Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / Method.cs / 1305376 / Method.cs
using System; using System.Runtime.InteropServices; using WbemClient_v1; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ////// ///Contains information about a WMI method. ////// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// public class MethodData { private ManagementObject parent; //needed to be able to get method qualifiers private string methodName; private IWbemClassObjectFreeThreaded wmiInParams; private IWbemClassObjectFreeThreaded wmiOutParams; private QualifierDataCollection qualifiers; internal MethodData(ManagementObject parent, string methodName) { this.parent = parent; this.methodName = methodName; RefreshMethodInfo(); qualifiers = null; } //This private function is used to refresh the information from the Wmi object before returning the requested data private void RefreshMethodInfo() { int status = (int)ManagementStatus.Failed; try { status = parent.wbemObject.GetMethod_(methodName, 0, out wmiInParams, out wmiOutParams); } catch (COMException e) { ManagementException.ThrowWithExtendedInfo(e); } if ((status & 0xfffff000) == 0x80041000) { ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); } else if ((status & 0x80000000) != 0) { Marshal.ThrowExceptionForHR(status); } } ///using System; /// using System.Management; /// /// // This example shows how to obtain meta data /// // about a WMI method with a given name in a given WMI class /// /// class Sample_MethodData /// { /// public static int Main(string[] args) { /// /// // Get the "SetPowerState" method in the Win32_LogicalDisk class /// ManagementClass diskClass = new ManagementClass("win32_logicaldisk"); /// MethodData m = diskClass.Methods["SetPowerState"]; /// /// // Get method name (albeit we already know it) /// Console.WriteLine("Name: " + m.Name); /// /// // Get the name of the top-most class where this specific method was defined /// Console.WriteLine("Origin: " + m.Origin); /// /// // List names and types of input parameters /// ManagementBaseObject inParams = m.InParameters; /// foreach(PropertyData pdata in inParams.Properties) { /// Console.WriteLine(); /// Console.WriteLine("InParam_Name: " + pdata.Name); /// Console.WriteLine("InParam_Type: " + pdata.Type); /// } /// /// // List names and types of output parameters /// ManagementBaseObject outParams = m.OutParameters; /// foreach(PropertyData pdata in outParams.Properties) { /// Console.WriteLine(); /// Console.WriteLine("OutParam_Name: " + pdata.Name); /// Console.WriteLine("OutParam_Type: " + pdata.Type); /// } /// /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This example shows how to obtain meta data /// ' about a WMI method with a given name in a given WMI class /// /// Class Sample_ManagementClass /// Overloads Public Shared Function Main(args() As String) As Integer /// /// ' Get the "SetPowerState" method in the Win32_LogicalDisk class /// Dim diskClass As New ManagementClass("Win32_LogicalDisk") /// Dim m As MethodData = diskClass.Methods("SetPowerState") /// /// ' Get method name (albeit we already know it) /// Console.WriteLine("Name: " & m.Name) /// /// ' Get the name of the top-most class where /// ' this specific method was defined /// Console.WriteLine("Origin: " & m.Origin) /// /// ' List names and types of input parameters /// Dim inParams As ManagementBaseObject /// inParams = m.InParameters /// Dim pdata As PropertyData /// For Each pdata In inParams.Properties /// Console.WriteLine() /// Console.WriteLine("InParam_Name: " & pdata.Name) /// Console.WriteLine("InParam_Type: " & pdata.Type) /// Next pdata /// /// ' List names and types of output parameters /// Dim outParams As ManagementBaseObject /// outParams = m.OutParameters /// For Each pdata in outParams.Properties /// Console.WriteLine() /// Console.WriteLine("OutParam_Name: " & pdata.Name) /// Console.WriteLine("OutParam_Type: " & pdata.Type) /// Next pdata /// /// Return 0 /// End Function /// End Class ///
