Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / State / SessionState.cs / 1 / SessionState.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HttpSessionState * * Copyright (c) 1998-1999, Microsoft Corporation * */ namespace System.Web.SessionState { using System.Threading; using System.Runtime.InteropServices; using System.Web; using System.Collections; using System.Collections.Specialized; using System.Text; using System.Globalization; using System.Security.Permissions; ////// public enum SessionStateMode { ///[To be supplied.] ////// Off = 0, ///[To be supplied.] ////// InProc = 1, ///[To be supplied.] ////// StateServer = 2, ///[To be supplied.] ////// SQLServer = 3, ///[To be supplied.] ////// Custom = 4 }; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public interface IHttpSessionState { string SessionID { get; } /* * The length of a session before it times out, in minutes. */ ///[To be supplied.] ////// int Timeout { get; set; } /* * Is this a new session? */ ///[To be supplied.] ////// bool IsNewSession { get; } /* * Is session state in a separate process */ ///[To be supplied.] ////// SessionStateMode Mode { get; } /* * Is session state cookieless? */ bool IsCookieless { get; } HttpCookieMode CookieMode { get; } /* * Abandon the session. * */ ///[To be supplied.] ////// void Abandon(); ///[To be supplied.] ////// int LCID { get; set; } ///[To be supplied.] ////// int CodePage { get; set; } ///[To be supplied.] ////// HttpStaticObjectsCollection StaticObjects { get; } ///[To be supplied.] ////// Object this[String name] { get; set; } ///[To be supplied.] ////// Object this[int index] { get; set; } ///[To be supplied.] ////// void Add(String name, Object value); ///[To be supplied.] ////// void Remove(String name); ///[To be supplied.] ////// void RemoveAt(int index); ///[To be supplied.] ////// void Clear(); ///[To be supplied.] ////// void RemoveAll(); ///[To be supplied.] ////// int Count { get; } ///[To be supplied.] ////// NameObjectCollectionBase.KeysCollection Keys { get; } ///[To be supplied.] ////// IEnumerator GetEnumerator(); ///[To be supplied.] ////// void CopyTo(Array array, int index); ///[To be supplied.] ////// Object SyncRoot { get; } ///[To be supplied.] ////// bool IsReadOnly { get; } ///[To be supplied.] ////// bool IsSynchronized { get; } } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpSessionState : ICollection { private IHttpSessionState _container; internal HttpSessionState(IHttpSessionState container) { _container = container; } internal IHttpSessionState Container { get { return _container; } } /* * The Id of the session. */ ///[To be supplied.] ////// public String SessionID { get {return _container.SessionID;} } /* * The length of a session before it times out, in minutes. */ ///[To be supplied.] ////// public int Timeout { get {return _container.Timeout;} set {_container.Timeout = value;} } /* * Is this a new session? */ ///[To be supplied.] ////// public bool IsNewSession { get {return _container.IsNewSession;} } /* * Is session state in a separate process */ ///[To be supplied.] ////// public SessionStateMode Mode { get {return _container.Mode;} } /* * Is session state cookieless? */ public bool IsCookieless { get {return _container.IsCookieless;} } public HttpCookieMode CookieMode { get {return _container.CookieMode; } } /* * Abandon the session. * */ ///[To be supplied.] ////// public void Abandon() { _container.Abandon(); } ///[To be supplied.] ////// public int LCID { get { return _container.LCID; } set { _container.LCID = value; } } ///[To be supplied.] ////// public int CodePage { get { return _container.CodePage; } set { _container.CodePage = value; } } ///[To be supplied.] ////// public HttpSessionState Contents { get {return this;} } ///[To be supplied.] ////// public HttpStaticObjectsCollection StaticObjects { get { return _container.StaticObjects;} } ///[To be supplied.] ////// public Object this[String name] { get { return _container[name]; } set { _container[name] = value; } } ///[To be supplied.] ////// public Object this[int index] { get {return _container[index];} set {_container[index] = value;} } ///[To be supplied.] ////// public void Add(String name, Object value) { _container[name] = value; } ///[To be supplied.] ////// public void Remove(String name) { _container.Remove(name); } ///[To be supplied.] ////// public void RemoveAt(int index) { _container.RemoveAt(index); } ///[To be supplied.] ////// public void Clear() { _container.Clear(); } ///[To be supplied.] ////// public void RemoveAll() { Clear(); } ///[To be supplied.] ////// public int Count { get {return _container.Count;} } ///[To be supplied.] ////// public NameObjectCollectionBase.KeysCollection Keys { get {return _container.Keys;} } ///[To be supplied.] ////// public IEnumerator GetEnumerator() { return _container.GetEnumerator(); } ///[To be supplied.] ////// public void CopyTo(Array array, int index) { _container.CopyTo(array, index); } ///[To be supplied.] ////// public Object SyncRoot { get { return _container.SyncRoot;} } ///[To be supplied.] ////// public bool IsReadOnly { get { return _container.IsReadOnly;} } ///[To be supplied.] ////// public bool IsSynchronized { get { return _container.IsSynchronized;} } } }[To be supplied.] ///
Link Menu
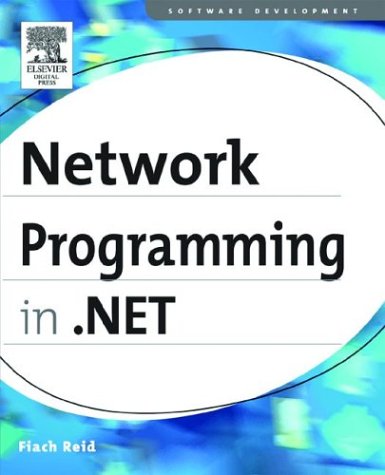
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlReader.cs
- WebServiceParameterData.cs
- SqlUserDefinedAggregateAttribute.cs
- _CommandStream.cs
- CapabilitiesSection.cs
- ComponentConverter.cs
- TouchesCapturedWithinProperty.cs
- BoundsDrawingContextWalker.cs
- Set.cs
- PersonalizationStateInfoCollection.cs
- FontSourceCollection.cs
- ActiveXSite.cs
- VisualStyleInformation.cs
- FormView.cs
- DependencyObjectProvider.cs
- ActivationArguments.cs
- ConfigXmlText.cs
- XmlHierarchicalEnumerable.cs
- TextEditorTyping.cs
- MultiView.cs
- TransformPattern.cs
- Double.cs
- Profiler.cs
- XMLSchema.cs
- UserMapPath.cs
- AliasedExpr.cs
- ByteKeyFrameCollection.cs
- ParallelLoopState.cs
- WorkflowDataContext.cs
- IsolatedStorageFile.cs
- Util.cs
- UniqueIdentifierService.cs
- TemplateControlParser.cs
- PasswordRecovery.cs
- DownloadProgressEventArgs.cs
- MultitargetUtil.cs
- MultiBinding.cs
- Mutex.cs
- LineInfo.cs
- _UriTypeConverter.cs
- FunctionImportMapping.cs
- PersonalizationStateInfo.cs
- NavigationPropertyEmitter.cs
- XsltSettings.cs
- XsltSettings.cs
- TextRangeBase.cs
- BuildProviderUtils.cs
- SynchronizedRandom.cs
- ConfigXmlReader.cs
- RoleService.cs
- ValidatingPropertiesEventArgs.cs
- MetadataWorkspace.cs
- Timer.cs
- IPEndPointCollection.cs
- Size3D.cs
- X509Chain.cs
- ResourceDefaultValueAttribute.cs
- WebPartEditorCancelVerb.cs
- LoginDesigner.cs
- IConvertible.cs
- LinkedResource.cs
- WindowsGraphics2.cs
- Roles.cs
- CommonObjectSecurity.cs
- TextServicesCompartmentEventSink.cs
- DataGridViewButtonColumn.cs
- WSSecurityOneDotOneReceiveSecurityHeader.cs
- Menu.cs
- PackageRelationshipCollection.cs
- XmlAttributeOverrides.cs
- ServiceInfoCollection.cs
- FunctionMappingTranslator.cs
- HTMLTextWriter.cs
- ButtonChrome.cs
- ReflectionHelper.cs
- DrawingImage.cs
- SafeHandles.cs
- InvokePattern.cs
- XmlElementAttribute.cs
- GroupQuery.cs
- DataBindingHandlerAttribute.cs
- CompilerCollection.cs
- SchemaImporterExtensionElement.cs
- PathData.cs
- Encoder.cs
- EntityCommandCompilationException.cs
- Trigger.cs
- Config.cs
- ArcSegment.cs
- ConstructorBuilder.cs
- CodeArgumentReferenceExpression.cs
- ImmComposition.cs
- XmlDesigner.cs
- ConnectionStringsSection.cs
- UserNameSecurityTokenAuthenticator.cs
- GridProviderWrapper.cs
- UIAgentRequest.cs
- GPRECT.cs
- HtmlWindowCollection.cs
- ObjectDataSourceFilteringEventArgs.cs