Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / FontFace / FontDifferentiator.cs / 1305600 / FontDifferentiator.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontDifferentiator class handles parsing font family and face names // and adjusting stretch, weight and style values. // // History: // 11/10/2005 : mleonov - Started integration from a prototype application created by DBrown. // 1/21/2009 : [....] - removed unused code. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.Text; using System.Windows; using System.Windows.Markup; // for XmlLanguage namespace MS.Internal.FontFace { ////// FontDifferentiator class handles parsing font family and face names /// and adjusting stretch, weight and style values. /// internal static class FontDifferentiator { internal static IDictionaryConstructFaceNamesByStyleWeightStretch( FontStyle style, FontWeight weight, FontStretch stretch) { string faceName = BuildFaceName(style, weight, stretch); // Default comparer calls CultureInfo.Equals, which works for our purposes. Dictionary faceNames = new Dictionary (1); faceNames.Add(XmlLanguage.GetLanguage("en-us"), faceName); return faceNames; } private static string BuildFaceName( FontStyle fontStyle, FontWeight fontWeight, FontStretch fontStretch ) { string parsedStyleName = null; string parsedWeightName = null; string parsedStretchName = null; string regularFaceName = "Regular"; if (fontWeight != FontWeights.Normal) parsedWeightName = ((IFormattable)fontWeight).ToString(null, CultureInfo.InvariantCulture); if (fontStretch != FontStretches.Normal) parsedStretchName = ((IFormattable)fontStretch).ToString(null, CultureInfo.InvariantCulture); if (fontStyle != FontStyles.Normal) parsedStyleName = ((IFormattable)fontStyle).ToString(null, CultureInfo.InvariantCulture); // Build correct face string. // Set the initial capacity to be able to hold the word "Regular". StringBuilder faceNameBuilder = new StringBuilder(7); if (parsedStretchName != null) { faceNameBuilder.Append(parsedStretchName); } if (parsedWeightName != null) { if (faceNameBuilder.Length > 0) { faceNameBuilder.Append(" "); } faceNameBuilder.Append(parsedWeightName); } if (parsedStyleName != null) { if (faceNameBuilder.Length > 0) { faceNameBuilder.Append(" "); } faceNameBuilder.Append(parsedStyleName); } if (faceNameBuilder.Length == 0) { faceNameBuilder.Append(regularFaceName); } return faceNameBuilder.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontDifferentiator class handles parsing font family and face names // and adjusting stretch, weight and style values. // // History: // 11/10/2005 : mleonov - Started integration from a prototype application created by DBrown. // 1/21/2009 : [....] - removed unused code. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.Text; using System.Windows; using System.Windows.Markup; // for XmlLanguage namespace MS.Internal.FontFace { /// /// FontDifferentiator class handles parsing font family and face names /// and adjusting stretch, weight and style values. /// internal static class FontDifferentiator { internal static IDictionaryConstructFaceNamesByStyleWeightStretch( FontStyle style, FontWeight weight, FontStretch stretch) { string faceName = BuildFaceName(style, weight, stretch); // Default comparer calls CultureInfo.Equals, which works for our purposes. Dictionary faceNames = new Dictionary (1); faceNames.Add(XmlLanguage.GetLanguage("en-us"), faceName); return faceNames; } private static string BuildFaceName( FontStyle fontStyle, FontWeight fontWeight, FontStretch fontStretch ) { string parsedStyleName = null; string