Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / System.ServiceModel.Activation / System / ServiceModel / Activation / ServiceRouteHandler.cs / 1305376 / ServiceRouteHandler.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Linq; using System.Runtime; using System.ServiceModel; using System.ServiceModel.Activation; using System.ServiceModel.Diagnostics; using System.ServiceModel.Diagnostics.Application; using System.Web; using System.Web.Hosting; using System.Web.Routing; class ServiceRouteHandler : IRouteHandler { string baseAddress; IHttpHandler handler; object locker = new object(); [Fx.Tag.Cache( typeof(ServiceDeploymentInfo), Fx.Tag.CacheAttrition.None, Scope = "instance of declaring class", SizeLimit = "unbounded", Timeout = "infinite" )] static Hashtable routeServiceTable = new Hashtable(StringComparer.CurrentCultureIgnoreCase); public ServiceRouteHandler(string baseAddress, ServiceHostFactoryBase serviceHostFactory, Type webServiceType) { this.baseAddress = string.Format(CultureInfo.CurrentCulture, "~/{0}", baseAddress); if (webServiceType == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("webServiceType")); } if (serviceHostFactory == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("serviceHostFactory")); } string serviceType = webServiceType.AssemblyQualifiedName; AddServiceInfo(this.baseAddress, new ServiceDeploymentInfo(this.baseAddress, serviceHostFactory, serviceType)); } public IHttpHandler GetHttpHandler(RequestContext requestContext) { // we create httphandler only we the request map to the corresponding route. // we thus do not need to check whether the baseAddress has been added // even though Asp.Net allows duplicated routes but it picks the first match if (handler == null) { // use local lock to prevent multiple httphanders from being created lock (locker) { if (handler == null) { handler = new AspNetRouteServiceHttpHandler(this.baseAddress); MarkRouteAsActive(this.baseAddress); } } } return handler; } static void AddServiceInfo(string virtualPath, ServiceDeploymentInfo serviceInfo) { Fx.Assert(!string.IsNullOrEmpty(virtualPath), "virtualPath should not be empty or null"); Fx.Assert(serviceInfo != null, "serviceInfo should not be null"); // We cannot support dulicated route routes even Asp.Net route allows it try { routeServiceTable.Add(virtualPath, serviceInfo); } catch (ArgumentException) { throw FxTrace.Exception.Argument("virtualPath", SR.Hosting_RouteHasAlreadyBeenAdded(virtualPath)); } } public static ServiceDeploymentInfo GetServiceInfo(string normalizedVirtualPath) { return (ServiceDeploymentInfo)routeServiceTable[normalizedVirtualPath]; } public static bool IsActiveAspNetRoute(string virtualPath) { bool isRouteService = false; if (!string.IsNullOrEmpty(virtualPath)) { ServiceDeploymentInfo serviceInfo = (ServiceDeploymentInfo)routeServiceTable[virtualPath]; if (serviceInfo != null) { isRouteService = serviceInfo.MessageHandledByRoute; } } return isRouteService; } // A route in routetable does not always mean the route will be picked // we update IsRouteService only when Asp.Net picks this route public static void MarkRouteAsActive(string normalizedVirtualPath) { ServiceDeploymentInfo serviceInfo = (ServiceDeploymentInfo)routeServiceTable[normalizedVirtualPath]; if (serviceInfo != null) { serviceInfo.MessageHandledByRoute = true; } } // a route should be marked as inactive in the case that CBA should be used public static void MarkARouteAsInactive(string normalizedVirtualPath) { ServiceDeploymentInfo serviceInfo = (ServiceDeploymentInfo)routeServiceTable[normalizedVirtualPath]; if (serviceInfo != null) { serviceInfo.MessageHandledByRoute = false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Linq; using System.Runtime; using System.ServiceModel; using System.ServiceModel.Activation; using System.ServiceModel.Diagnostics; using System.ServiceModel.Diagnostics.Application; using System.Web; using System.Web.Hosting; using System.Web.Routing; class ServiceRouteHandler : IRouteHandler { string baseAddress; IHttpHandler handler; object locker = new object(); [Fx.Tag.Cache( typeof(ServiceDeploymentInfo), Fx.Tag.CacheAttrition.None, Scope = "instance of declaring class", SizeLimit = "unbounded", Timeout = "infinite" )] static Hashtable routeServiceTable = new Hashtable(StringComparer.CurrentCultureIgnoreCase); public ServiceRouteHandler(string baseAddress, ServiceHostFactoryBase serviceHostFactory, Type webServiceType) { this.baseAddress = string.Format(CultureInfo.CurrentCulture, "~/{0}", baseAddress); if (webServiceType == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("webServiceType")); } if (serviceHostFactory == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("serviceHostFactory")); } string serviceType = webServiceType.AssemblyQualifiedName; AddServiceInfo(this.baseAddress, new