Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / WebControls / DropDownList.cs / 1 / DropDownList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Collections.Specialized; using System.Drawing; using System.Web; using System.Web.UI; using System.Web.UI.WebControls.Adapters; using System.Security.Permissions; ////// [ SupportsEventValidation, ValidationProperty("SelectedItem") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class DropDownList : ListControl, IPostBackDataHandler { ///Creates a control that allows the user to select a single item from a /// drop-down list. ////// public DropDownList() { } ///Initializes a new instance of the ///class. /// [ Browsable(false) ] public override Color BorderColor { get { return base.BorderColor; } set { base.BorderColor = value; } } ///[To be supplied.] ////// [ Browsable(false) ] public override BorderStyle BorderStyle { get { return base.BorderStyle; } set { base.BorderStyle = value; } } ///[To be supplied.] ////// [ Browsable(false) ] public override Unit BorderWidth { get { return base.BorderWidth; } set { base.BorderWidth = value; } } ///[To be supplied.] ////// [ WebCategory("Behavior"), DefaultValue(0), WebSysDescription(SR.WebControl_SelectedIndex), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override int SelectedIndex { get { int selectedIndex = base.SelectedIndex; if (selectedIndex < 0 && Items.Count > 0) { Items[0].Selected = true; selectedIndex = 0; } return selectedIndex; } set { base.SelectedIndex = value; } } internal override ArrayList SelectedIndicesInternal { get { int sideEffect = SelectedIndex; return base.SelectedIndicesInternal; } } protected override void AddAttributesToRender(HtmlTextWriter writer) { string uniqueID = UniqueID; if (uniqueID != null) { writer.AddAttribute(HtmlTextWriterAttribute.Name, uniqueID); } base.AddAttributesToRender(writer); } protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } ///Gets or sets the index of the item selected by the user /// from the ////// control. /// /// bool IPostBackDataHandler.LoadPostData(String postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } ///Process posted data for the ///control. /// /// protected virtual bool LoadPostData(String postDataKey, NameValueCollection postCollection) { // When a DropDownList is disabled, then there is no postback data for it. // Since DropDownList doesn't call RegisterRequiresPostBack, this method will // never be called, so we don't need to worry about ignoring empty postback data. string [] selectedItems = postCollection.GetValues(postDataKey); EnsureDataBound(); if (selectedItems != null) { ValidateEvent(postDataKey, selectedItems[0]); int n = Items.FindByValueInternal(selectedItems[0], false); if (SelectedIndex != n) { SetPostDataSelection(n); return true; } } return false; } ///Process posted data for the ///control. /// /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ///Raises events for the ///control on post back. /// /// protected virtual void RaisePostDataChangedEvent() { if (AutoPostBack && !Page.IsPostBackEventControlRegistered) { // VSWhidbey 204824 Page.AutoPostBackControl = this; if (CausesValidation) { Page.Validate(ValidationGroup); } } OnSelectedIndexChanged(EventArgs.Empty); } protected internal override void VerifyMultiSelect() { throw new HttpException(SR.GetString(SR.Cant_Multiselect, "DropDownList")); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Raises events for the ///control on post back. // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Collections.Specialized; using System.Drawing; using System.Web; using System.Web.UI; using System.Web.UI.WebControls.Adapters; using System.Security.Permissions; ////// [ SupportsEventValidation, ValidationProperty("SelectedItem") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class DropDownList : ListControl, IPostBackDataHandler { ///Creates a control that allows the user to select a single item from a /// drop-down list. ////// public DropDownList() { } ///Initializes a new instance of the ///class. /// [ Browsable(false) ] public override Color BorderColor { get { return base.BorderColor; } set { base.BorderColor = value; } } ///[To be supplied.] ////// [ Browsable(false) ] public override BorderStyle BorderStyle { get { return base.BorderStyle; } set { base.BorderStyle = value; } } ///[To be supplied.] ////// [ Browsable(false) ] public override Unit BorderWidth { get { return base.BorderWidth; } set { base.BorderWidth = value; } } ///[To be supplied.] ////// [ WebCategory("Behavior"), DefaultValue(0), WebSysDescription(SR.WebControl_SelectedIndex), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override int SelectedIndex { get { int selectedIndex = base.SelectedIndex; if (selectedIndex < 0 && Items.Count > 0) { Items[0].Selected = true; selectedIndex = 0; } return selectedIndex; } set { base.SelectedIndex = value; } } internal override ArrayList SelectedIndicesInternal { get { int sideEffect = SelectedIndex; return base.SelectedIndicesInternal; } } protected override void AddAttributesToRender(HtmlTextWriter writer) { string uniqueID = UniqueID; if (uniqueID != null) { writer.AddAttribute(HtmlTextWriterAttribute.Name, uniqueID); } base.AddAttributesToRender(writer); } protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } ///Gets or sets the index of the item selected by the user /// from the ////// control. /// /// bool IPostBackDataHandler.LoadPostData(String postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } ///Process posted data for the ///control. /// /// protected virtual bool LoadPostData(String postDataKey, NameValueCollection postCollection) { // When a DropDownList is disabled, then there is no postback data for it. // Since DropDownList doesn't call RegisterRequiresPostBack, this method will // never be called, so we don't need to worry about ignoring empty postback data. string [] selectedItems = postCollection.GetValues(postDataKey); EnsureDataBound(); if (selectedItems != null) { ValidateEvent(postDataKey, selectedItems[0]); int n = Items.FindByValueInternal(selectedItems[0], false); if (SelectedIndex != n) { SetPostDataSelection(n); return true; } } return false; } ///Process posted data for the ///control. /// /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ///Raises events for the ///control on post back. /// /// protected virtual void RaisePostDataChangedEvent() { if (AutoPostBack && !Page.IsPostBackEventControlRegistered) { // VSWhidbey 204824 Page.AutoPostBackControl = this; if (CausesValidation) { Page.Validate(ValidationGroup); } } OnSelectedIndexChanged(EventArgs.Empty); } protected internal override void VerifyMultiSelect() { throw new HttpException(SR.GetString(SR.Cant_Multiselect, "DropDownList")); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Raises events for the ///control on post back.
