Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Controls / ContentControl.cs / 1 / ContentControl.cs
using System; using System.Collections; using System.ComponentModel; using System.Windows.Threading; using System.Diagnostics; using System.Windows.Data; using System.Windows.Media; using System.Windows.Markup; using MS.Utility; using MS.Internal; using MS.Internal.Controls; using MS.Internal.Data; using MS.Internal.KnownBoxes; using MS.Internal.PresentationFramework; using System.Text; namespace System.Windows.Controls { ////// The base class for all controls with a single piece of content. /// ////// ContentControl adds Content, ContentTemplate, ContentTemplateSelector and Part features to a Control. /// [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public class ContentControl : Control, IAddChild { #region Constructors ////// Default DependencyObject constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public ContentControl() : base() { } static ContentControl() { DefaultStyleKeyProperty.OverrideMetadata(typeof(ContentControl), new FrameworkPropertyMetadata(typeof(ContentControl))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ContentControl)); } #endregion #region LogicalTree ////// Returns enumerator to logical children /// protected internal override IEnumerator LogicalChildren { get { object content = Content; if (ContentIsNotLogical || content == null) { return EmptyEnumerator.Instance; } // If the current ContentControl is in a Template.VisualTree and is meant to host // the content for the container then that content shows up as the logical child // for the container and not for the current ContentControl. DependencyObject templatedParent = this.TemplatedParent; if (templatedParent != null) { DependencyObject d = content as DependencyObject; if (d != null) { DependencyObject logicalParent = LogicalTreeHelper.GetParent(d); if (logicalParent != null && logicalParent != this) { return EmptyEnumerator.Instance; } } } return new ContentModelTreeEnumerator(this, content); } } #endregion #region Internal Methods ////// Gives a string representation of this object. /// ///internal override string GetPlainText() { return ContentObjectToString(Content); } internal static string ContentObjectToString(object content) { if (content != null) { FrameworkElement feContent = content as FrameworkElement; if (feContent != null) { return feContent.GetPlainText(); } return content.ToString(); } return String.Empty; } /// /// Prepare to display the item. /// internal void PrepareContentControl(object item, DataTemplate itemTemplate, DataTemplateSelector itemTemplateSelector, string itemStringFormat) { if (item != this) { // don't treat Content as a logical child ContentIsNotLogical = true; // copy styles from the ItemsControl if (ContentIsItem || !HasNonDefaultValue(ContentProperty)) { Content = item; ContentIsItem = true; } if (itemTemplate != null) SetValue(ContentTemplateProperty, itemTemplate); if (itemTemplateSelector != null) SetValue(ContentTemplateSelectorProperty, itemTemplateSelector); if (itemStringFormat != null) SetValue(ContentStringFormatProperty, itemStringFormat); } else { ContentIsNotLogical = false; } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// // Lets derived classes control the serialization behavior for Content DP [EditorBrowsable(EditorBrowsableState.Never)] public virtual bool ShouldSerializeContent() { return ReadLocalValue(ContentProperty) != DependencyProperty.UnsetValue; } #endregion #region IAddChild ////// Add an object child to this control /// void IAddChild.AddChild(object value) { AddChild(value); } ////// Add an object child to this control /// protected virtual void AddChild(object value) { // if conent is the first child or being cleared, set directly if (Content == null || value == null) { Content = value; } else { throw new InvalidOperationException(SR.Get(SRID.ContentControlCannotHaveMultipleContent)); } } ////// Add a text string to this control /// void IAddChild.AddText(string text) { AddText(text); } ////// Add a text string to this control /// protected virtual void AddText(string text) { AddChild(text); } #endregion IAddChild #region Properties ////// The DependencyProperty for the Content property. /// Flags: None /// Default Value: null /// [CommonDependencyProperty] public