Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / EdmMember.cs / 1305376 / EdmMember.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Diagnostics; using System.Text; namespace System.Data.Metadata.Edm { ////// Represents the edm member class /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public abstract class EdmMember : MetadataItem { ////// Initializes a new instance of EdmMember class /// /// name of the member /// type information containing info about member's type and its facet internal EdmMember(string name, TypeUsage memberTypeUsage) { EntityUtil.CheckStringArgument(name, "name"); EntityUtil.GenericCheckArgumentNull(memberTypeUsage, "memberTypeUsage"); _name = name; _typeUsage = memberTypeUsage; } private TypeUsage _typeUsage; private string _name; private StructuralType _declaringType; ////// Returns the identity of the member /// internal override string Identity { get { return this.Name; } } ////// Returns the name of the member /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _name; } } ////// Returns the declaring type of the member /// public StructuralType DeclaringType { get { return _declaringType; } } ////// Returns the TypeUsage object containing the type information and facets /// about the type /// [MetadataProperty(BuiltInTypeKind.TypeUsage, false)] public TypeUsage TypeUsage { get { return _typeUsage; } } ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return Name; } ////// Sets the member to read only mode. Once this is done, there are no changes /// that can be done to this class /// internal override void SetReadOnly() { if (!IsReadOnly) { base.SetReadOnly(); // TypeUsage is always readonly, no need to set it } } ////// Change the declaring type without doing fixup in the member collection /// internal void ChangeDeclaringTypeWithoutCollectionFixup(StructuralType newDeclaringType) { _declaringType = newDeclaringType; } ////// Tells whether this member is marked as a Computed member in the EDM definition /// internal bool IsStoreGeneratedComputed { get { Facet item=null; if (TypeUsage.Facets.TryGetValue(EdmProviderManifest.StoreGeneratedPatternFacetName, false, out item)) { return ((StoreGeneratedPattern)item.Value) == StoreGeneratedPattern.Computed; } return false; } } ////// Tells whether this member's Store generated pattern is marked as Identity in the EDM definition /// internal bool IsStoreGeneratedIdentity { get { Facet item = null; if (TypeUsage.Facets.TryGetValue(EdmProviderManifest.StoreGeneratedPatternFacetName, false, out item)) { return ((StoreGeneratedPattern)item.Value) == StoreGeneratedPattern.Identity; } return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
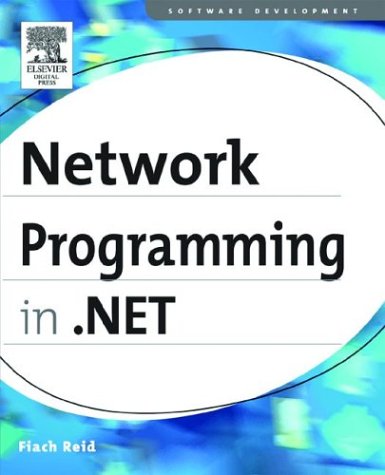
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KoreanCalendar.cs
- TextRunProperties.cs
- StateBag.cs
- MultiPartWriter.cs
- DBCSCodePageEncoding.cs
- AssemblyAttributes.cs
- DecoderFallback.cs
- PropagatorResult.cs
- QilGenerator.cs
- MergeFilterQuery.cs
- ListItemConverter.cs
- WorkflowInstanceExtensionCollection.cs
- MsmqIntegrationBinding.cs
- RandomNumberGenerator.cs
- CacheSection.cs
- DESCryptoServiceProvider.cs
- CacheManager.cs
- DependencyObjectProvider.cs
- InternalPolicyElement.cs
- ThreadAttributes.cs
- SqlOuterApplyReducer.cs
- DomainConstraint.cs
- ProcessModule.cs
- DataGridViewColumnCollectionEditor.cs
- VirtualizingPanel.cs
- TypefaceCollection.cs
- Types.cs
- Debug.cs
- FloaterParaClient.cs
- SystemThemeKey.cs
- ContravarianceAdapter.cs
- Rotation3DAnimation.cs
- InlineUIContainer.cs
- AutoSizeToolBoxItem.cs
- CommandEventArgs.cs
- DesignTimeTemplateParser.cs
- HiddenField.cs
- COM2PropertyDescriptor.cs
- ReachFixedDocumentSerializer.cs
- Memoizer.cs
- BinaryConverter.cs
- CalendarButtonAutomationPeer.cs
- LookupNode.cs
- WebPartCatalogCloseVerb.cs
- OciHandle.cs
- WebColorConverter.cs
- RawStylusActions.cs
- validation.cs
- ToggleButtonAutomationPeer.cs
- columnmapkeybuilder.cs
- ToolBarTray.cs
- FieldBuilder.cs
- ServiceObjectContainer.cs
- ScrollViewer.cs
- LoggedException.cs
- DesignerDeviceConfig.cs
- OrderedDictionaryStateHelper.cs
- InkPresenter.cs
- WindowPatternIdentifiers.cs
- ListDictionaryInternal.cs
- EventLogQuery.cs
- XPathSelfQuery.cs
- SmtpLoginAuthenticationModule.cs
- View.cs
- RouteItem.cs
- CoTaskMemUnicodeSafeHandle.cs
- SingleAnimation.cs
- DynamicMethod.cs
- SerializationObjectManager.cs
- ReceiveSecurityHeaderEntry.cs
- AvtEvent.cs
- IODescriptionAttribute.cs
- TextLine.cs
- CounterSample.cs
- FragmentQueryKB.cs
- HttpCacheVary.cs
- PrimitiveList.cs
- DefaultObjectMappingItemCollection.cs
- Condition.cs
- MemberPath.cs
- SHA1Cng.cs
- FlowDocumentReaderAutomationPeer.cs
- DataSetMappper.cs
- WindowsSolidBrush.cs
- RecipientIdentity.cs
- SamlAssertion.cs
- Lasso.cs
- DataGridViewAccessibleObject.cs
- RC2.cs
- Tablet.cs
- RemoteEndpointMessageProperty.cs
- SingleConverter.cs
- ValidationSummary.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- Span.cs
- DataTableReader.cs
- SqlClientWrapperSmiStream.cs
- ContextInformation.cs
- EllipseGeometry.cs
- ObjectMemberMapping.cs