Link Menu
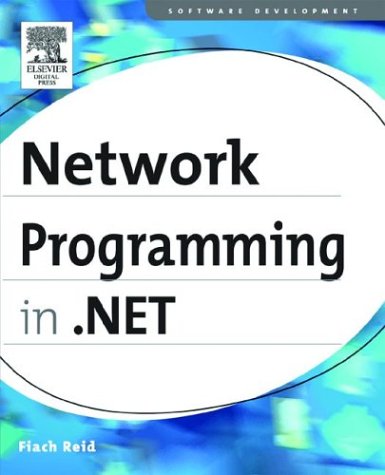
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewCellMouseEventArgs.cs
- BinaryCommonClasses.cs
- EventDescriptor.cs
- DialogWindow.cs
- MulticastIPAddressInformationCollection.cs
- sapiproxy.cs
- PickDesigner.xaml.cs
- BamlTreeNode.cs
- namescope.cs
- StringBuilder.cs
- StylusEditingBehavior.cs
- GridEntry.cs
- Literal.cs
- Oid.cs
- PeerPresenceInfo.cs
- TransportElement.cs
- Pair.cs
- MimeImporter.cs
- DataViewManagerListItemTypeDescriptor.cs
- Executor.cs
- BinarySerializer.cs
- SafeNativeMethodsOther.cs
- ContextQuery.cs
- CodeDelegateInvokeExpression.cs
- ConfigXmlAttribute.cs
- GradientStop.cs
- ProfilePropertySettings.cs
- SerialStream.cs
- XmlWhitespace.cs
- Win32Exception.cs
- X509CertificateStore.cs
- safesecurityhelperavalon.cs
- NavigationPropertyEmitter.cs
- MessageQueuePermissionEntryCollection.cs
- StrokeCollection.cs
- XsdBuilder.cs
- XMLSchema.cs
- CmsInterop.cs
- ColumnHeaderConverter.cs
- GZipDecoder.cs
- XmlQualifiedName.cs
- Operators.cs
- GradientStop.cs
- NameSpaceExtractor.cs
- OleDbConnection.cs
- FixedSOMFixedBlock.cs
- WpfMemberInvoker.cs
- QueryComponents.cs
- WebPartTransformerAttribute.cs
- Queue.cs
- IdnMapping.cs
- BaseTemplateCodeDomTreeGenerator.cs
- UserValidatedEventArgs.cs
- InvalidProgramException.cs
- ResXBuildProvider.cs
- HuffCodec.cs
- WindowsGraphicsCacheManager.cs
- login.cs
- SmiRequestExecutor.cs
- DesignerContextDescriptor.cs
- MsmqChannelFactory.cs
- C14NUtil.cs
- StylusPointDescription.cs
- PointLight.cs
- ScaleTransform.cs
- QueryCursorEventArgs.cs
- LayoutEditorPart.cs
- Point.cs
- NetworkInterface.cs
- TrustSection.cs
- CopyAttributesAction.cs
- XmlSchemaChoice.cs
- HttpResponse.cs
- SchemaImporterExtensionElement.cs
- XmlAttributeOverrides.cs
- IdentitySection.cs
- FirstQueryOperator.cs
- SiteMapProvider.cs
- LocalizableResourceBuilder.cs
- TcpTransportSecurity.cs
- XmlSerializerAssemblyAttribute.cs
- StateRuntime.cs
- AttributeTable.cs
- CfgRule.cs
- PropertyValidationContext.cs
- RoutedEventHandlerInfo.cs
- MatrixTransform.cs
- ServiceHttpModule.cs
- DynamicRenderer.cs
- DigestTraceRecordHelper.cs
- DynamicQueryableWrapper.cs
- IPEndPoint.cs
- ControlCachePolicy.cs
- QuaternionRotation3D.cs
- TypeSchema.cs
- AssemblyInfo.cs
- WebPartZoneDesigner.cs
- Menu.cs
- DropAnimation.xaml.cs
- DesignerDataSourceView.cs