Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / ReachFixedDocumentSerializer.cs / 1 / ReachFixedDocumentSerializer.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: ReachFixedDocumentSerializer.cs Abstract: This file contains the definition of a class that defines the common functionality required to serialize a FixedDocument Author: [....] ([....]) 1-December-2004 Revision History: --*/ using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Markup; namespace System.Windows.Xps.Serialization { ////// Class defining common functionality required to /// serialize a FixedDocument. /// internal class FixedDocumentSerializer : ReachSerializer { #region Constructor ////// Constructor for class FixedDocumentSerializer /// /// /// The serialization manager, the services of which are /// used later in the serialization process of the type. /// public FixedDocumentSerializer( PackageSerializationManager manager ): base(manager) { } #endregion Constructor #region Public Methods ////// The main method that is called to serialize a FixedDocument. /// /// /// Instance of object to be serialized. /// public override void SerializeObject( Object serializedObject ) { // // Create the ImageTable required by the Type Converters // The Image table at this time is shared / document // ((XpsSerializationManager)SerializationManager).ResourcePolicy.ImageCrcTable = new Dictionary(); ((XpsSerializationManager)SerializationManager).ResourcePolicy.ImageUriHashTable = new Dictionary (); // // Create the ColorContextTable required by the Type Converters // The Image table at this time is shared / document // ((XpsSerializationManager)SerializationManager).ResourcePolicy.ColorContextTable = new Dictionary (); base.SerializeObject(serializedObject); } #endregion Public Methods #region Internal Methods /// /// The main method that is called to serialize the FixedDocument /// and that is usually called from within the serialization manager /// when a node in the graph of objects is at a turn where it should /// be serialized. /// /// /// The context of the property being serialized at this time and /// it points internally to the object encapsulated by that node. /// internal override void SerializeObject( SerializablePropertyContext serializedProperty ) { // // Create the ImageTable required by the Type Converters // The Image table at this time is shared / document // ((XpsSerializationManager)SerializationManager).ResourcePolicy.ImageCrcTable = new Dictionary(); ((XpsSerializationManager)SerializationManager).ResourcePolicy.ImageUriHashTable = new Dictionary (); // // Create the ColorContextTable required by the Type Converters // The Image table at this time is shared / document // ((XpsSerializationManager)SerializationManager).ResourcePolicy.ColorContextTable = new Dictionary (); base.SerializeObject(serializedProperty); } /// /// The method is called once the object data is discovered at that /// point of the serizlization process. /// /// /// The context of the object to be serialized at this time. /// internal override void PersistObjectData( SerializableObjectContext serializableObjectContext ) { if(serializableObjectContext == null) { throw new ArgumentNullException("serializableObjectContext"); } String xmlnsForType = SerializationManager.GetXmlNSForType(typeof(FixedDocument)); if( SerializationManager is XpsSerializationManager) { (SerializationManager as XpsSerializationManager).RegisterDocumentStart(); } if(xmlnsForType == null) { XmlWriter.WriteStartElement(serializableObjectContext.Name); } else { XmlWriter.WriteStartElement(serializableObjectContext.Name, xmlnsForType); } { if(serializableObjectContext.IsComplexValue) { XpsSerializationPrintTicketRequiredEventArgs e = new XpsSerializationPrintTicketRequiredEventArgs(PrintTicketLevel.FixedDocumentPrintTicket, 0); ((XpsSerializationManager)SerializationManager).OnXPSSerializationPrintTicketRequired(e); // // Serialize the data for the PrintTicket // if(e.Modified) { if(e.PrintTicket != null) { PrintTicketSerializer serializer = new PrintTicketSerializer(SerializationManager); serializer.SerializeObject(e.PrintTicket); } } SerializeObjectCore(serializableObjectContext); } else { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_WrongPropertyTypeForFixedDocument)); } } // // Clear off the table from the packaging policy // ((XpsSerializationManager)SerializationManager).ResourcePolicy.ImageCrcTable = null; ((XpsSerializationManager)SerializationManager).ResourcePolicy.ImageUriHashTable = null; XmlWriter.WriteEndElement(); XmlWriter = null; // // Signal to any registered callers that the Document has been serialized // XpsSerializationProgressChangedEventArgs progressEvent = new XpsSerializationProgressChangedEventArgs(XpsWritingProgressChangeLevel.FixedDocumentWritingProgress, 0, 0, null); ((XpsSerializationManager)SerializationManager).OnXPSSerializationProgressChanged(progressEvent); if( SerializationManager