Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / xsp / System / Web / Extensions / ui / RegisteredScript.cs / 2 / RegisteredScript.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), ] public sealed class RegisteredScript { private RegisteredScriptType _scriptType; private Control _control; private string _key; private string _script; private Type _type; private bool _addScriptTags; private string _url; internal RegisteredScript(Control control, Type type, string key, string url) { Debug.Assert(control != null); Debug.Assert(type != null); Debug.Assert(!String.IsNullOrEmpty(url)); // null and empty "key" are treated different by asp.net script duplicate detection so null is allowed. _scriptType = RegisteredScriptType.ClientScriptInclude; _control = control; _type = type; _key = key; _url = url; } internal RegisteredScript(RegisteredScriptType scriptType, Control control, Type type, string key, string script, bool addScriptTags) { Debug.Assert(control != null); Debug.Assert( scriptType != RegisteredScriptType.OnSubmitStatement || !addScriptTags, "OnSubmitStatements cannot have addScriptTags."); Debug.Assert(type != null); // null and empty "key" are treated different by asp.net script duplicate detection so null is allowed. // null script allowed _scriptType = scriptType; _control = control; _type = type; _key = key; _script = script; _addScriptTags = addScriptTags; } public bool AddScriptTags { get { return _addScriptTags; } } public Control Control { get { return _control; } } public string Key { get { // may be null return _key; } } public string Script { get { // may be null return _script; } } public RegisteredScriptType ScriptType { get { return _scriptType; } } [SuppressMessage("Microsoft.Naming", "CA1721:PropertyNamesShouldNotMatchGetMethods", Justification = "Refers to a Control, not my Object.GetType()")] public Type Type { get { return _type; } } [SuppressMessage("Microsoft.Design", "CA1056:UriPropertiesShouldNotBeStrings", Justification = "Consistent with RegisterClientScriptInclude.")] public string Url { get { // null if this is not a client script include or resource return _url; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), ] public sealed class RegisteredScript { private RegisteredScriptType _scriptType; private Control _control; private string _key; private string _script; private Type _type; private bool _addScriptTags; private string _url; internal RegisteredScript(Control control, Type type, string key, string url) { Debug.Assert(control != null); Debug.Assert(type != null); Debug.Assert(!String.IsNullOrEmpty(url)); // null and empty "key" are treated different by asp.net script duplicate detection so null is allowed. _scriptType = RegisteredScriptType.ClientScriptInclude; _control = control; _type = type; _key = key; _url = url; } internal RegisteredScript(RegisteredScriptType scriptType, Control control, Type type, string key, string script, bool addScriptTags) { Debug.Assert(control != null); Debug.Assert( scriptType != RegisteredScriptType.OnSubmitStatement || !addScriptTags, "OnSubmitStatements cannot have addScriptTags."); Debug.Assert(type != null); // null and empty "key" are treated different by asp.net script duplicate detection so null is allowed. // null script allowed _scriptType = scriptType; _control = control; _type = type; _key = key; _script = script; _addScriptTags = addScriptTags; } public bool AddScriptTags { get { return _addScriptTags; } } public Control Control { get { return _control; } } public string Key { get { // may be null return _key; } } public string Script { get { // may be null return _script; } } public RegisteredScriptType ScriptType { get { return _scriptType; } } [SuppressMessage("Microsoft.Naming", "CA1721:PropertyNamesShouldNotMatchGetMethods", Justification = "Refers to a Control, not my Object.GetType()")] public Type Type { get { return _type; } } [SuppressMessage("Microsoft.Design", "CA1056:UriPropertiesShouldNotBeStrings", Justification = "Consistent with RegisterClientScriptInclude.")] public string Url { get { // null if this is not a client script include or resource return _url; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
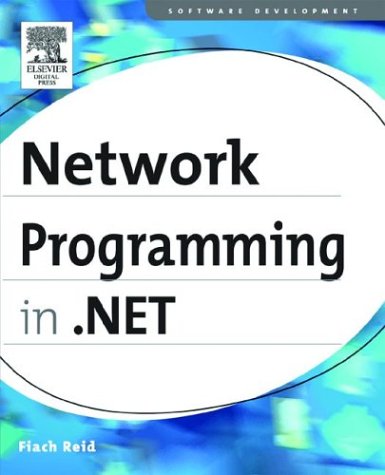
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLConvert.cs
- ObjectDataSourceFilteringEventArgs.cs
- CallbackHandler.cs
- XmlHierarchicalDataSourceView.cs
- PeerNameRecordCollection.cs
- RowUpdatingEventArgs.cs
- Buffer.cs
- Mutex.cs
- PolicyLevel.cs
- ExpressionBuilderCollection.cs
- VSWCFServiceContractGenerator.cs
- HtmlControl.cs
- XmlSigningNodeWriter.cs
- DiscoveryReference.cs
- XmlSchemaImporter.cs
- FontWeightConverter.cs
- CommonXSendMessage.cs
- GAC.cs
- TextEditorTables.cs
- SerializationFieldInfo.cs
- entityreference_tresulttype.cs
- DictionaryChange.cs
- MeasurementDCInfo.cs
- DragEventArgs.cs
- FontInfo.cs
- DataSourceSelectArguments.cs
- LineInfo.cs
- ClaimTypeRequirement.cs
- TraceContextEventArgs.cs
- DynamicDataResources.Designer.cs
- Int16KeyFrameCollection.cs
- PageMediaType.cs
- FamilyMapCollection.cs
- SectionXmlInfo.cs
- EventProviderClassic.cs
- Point3DAnimationUsingKeyFrames.cs
- InteropExecutor.cs
- RangeValueProviderWrapper.cs
- CallbackException.cs
- KeyValueConfigurationCollection.cs
- QilTernary.cs
- LongMinMaxAggregationOperator.cs
- DataServiceContext.cs
- SchemaType.cs
- SchemaCollectionCompiler.cs
- StylusLogic.cs
- SqlTriggerContext.cs
- ComponentManagerBroker.cs
- JsonStringDataContract.cs
- BrowserTree.cs
- AccessibilityHelperForVista.cs
- PropertyDescriptors.cs
- String.cs
- SettingsProviderCollection.cs
- MetadataArtifactLoader.cs
- CancellationHandlerDesigner.cs
- BuildProvidersCompiler.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- CompositeActivityTypeDescriptor.cs
- MarginCollapsingState.cs
- BindingMemberInfo.cs
- LinearQuaternionKeyFrame.cs
- Decoder.cs
- NumericUpDownAccelerationCollection.cs
- HyperLink.cs
- HostingEnvironmentSection.cs
- BatchParser.cs
- HeaderUtility.cs
- ZoneIdentityPermission.cs
- ConstructorNeedsTagAttribute.cs
- MessageQueueInstaller.cs
- ReflectionUtil.cs
- Divide.cs
- CommonProperties.cs
- ObjectDataSourceMethodEventArgs.cs
- StandardBindingImporter.cs
- DataSourceControl.cs
- MD5Cng.cs
- DbModificationCommandTree.cs
- FontDriver.cs
- XmlWriterDelegator.cs
- WindowPattern.cs
- SpnegoTokenAuthenticator.cs
- DataGridViewRowHeaderCell.cs
- Group.cs
- ICspAsymmetricAlgorithm.cs
- XmlSchemas.cs
- AutoScrollExpandMessageFilter.cs
- UIElementParaClient.cs
- Canvas.cs
- DataDocumentXPathNavigator.cs
- ToolZoneDesigner.cs
- ProgressBar.cs
- XamlSerializer.cs
- AnimatedTypeHelpers.cs
- PathSegment.cs
- CompareValidator.cs
- NCryptNative.cs
- TransformCollection.cs
- IntranetCredentialPolicy.cs