Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / UIAutomation / UIAutomationClient / MS / Internal / Automation / WindowInteractionStateTracker.cs / 1 / WindowInteractionStateTracker.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Class used to track new UI appearing and make sure any events // are propogated to that new UI. // // History: // 01/05/2005 : Created // //--------------------------------------------------------------------------- using System; using System.Globalization; using System.Text; using System.Windows.Automation; using System.Diagnostics; using MS.Win32; namespace MS.Internal.Automation { // Class used to track new UI appearing and make sure any events // are propogated to that new UI. internal class WindowInteractionStateTracker : WinEventWrap { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal WindowInteractionStateTracker() : base(new int[] { NativeMethods.EVENT_OBJECT_STATECHANGE }) { // Intentionally not setting the callback for the base WinEventWrap since the WinEventProc override // in this class calls RaiseEventInThisClientOnly to actually raise the event to the client. } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal override void WinEventProc(int eventId, IntPtr hwnd, int idObject, int idChild, uint eventTime) { // ignore any event not pertaining directly to the window if (idObject != UnsafeNativeMethods.OBJID_WINDOW) { return; } // Ignore if this is a bogus hwnd (shouldn't happen) if (hwnd == IntPtr.Zero) { return; } OnStateChange(hwnd, idObject, idChild); } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods private void OnStateChange(IntPtr hwnd, int idObject, int idChild) { NativeMethods.HWND nativeHwnd = NativeMethods.HWND.Cast(hwnd); // Ignore windows that have been destroyed if (!SafeNativeMethods.IsWindow(nativeHwnd)) { return; } AutomationElement rawEl = AutomationElement.FromHandle(hwnd); try { rawEl.GetCurrentPattern(WindowPattern.Pattern); } catch (InvalidOperationException) { // Only raise this event for elements with the WindowPattern. return; } Object windowInteractionState = rawEl.GetPatternPropertyValue(WindowPattern.WindowInteractionStateProperty, false); // if has no state value just return if (!(windowInteractionState is WindowInteractionState)) { return; } WindowInteractionState state = (WindowInteractionState)windowInteractionState; // Filter... avoid duplicate events if (hwnd == _lastHwnd && state == _lastState) { return; } AutomationPropertyChangedEventArgs e = new AutomationPropertyChangedEventArgs( WindowPattern.WindowInteractionStateProperty, hwnd == _lastHwnd ? _lastState : WindowInteractionState.Running, state); ClientEventManager.RaiseEventInThisClientOnly(AutomationElement.AutomationPropertyChangedEvent, rawEl, e); // save the last hwnd/rect for filtering out duplicates _lastHwnd = hwnd; _lastState = state; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private WindowInteractionState _lastState; // keep track of last interaction state private IntPtr _lastHwnd; // and hwnd for dup checking #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Class used to track new UI appearing and make sure any events // are propogated to that new UI. // // History: // 01/05/2005 : Created // //--------------------------------------------------------------------------- using System; using System.Globalization; using System.Text; using System.Windows.Automation; using System.Diagnostics; using MS.Win32; namespace MS.Internal.Automation { // Class used to track new UI appearing and make sure any events // are propogated to that new UI. internal class WindowInteractionStateTracker : WinEventWrap { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal WindowInteractionStateTracker() : base(new int[] { NativeMethods.EVENT_OBJECT_STATECHANGE }) { // Intentionally not setting the callback for the base WinEventWrap since the WinEventProc override // in this class calls RaiseEventInThisClientOnly to actually raise the event to the client. } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal override void WinEventProc(int eventId, IntPtr hwnd, int idObject, int idChild, uint eventTime) { // ignore any event not pertaining directly to the window if (idObject != UnsafeNativeMethods.OBJID_WINDOW) { return; } // Ignore if this is a bogus hwnd (shouldn't happen) if (hwnd == IntPtr.Zero) { return; } OnStateChange(hwnd, idObject, idChild); } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods private void OnStateChange(IntPtr hwnd, int idObject, int idChild) { NativeMethods.HWND nativeHwnd = NativeMethods.HWND.Cast(hwnd); // Ignore windows that have been destroyed if (!SafeNativeMethods.IsWindow(nativeHwnd)) { return; } AutomationElement rawEl = AutomationElement.FromHandle(hwnd); try { rawEl.GetCurrentPattern(WindowPattern.Pattern); } catch (InvalidOperationException) { // Only raise this event for elements with the WindowPattern. return; } Object windowInteractionState = rawEl.GetPatternPropertyValue(WindowPattern.WindowInteractionStateProperty, false); // if has no state value just return if (!(windowInteractionState is WindowInteractionState)) { return; } WindowInteractionState state = (WindowInteractionState)windowInteractionState; // Filter... avoid duplicate events if (hwnd == _lastHwnd && state == _lastState) { return; } AutomationPropertyChangedEventArgs e = new AutomationPropertyChangedEventArgs( WindowPattern.WindowInteractionStateProperty, hwnd == _lastHwnd ? _lastState : WindowInteractionState.Running, state); ClientEventManager.RaiseEventInThisClientOnly(AutomationElement.AutomationPropertyChangedEvent, rawEl, e); // save the last hwnd/rect for filtering out duplicates _lastHwnd = hwnd; _lastState = state; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private WindowInteractionState _lastState; // keep track of last interaction state private IntPtr _lastHwnd; // and hwnd for dup checking #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
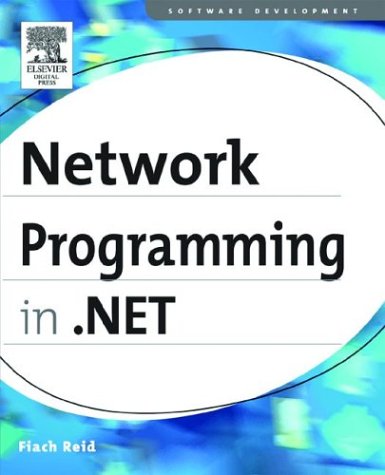
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnsafeNativeMethodsMilCoreApi.cs
- AncestorChangedEventArgs.cs
- WebRequestModulesSection.cs
- ControlTemplate.cs
- SpoolingTaskBase.cs
- IPPacketInformation.cs
- AlternateView.cs
- FieldNameLookup.cs
- DefaultPropertyAttribute.cs
- DataListCommandEventArgs.cs
- ListViewPagedDataSource.cs
- TraceListener.cs
- Descriptor.cs
- Int64AnimationUsingKeyFrames.cs
- DoubleLinkList.cs
- ScrollBarRenderer.cs
- RadioButtonList.cs
- AppDomainFactory.cs
- DesignerActionList.cs
- SourceFileInfo.cs
- XmlComplianceUtil.cs
- TagPrefixAttribute.cs
- PDBReader.cs
- AppDomainUnloadedException.cs
- SqlProfileProvider.cs
- OpenTypeCommon.cs
- TreeView.cs
- CallbackValidatorAttribute.cs
- DesignerOptionService.cs
- XmlStreamStore.cs
- ProcessInputEventArgs.cs
- Run.cs
- SqlFunctionAttribute.cs
- XmlNavigatorFilter.cs
- GridViewDeletedEventArgs.cs
- RuleSet.cs
- EnterpriseServicesHelper.cs
- CopyCodeAction.cs
- ForeignKeyConstraint.cs
- Win32SafeHandles.cs
- SqlServer2KCompatibilityAnnotation.cs
- ScaleTransform.cs
- XmlAtomicValue.cs
- PointUtil.cs
- RangeBase.cs
- DataGridViewDataErrorEventArgs.cs
- ItemsChangedEventArgs.cs
- DataGridBoolColumn.cs
- NGCPageContentCollectionSerializerAsync.cs
- ArglessEventHandlerProxy.cs
- WebSysDefaultValueAttribute.cs
- IdentifierElement.cs
- DataRecordInfo.cs
- ProxyGenerator.cs
- Calendar.cs
- DecodeHelper.cs
- AssemblyFilter.cs
- DataGridCellClipboardEventArgs.cs
- AssemblyAttributesGoHere.cs
- MultiPageTextView.cs
- DoubleLinkList.cs
- WebResourceAttribute.cs
- ExceptionUtility.cs
- HostedHttpRequestAsyncResult.cs
- PhysicalFontFamily.cs
- TimeBoundedCache.cs
- MenuItemStyle.cs
- MetadataProperty.cs
- Timeline.cs
- AutomationInteropProvider.cs
- XslNumber.cs
- ImageMap.cs
- StylusPoint.cs
- ExpanderAutomationPeer.cs
- XmlNodeChangedEventManager.cs
- XamlTemplateSerializer.cs
- SerialPinChanges.cs
- HwndHost.cs
- DaylightTime.cs
- PropertyChangeTracker.cs
- XmlSchemaValidationException.cs
- rsa.cs
- SqlDataSourceView.cs
- PropertyValueUIItem.cs
- SystemIPInterfaceProperties.cs
- QilName.cs
- DataConnectionHelper.cs
- XmlAnyElementAttributes.cs
- VirtualizingStackPanel.cs
- FaultReasonText.cs
- LoginName.cs
- ServiceContractListItemList.cs
- EventKeyword.cs
- CheckBoxFlatAdapter.cs
- FlowDocumentView.cs
- ListViewGroup.cs
- ConnectionStringsSection.cs
- EndpointDiscoveryElement.cs
- Int32CollectionValueSerializer.cs
- LinqDataSourceSelectEventArgs.cs