Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / Parameter.cs / 2 / Parameter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Metadata.Edm; using System.Reflection; using System.IO; using System.Globalization; namespace System.Data.EntityModel.SchemaObjectModel { ////// Summary description for StructuredProperty. /// internal class Parameter : FacetEnabledSchemaElement { #region Instance Fields private ParameterDirection _parameterDirection = ParameterDirection.Input; private CollectionKind _collectionKind = CollectionKind.None; #endregion #region constructor ////// /// /// internal Parameter(Function parentElement) : base(parentElement) { _typeUsageBuilder = new TypeUsageBuilder(this); } #endregion #region Public Properties public ParameterDirection ParameterDirection { get { return _parameterDirection; } } public CollectionKind CollectionKind { get { return _collectionKind; } internal set { _collectionKind = value; } } #endregion internal override SchemaElement Clone(SchemaElement parentElement) { Parameter parameter = new Parameter((Function)parentElement); parameter._collectionKind = _collectionKind; parameter._parameterDirection = _parameterDirection; parameter._type = _type; parameter.Name = this.Name; parameter._typeUsageBuilder = this._typeUsageBuilder; return parameter; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.TypeElement)) { HandleTypeAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.Mode)) { HandleModeAttribute(reader); return true; } else if (_typeUsageBuilder.HandleAttribute(reader)) { return true; } return false; } #region Private Methods private void HandleTypeAttribute(XmlReader reader) { Debug.Assert(reader != null); Debug.Assert(UnresolvedType == null); string type; if (!Utils.GetString(Schema, reader, out type)) return; switch (Function.RemoveTypeModifier(ref type)) { case TypeModifier.Array: CollectionKind = CollectionKind.Bag; break; case TypeModifier.None: break; default: AddError(ErrorCode.BadType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.BadTypeModifier(FQName, reader.Value)); break; } if (!Utils.ValidateDottedName(Schema, reader, type)) return; UnresolvedType = type; } private void HandleModeAttribute(XmlReader reader) { Debug.Assert(reader != null); string value = reader.Value; if (String.IsNullOrEmpty(value)) { return; } value = value.Trim(); if (!String.IsNullOrEmpty(value)) { switch (value) { case XmlConstants.In: _parameterDirection = ParameterDirection.Input; break; case XmlConstants.Out: _parameterDirection = ParameterDirection.Output; break; case XmlConstants.InOut: _parameterDirection = ParameterDirection.InputOutput; break; default: { // don't try to identify the parameter by any of the attributes // because we are still parsing attributes, and we don't know which ones // have been parsed yet. int index = ParentElement.Parameters.Count; AddError(ErrorCode.BadParameterDirection, EdmSchemaErrorSeverity.Error, reader, System.Data.Entity.Strings.BadParameterDirection( value, index, this.ParentElement.Name, this.ParentElement.ParentElement.FQName)); } break; } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Metadata.Edm; using System.Reflection; using System.IO; using System.Globalization; namespace System.Data.EntityModel.SchemaObjectModel { ////// Summary description for StructuredProperty. /// internal class Parameter : FacetEnabledSchemaElement { #region Instance Fields private ParameterDirection _parameterDirection = ParameterDirection.Input; private CollectionKind _collectionKind = CollectionKind.None; #endregion #region constructor ////// /// /// internal Parameter(Function parentElement) : base(parentElement) { _typeUsageBuilder = new TypeUsageBuilder(this); } #endregion #region Public Properties public ParameterDirection ParameterDirection { get { return _parameterDirection; } } public CollectionKind CollectionKind { get { return _collectionKind; } internal set { _collectionKind = value; } } #endregion internal override SchemaElement Clone(SchemaElement parentElement) { Parameter parameter = new Parameter((Function)parentElement); parameter._collectionKind = _collectionKind; parameter._parameterDirection = _parameterDirection; parameter._type = _type; parameter.Name = this.Name; parameter._typeUsageBuilder = this._typeUsageBuilder; return