControlConverter.cs
- HttpRequestCacheValidator.cs
- ScrollItemProviderWrapper.cs
- DataRowExtensions.cs
- CollectionViewGroupInternal.cs
- WebServiceClientProxyGenerator.cs
- XmlSortKey.cs
- QuotedPairReader.cs
- ArrayTypeMismatchException.cs
- Tile.cs
- Events.cs
- BitmapSourceSafeMILHandle.cs
- IntranetCredentialPolicy.cs
- EntityDataSourceContextCreatedEventArgs.cs
- DataGridViewComboBoxCell.cs
- HtmlShimManager.cs
- FontDifferentiator.cs
- ISAPIRuntime.cs
- NameSpaceExtractor.cs
- LowerCaseStringConverter.cs
- SQLCharsStorage.cs
- CheckBoxAutomationPeer.cs
- PatternMatcher.cs
- FileNotFoundException.cs
- AssertSection.cs
- InvalidComObjectException.cs
- DetailsViewInsertEventArgs.cs
- ListenerServiceInstallComponent.cs
- ReadWriteObjectLock.cs
- DataGridHelper.cs
- CompilerState.cs
- SevenBitStream.cs
- ColumnMapVisitor.cs