////// ///Gets or sets the name of the method. ////// public string Name { get { return methodName != null ? methodName : ""; } } ///The name of the method. ////// ///Gets or sets the input parameters to the method. Each /// parameter is described as a property in the object. If a parameter is both in /// and out, it appears in both the ///and /// properties. /// ////// A ////// containing all the input parameters to the /// method. /// public ManagementBaseObject InParameters { get { RefreshMethodInfo(); return (null == wmiInParams) ? null : new ManagementBaseObject(wmiInParams); } } ///Each parameter in the object should have an /// ////// qualifier, identifying the order of the parameters in the method call. /// ///Gets or sets the output parameters to the method. Each /// parameter is described as a property in the object. If a parameter is both in /// and out, it will appear in both the ///and /// properties. /// ///A ///containing all the output parameters to the method. /// public ManagementBaseObject OutParameters { get { RefreshMethodInfo(); return (null == wmiOutParams) ? null : new ManagementBaseObject(wmiOutParams); } } ///Each parameter in this object should have an /// ///qualifier to identify the /// order of the parameters in the method call. The ReturnValue property is a special property of /// the ////// object and /// holds the return value of the method. /// ///Gets the name of the management class in which the method was first /// introduced in the class inheritance hierarchy. ////// A string representing the originating /// management class name. /// public string Origin { get { string className = null; int status = parent.wbemObject.GetMethodOrigin_(methodName, out className); if (status < 0) { if (status == (int)tag_WBEMSTATUS.WBEM_E_INVALID_OBJECT) className = String.Empty; // Interpret as an unspecified property - return "" else if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return className; } } ////// ///Gets a collection of qualifiers defined in the /// method. Each element is of type ////// and contains information such as the qualifier name, value, and /// flavor. /// A ///containing the /// qualifiers for this method. /// public QualifierDataCollection Qualifiers { get { if (qualifiers == null) qualifiers = new QualifierDataCollection(parent, methodName, QualifierType.MethodQualifier); return qualifiers; } } }//MethodData } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
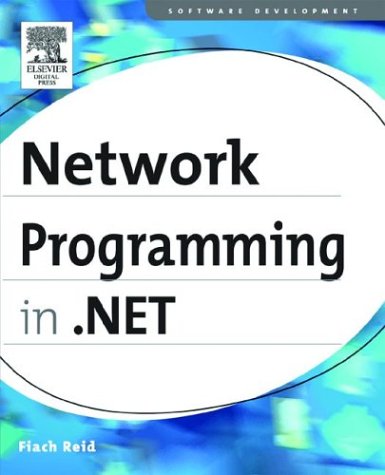
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedSOMFixedBlock.cs
- XPathException.cs
- ProfileGroupSettings.cs
- CoreSwitches.cs
- ValidatorCompatibilityHelper.cs
- EventLogLink.cs
- ToolboxItemAttribute.cs
- FontStretchConverter.cs
- MobileComponentEditorPage.cs
- MenuEventArgs.cs
- ServicePointManager.cs
- Calendar.cs
- ValidationRule.cs
- smtppermission.cs
- CompensateDesigner.cs
- FixedTextContainer.cs
- FontCacheLogic.cs
- GlyphRunDrawing.cs
- Path.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- Add.cs
- CommonObjectSecurity.cs
- ConfigsHelper.cs
- AuthenticationSection.cs
- BoolExpressionVisitors.cs
- UInt32.cs
- QuotedPrintableStream.cs
- DiagnosticsConfiguration.cs
- DesignerForm.cs
- ToolStripControlHost.cs
- Nullable.cs
- TimeManager.cs
- SystemWebExtensionsSectionGroup.cs
- DefaultValueConverter.cs
- StandardBindingElement.cs
- OutputCacheProfileCollection.cs
- ToolStripItem.cs
- ReturnType.cs
- SwitchLevelAttribute.cs
- Triplet.cs
- Boolean.cs
- PixelShader.cs
- OutputChannelBinder.cs
- DivideByZeroException.cs
- UriExt.cs
- KeyGesture.cs
- XmlCharCheckingWriter.cs
- GestureRecognizer.cs
- Rules.cs
- RegexGroupCollection.cs
- RsaSecurityTokenAuthenticator.cs
- XmlSchemaExternal.cs
- WebPartMinimizeVerb.cs
- ObjectItemAttributeAssemblyLoader.cs
- XmlWrappingReader.cs
- TableProviderWrapper.cs
- DetailsViewUpdatedEventArgs.cs
- SizeF.cs
- ToolStripItemGlyph.cs
- PropertyStore.cs
- KeyGestureConverter.cs
- EntityProviderServices.cs
- ForeignConstraint.cs
- ImportContext.cs
- AssemblyAttributesGoHere.cs
- CTreeGenerator.cs
- TextTreeInsertElementUndoUnit.cs
- NonBatchDirectoryCompiler.cs
- ExtractorMetadata.cs
- Floater.cs
- CleanUpVirtualizedItemEventArgs.cs
- DataViewListener.cs
- IntSecurity.cs
- DriveInfo.cs
- Pair.cs
- LayoutInformation.cs
- hresults.cs
- XmlAttributeProperties.cs
- StandardBindingOptionalReliableSessionElement.cs
- XmlSchemaAttributeGroupRef.cs
- OdbcConnection.cs
- PersonalizationAdministration.cs
- ThumbButtonInfoCollection.cs
- Pkcs7Recipient.cs
- SortKey.cs
- SqlException.cs
- MenuBindingsEditorForm.cs
- ServiceRouteHandler.cs
- Accessible.cs
- ellipse.cs
- SqlDataSourceCache.cs
- TextDpi.cs
- ObjectSet.cs
- SqlFormatter.cs
- NavigationCommands.cs
- DelegatedStream.cs
- ExtenderProviderService.cs
- AtlasWeb.Designer.cs
- recordstatefactory.cs
- UInt16Storage.cs