is XpsSerializationManager) { (SerializationManager as XpsSerializationManager).RegisterDocumentEnd(); } } ////// This method is the one that writed out the attribute within /// the xml stream when serializing simple properties. /// /// /// The property that is to be serialized as an attribute at this time. /// internal override void WriteSerializedAttribute( SerializablePropertyContext serializablePropertyContext ) { if(serializablePropertyContext == null) { throw new ArgumentNullException("serializablePropertyContext"); } String attributeValue = String.Empty; attributeValue = GetValueOfAttributeAsString(serializablePropertyContext); if ( (attributeValue != null) && (attributeValue.Length > 0) ) { // // Emit name="value" attribute // XmlWriter.WriteAttributeString(serializablePropertyContext.Name, attributeValue); } } ////// Converts the Value of the Attribute to a String by calling into /// the appropriate type converters. /// /// /// The property that is to be serialized as an attribute at this time. /// internal String GetValueOfAttributeAsString( SerializablePropertyContext serializablePropertyContext ) { if(serializablePropertyContext == null) { throw new ArgumentNullException("serializablePropertyContext"); } String valueAsString = null; Object targetObjectContainingProperty = serializablePropertyContext.TargetObject; Object propertyValue = serializablePropertyContext.Value; if(propertyValue != null) { TypeConverter typeConverter = serializablePropertyContext.TypeConverter; valueAsString = typeConverter.ConvertToInvariantString(new XpsTokenContext(SerializationManager, serializablePropertyContext), propertyValue); if (typeof(Type).IsInstanceOfType(propertyValue)) { int index = valueAsString.LastIndexOf('.'); if (index > 0) { valueAsString = valueAsString.Substring(index + 1); } valueAsString = XpsSerializationManager.TypeOfString + valueAsString + "}"; } } else { valueAsString = XpsSerializationManager.NullString; } return valueAsString; } #endregion Internal Methods #region Public Properties ////// Queries / Set the XmlWriter for a FixedDocument /// public override XmlWriter XmlWriter { get { if(base.XmlWriter == null) { base.XmlWriter = SerializationManager.AcquireXmlWriter(typeof(FixedDocument)); } return base.XmlWriter; } set { base.XmlWriter = null; SerializationManager.ReleaseXmlWriter(typeof(FixedDocument)); } } #endregion Public Properties }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
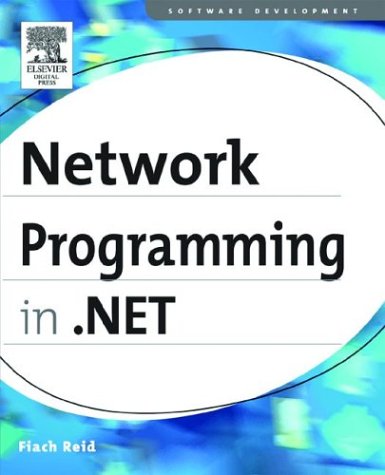
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IsolatedStorageFilePermission.cs
- AnonymousIdentificationSection.cs
- SqlProfileProvider.cs
- HeaderCollection.cs
- ConfigXmlText.cs
- BaseParaClient.cs
- StringFormat.cs
- TraceHandler.cs
- RequestUriProcessor.cs
- UxThemeWrapper.cs
- SafeWaitHandle.cs
- TraceSource.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- ReverseInheritProperty.cs
- AssemblyAssociatedContentFileAttribute.cs
- SymbolTable.cs
- ByteAnimationUsingKeyFrames.cs
- XmlCharCheckingReader.cs
- ISAPIApplicationHost.cs
- Parameter.cs
- ISFClipboardData.cs
- RepeaterItem.cs
- DbParameterHelper.cs
- BitmapEffectDrawing.cs
- UnsafeNativeMethods.cs
- COM2Properties.cs
- PersonalizationEntry.cs
- GacUtil.cs
- COM2PropertyDescriptor.cs
- FocusTracker.cs
- InputScope.cs
- StatusBarPanel.cs
- MarkedHighlightComponent.cs
- EmptyEnumerator.cs
- UnsafeNetInfoNativeMethods.cs
- TypeDescriptorFilterService.cs
- _SslSessionsCache.cs
- TraceContextRecord.cs
- FactoryGenerator.cs
- BlobPersonalizationState.cs
- VisualTreeHelper.cs
- XmlKeywords.cs
- XmlElementCollection.cs
- DataSourceControlBuilder.cs
- DataGridViewToolTip.cs
- TrackBar.cs
- CollectionView.cs
- EqualityComparer.cs
- RangeExpression.cs
- Transform.cs
- XPathNavigatorReader.cs
- Policy.cs
- ISO2022Encoding.cs
- SqlRecordBuffer.cs
- Journaling.cs
- XmlSchemaExporter.cs
- CheckedPointers.cs
- AssemblyCache.cs
- ValidationErrorCollection.cs
- SortAction.cs
- ContextStack.cs
- FileStream.cs
- EncoderBestFitFallback.cs
- XmlSchemaAll.cs
- RegionIterator.cs
- SafeMemoryMappedFileHandle.cs
- XhtmlBasicObjectListAdapter.cs
- DtrList.cs
- GPRECTF.cs
- GraphicsContainer.cs
- OdbcConnectionHandle.cs
- CatalogZone.cs
- _Events.cs
- CacheOutputQuery.cs
- LinearGradientBrush.cs
- LookupNode.cs
- DBConcurrencyException.cs
- PreProcessInputEventArgs.cs
- ExtensionDataReader.cs
- DescendantBaseQuery.cs
- ValidationError.cs
- Duration.cs
- RegexInterpreter.cs
- BooleanConverter.cs
- SerializationInfoEnumerator.cs
- CharacterString.cs
- WindowsFormsSectionHandler.cs
- Operators.cs
- SharedStatics.cs
- BitStack.cs
- LongTypeConverter.cs
- WebUtil.cs
- WorkflowInstanceContextProvider.cs
- ScriptingRoleServiceSection.cs
- HMACSHA1.cs
- ExpressionList.cs
- ProxyBuilder.cs
- DataAccessor.cs
- RepeaterCommandEventArgs.cs
- XmlLanguage.cs