static readonly DependencyProperty ContentProperty = DependencyProperty.Register( "Content", typeof(object), typeof(ContentControl), new FrameworkPropertyMetadata( (object)null, new PropertyChangedCallback(OnContentChanged))); ////// Content is the data used to generate the child elements of this control. /// [Bindable(true), CustomCategory("Content")] public object Content { get { return GetValue(ContentProperty); } set { SetValue(ContentProperty, value); } } ////// Called when ContentProperty is invalidated on "d." /// private static void OnContentChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ContentControl ctrl = (ContentControl) d; ctrl.SetValue(HasContentPropertyKey, (e.NewValue != null) ? BooleanBoxes.TrueBox : BooleanBoxes.FalseBox); ctrl.OnContentChanged(e.OldValue, e.NewValue); } ////// This method is invoked when the Content property changes. /// /// The old value of the Content property. /// The new value of the Content property. protected virtual void OnContentChanged(object oldContent, object newContent) { // Remove the old content child RemoveLogicalChild(oldContent); // if Content should not be treated as a logical child, there's // nothing to do if (ContentIsNotLogical) return; // If the current ContentControl is in a Template.VisualTree and is meant to host // the content for the container then it must not logically connect the content. if (this.TemplatedParent != null) { DependencyObject d = newContent as DependencyObject; if (d != null && LogicalTreeHelper.GetParent(d) != null) return; } // Add the new content child AddLogicalChild(newContent); } ////// The key needed set a read-only property. /// private static readonly DependencyPropertyKey HasContentPropertyKey = DependencyProperty.RegisterReadOnly( "HasContent", typeof(bool), typeof(ContentControl), new FrameworkPropertyMetadata( BooleanBoxes.FalseBox, FrameworkPropertyMetadataOptions.None)); ////// The DependencyProperty for the HasContent property. /// Flags: None /// Other: Read-Only /// Default Value: false /// [CommonDependencyProperty] public static readonly DependencyProperty HasContentProperty = HasContentPropertyKey.DependencyProperty; ////// True if Content is non-null, false otherwise. /// [Browsable(false), ReadOnly(true)] public bool HasContent { get { return (bool) GetValue(HasContentProperty); } } ////// The DependencyProperty for the ContentTemplate property. /// Flags: None /// Default Value: null /// [CommonDependencyProperty] public static readonly DependencyProperty ContentTemplateProperty = DependencyProperty.Register( "ContentTemplate", typeof(DataTemplate), typeof(ContentControl), new FrameworkPropertyMetadata( (DataTemplate) null, new PropertyChangedCallback(OnContentTemplateChanged))); ////// ContentTemplate is the template used to display the content of the control. /// [Bindable(true), CustomCategory("Content")] public DataTemplate ContentTemplate { get { return (DataTemplate) GetValue(ContentTemplateProperty); } set { SetValue(ContentTemplateProperty, value); } } ////// Called when ContentTemplateProperty is invalidated on "d." /// private static void OnContentTemplateChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ContentControl ctrl = (ContentControl)d; ctrl.OnContentTemplateChanged((DataTemplate) e.OldValue, (DataTemplate) e.NewValue); } ////// This method is invoked when the ContentTemplate property changes. /// /// The old value of the ContentTemplate property. /// The new value of the ContentTemplate property. protected virtual void OnContentTemplateChanged(DataTemplate oldContentTemplate, DataTemplate newContentTemplate) { Helper.CheckTemplateAndTemplateSelector("Content", ContentTemplateProperty, ContentTemplateSelectorProperty, this); } ////// The DependencyProperty for the ContentTemplateSelector property. /// Flags: None /// Default Value: null /// [CommonDependencyProperty] public static readonly DependencyProperty ContentTemplateSelectorProperty = DependencyProperty.Register( "ContentTemplateSelector", typeof(DataTemplateSelector), typeof(ContentControl), new FrameworkPropertyMetadata( (DataTemplateSelector) null, new PropertyChangedCallback(OnContentTemplateSelectorChanged))); ////// ContentTemplateSelector allows the application writer to provide custom logic /// for choosing the template used to display the