parameter; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.TypeElement)) { HandleTypeAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.Mode)) { HandleModeAttribute(reader); return true; } else if (_typeUsageBuilder.HandleAttribute(reader)) { return true; } return false; } #region Private Methods private void HandleTypeAttribute(XmlReader reader) { Debug.Assert(reader != null); Debug.Assert(UnresolvedType == null); string type; if (!Utils.GetString(Schema, reader, out type)) return; switch (Function.RemoveTypeModifier(ref type)) { case TypeModifier.Array: CollectionKind = CollectionKind.Bag; break; case TypeModifier.None: break; default: AddError(ErrorCode.BadType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.BadTypeModifier(FQName, reader.Value)); break; } if (!Utils.ValidateDottedName(Schema, reader, type)) return; UnresolvedType = type; } private void HandleModeAttribute(XmlReader reader) { Debug.Assert(reader != null); string value = reader.Value; if (String.IsNullOrEmpty(value)) { return; } value = value.Trim(); if (!String.IsNullOrEmpty(value)) { switch (value) { case XmlConstants.In: _parameterDirection = ParameterDirection.Input; break; case XmlConstants.Out: _parameterDirection = ParameterDirection.Output; break; case XmlConstants.InOut: _parameterDirection = ParameterDirection.InputOutput; break; default: { // don't try to identify the parameter by any of the attributes // because we are still parsing attributes, and we don't know which ones // have been parsed yet. int index = ParentElement.Parameters.Count; AddError(ErrorCode.BadParameterDirection, EdmSchemaErrorSeverity.Error, reader, System.Data.Entity.Strings.BadParameterDirection( value, index, this.ParentElement.Name, this.ParentElement.ParentElement.FQName)); } break; } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
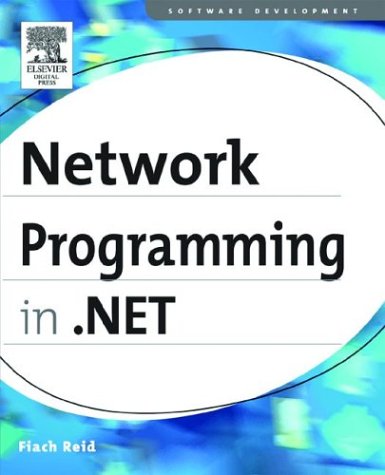
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MessageAction.cs
- ShaderEffect.cs
- CodePageUtils.cs
- StandardTransformFactory.cs
- RTLAwareMessageBox.cs
- TextRange.cs
- WebHostedComPlusServiceHost.cs
- AffineTransform3D.cs
- smtpconnection.cs
- ConfigXmlWhitespace.cs
- ObjectPersistData.cs
- DetailsViewInsertedEventArgs.cs
- TryLoadRunnableWorkflowCommand.cs
- IList.cs
- ToolStripTextBox.cs
- ReachPrintTicketSerializer.cs
- SqlOuterApplyReducer.cs
- KeySplineConverter.cs
- DependencyObjectValidator.cs
- MatrixStack.cs
- CompleteWizardStep.cs
- ScriptingProfileServiceSection.cs
- Scheduling.cs
- __ConsoleStream.cs
- GlyphInfoList.cs
- mediaeventargs.cs
- EntityCommandDefinition.cs
- HitTestResult.cs
- RegexStringValidatorAttribute.cs
- FixedDocumentSequencePaginator.cs
- TextCompositionManager.cs
- XmlDataSourceNodeDescriptor.cs
- RadioButton.cs
- FilterableAttribute.cs
- XmlSchemaInferenceException.cs
- UIAgentMonitorHandle.cs
- translator.cs
- OuterGlowBitmapEffect.cs
- FieldMetadata.cs
- SyndicationDeserializer.cs
- OleCmdHelper.cs
- ImplicitInputBrush.cs
- DateTime.cs
- ReferentialConstraintRoleElement.cs
- AutomationEventArgs.cs
- SmiEventSink_DeferedProcessing.cs
- WorkflowViewService.cs
- WebPartDescriptionCollection.cs
- StringPropertyBuilder.cs
- Utils.cs
- ContentValidator.cs
- _OverlappedAsyncResult.cs
- HttpCapabilitiesSectionHandler.cs
- GradientBrush.cs
- MatrixTransform.cs
- XmlDictionaryWriter.cs
- ProvidePropertyAttribute.cs
- HostSecurityManager.cs
- StylusPointPropertyInfo.cs
- Assembly.cs
- DataColumnCollection.cs
- ObjectListCommandCollection.cs
- PropertyInfoSet.cs
- ColorTransform.cs
- SendKeys.cs
- DrawingGroupDrawingContext.cs
- Window.cs
- Contracts.cs
- ListViewInsertEventArgs.cs
- InvalidDataException.cs
- DataControlButton.cs
- HashHelper.cs
- SpnegoTokenProvider.cs
- _AcceptOverlappedAsyncResult.cs
- TreeNodeEventArgs.cs
- ECDiffieHellmanCng.cs
- CatalogPart.cs
- SQLRoleProvider.cs
- CaseStatementSlot.cs
- Latin1Encoding.cs
- CapabilitiesPattern.cs
- AmbientEnvironment.cs
- X509SecurityToken.cs
- FrameworkElementAutomationPeer.cs
- LoginCancelEventArgs.cs
- WmiEventSink.cs
- Interlocked.cs
- ObjRef.cs
- Html32TextWriter.cs
- MetadataHelper.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- OperandQuery.cs
- Margins.cs
- DataBoundControlAdapter.cs
- AQNBuilder.cs
- ToolStripScrollButton.cs
- BaseHashHelper.cs
- DerivedKeySecurityToken.cs
- AppliedDeviceFiltersEditor.cs
- MsmqIntegrationMessageProperty.cs