parsedWeightName = null; string parsedStretchName = null; string regularFaceName = "Regular"; if (fontWeight != FontWeights.Normal) parsedWeightName = ((IFormattable)fontWeight).ToString(null, CultureInfo.InvariantCulture); if (fontStretch != FontStretches.Normal) parsedStretchName = ((IFormattable)fontStretch).ToString(null, CultureInfo.InvariantCulture); if (fontStyle != FontStyles.Normal) parsedStyleName = ((IFormattable)fontStyle).ToString(null, CultureInfo.InvariantCulture); // Build correct face string. // Set the initial capacity to be able to hold the word "Regular". StringBuilder faceNameBuilder = new StringBuilder(7); if (parsedStretchName != null) { faceNameBuilder.Append(parsedStretchName); } if (parsedWeightName != null) { if (faceNameBuilder.Length > 0) { faceNameBuilder.Append(" "); } faceNameBuilder.Append(parsedWeightName); } if (parsedStyleName != null) { if (faceNameBuilder.Length > 0) { faceNameBuilder.Append(" "); } faceNameBuilder.Append(parsedStyleName); } if (faceNameBuilder.Length == 0) { faceNameBuilder.Append(regularFaceName); } return faceNameBuilder.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
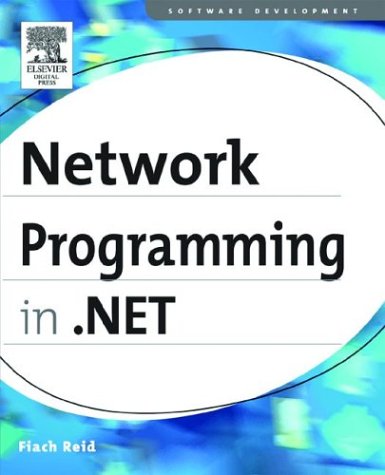
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SimpleFileLog.cs
- CodeNamespaceImport.cs
- JapaneseLunisolarCalendar.cs
- PartBasedPackageProperties.cs
- TableItemStyle.cs
- ClrPerspective.cs
- Hex.cs
- XmlValueConverter.cs
- FakeModelItemImpl.cs
- SoapTransportImporter.cs
- CodeArgumentReferenceExpression.cs
- SpeechDetectedEventArgs.cs
- OracleConnectionString.cs
- TypeSystemHelpers.cs
- EntitySetBaseCollection.cs
- BindableTemplateBuilder.cs
- CheckBox.cs
- DbConnectionOptions.cs
- WindowsAltTab.cs
- cache.cs
- ObfuscationAttribute.cs
- SimpleTextLine.cs
- WebRequestModulesSection.cs
- ToolStripScrollButton.cs
- StringComparer.cs
- DynamicRouteExpression.cs
- Token.cs
- TextTreeInsertElementUndoUnit.cs
- DebugView.cs
- RegistrationServices.cs
- DbSourceCommand.cs
- AutomationIdentifier.cs
- PropertyChangedEventArgs.cs
- IntranetCredentialPolicy.cs
- ImageCollectionCodeDomSerializer.cs
- MergeFilterQuery.cs
- ColumnWidthChangedEvent.cs
- ContextBase.cs
- DataServiceQuery.cs
- SQLInt16Storage.cs
- AssemblyCacheEntry.cs
- BoundField.cs
- SBCSCodePageEncoding.cs
- InlineObject.cs
- CharKeyFrameCollection.cs
- PropertyGridCommands.cs
- SerializationTrace.cs
- FixedFindEngine.cs
- DataControlField.cs
- SqlSelectClauseBuilder.cs
- CustomAssemblyResolver.cs
- ScriptReferenceBase.cs
- ComboBox.cs
- DrawingGroup.cs
- PrePostDescendentsWalker.cs
- FileIOPermission.cs
- ByteViewer.cs
- DataControlPagerLinkButton.cs
- Decoder.cs
- SafeProcessHandle.cs
- WhiteSpaceTrimStringConverter.cs
- TextPointer.cs
- SqlProvider.cs
- ScalarOps.cs
- ConfigurationElementCollection.cs
- UriPrefixTable.cs
- TextTreeObjectNode.cs
- RowToFieldTransformer.cs
- NamespaceInfo.cs
- Color.cs
- Properties.cs
- WebPartConnectionsConfigureVerb.cs
- ImageInfo.cs
- ProcessThreadCollection.cs
- RTLAwareMessageBox.cs
- DropDownList.cs
- ResourceType.cs
- RadioButton.cs
- _NtlmClient.cs
- FrameworkContentElementAutomationPeer.cs
- LockedAssemblyCache.cs
- MetadataSource.cs
- QueueProcessor.cs
- ParseNumbers.cs
- Calendar.cs
- PresentationTraceSources.cs
- BinaryOperationBinder.cs
- ExtendedPropertyCollection.cs
- ColorKeyFrameCollection.cs
- RadioButton.cs
- AssertUtility.cs
- DescendantOverDescendantQuery.cs
- NestPullup.cs
- Message.cs
- TextContainerChangedEventArgs.cs
- ArrangedElement.cs
- XmlSchemaObject.cs
- SettingsContext.cs
- RegexInterpreter.cs
- BlobPersonalizationState.cs