content of the control. /// ////// This property is ignored if [Bindable(true), CustomCategory("Content")] [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public DataTemplateSelector ContentTemplateSelector { get { return (DataTemplateSelector) GetValue(ContentTemplateSelectorProperty); } set { SetValue(ContentTemplateSelectorProperty, value); } } ///is set. /// /// Called when ContentTemplateSelectorProperty is invalidated on "d." /// private static void OnContentTemplateSelectorChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ContentControl ctrl = (ContentControl) d; ctrl.OnContentTemplateSelectorChanged((DataTemplateSelector) e.NewValue, (DataTemplateSelector) e.NewValue); } ////// This method is invoked when the ContentTemplateSelector property changes. /// /// The old value of the ContentTemplateSelector property. /// The new value of the ContentTemplateSelector property. protected virtual void OnContentTemplateSelectorChanged(DataTemplateSelector oldContentTemplateSelector, DataTemplateSelector newContentTemplateSelector) { Helper.CheckTemplateAndTemplateSelector("Content", ContentTemplateProperty, ContentTemplateSelectorProperty, this); } ////// The DependencyProperty for the ContentStringFormat property. /// Flags: None /// Default Value: null /// [CommonDependencyProperty] public static readonly DependencyProperty ContentStringFormatProperty = DependencyProperty.Register( "ContentStringFormat", typeof(String), typeof(ContentControl), new FrameworkPropertyMetadata( (String) null, new PropertyChangedCallback(OnContentStringFormatChanged))); ////// ContentStringFormat is the format used to display the content of /// the control as a string. This arises only when no template is /// available. /// [Bindable(true), CustomCategory("Content")] public String ContentStringFormat { get { return (String) GetValue(ContentStringFormatProperty); } set { SetValue(ContentStringFormatProperty, value); } } ////// Called when ContentStringFormatProperty is invalidated on "d." /// private static void OnContentStringFormatChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ContentControl ctrl = (ContentControl)d; ctrl.OnContentStringFormatChanged((String) e.OldValue, (String) e.NewValue); } ////// This method is invoked when the ContentStringFormat property changes. /// /// The old value of the ContentStringFormat property. /// The new value of the ContentStringFormat property. protected virtual void OnContentStringFormatChanged(String oldContentStringFormat, String newContentStringFormat) { } #endregion #region Private methods // // Private Methods // ////// Indicates whether Content should be a logical child or not. /// internal bool ContentIsNotLogical { get { return ReadControlFlag(ControlBoolFlags.ContentIsNotLogical); } set { WriteControlFlag(ControlBoolFlags.ContentIsNotLogical, value); } } ////// Indicates whether Content is a data item /// internal bool ContentIsItem { get { return ReadControlFlag(ControlBoolFlags.ContentIsItem); } set { WriteControlFlag(ControlBoolFlags.ContentIsItem, value); } } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 4; } } #endregion Private methods #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.ComponentModel; using System.Windows.Threading; using System.Diagnostics; using System.Windows.Data; using System.Windows.Media; using System.Windows.Markup; using MS.Utility; using MS.Internal; using MS.Internal.Controls; using MS.Internal.Data; using MS.Internal.KnownBoxes; using MS.Internal.PresentationFramework; using System.Text; namespace System.Windows.Controls { ////// The base class for all controls with a single piece of content. /// ////// ContentControl adds Content, ContentTemplate, ContentTemplateSelector and Part features to a Control. /// [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public class ContentControl : Control, IAddChild { #region Constructors ////// Default DependencyObject constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public ContentControl() : base() { } static ContentControl() { DefaultStyleKeyProperty.OverrideMetadata(typeof(ContentControl), new FrameworkPropertyMetadata(typeof(ContentControl))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ContentControl)); } #endregion #region LogicalTree ////// Returns enumerator to logical children /// protected internal override IEnumerator LogicalChildren { get { object content = Content; if (ContentIsNotLogical || content == null) { return EmptyEnumerator.Instance; } // If the current ContentControl is in a Template.VisualTree and is meant to host // the content for the container then that content shows up as the logical child // for the container and not for the current ContentControl. DependencyObject templatedParent = this.TemplatedParent; if (templatedParent != null) { DependencyObject d = content as DependencyObject; if (d != null) { DependencyObject logicalParent = LogicalTreeHelper.GetParent(d); if (logicalParent != null && logicalParent != this) { return EmptyEnumerator.Instance; } } } return new ContentModelTreeEnumerator(this, content); } } #endregion #region Internal Methods ////// Gives a string representation of this object. /// ///internal override string GetPlainText() { return ContentObjectToString(Content); } internal static string ContentObjectToString(object content) { if (content != null) { FrameworkElement feContent = content as FrameworkElement; if (feContent != null) { return feContent.GetPlainText(); } return content.ToString(); } return String.Empty; } /// /// Prepare to display the item. /// internal void PrepareContentControl(object item, DataTemplate itemTemplate, DataTemplateSelector itemTemplateSelector, string itemStringFormat) { if (item != this) { // don't treat Content as a logical child ContentIsNotLogical = true; // copy styles from the ItemsControl if (ContentIsItem || !HasNonDefaultValue(ContentProperty)) { Content = item; ContentIsItem = true; } if (itemTemplate != null) SetValue(ContentTemplateProperty, itemTemplate); if (itemTemplateSelector != null) SetValue(ContentTemplateSelectorProperty, itemTemplateSelector); if (itemStringFormat != null) SetValue(ContentStringFormatProperty, itemStringFormat); } else { ContentIsNotLogical = false; } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// // Lets derived classes control the serialization behavior for Content DP [EditorBrowsable(EditorBrowsableState.Never)] public virtual bool ShouldSerializeContent() { return ReadLocalValue(ContentProperty) != DependencyProperty.UnsetValue; } #endregion #region IAddChild ////// Add an object child to this control /// void IAddChild.AddChild(object value) { AddChild(value); } ////// Add an object child to this control /// protected virtual void AddChild(object value) { // if conent is the first child or being cleared, set directly if (Content == null || value == null) { Content = value; } else { throw new InvalidOperationException(SR.Get(SRID.ContentControlCannotHaveMultipleContent)); } } ////// Add a text string to this control /// void IAddChild.AddText(string text) { AddText(text); } ////// Add a text string to this control /// protected virtual void AddText(string text) { AddChild(text); } #endregion IAddChild #region Properties ////// The DependencyProperty for the Content property. /// Flags: None /// Default Value: null /// [CommonDependencyProperty] public static readonly DependencyProperty ContentProperty = DependencyProperty.Register( "Content", typeof(object), typeof(ContentControl), new FrameworkPropertyMetadata( (object)null, new PropertyChangedCallback(OnContentChanged))); ////// Content is the data used to generate the child elements of this control. /// [Bindable(true), CustomCategory("Content")] public object Content { get { return GetValue(ContentProperty); } set { SetValue(ContentProperty, value); } } ////// Called when ContentProperty is invalidated on "d." /// private static void OnContentChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ContentControl ctrl = (ContentControl) d; ctrl.SetValue(HasContentPropertyKey, (e.NewValue != null) ? BooleanBoxes.TrueBox : BooleanBoxes.FalseBox); ctrl.OnContentChanged(e.OldValue, e.NewValue); } ////// This method is invoked when the Content property changes. /// /// The old value of the Content property. /// The new value of the Content property. protected virtual void OnContentChanged(object oldContent, object newContent) { // Remove the old content child RemoveLogicalChild(oldContent); // if Content should not be treated as a logical child, there's // nothing to do if (ContentIsNotLogical) return; // If the current ContentControl is in a