Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewRowPrePaintEventArgs.cs / 1 / DataGridViewRowPrePaintEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.ComponentModel; using System.Diagnostics; ///public class DataGridViewRowPrePaintEventArgs : HandledEventArgs { private DataGridView dataGridView; private Graphics graphics; private Rectangle clipBounds; private Rectangle rowBounds; private DataGridViewCellStyle inheritedRowStyle; private int rowIndex; private DataGridViewElementStates rowState; private string errorText; private bool isFirstDisplayedRow; private bool isLastVisibleRow; private DataGridViewPaintParts paintParts; /// public DataGridViewRowPrePaintEventArgs(DataGridView dataGridView, Graphics graphics, Rectangle clipBounds, Rectangle rowBounds, int rowIndex, DataGridViewElementStates rowState, string errorText, DataGridViewCellStyle inheritedRowStyle, bool isFirstDisplayedRow, bool isLastVisibleRow) { if (dataGridView == null) { throw new ArgumentNullException("dataGridView"); } if (graphics == null) { throw new ArgumentNullException("graphics"); } if (inheritedRowStyle == null) { throw new ArgumentNullException("inheritedRowStyle"); } this.dataGridView = dataGridView; this.graphics = graphics; this.clipBounds = clipBounds; this.rowBounds = rowBounds; this.rowIndex = rowIndex; this.rowState = rowState; this.errorText = errorText; this.inheritedRowStyle = inheritedRowStyle; this.isFirstDisplayedRow = isFirstDisplayedRow; this.isLastVisibleRow = isLastVisibleRow; this.paintParts = DataGridViewPaintParts.All; } internal DataGridViewRowPrePaintEventArgs(DataGridView dataGridView) { Debug.Assert(dataGridView != null); this.dataGridView = dataGridView; } /// public Rectangle ClipBounds { get { return this.clipBounds; } set { this.clipBounds = value; } } /// public string ErrorText { get { return this.errorText; } } /// public Graphics Graphics { get { return this.graphics; } } /// public DataGridViewCellStyle InheritedRowStyle { get { return this.inheritedRowStyle; } } /// public bool IsFirstDisplayedRow { get { return this.isFirstDisplayedRow; } } /// public bool IsLastVisibleRow { get { return this.isLastVisibleRow; } } /// public DataGridViewPaintParts PaintParts { get { return this.paintParts; } set { if ((value & ~DataGridViewPaintParts.All) != 0) { throw new ArgumentException(SR.GetString(SR.DataGridView_InvalidDataGridViewPaintPartsCombination, "value")); } this.paintParts = value; } } /// public Rectangle RowBounds { get { return this.rowBounds; } } /// public int RowIndex { get { return this.rowIndex; } } /// public DataGridViewElementStates State { get { return this.rowState; } } /// public void DrawFocus(Rectangle bounds, bool cellsPaintSelectionBackground) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(this.rowIndex).DrawFocus(this.graphics, this.clipBounds, bounds, this.rowIndex, this.rowState, this.inheritedRowStyle, cellsPaintSelectionBackground); } /// public void PaintCells(Rectangle clipBounds, DataGridViewPaintParts paintParts) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(this.rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } /// public void PaintCellsBackground(Rectangle clipBounds, bool cellsPaintSelectionBackground) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } DataGridViewPaintParts paintParts = DataGridViewPaintParts.Background | DataGridViewPaintParts.Border; if (cellsPaintSelectionBackground) { paintParts |= DataGridViewPaintParts.SelectionBackground; } this.dataGridView.Rows.SharedRow(this.rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } /// public void PaintCellsContent(Rectangle clipBounds) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(this.rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, DataGridViewPaintParts.ContentBackground | DataGridViewPaintParts.ContentForeground | DataGridViewPaintParts.ErrorIcon); } /// public void PaintHeader(bool paintSelectionBackground) { DataGridViewPaintParts paintParts = DataGridViewPaintParts.Background | DataGridViewPaintParts.Border | DataGridViewPaintParts.ContentBackground | DataGridViewPaintParts.ContentForeground | DataGridViewPaintParts.ErrorIcon; if (paintSelectionBackground) { paintParts |= DataGridViewPaintParts.SelectionBackground; } PaintHeader(paintParts); } /// public