ServiceDeploymentInfo(this.baseAddress, serviceHostFactory, serviceType)); } public IHttpHandler GetHttpHandler(RequestContext requestContext) { // we create httphandler only we the request map to the corresponding route. // we thus do not need to check whether the baseAddress has been added // even though Asp.Net allows duplicated routes but it picks the first match if (handler == null) { // use local lock to prevent multiple httphanders from being created lock (locker) { if (handler == null) { handler = new AspNetRouteServiceHttpHandler(this.baseAddress); MarkRouteAsActive(this.baseAddress); } } } return handler; } static void AddServiceInfo(string virtualPath, ServiceDeploymentInfo serviceInfo) { Fx.Assert(!string.IsNullOrEmpty(virtualPath), "virtualPath should not be empty or null"); Fx.Assert(serviceInfo != null, "serviceInfo should not be null"); // We cannot support dulicated route routes even Asp.Net route allows it try { routeServiceTable.Add(virtualPath, serviceInfo); } catch (ArgumentException) { throw FxTrace.Exception.Argument("virtualPath", SR.Hosting_RouteHasAlreadyBeenAdded(virtualPath)); } } public static ServiceDeploymentInfo GetServiceInfo(string normalizedVirtualPath) { return (ServiceDeploymentInfo)routeServiceTable[normalizedVirtualPath]; } public static bool IsActiveAspNetRoute(string virtualPath) { bool isRouteService = false; if (!string.IsNullOrEmpty(virtualPath)) { ServiceDeploymentInfo serviceInfo = (ServiceDeploymentInfo)routeServiceTable[virtualPath]; if (serviceInfo != null) { isRouteService = serviceInfo.MessageHandledByRoute; } } return isRouteService; } // A route in routetable does not always mean the route will be picked // we update IsRouteService only when Asp.Net picks this route public static void MarkRouteAsActive(string normalizedVirtualPath) { ServiceDeploymentInfo serviceInfo = (ServiceDeploymentInfo)routeServiceTable[normalizedVirtualPath]; if (serviceInfo != null) { serviceInfo.MessageHandledByRoute = true; } } // a route should be marked as inactive in the case that CBA should be used public static void MarkARouteAsInactive(string normalizedVirtualPath) { ServiceDeploymentInfo serviceInfo = (ServiceDeploymentInfo)routeServiceTable[normalizedVirtualPath]; if (serviceInfo != null) { serviceInfo.MessageHandledByRoute = false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
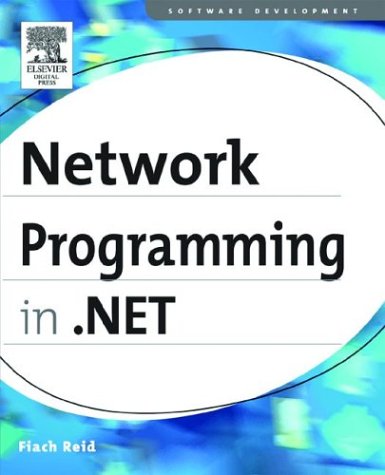
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormsAuthenticationUser.cs
- ConfigurationManagerInternalFactory.cs
- DependencyPropertyChangedEventArgs.cs
- ToolStripHighContrastRenderer.cs
- WaitHandleCannotBeOpenedException.cs
- UserControl.cs
- ConnectionManagementElement.cs
- Graph.cs
- Transform3DCollection.cs
- EdmError.cs
- ButtonChrome.cs
- LambdaCompiler.Generated.cs
- CompModSwitches.cs
- ExpandCollapseProviderWrapper.cs
- IfJoinedCondition.cs
- NodeLabelEditEvent.cs
- XmlMembersMapping.cs
- DragStartedEventArgs.cs
- CipherData.cs
- AndMessageFilter.cs
- LinkGrep.cs
- WorkItem.cs
- Size.cs
- _LocalDataStoreMgr.cs
- DataGridAutomationPeer.cs
- BackEase.cs
- TextTreeRootNode.cs
- WebPartChrome.cs
- DataControlPagerLinkButton.cs
- SizeLimitedCache.cs
- SimpleFieldTemplateUserControl.cs
- PolicyUnit.cs
- BufferedWebEventProvider.cs
- SiteMapNode.cs
- OptimizerPatterns.cs
- ContentElementAutomationPeer.cs
- SrgsItemList.cs
- DataGridViewRowPrePaintEventArgs.cs
- DefaultValueAttribute.cs
- LingerOption.cs
- DetailsViewRowCollection.cs
- ScriptReferenceBase.cs
- XmlNodeList.cs
- TemplateControl.cs
- KeySpline.cs
- OAVariantLib.cs
- ListenDesigner.cs
- ManipulationDevice.cs
- ItemContainerPattern.cs
- TemplateInstanceAttribute.cs
- OptimisticConcurrencyException.cs
- ComponentEditorPage.cs
- ListView.cs
- GregorianCalendar.cs
- TypeHelpers.cs
- GatewayIPAddressInformationCollection.cs
- TableProviderWrapper.cs
- ToolStripPanel.cs
- IteratorDescriptor.cs
- TableAdapterManagerNameHandler.cs
- Executor.cs
- ShaderRenderModeValidation.cs
- InvalidOleVariantTypeException.cs
- Bezier.cs
- CleanUpVirtualizedItemEventArgs.cs
- TextUtf8RawTextWriter.cs
- DiscriminatorMap.cs
- MessageBox.cs
- contentDescriptor.cs
- XslCompiledTransform.cs
- LinearKeyFrames.cs
- DateTimeParse.cs
- DataGridColumnCollectionEditor.cs
- Function.cs
- DocumentAutomationPeer.cs
- CodeDomComponentSerializationService.cs
- SiteMapNode.cs
- PrintPageEvent.cs
- NativeMethods.cs
- TabControlEvent.cs
- DrawItemEvent.cs
- ProfileServiceManager.cs
- RelatedView.cs
- ChtmlCommandAdapter.cs
- DataSourceCacheDurationConverter.cs
- InputLangChangeRequestEvent.cs
- SessionStateItemCollection.cs
- CompilationRelaxations.cs
- AttachedAnnotationChangedEventArgs.cs
- GridViewSelectEventArgs.cs
- NativeMethods.cs
- Profiler.cs
- SimpleMailWebEventProvider.cs
- Geometry.cs
- TraversalRequest.cs
- LinqDataView.cs
- SmiEventSink.cs
- EventLogPermissionEntry.cs
- EntitySqlException.cs
- EasingKeyFrames.cs