Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / Scheduling / SpoolingTaskBase.cs / 1305376 / SpoolingTaskBase.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // SpoolingTaskBase.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Threading; using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// A spooling task handles marshaling data from a producer to a consumer. It simply /// takes data from a producer and hands it off to a consumer. This class is the base /// class from which other concrete spooling tasks derive, encapsulating some common /// logic (such as capturing exceptions). /// internal abstract class SpoolingTaskBase : QueryTask { //------------------------------------------------------------------------------------ // Constructs a new spooling task. // // Arguments: // taskIndex - the unique index of this task // protected SpoolingTaskBase(int taskIndex, QueryTaskGroupState groupState) : base(taskIndex, groupState) { } //----------------------------------------------------------------------------------- // The implementation of the Work API just enumerates the producer's data, and // enqueues it into the consumer channel. Well, really, it just defers to extension // points (below) implemented by subclasses to do these things. // protected override void Work() { try { // Defer to the base class for the actual work. SpoolingWork(); } catch (Exception ex) { OperationCanceledException oce = ex as OperationCanceledException; if (oce != null && oce.CancellationToken == m_groupState.CancellationState.MergedCancellationToken && m_groupState.CancellationState.MergedCancellationToken.IsCancellationRequested) { //an expected internal cancellation has occurred. suppress this exception. } else { // TPL will catch and store the exception on the task object. We'll then later // turn around and wait on it, having the effect of propagating it. In the meantime, // we want to cooperative cancel all workers. m_groupState.CancellationState.InternalCancellationTokenSource.Cancel(); // And then repropagate to let TPL catch it. throw; } } finally { SpoolingFinally(); //dispose resources etc. } } //----------------------------------------------------------------------------------- // This method is responsible for enumerating results and enqueueing them to // the output channel(s) as appropriate. Each base class implements its own. // protected abstract void SpoolingWork(); //----------------------------------------------------------------------------------- // If the subclass needs to do something in the finally block of the main work loop, // it should override this and do it. Purely optional. // protected virtual void SpoolingFinally() { // (Intentionally left blank.) } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
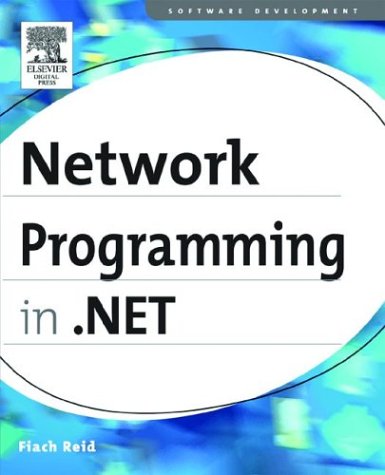
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridItemCollection.cs
- ControlBuilderAttribute.cs
- DataGridBoolColumn.cs
- PolicyLevel.cs
- EntityDataSourceContextCreatingEventArgs.cs
- DetailsViewInsertEventArgs.cs
- ExtentJoinTreeNode.cs
- HwndTarget.cs
- TypeUtil.cs
- RectangleGeometry.cs
- DBSqlParserColumn.cs
- ExpandSegmentCollection.cs
- DataGridItemCollection.cs
- SqlResolver.cs
- Comparer.cs
- WindowsRichEditRange.cs
- parserscommon.cs
- NodeFunctions.cs
- ProvidersHelper.cs
- MultipleViewPattern.cs
- XmlSchemaDatatype.cs
- TypedReference.cs
- LinqDataSourceStatusEventArgs.cs
- ChtmlTextWriter.cs
- SimpleBitVector32.cs
- _NegoState.cs
- ObjectManager.cs
- EventListenerClientSide.cs
- JobStaple.cs
- RenderData.cs
- FrameworkTextComposition.cs
- FormViewPageEventArgs.cs
- LinqDataSource.cs
- HostingPreferredMapPath.cs
- RTLAwareMessageBox.cs
- FieldToken.cs
- AnimationClockResource.cs
- ContainerControl.cs
- AsymmetricSignatureFormatter.cs
- IncrementalReadDecoders.cs
- RepeatButtonAutomationPeer.cs
- LineServicesRun.cs
- EntityStoreSchemaFilterEntry.cs
- ImageMapEventArgs.cs
- DependencyPropertyKey.cs
- DataErrorValidationRule.cs
- EncodingInfo.cs
- GeneratedView.cs
- DrawingDrawingContext.cs
- CompressedStack.cs
- ActiveXHost.cs
- OletxTransactionManager.cs
- Sequence.cs
- SystemResources.cs
- XmlTextReaderImpl.cs
- MarkedHighlightComponent.cs
- XmlComplianceUtil.cs
- QilTypeChecker.cs
- IList.cs
- StylusDownEventArgs.cs
- Transaction.cs
- WindowsGraphicsWrapper.cs
- Messages.cs
- AdvancedBindingEditor.cs
- ThicknessConverter.cs
- WindowInteropHelper.cs
- SafeArrayTypeMismatchException.cs
- SystemIdentity.cs
- PaperSource.cs
- TreeViewAutomationPeer.cs
- DynamicPropertyHolder.cs
- MethodResolver.cs
- StrokeFIndices.cs
- SoapReflectionImporter.cs
- UriSection.cs
- WebPartAddingEventArgs.cs
- remotingproxy.cs
- dtdvalidator.cs
- RuntimeWrappedException.cs
- EmptyStringExpandableObjectConverter.cs
- NavigatorOutput.cs
- MemoryStream.cs
- AcceleratedTokenProvider.cs
- SplineKeyFrames.cs
- DataGridViewSelectedRowCollection.cs
- SoapFormatterSinks.cs
- Calendar.cs
- DbTypeMap.cs
- RpcCryptoContext.cs
- ExeConfigurationFileMap.cs
- AddInController.cs
- AttributeTableBuilder.cs
- UnionExpr.cs
- SBCSCodePageEncoding.cs
- HostingPreferredMapPath.cs
- Collection.cs
- MultiPropertyDescriptorGridEntry.cs
- ValueProviderWrapper.cs
- StrokeSerializer.cs
- RegisteredScript.cs