void PaintHeader(DataGridViewPaintParts paintParts) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(this.rowIndex).PaintHeader(this.graphics, this.clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } internal void SetProperties(Graphics graphics, Rectangle clipBounds, Rectangle rowBounds, int rowIndex, DataGridViewElementStates rowState, string errorText, DataGridViewCellStyle inheritedRowStyle, bool isFirstDisplayedRow, bool isLastVisibleRow) { Debug.Assert(graphics != null); Debug.Assert(inheritedRowStyle != null); this.graphics = graphics; this.clipBounds = clipBounds; this.rowBounds = rowBounds; this.rowIndex = rowIndex; this.rowState = rowState; this.errorText = errorText; this.inheritedRowStyle = inheritedRowStyle; this.isFirstDisplayedRow = isFirstDisplayedRow; this.isLastVisibleRow = isLastVisibleRow; this.paintParts = DataGridViewPaintParts.All; this.Handled = false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.ComponentModel; using System.Diagnostics; ///public class DataGridViewRowPrePaintEventArgs : HandledEventArgs { private DataGridView dataGridView; private Graphics graphics; private Rectangle clipBounds; private Rectangle rowBounds; private DataGridViewCellStyle inheritedRowStyle; private int rowIndex; private DataGridViewElementStates rowState; private string errorText; private bool isFirstDisplayedRow; private bool isLastVisibleRow; private DataGridViewPaintParts paintParts; /// public DataGridViewRowPrePaintEventArgs(DataGridView dataGridView, Graphics graphics, Rectangle clipBounds, Rectangle rowBounds, int rowIndex, DataGridViewElementStates rowState, string errorText, DataGridViewCellStyle inheritedRowStyle, bool isFirstDisplayedRow, bool isLastVisibleRow) { if (dataGridView == null) { throw new ArgumentNullException("dataGridView"); } if (graphics == null) { throw new ArgumentNullException("graphics"); } if (inheritedRowStyle == null) { throw new ArgumentNullException("inheritedRowStyle"); } this.dataGridView = dataGridView; this.graphics = graphics; this.clipBounds = clipBounds; this.rowBounds = rowBounds; this.rowIndex = rowIndex; this.rowState = rowState; this.errorText = errorText; this.inheritedRowStyle = inheritedRowStyle; this.isFirstDisplayedRow = isFirstDisplayedRow; this.isLastVisibleRow = isLastVisibleRow; this.paintParts = DataGridViewPaintParts.All; } internal DataGridViewRowPrePaintEventArgs(DataGridView dataGridView) { Debug.Assert(dataGridView != null); this.dataGridView = dataGridView; } /// public Rectangle ClipBounds { get { return this.clipBounds; } set { this.clipBounds = value; } } /// public string ErrorText { get { return this.errorText; } } /// public Graphics Graphics { get { return this.graphics; } } /// public DataGridViewCellStyle InheritedRowStyle { get { return this.inheritedRowStyle; } } /// public bool IsFirstDisplayedRow { get { return this.isFirstDisplayedRow; } } /// public bool IsLastVisibleRow { get { return this.isLastVisibleRow; } } /// public DataGridViewPaintParts PaintParts { get { return this.paintParts; } set { if ((value & ~DataGridViewPaintParts.All) != 0) { throw new ArgumentException(SR.GetString(SR.DataGridView_InvalidDataGridViewPaintPartsCombination, "value")); } this.paintParts = value; } } /// public Rectangle RowBounds { get { return this.rowBounds; } } /// public int RowIndex { get { return this.rowIndex; } } /// public DataGridViewElementStates State { get { return this.rowState; } } /// public void DrawFocus(Rectangle bounds, bool cellsPaintSelectionBackground) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(this.rowIndex).DrawFocus(this.graphics, this.clipBounds, bounds, this.rowIndex, this.rowState, this.inheritedRowStyle, cellsPaintSelectionBackground); } /// public void PaintCells(Rectangle clipBounds, DataGridViewPaintParts paintParts) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(this.rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } /// public void PaintCellsBackground(Rectangle clipBounds, bool cellsPaintSelectionBackground) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } DataGridViewPaintParts paintParts = DataGridViewPaintParts.Background | DataGridViewPaintParts.Border; if (cellsPaintSelectionBackground) { paintParts |= DataGridViewPaintParts.SelectionBackground; } this.dataGridView.Rows.SharedRow(this.rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } /// public void PaintCellsContent(Rectangle clipBounds) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(this.rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, DataGridViewPaintParts.ContentBackground | DataGridViewPaintParts.ContentForeground | DataGridViewPaintParts.ErrorIcon); } /// public void PaintHeader(bool paintSelectionBackground) { DataGridViewPaintParts paintParts = DataGridViewPaintParts.Background | DataGridViewPaintParts.Border | DataGridViewPaintParts.ContentBackground | DataGridViewPaintParts.ContentForeground | DataGridViewPaintParts.ErrorIcon; if (paintSelectionBackground) { paintParts |= DataGridViewPaintParts.SelectionBackground; } PaintHeader(paintParts); } /// public void PaintHeader(DataGridViewPaintParts paintParts) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(this.rowIndex).PaintHeader(this.graphics, this.clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } internal void SetProperties(Graphics graphics, Rectangle clipBounds, Rectangle rowBounds, int rowIndex, DataGridViewElementStates rowState, string errorText, DataGridViewCellStyle inheritedRowStyle, bool isFirstDisplayedRow, bool isLastVisibleRow) { Debug.Assert(graphics != null); Debug.Assert(inheritedRowStyle != null); this.graphics = graphics; this.clipBounds = clipBounds; this.rowBounds = rowBounds; this.rowIndex = rowIndex; this.rowState = rowState; this.errorText = errorText; this.inheritedRowStyle = inheritedRowStyle; this.isFirstDisplayedRow = isFirstDisplayedRow; this.isLastVisibleRow = isLastVisibleRow; this.paintParts = DataGridViewPaintParts.All; this.Handled = false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
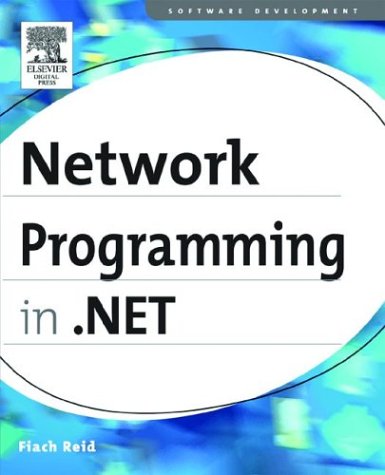
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WinEventQueueItem.cs
- PipelineComponent.cs
- RegexFCD.cs
- PasswordDeriveBytes.cs
- ToolboxItem.cs
- ArcSegment.cs
- WindowsIdentity.cs
- WSHttpBinding.cs
- ComponentEvent.cs
- CacheMode.cs
- X509Certificate2Collection.cs
- PropertyPathWorker.cs
- InputProviderSite.cs
- ReturnEventArgs.cs
- SectionVisual.cs
- ImageIndexConverter.cs
- NotifyParentPropertyAttribute.cs
- InvalidCastException.cs
- CultureInfo.cs
- webproxy.cs
- DataGridItem.cs
- ListBoxItem.cs
- DaylightTime.cs
- ToolStripContentPanelRenderEventArgs.cs
- TableLayoutStyle.cs
- PersonalizationStateQuery.cs
- WindowsGraphics.cs
- Keyboard.cs
- GeometryCollection.cs
- HttpHeaderCollection.cs
- LayoutEvent.cs
- AddingNewEventArgs.cs
- SqlNotificationRequest.cs
- SettingsBindableAttribute.cs
- TemplateComponentConnector.cs
- FactoryRecord.cs
- LiteralControl.cs
- WebBaseEventKeyComparer.cs
- SapiAttributeParser.cs
- TextEditorCopyPaste.cs
- AsyncSerializedWorker.cs
- DataContractSerializerSection.cs
- URIFormatException.cs
- _BaseOverlappedAsyncResult.cs
- ScaleTransform.cs
- SqlServices.cs
- BitmapEffectCollection.cs
- TextUtf8RawTextWriter.cs
- FixedTextSelectionProcessor.cs
- DeclarativeCatalogPart.cs
- EntityType.cs
- __TransparentProxy.cs
- PolyBezierSegment.cs
- DocumentViewerBaseAutomationPeer.cs
- ScrollChrome.cs
- Vector3DCollection.cs
- XamlGridLengthSerializer.cs
- PartialToken.cs
- Stopwatch.cs
- RuleSettings.cs
- PageSettings.cs
- InvalidEnumArgumentException.cs
- Native.cs
- GeometryHitTestParameters.cs
- WindowsButton.cs
- ContainerUtilities.cs
- OdbcConnection.cs
- UInt32Converter.cs
- SingleBodyParameterMessageFormatter.cs
- TranslateTransform3D.cs
- ListViewGroupConverter.cs
- WmlTextViewAdapter.cs
- SimpleHandlerBuildProvider.cs
- LocationSectionRecord.cs
- SqlDataSourceView.cs
- EventHandlers.cs
- ProtocolsConfiguration.cs
- ContentControl.cs
- ColumnCollection.cs
- AsyncPostBackErrorEventArgs.cs
- ChangesetResponse.cs
- BrowserCapabilitiesCodeGenerator.cs
- MapPathBasedVirtualPathProvider.cs
- securestring.cs
- StateValidator.cs
- GreenMethods.cs
- CheckBoxBaseAdapter.cs
- Source.cs
- WebPartConnectVerb.cs
- TypefaceCollection.cs
- BasicSecurityProfileVersion.cs
- WebPartCancelEventArgs.cs
- SystemFonts.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- XmlSchemaImport.cs
- DropDownButton.cs
- LocalizationComments.cs
- XPathParser.cs
- InheritanceRules.cs
- Boolean.cs