Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Security / Util / Config.cs / 3 / Config.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Config.cs // namespace System.Security.Util { using System; using System.Security.Util; using System.Security.Policy; using System.Security.Permissions; using System.Collections; using System.IO; using System.Reflection; using System.Globalization; using System.Text; using System.Runtime.Serialization.Formatters.Binary; using System.Threading; using System.Runtime.CompilerServices; // Duplicated in vm\COMSecurityConfig.h [Serializable,Flags] internal enum QuickCacheEntryType { FullTrustZoneMyComputer = 0x1000000, FullTrustZoneIntranet = 0x2000000, FullTrustZoneInternet = 0x4000000, FullTrustZoneTrusted = 0x8000000, FullTrustZoneUntrusted = 0x10000000, FullTrustAll = 0x20000000, } internal static class Config { private static string m_machineConfig; private static string m_userConfig; private static void GetFileLocales() { if (m_machineConfig == null) m_machineConfig = _GetMachineDirectory(); if (m_userConfig == null) m_userConfig = _GetUserDirectory(); } internal static string MachineDirectory { get { GetFileLocales(); return m_machineConfig; } } internal static string UserDirectory { get { GetFileLocales(); return m_userConfig; } } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern bool SaveDataByte(string path, byte[] data, int offset, int length); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern bool RecoverData(ConfigId id); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern void SetQuickCache(ConfigId id, QuickCacheEntryType quickCacheFlags); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern bool GetCacheEntry(ConfigId id, int numKey, char[] key, out byte[] data); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern void AddCacheEntry(ConfigId id, int numKey, char[] key, byte[] data); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern void ResetCacheData(ConfigId id); [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern string _GetMachineDirectory(); [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern string _GetUserDirectory(); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern bool WriteToEventLog(string message); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
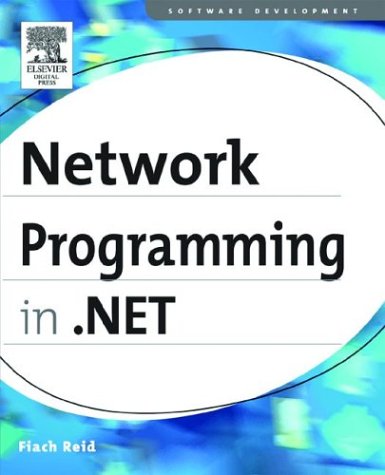
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Validator.cs
- StatusBarPanelClickEvent.cs
- OperandQuery.cs
- LockCookie.cs
- TextElementCollection.cs
- ToolStripGripRenderEventArgs.cs
- FileUtil.cs
- DbConnectionPool.cs
- securitycriticaldataClass.cs
- FastPropertyAccessor.cs
- SqlExpressionNullability.cs
- StagingAreaInputItem.cs
- EdmMember.cs
- PeerCollaborationPermission.cs
- AtomMaterializerLog.cs
- MediaTimeline.cs
- DocumentPageViewAutomationPeer.cs
- WindowsPrincipal.cs
- MsmqAppDomainProtocolHandler.cs
- FontTypeConverter.cs
- Errors.cs
- ForeignKeyConstraint.cs
- SelectingProviderEventArgs.cs
- IPAddress.cs
- FixedPosition.cs
- Base64Decoder.cs
- BrowserCapabilitiesCodeGenerator.cs
- FileDialog_Vista.cs
- VerticalAlignConverter.cs
- Brush.cs
- BindingCollection.cs
- RuleSettings.cs
- FacetValues.cs
- ReadOnlyCollection.cs
- ColorConverter.cs
- Simplifier.cs
- UICuesEvent.cs
- CodeSnippetCompileUnit.cs
- MailBnfHelper.cs
- VirtualPathUtility.cs
- BinaryFormatter.cs
- StrokeDescriptor.cs
- SchemaCollectionCompiler.cs
- Int32Rect.cs
- Identifier.cs
- EditCommandColumn.cs
- HttpProfileGroupBase.cs
- DispatcherProcessingDisabled.cs
- ValueChangedEventManager.cs
- UserControlDesigner.cs
- GroupBox.cs
- StreamGeometry.cs
- ContentWrapperAttribute.cs
- DataBinding.cs
- ResourceSet.cs
- Perspective.cs
- ping.cs
- CommonServiceBehaviorElement.cs
- SeekStoryboard.cs
- AsyncCompletedEventArgs.cs
- QueryReaderSettings.cs
- SqlCommandBuilder.cs
- SigningProgress.cs
- ClonableStack.cs
- ToolStripSplitButton.cs
- CodeCommentStatementCollection.cs
- TraceListeners.cs
- ObjectReaderCompiler.cs
- DataSet.cs
- FileDetails.cs
- PersianCalendar.cs
- ListViewGroupItemCollection.cs
- CultureInfoConverter.cs
- ResourcePool.cs
- ReferenceConverter.cs
- StructuredProperty.cs
- metadatamappinghashervisitor.hashsourcebuilder.cs
- KeyFrames.cs
- OdbcConnection.cs
- Compilation.cs
- TypedReference.cs
- SafeProcessHandle.cs
- MiniParameterInfo.cs
- EncryptedKeyIdentifierClause.cs
- XmlAtomErrorReader.cs
- PropertyValueUIItem.cs
- Hyperlink.cs
- ChangeProcessor.cs
- WebContext.cs
- DayRenderEvent.cs
- Transform3D.cs
- oledbconnectionstring.cs
- TargetInvocationException.cs
- Exceptions.cs
- DataTableClearEvent.cs
- AttributeCollection.cs
- NegotiateStream.cs
- MemberProjectionIndex.cs
- DeferredRunTextReference.cs
- ListViewHitTestInfo.cs