Template.VisualTree and is meant to host // the content for the container then it must not logically connect the content. if (this.TemplatedParent != null) { DependencyObject d = newContent as DependencyObject; if (d != null && LogicalTreeHelper.GetParent(d) != null) return; } // Add the new content child AddLogicalChild(newContent); } ////// The key needed set a read-only property. /// private static readonly DependencyPropertyKey HasContentPropertyKey = DependencyProperty.RegisterReadOnly( "HasContent", typeof(bool), typeof(ContentControl), new FrameworkPropertyMetadata( BooleanBoxes.FalseBox, FrameworkPropertyMetadataOptions.None)); ////// The DependencyProperty for the HasContent property. /// Flags: None /// Other: Read-Only /// Default Value: false /// [CommonDependencyProperty] public static readonly DependencyProperty HasContentProperty = HasContentPropertyKey.DependencyProperty; ////// True if Content is non-null, false otherwise. /// [Browsable(false), ReadOnly(true)] public bool HasContent { get { return (bool) GetValue(HasContentProperty); } } ////// The DependencyProperty for the ContentTemplate property. /// Flags: None /// Default Value: null /// [CommonDependencyProperty] public static readonly DependencyProperty ContentTemplateProperty = DependencyProperty.Register( "ContentTemplate", typeof(DataTemplate), typeof(ContentControl), new FrameworkPropertyMetadata( (DataTemplate) null, new PropertyChangedCallback(OnContentTemplateChanged))); ////// ContentTemplate is the template used to display the content of the control. /// [Bindable(true), CustomCategory("Content")] public DataTemplate ContentTemplate { get { return (DataTemplate) GetValue(ContentTemplateProperty); } set { SetValue(ContentTemplateProperty, value); } } ////// Called when ContentTemplateProperty is invalidated on "d." /// private static void OnContentTemplateChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ContentControl ctrl = (ContentControl)d; ctrl.OnContentTemplateChanged((DataTemplate) e.OldValue, (DataTemplate) e.NewValue); } ////// This method is invoked when the ContentTemplate property changes. /// /// The old value of the ContentTemplate property. /// The new value of the ContentTemplate property. protected virtual void OnContentTemplateChanged(DataTemplate oldContentTemplate, DataTemplate newContentTemplate) { Helper.CheckTemplateAndTemplateSelector("Content", ContentTemplateProperty, ContentTemplateSelectorProperty, this); } ////// The DependencyProperty for the ContentTemplateSelector property. /// Flags: None /// Default Value: null /// [CommonDependencyProperty] public static readonly DependencyProperty ContentTemplateSelectorProperty = DependencyProperty.Register( "ContentTemplateSelector", typeof(DataTemplateSelector), typeof(ContentControl), new FrameworkPropertyMetadata( (DataTemplateSelector) null, new PropertyChangedCallback(OnContentTemplateSelectorChanged))); ////// ContentTemplateSelector allows the application writer to provide custom logic /// for choosing the template used to display the content of the control. /// ////// This property is ignored if [Bindable(true), CustomCategory("Content")] [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public DataTemplateSelector ContentTemplateSelector { get { return (DataTemplateSelector) GetValue(ContentTemplateSelectorProperty); } set { SetValue(ContentTemplateSelectorProperty, value); } } ///is set. /// /// Called when ContentTemplateSelectorProperty is invalidated on "d." /// private static void OnContentTemplateSelectorChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ContentControl ctrl = (ContentControl) d; ctrl.OnContentTemplateSelectorChanged((DataTemplateSelector) e.NewValue, (DataTemplateSelector) e.NewValue); } ////// This method is invoked when the ContentTemplateSelector property changes. /// /// The old value of the ContentTemplateSelector property. /// The new value of the ContentTemplateSelector property. protected virtual void OnContentTemplateSelectorChanged(DataTemplateSelector oldContentTemplateSelector, DataTemplateSelector newContentTemplateSelector) { Helper.CheckTemplateAndTemplateSelector("Content", ContentTemplateProperty, ContentTemplateSelectorProperty, this); } ////// The DependencyProperty for the ContentStringFormat property. /// Flags: None /// Default Value: null /// [CommonDependencyProperty] public static readonly DependencyProperty ContentStringFormatProperty = DependencyProperty.Register( "ContentStringFormat", typeof(String), typeof(ContentControl), new FrameworkPropertyMetadata( (String) null, new PropertyChangedCallback(OnContentStringFormatChanged))); ////// ContentStringFormat is the format used to display the content of /// the control as a string. This arises only when no template is /// available. /// [Bindable(true), CustomCategory("Content")] public String ContentStringFormat { get { return (String) GetValue(ContentStringFormatProperty); } set { SetValue(ContentStringFormatProperty, value); } } ////// Called when ContentStringFormatProperty is invalidated on "d." /// private static void OnContentStringFormatChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ContentControl ctrl = (ContentControl)d; ctrl.OnContentStringFormatChanged((String) e.OldValue, (String) e.NewValue); } ////// This method is invoked when the ContentStringFormat property changes. /// /// The old value of the ContentStringFormat property. /// The new value of the ContentStringFormat property. protected virtual void OnContentStringFormatChanged(String oldContentStringFormat, String newContentStringFormat) { } #endregion #region Private methods // // Private Methods // ////// Indicates whether Content should be a logical child or not. /// internal bool ContentIsNotLogical { get { return ReadControlFlag(ControlBoolFlags.ContentIsNotLogical); } set { WriteControlFlag(ControlBoolFlags.ContentIsNotLogical, value); } } ////// Indicates whether Content is a data item /// internal bool ContentIsItem { get { return ReadControlFlag(ControlBoolFlags.ContentIsItem); } set { WriteControlFlag(ControlBoolFlags.ContentIsItem, value); } } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 4; } } #endregion Private methods #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
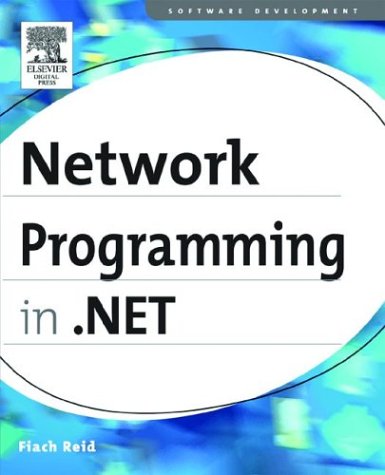
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- controlskin.cs
- TextDecorationCollection.cs
- IDictionary.cs
- InfoCardTrace.cs
- HtmlTableCell.cs
- DataTrigger.cs
- PackagingUtilities.cs
- WriteTimeStream.cs
- SessionStateSection.cs
- ExpressionPrefixAttribute.cs
- ToolStripDesignerUtils.cs
- DeleteWorkflowOwnerCommand.cs
- DesignerGenericWebPart.cs
- ObjectCache.cs
- ObjectAnimationBase.cs
- StringFunctions.cs
- Image.cs
- GiveFeedbackEvent.cs
- ModelUtilities.cs
- SafeSecurityHelper.cs
- XmlQueryTypeFactory.cs
- DayRenderEvent.cs
- SqlAggregateChecker.cs
- _OSSOCK.cs
- View.cs
- TableHeaderCell.cs
- TypeExtension.cs
- SafeCryptoHandles.cs
- SourceFileBuildProvider.cs
- SqlFlattener.cs
- AQNBuilder.cs
- PeerToPeerException.cs
- BamlLocalizationDictionary.cs
- CompiledIdentityConstraint.cs
- SourceFileBuildProvider.cs
- ChannelServices.cs
- TextRangeEditTables.cs
- AddInPipelineAttributes.cs
- EdmComplexTypeAttribute.cs
- HttpContext.cs
- GridPatternIdentifiers.cs
- ButtonBase.cs
- FamilyCollection.cs
- SessionState.cs
- ObjectTokenCategory.cs
- PngBitmapDecoder.cs
- CodeExpressionCollection.cs
- SchemaTypeEmitter.cs
- ProfileSection.cs
- ErrorsHelper.cs
- TraceContextRecord.cs
- TableLayoutPanel.cs
- PrinterUnitConvert.cs
- ParentUndoUnit.cs
- SwitchExpression.cs
- RSAProtectedConfigurationProvider.cs
- processwaithandle.cs
- WCFServiceClientProxyGenerator.cs
- SafeEventHandle.cs
- TextContainer.cs
- SecurityDescriptor.cs
- DataMisalignedException.cs
- ReplacementText.cs
- LinkArea.cs
- TrackingConditionCollection.cs
- ItemContainerGenerator.cs
- FontWeights.cs
- KeyBinding.cs
- MsmqHostedTransportManager.cs
- XmlSerializerNamespaces.cs
- WindowShowOrOpenTracker.cs
- SqlDependencyUtils.cs
- RecognitionResult.cs
- KeySpline.cs
- DataGridViewEditingControlShowingEventArgs.cs
- ToolTipAutomationPeer.cs
- HtmlShim.cs
- XhtmlBasicFormAdapter.cs
- ActivityTypeDesigner.xaml.cs
- SiteOfOriginPart.cs
- WebResourceAttribute.cs
- CustomAttributeSerializer.cs
- SiteMap.cs
- Brush.cs
- infer.cs
- GeneralTransform2DTo3D.cs
- HwndStylusInputProvider.cs
- ApplicationDirectory.cs
- Char.cs
- ConnectionManager.cs
- WorkflowRuntimeService.cs
- HandlerBase.cs
- DES.cs
- DesignerActionItemCollection.cs
- FormView.cs
- TreeNodeBindingCollection.cs
- Int64.cs
- LogicalTreeHelper.cs
- Publisher.cs
- ConnectAlgorithms.cs